Java中如何將文件讀入ArrayList?
在Java 中將文件讀入ArrayList
在處理文字資料時,將其儲存在資料結構中通常很有用,以方便使用操縱和處理。在 Java 中,ArrayList 是常用的集合,用於保存物件列表,包括字串。本文示範如何將檔案的內容讀取到字串的 ArrayList 中。
文件準備
首先,請確保您有一個包含行分隔資料的文字文件,如提供的範例所示:
cat house dog ...
讀取的Java程式碼檔案
要將檔案的內容讀入字串ArrayList,請使用以下程式碼:
import java.util.ArrayList; import java.util.Scanner; import java.io.File; public class FileToList { public static void main(String[] args) { try { // Create a Scanner object for the file Scanner s = new Scanner(new File("filepath")); // Create an ArrayList to store the words ArrayList<String> list = new ArrayList<>(); // Loop through the file and add each word to the ArrayList while (s.hasNext()) { list.add(s.next()); } // Close the Scanner s.close(); // Print the ArrayList to the console System.out.println(list); } catch (Exception e) { e.printStackTrace(); } } }
說明
- 程式碼實例化一個Scanner 物件來讀取檔案。
- 它會建立一個空的 ArrayList 來儲存字串。
- while 循環用於迭代文件中的每個單字。
- s.next() 方法會擷取下一個單字並將其新增至ArrayList。
- 讀取整個檔案後,掃描器關閉。
- ArrayList 被印到控制台檢查。
逐行讀取的替代方法
如果您喜歡逐行而不是逐字讀取文件,請將程式碼修改如下:
... // Loop through the file and add each line to the ArrayList while (s.hasNextLine()) { list.add(s.nextLine()); } ...
在這種情況下,s.hasNextLine() 檢查是否有新行,並且s.nextLine()檢索整行。
以上是Java中如何將文件讀入ArrayList?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
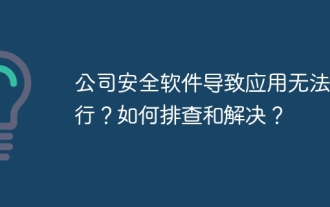
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
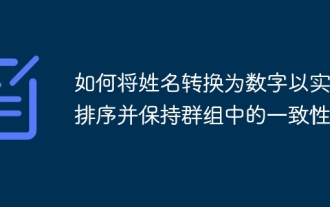
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
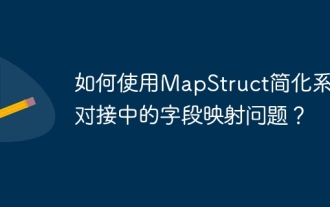
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
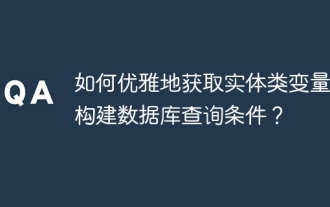
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
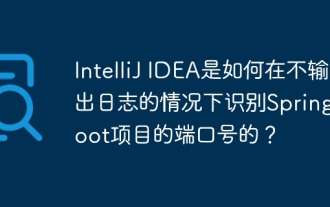
在使用IntelliJIDEAUltimate版本啟動Spring...
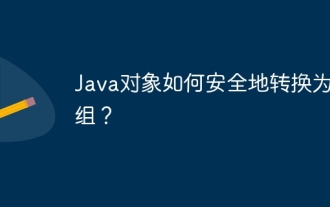
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
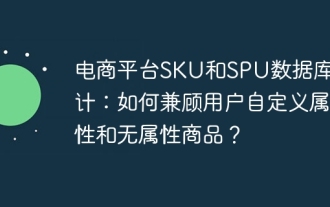
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
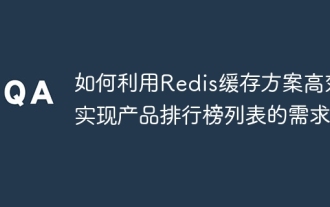
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
