考慮循環數組和切片,如何在 Go 中高效實現隊列?
在 Go 中實作佇列
佇列,一種重要的資料結構,常出現在程式設計場景中。然而,Go 庫缺乏內建的佇列功能。本文探討了一種利用循環數組作為底層資料結構的實現方法,遵循開創性著作「電腦程式設計的藝術」中概述的演算法。
初始實作
最初的實作使用了一個簡單的循環數組,追蹤隊列的頭部(刪除點)和尾部(插入點)位置。然而,它還是不夠,正如輸出中所反映的那樣:出隊操作未能正確刪除超出佇列初始容量的元素。
改進的實現
改進的版本透過引入布林變數來驗證尾部是否可以前進來解決這個問題。這保證了尾部只有在有空間的情況下才能移動,防止佇列溢出。產生的程式碼準確地模擬了隊列行為。
使用切片的替代方法
Go 的切片機制提供了另一種實現隊列的方法。佇列可以表示為元素切片,並具有用於入隊和出隊操作的常規切片追加和刪除。此方法消除了對顯式佇列資料結構的需求。
效能注意事項
雖然切片方法消除了維護自包含佇列資料結構的開銷,但它確實有一個警告。追加到切片偶爾會觸發重新分配,這在時間緊迫的情況下可能會成為問題。
示例
以下代碼片段演示了兩種實現:
package main import ( "fmt" "time" ) // Queue implementation using a circular array type Queue struct { head, tail int array []int } func (q *Queue) Enqueue(x int) bool { // Check if queue is full if (q.tail+1)%len(q.array) == q.head { return false } // Add element to the tail of the queue q.array[q.tail] = x q.tail = (q.tail + 1) % len(q.array) return true } func (q *Queue) Dequeue() (int, bool) { // Check if queue is empty if q.head == q.tail { return 0, false } // Remove element from the head of the queue x := q.array[q.head] q.head = (q.head + 1) % len(q.array) return x, true } // Queue implementation using slices type QueueSlice []int func (q *QueueSlice) Enqueue(x int) { *q = append(*q, x) } func (q *QueueSlice) Dequeue() (int, bool) { if len(*q) == 0 { return 0, false } x := (*q)[0] *q = (*q)[1:] return x, true } func main() { // Performance comparison between the two queue implementations loopCount := 10000000 fmt.Println("Queue using circular array:") q1 := &Queue{array: make([]int, loopCount)} start := time.Now() for i := 0; i < loopCount; i++ { q1.Enqueue(i) } for i := 0; i < loopCount; i++ { q1.Dequeue() } elapsed := time.Since(start) fmt.Println(elapsed) fmt.Println("\nQueue using slices:") q2 := &QueueSlice{} start = time.Now() for i := 0; i < loopCount; i++ { q2.Enqueue(i) } for i := 0; i < loopCount; i++ { q2.Dequeue() } elapsed = time.Since(start) fmt.Println(elapsed) }
結論
兩者隊列實作有其自身的優點和缺點。基於循環數組的佇列在時間敏感的場景中提供更好的效能,而基於片的佇列更簡單並且消除了分配。方法的選擇取決於應用程式的特定要求。
以上是考慮循環數組和切片,如何在 Go 中高效實現隊列?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
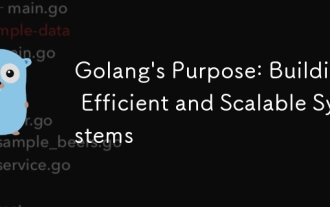
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
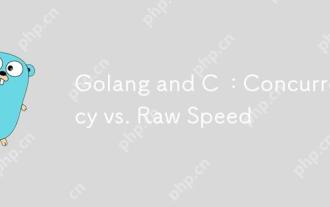
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
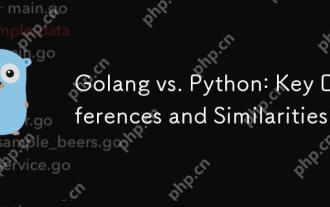
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。Golang以其并发模型和高效性能著称,Python则以简洁语法和丰富库生态系统著称。
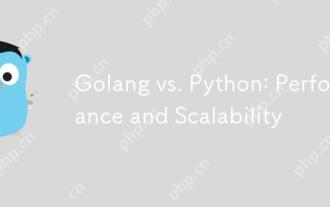
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
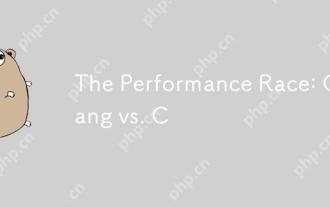
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
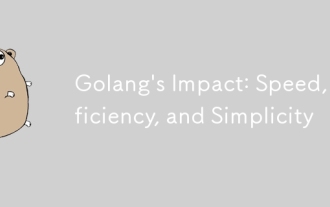
goimpactsdevelopmentpositationality throughspeed,效率和模擬性。 1)速度:gocompilesquicklyandrunseff,IdealforlargeProjects.2)效率:效率:ITScomprehenSevestAndardArdardArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdArdEcceSteral Depentencies,增強的Depleflovelmentimency.3)簡單性。
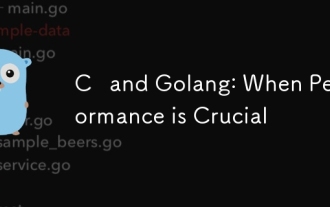
C 更適合需要直接控制硬件資源和高性能優化的場景,而Golang更適合需要快速開發和高並發處理的場景。 1.C 的優勢在於其接近硬件的特性和高度的優化能力,適合遊戲開發等高性能需求。 2.Golang的優勢在於其簡潔的語法和天然的並發支持,適合高並發服務開發。
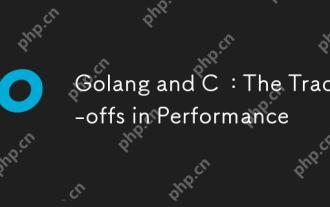
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
