如何將'std::map”與浮點鍵一起使用:比較不準確的解決方案?
std::map 浮點鍵比較
使用浮點值作為std::map 中的鍵可能會帶來挑戰,因為浮點運算固有的不精確性。一個常見問題是,使用嚴格相等 (==) 比較浮點鍵可能不會總是按預期工作,因為即使看似精確的值也可能由於精度錯誤而不匹配。
在給定的程式碼範例中,循環嘗試在std::map 中尋找鍵3.0,但失敗,因為循環使用= 0.1 遞增搜尋鍵,由於浮點精度不準確,這可能與預期鍵值不精確匹配。
要解決此問題,您可以在程式中使用 std::set precision 函數來指定比較鍵時要考慮的小數位數。但是,這種方法仍然不可靠,因為它不能保證鍵能夠精確比較。
更好的解決方案是在 std::map 中使用近似比較函數。您可以定義一個自訂比較器,使用 epsilon 閾值來確定鍵相等性。這允許您在一定容差內比較鍵,有效地忽略微小的精度差異:
struct fuzzy_double_comparator { bool operator() (const double a, const double b) const { return std::fabs(a - b) < epsilon; } };
然後可以將此比較器函數傳遞給std::map 構造函數以使用近似比較:
std::map<double, double, fuzzy_double_comparator> mymap;
透過這種方法,您可以在std::map 中找到鍵3.0,即使它的實際值由於浮點精度限製而略有不同。
以上是如何將'std::map”與浮點鍵一起使用:比較不準確的解決方案?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
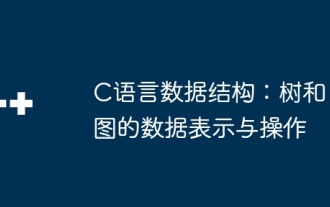
C語言數據結構:樹和圖的數據表示與操作樹是一個層次結構的數據結構由節點組成,每個節點包含一個數據元素和指向其子節點的指針二叉樹是一種特殊類型的樹,其中每個節點最多有兩個子節點數據表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作創建樹遍歷樹(先序、中序、後序)搜索樹插入節點刪除節點圖是一個集合的數據結構,其中的元素是頂點,它們通過邊連接在一起邊可以是帶權或無權的數據表示鄰
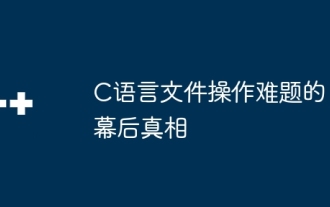
文件操作難題的真相:文件打開失敗:權限不足、路徑錯誤、文件被佔用。數據寫入失敗:緩衝區已滿、文件不可寫、磁盤空間不足。其他常見問題:文件遍歷緩慢、文本文件編碼不正確、二進製文件讀取錯誤。
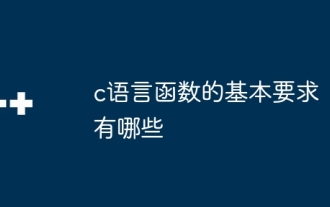
C語言函數是代碼模塊化和程序搭建的基礎。它們由聲明(函數頭)和定義(函數體)組成。 C語言默認使用值傳遞參數,但也可使用地址傳遞修改外部變量。函數可以有返回值或無返回值,返回值類型必須與聲明一致。函數命名應清晰易懂,使用駝峰或下劃線命名法。遵循單一職責原則,保持函數簡潔性,以提高可維護性和可讀性。
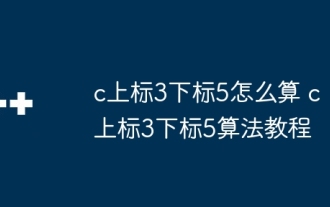
C35 的計算本質上是組合數學,代表從 5 個元素中選擇 3 個的組合數,其計算公式為 C53 = 5! / (3! * 2!),可通過循環避免直接計算階乘以提高效率和避免溢出。另外,理解組合的本質和掌握高效的計算方法對於解決概率統計、密碼學、算法設計等領域的許多問題至關重要。
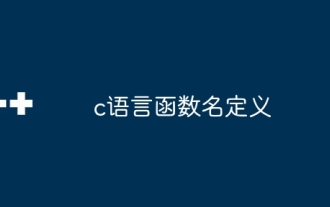
C語言函數名定義包括:返回值類型、函數名、參數列表和函數體。函數名應清晰、簡潔、統一風格,避免與關鍵字衝突。函數名具有作用域,可在聲明後使用。函數指針允許將函數作為參數傳遞或賦值。常見錯誤包括命名衝突、參數類型不匹配和未聲明的函數。性能優化重點在函數設計和實現上,而清晰、易讀的代碼至關重要。
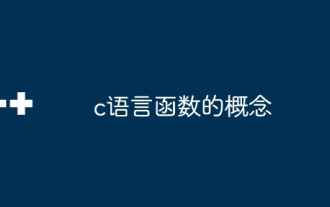
C語言函數是可重複利用的代碼塊,它接收輸入,執行操作,返回結果,可將代碼模塊化提高可複用性,降低複雜度。函數內部機制包含參數傳遞、函數執行、返回值,整個過程涉及優化如函數內聯。編寫好的函數遵循單一職責原則、參數數量少、命名規範、錯誤處理。指針與函數結合能實現更強大的功能,如修改外部變量值。函數指針將函數作為參數傳遞或存儲地址,用於實現動態調用函數。理解函數特性和技巧是編寫高效、可維護、易理解的C語言程序的關鍵。
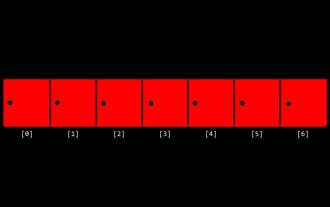
算法是解決問題的指令集,其執行速度和內存佔用各不相同。編程中,許多算法都基於數據搜索和排序。本文將介紹幾種數據檢索和排序算法。線性搜索假設有一個數組[20,500,10,5,100,1,50],需要查找數字50。線性搜索算法會逐個檢查數組中的每個元素,直到找到目標值或遍歷完整個數組。算法流程圖如下:線性搜索的偽代碼如下:檢查每個元素:如果找到目標值:返回true返回falseC語言實現:#include#includeintmain(void){i
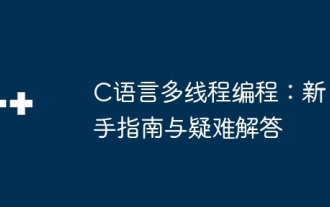
C語言多線程編程指南:創建線程:使用pthread_create()函數,指定線程ID、屬性和線程函數。線程同步:通過互斥鎖、信號量和條件變量防止數據競爭。實戰案例:使用多線程計算斐波那契數,將任務分配給多個線程並同步結果。疑難解答:解決程序崩潰、線程停止響應和性能瓶頸等問題。
