為角鬥士之戰建立動態新聞頁面:主要功能和實現
在開發《角斗士之戰》的新聞頁面時,我們創建了一個功能豐富的交互式部分,允許用戶探索最新更新、管理個性化閱讀列表並輕鬆搜索各種內容文章。讓我們深入了解該頁面的主要組件、它們的獨特功能以及它們如何增強用戶體驗。
關鍵組件與功能
- NewsSection:新聞文章集中展示
NewsSection 元件是新聞頁面的核心,負責以有組織、可存取的格式收集和呈現所有新聞文章。該組件可作為整合所有其他功能(例如搜尋、過濾和閱讀清單功能)的中心,為使用者提供無縫的互動體驗。
主要特性與功能:
動態資料載入
Firebase 整合:NewsSection 從 Firebase 取得新聞文章,確保頁面在新增至資料庫後立即更新為最新內容。
即時更新:Firebase 的即時功能可確保任何新文章或更新立即出現,無需刷新頁面,從而保持用戶的參與度和最新狀態。
響應式網格佈局
基於網格的設計:新聞文章以響應式網格佈局排列,可根據螢幕尺寸進行調整。此佈局旨在實現最佳可讀性,無論用戶使用桌上型電腦、平板電腦或行動裝置。
自適應樣式:使用 CSS Flexbox 和媒體查詢,新聞版塊可以適應各種設備分辨率,從而在跨平台上提供一致且愉快的體驗。
類別過濾
組件內過濾:NewsSection 整合了類別過濾功能,使用戶可以根據特定類別(例如開發部落格、歷史、遊戲)縮小顯示的文章範圍。每個類別都可以透過下拉式選單或按鈕介面進行選擇,為使用者提供尋找相關內容的直接方式。
即時更新:選擇類別後,顯示的文章會立即過濾,僅提供使用者感興趣的內容,從而增強使用者體驗。
搜尋與 NewsSearch 整合
搜尋和顯示協調:NewsSection 與 NewsSearch 元件協調,根據使用者的搜尋輸入動態顯示文章。這種無縫整合意味著當使用者鍵入時,顯示的文章會即時過濾,而無需重新載入頁面。
使用者體驗最佳化:透過僅顯示與使用者查詢相關的文章,NewsSection 使用戶可以輕鬆有效地找到特定內容,從而提高滿意度和參與度。
與新聞卡整合個別文章
單篇文章顯示:NewsSection 中的每篇文章都由 NewsCard 組件表示,該組件顯示基本信息,包括文章標題、預覽文本、發布日期和類別。
視覺一致性:新聞卡的標準化樣式確保了文章之間的視覺一致性,使新聞版塊美觀且易於導航。
可點擊的卡片:每個新聞卡都是可點擊的,引導使用者進入完整的文章頁面。這種直覺的互動增強了可訪問性,使用戶能夠輕鬆存取內容。
個人化閱讀清單整合
新增至閱讀清單功能:NewsSection 與 ReadingListButton 元件集成,讓使用者直接從動態消息將文章新增至其個人化閱讀清單。用戶稍後可以從閱讀清單中存取已儲存的文章,從而促進參與和重新訪問。
回饋機制:當一篇文章被加入閱讀清單時,使用者會收到視覺回饋(例如按鈕顏色的變化或圖示的更新),以確認操作。這種回饋有助於加強參與度並增強使用者體驗。
熱門類別整合
熱門主題顯示:NewsSection 具有由 PopularCategories 組件提供支援的「熱門類別」部分。本部分重點介紹基於使用者互動和文章瀏覽的趨勢類別,鼓勵探索熱門主題。
快速類別過濾:點擊熱門類別中的類別即可立即過濾顯示的文章,簡化導航並幫助用戶發現高興趣的內容。
視覺增強與使用者友善的設計
一致的設計語言:NewsSection 遵循《角鬥士之戰》的整體設計語言,融合了與網站角鬥士風格的美學相一致的主題、顏色和字體。這種設計選擇增強了沉浸式體驗並強化了品牌形象。
懸停效果和動畫:每個新聞卡和按鈕上的微妙懸停效果增添了一絲互動性,使頁面感覺現代且引人入勝。這些視覺提示引導使用者瀏覽內容並提供更動態的瀏覽體驗。
範例程式碼片段:取得和顯示文章
以下是如何從 Firebase 取得文章並在 NewsSection 元件中顯示的簡化範例。
import React, { useState, useEffect } from 'react'; import { db } from '../firebase-config'; import { collection, onSnapshot } from 'firebase/firestore'; import NewsCard from './NewsCard'; const NewsSection = () => { const [articles, setArticles] = useState([]); useEffect(() => { const unsubscribe = onSnapshot(collection(db, 'articles'), (snapshot) => { const fetchedArticles = snapshot.docs.map((doc) => ({ id: doc.id, ...doc.data(), })); setArticles(fetchedArticles); }); return () => unsubscribe(); }, []); return ( <div className="news-section"> {articles.map((article) => ( <NewsCard key={article.id} article={article} /> ))} </div> ); }; export default NewsSection;
對使用者體驗與參與度的影響
NewsSection 元件是使用者探索、參與和傳回 Gladiators Battle 新聞內容的主要接觸點。透過提供具有凝聚力、易於導航和視覺吸引力的佈局以及個人化功能,NewsSection 增強了用戶保留率並創建了一個吸引人的內容發現平台。它與 Firebase 的整合可確保內容保持新鮮且可即時訪問,從而打造符合現代網路標準和用戶期望的無縫體驗。
- 新聞卡:互動文章卡
新聞卡元件對於以引人入勝且易於導航的方式顯示每篇文章的基本資訊至關重要。其設計的重點是使每篇文章在視覺上有吸引力,同時在整個新聞頁面中保持一致的風格。
主要特性與功能
基本資訊顯示
標題和類別:每張新聞卡都會突出顯示文章標題以吸引註意力,旁邊有類別標籤,讓用戶立即了解文章的內容類型。
簡要描述:為了向使用者提供快速概述,包含簡短描述或預覽文本,幫助使用者決定是否要閱讀更多內容。
發布日期:顯示日期新增上下文,幫助使用者區分新文章和舊內容。
基於類別的動態樣式
基於類別的樣式:新聞卡根據文章類別應用獨特的樣式,例如“開發部落格”、“歷史”或“遊戲”,使用戶可以輕鬆區分內容類型。
