如何在 JavaScript 中為 Canvas 元素新增 onClick 事件處理程序?
如何將onClick 事件附加到Canvas 元素
簡介:
簡介:Canvas 元素提供了一種在網頁上繪製和操作圖形的強大方法。然而,向畫布元素添加事件處理程序對於初學者來說可能很棘手。本文將示範如何為畫布元素添加簡單的 onClick 事件處理程序。
問題:經驗豐富的 Java 開發人員在向畫布元素添加 onClick 事件處理程序時遇到困難JavaScript 中的畫布元素。多次嘗試均失敗,包括使用 onclick 屬性、分配函數以及將屬性設為字串。
解:在 canvas 元素上繪圖時,元素本身除了像素和顏色之外沒有任何表示。因此,要偵測元素的點擊,需要捕捉canvas HTML元素上的點擊事件,並使用數學計算來確定哪個元素被點擊。 要為canvas元素新增點選事件,請使用下列程式碼語法:要確定按一下的元素,請使用下列程式碼語法:
要確定按一下的元素,請使用下列指令代碼:
說明:
- 此程式碼將按一下事件附加到畫布元素,並將單一元素新增至元素陣列。程式碼循環遍歷元素,將點擊座標與元素座標進行比較,並在元素上發生點擊時發出警報。
- 嘗試失敗的原因:
以上是如何在 JavaScript 中為 Canvas 元素新增 onClick 事件處理程序?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
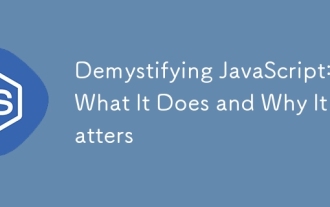
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
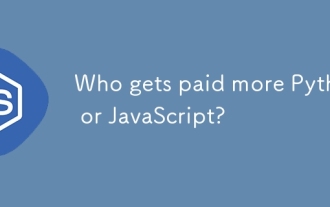
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
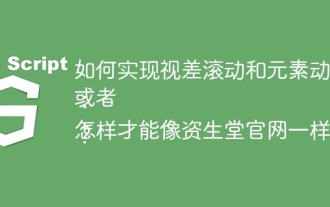
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
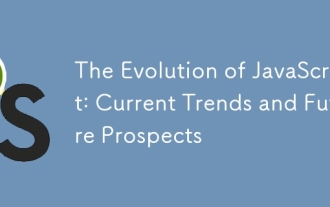
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
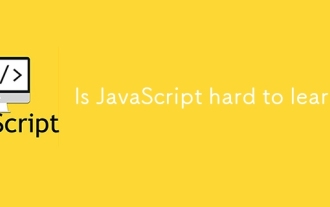
學習JavaScript不難,但有挑戰。 1)理解基礎概念如變量、數據類型、函數等。 2)掌握異步編程,通過事件循環實現。 3)使用DOM操作和Promise處理異步請求。 4)避免常見錯誤,使用調試技巧。 5)優化性能,遵循最佳實踐。
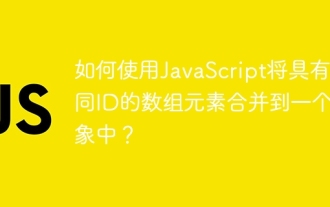
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
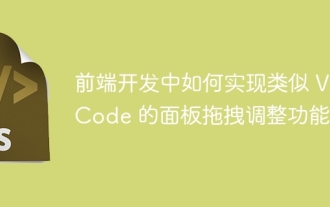
探索前端中類似VSCode的面板拖拽調整功能的實現在前端開發中,如何實現類似於VSCode...
