如何在 React.js 中將子 Props 傳遞給父級?
在React.js 中將子元件傳遞給父元件
Context
React 透過父元件和子元件之間提供了強大的通信機制。但是,在某些情況下,您可能想要將整個子元件及其 props 傳遞給父元件。當您想要將複雜的邏輯封裝在子級中並向父級提供更簡單的介面時,這非常有用。
關於子級到父級Prop 傳遞的擔憂
歷史上,一些開發人員建議使用事件將子props 傳遞給父級,但這種方法引起了對耦合和封裝的擔憂。直接在父元件中存取子元件的 props 和實作細節可能會使元件緊密耦合並阻礙重構工作。
建議方法
相反,建議利用現有的父 prop 機制來存取孩子的資料。這使得父級能夠利用它提供給子級的 props,從而避免需要從子級本身中檢索子級的 props。
使用類組件實現
子組件:
class Child extends Component { render() { return <button onClick={this.props.onClick}>{this.props.text}</button>; } }
家長組件:
class Parent extends Component { getInitialState() { return { childText: "Click me! (parent prop)" }; } render() { return ( <Child onClick={this.handleChildClick} text={this.state.childText} /> ); } handleChildClick(event) { const childText = this.state.childText; // Parent has access to child prop alert(`The Child button text is: ${childText}`); // Parent has access to event target alert(`The Child HTML is: ${event.target.outerHTML}`); } }
使用功能組件實現
子組件:
const Child = ({ onClick, text }) => { return <button onClick={onClick}>{text}</button>; };
父組件組件:
const Parent = ({ childrenData }) => { const handleChildClick = (childData, event) => { const childText = childData.childText; const childNumber = childData.childNumber; // Parent has access to child prop alert(`The Child button data is: ${childText} - ${childNumber}`); // Parent has access to event target alert(`The Child HTML is: ${event.target.outerHTML}`); }; return ( <div> {childrenData.map((childData) => ( <Child key={childData.childNumber} onClick={(event) => handleChildClick(childData, event)} text={childData.childText} /> ))} </div> ); };
父組件組件:
父組件組件:父組件組件:透過擁抱React中現有的prop機制,您可以有效地在父子元件之間傳遞數據,而不會引入不必要的耦合或違反封裝原則。這種方法確保組件保持獨立和靈活,從而實現更清晰和更可維護的程式碼。以上是如何在 React.js 中將子 Props 傳遞給父級?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
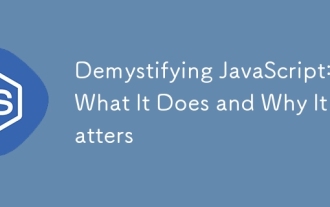
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
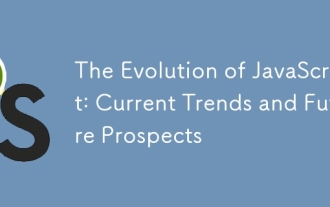
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
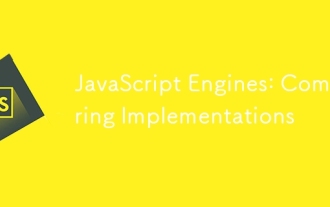
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
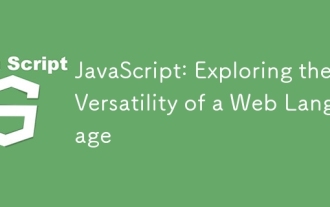
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
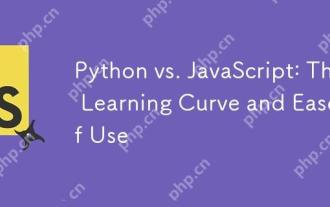
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
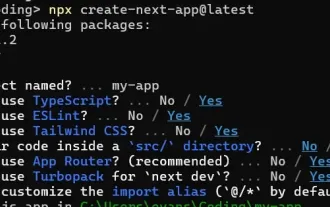
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
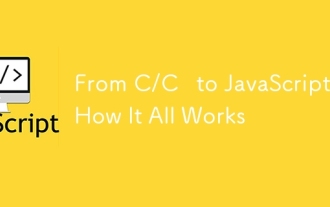
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
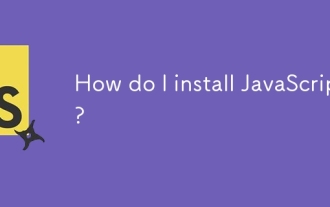
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。