一致的品牌:透過保持一致的設計語言,每張卡片都強化了角鬥士之戰網站的整體美感,創造了與角鬥士主題相一致的有凝聚力的外觀。
顏色編碼:特定的配色方案或強調色應用於不同的類別,使用戶在瀏覽時可以輕鬆快速識別文章類型。
互動式與響應式設計
懸停和點擊效果:每個新聞卡都包含微妙的懸停效果(例如輕微的陰影或放大),以表明該卡是可點擊的。這種互動有助於引導使用者直觀地瀏覽內容。
可點擊重定向:點擊新聞卡可將使用者直接帶到完整的文章頁面,提供從摘要內容到詳細內容的平滑過渡。
行動最佳化:新聞卡旨在適應各種螢幕尺寸。對於行動用戶,佈局可調整以保持可讀性,而觸控友善的元素可確保流暢的導航。
增強用戶參與度
稍後閱讀選項:每個新聞卡都可以包含一個由 ReadingListButton 元件支援的「新增至閱讀清單」按鈕。此功能可讓使用者保存文章以供日後使用,從而營造更個人化和引人入勝的瀏覽體驗。
操作即時回饋:當使用者將文章新增至閱讀清單時,新聞卡可以直觀地更新以顯示其已儲存,從而提供清晰的回饋並增強互動性。
效能最佳化
圖像的延遲加載:為了提高頁面效能,每個新聞卡中的圖像都會延遲加載,這意味著它們僅在即將進入視口時才加載。這減少了初始頁面載入時間,特別是在包含大量文章的頁面上。
高效渲染:每個新聞卡都旨在僅渲染必要的數據,防止不必要的重新渲染,從而增強頁面的整體響應能力。
輔助功能
鍵盤導航:新聞卡可透過鍵盤導航訪問,使依賴鍵盤或螢幕閱讀器的用戶能夠與內容無縫互動。
ARIA 標籤:NewsCard 中的每個互動元素都標有 ARIA 屬性,確保螢幕閱讀器可以準確地將訊息傳達給視障用戶。
範例程式碼片段:動態類別樣式
以下是如何在 NewsCard 元件中套用動態類別樣式的範例。此程式碼示範如何使用條件樣式為每個類別賦予獨特的外觀。
import React, { useState, useEffect } from 'react'; import { db } from '../firebase-config'; import { collection, onSnapshot } from 'firebase/firestore'; import NewsCard from './NewsCard'; const NewsSection = () => { const [articles, setArticles] = useState([]); useEffect(() => { const unsubscribe = onSnapshot(collection(db, 'articles'), (snapshot) => { const fetchedArticles = snapshot.docs.map((doc) => ({ id: doc.id, ...doc.data(), })); setArticles(fetchedArticles); }); return () => unsubscribe(); }, []); return ( <div className="news-section"> {articles.map((article) => ( <NewsCard key={article.id} article={article} /> ))} </div> ); }; export default NewsSection;
在此範例中:
categories 屬性包含趨勢類別清單。
每個類別按鈕在點擊時都會呼叫 onCategorySelect 函數,然後觸發 NewsSection 元件中的篩選。
熱門類別對使用者體驗的好處
高效的內容瀏覽:透過將用戶引導到高興趣的主題,熱門類別可以幫助用戶快速找到他們可能喜歡的內容,減少瀏覽時間並提高用戶滿意度。
提高網站參與度:突出顯示趨勢類別鼓勵用戶探索更多內容,可能會帶來更高的互動率和重複訪問。
即時回應:透過根據用戶活動自動更新,熱門類別保持相關性並適應用戶興趣的變化,有助於保持內容新鮮並符合受眾需求。
PopularCategories 元件是一個強大的功能,用於指導角鬥士之戰網站上的使用者互動。透過促進對熱門內容的快速訪問,它增強了導航,使用戶參與熱門主題,並提供了一種有效的方式來探索平台上可用的各種主題。
- NewsSearch:即時搜尋功能
NewsSearch 元件提供強大、用戶友好的搜尋體驗,旨在幫助用戶快速找到 Gladiators Battle 新聞頁面上的文章和內容。它提供即時、響應式搜索,在用戶鍵入時顯示結果,確保無縫體驗,讓用戶無需重新加載頁面即可保持參與。
主要特性與功能
高效率的搜尋機制
即時搜尋結果:NewsSearch 元件會在使用者輸入時即時更新搜尋結果,利用 React 的狀態管理來即時篩選文章。此功能可透過減少等待時間並提供即時回饋來增強使用者體驗。
與 NewsSection 平滑整合:NewsSearch 與 NewsSection 元件緊密整合,以便在現有頁面結構中過濾結果。用戶可以直接在新聞版塊中查看過濾後的文章,使搜尋體驗直觀且視覺上一致。
響應式設計與輔助功能
React Bootstrap 樣式:NewsSearch 使用 React Bootstrap 構建,提供精美、專業的外觀以及與角鬥士之戰網站的整體主題相匹配的一致樣式。 Bootstrap 的網格和元件系統確保了反應能力,使搜尋欄能夠平滑地適應桌面和行動佈局。
圖示增強:搜尋列中使用圖示以提高視覺清晰度。例如,放大鏡圖標表示搜尋功能,一旦使用者開始輸入,搜尋欄位中就會顯示清除或重置圖標,表示他們可以刪除搜尋查詢。
清晰的按鈕增強可用性
快速存取清除按鈕:使用者只需使用清除按鈕點擊一次即可隨時重置搜尋查詢。當存在活動搜尋查詢時,此按鈕將有條件地顯示,幫助使用者重置搜尋並返回完整的文章列表,而無需重新載入頁面。
鍵盤可訪問性:清除按鈕可透過鍵盤訪問,允許依賴鍵盤導航的使用者輕鬆清除搜尋欄位。對可訪問性的關注確保了該功能對所有用戶都有用,無論他們如何與網站互動。
效能最佳化
去抖動輸入處理:為了防止不必要的重新渲染或過多的API 調用,搜尋輸入是去搖晃的,這意味著它僅在用戶停止輸入時在短暫延遲(例如300 毫秒)後觸發搜索。這可確保平穩的效能並減少客戶端和伺服器的壓力。
最小狀態更新:透過使用 React 的受控元件和最小狀態更新,NewsSearch 可以保持效能最佳化,即使使用者輸入並清除多個搜尋查詢也是如此。
增強使用者體驗
即時回饋:當使用者鍵入時,結果會立即顯示,提供即時回饋並創建流暢、引人入勝的搜尋體驗。
突出顯示搜尋查詢:在某些實作中,搜尋結果可以突出顯示匹配的關鍵字,使用戶可以輕鬆快速地發現相關內容。這有助於用戶在視覺上將他們的搜尋查詢與結果聯繫起來。
範例程式碼片段:建立即時搜尋列
以下是一個基本範例,示範如何使用 React 和 Bootstrap 來實作 NewsSearch 元件。此程式碼展示了即時搜尋更新和清除按鈕功能。
import React, { useState, useEffect } from 'react'; import { db } from '../firebase-config'; import { collection, onSnapshot } from 'firebase/firestore'; import NewsCard from './NewsCard'; const NewsSection = () => { const [articles, setArticles] = useState([]); useEffect(() => { const unsubscribe = onSnapshot(collection(db, 'articles'), (snapshot) => { const fetchedArticles = snapshot.docs.map((doc) => ({ id: doc.id, ...doc.data(), })); setArticles(fetchedArticles); }); return () => unsubscribe(); }, []); return ( <div className="news-section"> {articles.map((article) => ( <NewsCard key={article.id} article={article} /> ))} </div> ); }; export default NewsSection;
在此範例中:
onSearch 屬性是一個向下傳遞的函數,用於處理父元件中的搜尋查詢,通常會過濾 NewsSection 元件中顯示的文章。
react-icons 中的 FaSearch 和 FaTimes 圖示提供了搜尋和清除輸入的視覺提示。
clearSearch功能會重設查詢狀態和搜尋結果,方便使用者開始新的搜尋或返回瀏覽所有文章。
NewsSearch 對使用者體驗的好處
增強的內容可發現性:即時搜尋功能可協助使用者快速找到感興趣的文章,減少摩擦並提高在網站上保留使用者註意力的機會。
提高可訪問性:透過響應式設計、鍵盤可存取性和清晰的視覺指示器,NewsSearch 元件可確保各種能力的使用者都能有效地導航並利用搜尋功能。
提高站點效能:透過使用去抖動和最小狀態更新等技術,即使在頻繁使用的情況下,元件也能保持平穩的效能。
NewsSearch 元件顯著增強了 Gladiators Battle 新聞頁面的使用者體驗,為使用者提供了快速、高效且易於存取的內容定位方式。其直覺的設計與效能優化相結合,確保用戶享受無縫且響應迅速的搜尋體驗。
- 閱讀清單:個人化文章管理
ReadingList 元件是一項獨特的、以使用者為中心的功能,允許訪客保存文章以供將來閱讀。此功能創造了更個人化的體驗,非常適合希望追蹤相關新聞並在方便時返回的用戶。透過與 Firebase 集成,閱讀清單可確保用戶可以跨多個裝置無縫存取其保存的文章,使其成為參與 Gladiators Battle 網站的強大工具。
主要特性與功能
個人化文章管理
儲存以供以後使用:使用者只需單擊即可將文章新增至他們的閱讀清單中,從而使他們能夠管理自己的內容集合。此功能對於可能沒有時間立即閱讀完整文章但想稍後再返回的用戶特別有用。
有組織的、可存取的內容:閱讀清單以有組織的格式顯示已儲存的文章,允許使用者查看每篇文章的摘要,包括標題、類別和簡短描述。這種佈局可以輕鬆找到特定文章並鼓勵用戶閱讀更多內容。
用於持久性儲存的 Firebase 整合
特定於使用者的清單:透過與 Firebase 集成,每個使用者的閱讀清單都安全地儲存在雲端。當使用者登入其帳戶時,將從 Firebase 取得其個人化閱讀列表,確保可以跨會話和裝置存取已儲存的文章。
跨裝置同步:Firebase 允許使用者從不同裝置查看和管理他們的閱讀清單。例如,用戶可以在桌面上保存一篇文章,稍後在智慧型手機上存取它,從而創建無縫的跨平台體驗。
即時更新:Firebase 的即時資料庫功能可讓閱讀清單在使用者新增或刪除文章時立即更新,從而提供動態體驗,無需重新載入頁面。
動態、使用者友善的顯示
已儲存文章的卡片版面:儲存在閱讀清單中的文章以美觀的卡片格式顯示,顯示文章標題、簡短說明和類別等關鍵資訊。每張卡片都包含一個「立即閱讀」按鈕,可讓使用者快速存取全文。
清晰的視覺回饋:如果閱讀清單為空,則會顯示一則訊息,讓使用者知道他們尚未儲存任何文章。此功能提供了清晰的視覺提示,並溫和地鼓勵用戶開始將文章添加到他們的清單中。
增強的使用者互動
易於使用的儲存和刪除選項:此介麵包括用於新增和刪除文章的按鈕,使用戶可以控制其保存的內容。如果用戶不再感興趣,可以輕鬆地將每篇文章從清單中刪除,從而提供整潔且用戶友好的體驗。
通知和回饋機制:為了提高使用者體驗,可以實現簡短的通知來確認文章何時成功添加到閱讀清單或從閱讀清單中刪除。這種回饋強化了使用者操作並有助於確保流暢的互動。
輔助功能與響應式設計
行動裝置友善的佈局:ReadingList 元件設計為完全響應式,在桌面和行動裝置上提供最佳體驗。卡片佈局可調整以適應不同的螢幕尺寸,使保存的文章易於在任何裝置上閱讀和導航。
鍵盤輔助功能:每個保存和刪除按鈕都可以透過鍵盤訪問,確保所有用戶,包括依賴鍵盤導航的用戶,都可以輕鬆管理他們的閱讀清單。
範例程式碼片段:使用 Firebase 管理已儲存的文章
以下程式碼示範如何使用 Firebase 儲存和擷取已儲存的文章,將文章新增至 ReadingList 元件並在其中顯示。
import React, { useState, useEffect } from 'react'; import { db } from '../firebase-config'; import { collection, onSnapshot } from 'firebase/firestore'; import NewsCard from './NewsCard'; const NewsSection = () => { const [articles, setArticles] = useState([]); useEffect(() => { const unsubscribe = onSnapshot(collection(db, 'articles'), (snapshot) => { const fetchedArticles = snapshot.docs.map((doc) => ({ id: doc.id, ...doc.data(), })); setArticles(fetchedArticles); }); return () => unsubscribe(); }, []); return ( <div className="news-section"> {articles.map((article) => ( <NewsCard key={article.id} article={article} /> ))} </div> ); }; export default NewsSection;
在此範例中:
fetchReadingList 函數在元件安裝時會從 Firebase 檢索已儲存的文章。
addToReadingList 和removeFromReadingList 函數允許使用者在閱讀清單中新增或刪除文章。
閱讀清單動態顯示,如果沒有儲存文章,則會顯示一則訊息。
ReadingList 元件對使用者體驗的好處
個人化內容管理:使用者可以保存文章以供日後使用,從而創造出更客製化的體驗,鼓勵與網站的定期互動。
提高用戶參與度:保存文章的功能可以激勵用戶返回網站,從而可能增加用戶在網站上花費的時間並提高整體參與度。
跨平台便利性:Firebase 整合可確保使用者可以從任何裝置存取其已儲存的文章,從而促進跨平台的無縫體驗。
ReadingList 元件為 Gladiators Battle 網站添加了強大的、用戶友好的功能,使用戶可以在方便時輕鬆管理和重新訪問內容。透過增強個人化、支援與 Firebase 即時同步以及提供響應式設計,閱讀清單在促進用戶參與度和為每位訪客打造量身定制的體驗方面發揮著關鍵作用。
- 閱讀清單按鈕:儲存以供稍後使用
ReadingListButton 元件在每個文章卡上新增了一個「稍後閱讀」按鈕,讓使用者只需單擊即可將文章新增至其閱讀清單。
Firebase 更新:當使用者將文章新增至其閱讀清單時,該動作將儲存在 Firebase 中,確保使用者每次重新登入時都可以存取該清單。
回饋機制:此按鈕提供即時視覺回饋,確認文章已成功新增。
程式碼與實作細節
以下是這些功能的一些關鍵程式碼片段的詳細介紹。
在閱讀清單中新增和顯示文章
ReadingList 元件使用 Firebase 來管理每個使用者的閱讀清單。當使用者點擊「立即閱讀」時,該文章將從其閱讀清單中刪除,從而為管理已儲存的文章提供了流暢的流程。
import React from 'react'; import { Link } from 'react-router-dom'; import './NewsCard.css'; const NewsCard = ({ article }) => { const categoryStyles = { Devblog: { borderColor: '#ff6347', color: '#ff6347' }, History: { borderColor: '#8b4513', color: '#8b4513' }, Games: { borderColor: '#4682b4', color: '#4682b4' }, // Add more categories as needed }; return ( <Link to={`/news/${article.id}`} className="news-card"> <p>Benefits of NewsCard for User Experience </p> <p>Consistent and Easy Navigation: The uniformity of the NewsCards, coupled with the visual distinctions for each category, makes the page easy to navigate and visually appealing. </p> <p>Increased Engagement: Interactive features like hover effects and the “Read Later” option encourage users to interact with content, enhancing engagement and return visits. </p> <p>Performance and Accessibility: Through lazy loading and ARIA compliance, NewsCard ensures an accessible and smooth experience for all users, even those on slower connections or with accessibility needs. </p> <p>The NewsCard component serves as the visual entry point to each article, combining style, interactivity, and performance in a way that enhances user engagement and reinforces the brand identity of Gladiators Battle. </p> <ol> <li>PopularCategories: Highlighting Trending Topics </li> </ol> <p>The PopularCategories component is designed to improve user engagement by prominently displaying trending topics on the site. By directing users to popular sections, it encourages exploration and helps users quickly access the most relevant content. The component works seamlessly with other elements like NewsSection, filtering articles based on selected categories. </p> <p>Key Features and Functionalities </p> <p>Highlighting High-Interest Topics </p> <p>Dynamic Trend Detection: PopularCategories pulls data on trending categories based on user interactions, such as the most viewed or frequently clicked categories. This dynamic trend detection ensures that users are directed to content that is currently popular and relevant. </p> <p>Automatic Updates: As user interests shift, PopularCategories automatically updates the displayed categories, reflecting real-time trends without requiring manual input. </p> <p>Interactive Filtering Mechanism </p> <p>Click-to-Filter Functionality: Each category in PopularCategories acts as a filter, allowing users to click on a category to instantly display relevant articles. This quick access to specific content is particularly useful for users who want to browse by interest, such as “Devblog,” “History,” or “Games.” </p> <p>Smooth Integration with NewsSection: Once a category is clicked, PopularCategories seamlessly filters the articles displayed in NewsSection. This real-time, integrated filtering improves the user experience by immediately showing only the relevant content without needing a page reload. </p> <p>Enhanced User Navigation </p> <p>Quick Content Discovery: By emphasizing trending categories, PopularCategories encourages users to explore different topics, facilitating quick discovery of high-interest articles and improving overall site engagement. </p> <p>Accessible Design: Each category is displayed as a clickable button or tag, visually distinguishable and easy to interact with. The layout is designed to be both desktop and mobile-friendly, ensuring accessibility across devices. </p> <p>Visual Consistency and Branding </p> <p>Thematic Styling: Each category button is styled to match the site’s gladiator theme, often incorporating colors and icons that reflect the aesthetic of Gladiators Battle. This thematic consistency reinforces brand identity and makes the browsing experience more immersive. </p> <p>Hover and Click Animations: Subtle hover and click animations give each category button an interactive feel, providing feedback when users interact with categories and enhancing the overall design polish of the page. </p> <p>Scalability for Future Topics </p> <p>Flexible Category Management: PopularCategories is built to handle any number of trending categories. As new content categories are added to the site, this component can dynamically include them, ensuring scalability and flexibility as the site grows. </p> <p>Customizable Display Logic: The component allows customization for how categories are ranked or highlighted, whether based on user engagement metrics, specific promotional goals, or seasonal content. </p> <p>Example Code Snippet: Displaying and Filtering with PopularCategories </p> <p>Here’s a simple example showing how PopularCategories might display trending topics and handle user clicks to filter content.<br> </p> <pre class="brush:php;toolbar:false">import React from 'react'; const PopularCategories = ({ categories, onCategorySelect }) => { return ( <div className="popular-categories"> <h3>Trending Categories</h3> <div className="category-list"> {categories.map((category) => ( <button key={category.id} className="category-button" onClick={() => onCategorySelect(category.name)} > {category.name} </button> ))} </div> </div> ); }; export default PopularCategories;
新聞搜尋中的即時搜尋
NewsSearch 元件包含即時搜尋功能,可依使用者輸入更新顯示的文章。這種方法可確保動態體驗,無需使用者離開頁面或重新整理內容。
import React, { useState, useEffect } from 'react'; import { db } from '../firebase-config'; import { collection, onSnapshot } from 'firebase/firestore'; import NewsCard from './NewsCard'; const NewsSection = () => { const [articles, setArticles] = useState([]); useEffect(() => { const unsubscribe = onSnapshot(collection(db, 'articles'), (snapshot) => { const fetchedArticles = snapshot.docs.map((doc) => ({ id: doc.id, ...doc.data(), })); setArticles(fetchedArticles); }); return () => unsubscribe(); }, []); return ( <div className="news-section"> {articles.map((article) => ( <NewsCard key={article.id} article={article} /> ))} </div> ); }; export default NewsSection;
快速過濾熱門類別
PopularCategories 元件可讓使用者點擊類別並立即按該標籤過濾新聞內容,從而增強了導航功能。此過濾在元件內動態管理,提高可用性並幫助使用者更快找到特定內容。
import React from 'react'; import { Link } from 'react-router-dom'; import './NewsCard.css'; const NewsCard = ({ article }) => { const categoryStyles = { Devblog: { borderColor: '#ff6347', color: '#ff6347' }, History: { borderColor: '#8b4513', color: '#8b4513' }, Games: { borderColor: '#4682b4', color: '#4682b4' }, // Add more categories as needed }; return ( <Link to={`/news/${article.id}`} className="news-card"> <p>Benefits of NewsCard for User Experience </p> <p>Consistent and Easy Navigation: The uniformity of the NewsCards, coupled with the visual distinctions for each category, makes the page easy to navigate and visually appealing. </p> <p>Increased Engagement: Interactive features like hover effects and the “Read Later” option encourage users to interact with content, enhancing engagement and return visits. </p> <p>Performance and Accessibility: Through lazy loading and ARIA compliance, NewsCard ensures an accessible and smooth experience for all users, even those on slower connections or with accessibility needs. </p> <p>The NewsCard component serves as the visual entry point to each article, combining style, interactivity, and performance in a way that enhances user engagement and reinforces the brand identity of Gladiators Battle. </p> <ol> <li>PopularCategories: Highlighting Trending Topics </li> </ol> <p>The PopularCategories component is designed to improve user engagement by prominently displaying trending topics on the site. By directing users to popular sections, it encourages exploration and helps users quickly access the most relevant content. The component works seamlessly with other elements like NewsSection, filtering articles based on selected categories. </p> <p>Key Features and Functionalities </p> <p>Highlighting High-Interest Topics </p> <p>Dynamic Trend Detection: PopularCategories pulls data on trending categories based on user interactions, such as the most viewed or frequently clicked categories. This dynamic trend detection ensures that users are directed to content that is currently popular and relevant. </p> <p>Automatic Updates: As user interests shift, PopularCategories automatically updates the displayed categories, reflecting real-time trends without requiring manual input. </p> <p>Interactive Filtering Mechanism </p> <p>Click-to-Filter Functionality: Each category in PopularCategories acts as a filter, allowing users to click on a category to instantly display relevant articles. This quick access to specific content is particularly useful for users who want to browse by interest, such as “Devblog,” “History,” or “Games.” </p> <p>Smooth Integration with NewsSection: Once a category is clicked, PopularCategories seamlessly filters the articles displayed in NewsSection. This real-time, integrated filtering improves the user experience by immediately showing only the relevant content without needing a page reload. </p> <p>Enhanced User Navigation </p> <p>Quick Content Discovery: By emphasizing trending categories, PopularCategories encourages users to explore different topics, facilitating quick discovery of high-interest articles and improving overall site engagement. </p> <p>Accessible Design: Each category is displayed as a clickable button or tag, visually distinguishable and easy to interact with. The layout is designed to be both desktop and mobile-friendly, ensuring accessibility across devices. </p> <p>Visual Consistency and Branding </p> <p>Thematic Styling: Each category button is styled to match the site’s gladiator theme, often incorporating colors and icons that reflect the aesthetic of Gladiators Battle. This thematic consistency reinforces brand identity and makes the browsing experience more immersive. </p> <p>Hover and Click Animations: Subtle hover and click animations give each category button an interactive feel, providing feedback when users interact with categories and enhancing the overall design polish of the page. </p> <p>Scalability for Future Topics </p> <p>Flexible Category Management: PopularCategories is built to handle any number of trending categories. As new content categories are added to the site, this component can dynamically include them, ensuring scalability and flexibility as the site grows. </p> <p>Customizable Display Logic: The component allows customization for how categories are ranked or highlighted, whether based on user engagement metrics, specific promotional goals, or seasonal content. </p> <p>Example Code Snippet: Displaying and Filtering with PopularCategories </p> <p>Here’s a simple example showing how PopularCategories might display trending topics and handle user clicks to filter content.<br> </p> <pre class="brush:php;toolbar:false">import React from 'react'; const PopularCategories = ({ categories, onCategorySelect }) => { return ( <div className="popular-categories"> <h3>Trending Categories</h3> <div className="category-list"> {categories.map((category) => ( <button key={category.id} className="category-button" onClick={() => onCategorySelect(category.name)} > {category.name} </button> ))} </div> </div> ); }; export default PopularCategories;
好處與使用者體驗增強
個人化與參與度
閱讀清單和熱門類別功能為用戶提供了量身定制的體驗,讓他們保持參與。透過儲存文章並輕鬆存取熱門主題,使用者更有可能與內容互動並返回頁面。
高效搜尋和過濾
即時搜尋和基於類別的過濾確保用戶可以快速找到相關文章,減少用戶體驗中的摩擦。這些元素使新聞頁面變得用戶友好,即使對於首次訪客也是如此。
即時數據更新
利用 Firebase 可以實現整個頁面的即時更新,例如立即反映使用者閱讀清單中的變更。這提供了一種響應靈敏且現代的無縫體驗。
結論
《角鬥士之戰》的新聞頁面體現了我們致力於透過精心設計的互動式組件提供引人入勝、用戶友好的體驗的承諾。從個人化的閱讀清單到新聞搜尋和熱門類別功能,我們創建了一個動態的響應式頁面,鼓勵用戶探索適合其興趣的內容。該頁面證明了了解用戶需求並結合強大的互動式元素以促進更深入的參與的重要性。
無論您是探索最新的角鬥士故事、通過單擊查找熱門話題,還是保存文章供日後使用,我們的新聞頁面的每項功能都經過精心設計,旨在增強用戶體驗,讓角斗士之戰栩栩如生。
?探索完整的新聞部分:https://gladiatorsbattle.com/all-news-gladiators
如果您受到我們的旅程的啟發並希望保持聯繫,請透過我們的管道關注我們以加入社區並查看更多令人興奮的更新:
網址:了解更多關於《角鬥士之戰》和我們身臨其境、受角鬥士啟發的遊戲的資訊:https://gladiatorsbattle.com
GitHub:深入研究我們的程式碼庫並為我們的專案做出貢獻:https://github.com/HanGPIErr
LinkedIn:與我們聯繫,以了解《角鬥士之戰》和我們的開發之旅的最新動態:https://www.linkedin.com/in/pierre-romain-lopez/
Twitter (X):追蹤《角鬥士之戰》,獲取新聞、更新,並一窺我們的角鬥士主題世界:https://x.com/GladiatorsBT
透過跟隨我們的旅程,您將深入了解內容豐富的互動式網頁的開發,並了解如何建立動態使用者體驗。加入我們,我們將繼續將歷史與技術融合,打造令人難忘的線上冒險。
以上是為角鬥士之戰建立動態新聞頁面:主要功能和實現的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
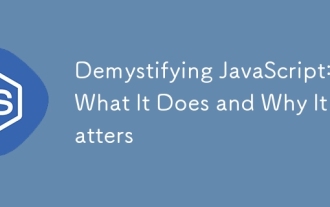
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
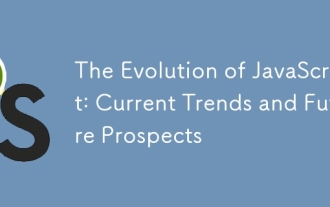
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
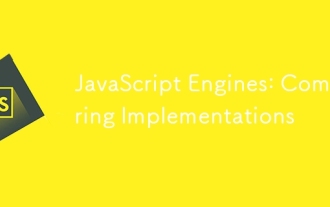
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
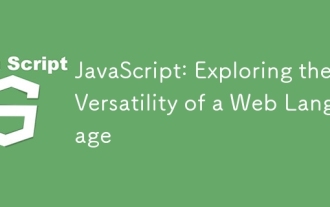
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
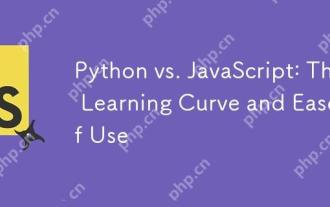
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
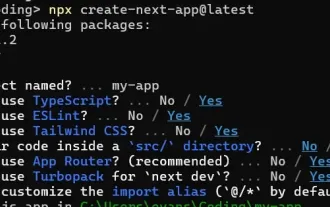
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
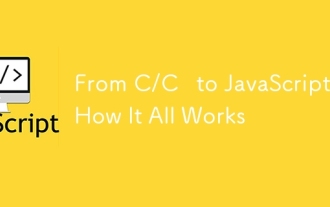
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
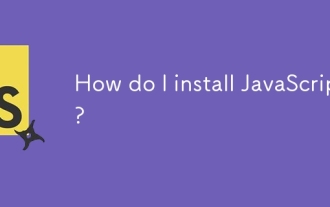
JavaScript不需要安裝,因為它已內置於現代瀏覽器中。你只需文本編輯器和瀏覽器即可開始使用。 1)在瀏覽器環境中,通過標籤嵌入HTML文件中運行。 2)在Node.js環境中,下載並安裝Node.js後,通過命令行運行JavaScript文件。
