Ionic:Angular CapacitorJS 和 SQLite
Angular 18 獨立應用程式和 CapacitorJS 中的 SQLite 集成
本教學介紹如何將 SQLite 整合到使用獨立元件和訊號的 Angular 18 應用程式中。我們將使用 @capacitor-community/sqlite 外掛程式為 Android 和 iOS 平台啟用 SQLite 功能。
先決條件
確保您已安裝以下軟體:
- Node.js 和 npm
- CapacitorJS
- 具有獨立組件和訊號的 Angular 18
第 1 步:安裝所需的軟體包
執行以下命令來安裝必要的依賴項:
npm install @capacitor-community/sqlite npx cap sync
這些指令安裝 SQLite 外掛程式並將變更與本機平台同步。
步驟2:建立資料庫服務
資料庫邏輯將封裝在服務中,以提高可維護性。
// src/app/services/database.service.ts import { Injectable } from '@angular/core'; import { CapacitorSQLite, SQLiteConnection, SQLiteDBConnection } from '@capacitor-community/sqlite'; @Injectable({ providedIn: 'root', }) export class DatabaseService { private sqlite: SQLiteConnection; private db: SQLiteDBConnection | null = null; constructor() { this.sqlite = new SQLiteConnection(CapacitorSQLite); } async initializeDatabase(): Promise<void> { try { // Check connection consistency const retCC = (await this.sqlite.checkConnectionsConsistency()).result; // Check is connected const isConnection = (await this.sqlite.isConnection('appDB', false)).result; if (!isConnection && !retCC) { // Create a new connection this.db = await this.sqlite.createConnection('appDB', false, 'no-encryption', 1, false); await this.db.open(); await this.createTables(); } else { // Retrieve existing connection this.db = await this.sqlite.retrieveConnection('appDB', false); await this.db.open(); } } catch (error) { console.error('Error initializing the database:', error); } } private async createTables(): Promise<void> { if (!this.db) throw new Error('Database connection is not open'); const createTableQuery = ` CREATE TABLE IF NOT EXISTS users ( id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT NOT NULL, age INTEGER NOT NULL ); `; await this.db.execute(createTableQuery); } async addUser(name: string, age: number): Promise<void> { if (!this.db) throw new Error('Database connection is not open'); const insertQuery = `INSERT INTO users (name, age) VALUES (?, ?);`; await this.db.run(insertQuery, [name, age]); } async getUsers(): Promise<any[]> { if (!this.db) throw new Error('Database connection is not open'); const result = await this.db.query('SELECT * FROM users;'); return result.values || []; } async closeDatabase(): Promise<void> { if (this.db) { await this.sqlite.closeConnection('appDB'); this.db = null; } } }
第三步:在根元件中初始化資料庫服務
在應用程式的根元件中,初始化 DatabaseService。
// src/app/app.component.ts import { Component, OnInit } from '@angular/core'; import { DatabaseService } from './services/database.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', }) export class AppComponent implements OnDestroy, AfterViewInit { constructor(private databaseService: DatabaseService) {} // Important: Initialize the database connection when the component is created async ngAfterViewInit() { await this.databaseService.initializeDatabase(); } // Important: Close the database connection when the component is destroyed async ngOnDestroy() { await this.databaseService.closeDatabase(); } }
第四步:在元件中使用資料庫服務
您現在可以在任何元件中使用DatabaseService來執行CRUD操作。
// src/app/components/user-list/user-list.component.ts import { Component, OnInit } from '@angular/core'; import { DatabaseService } from '../../services/database.service'; @Component({ selector: 'app-user-list', templateUrl: './user-list.component.html', }) export class UserListComponent implements OnInit { users: any[] = []; constructor(private databaseService: DatabaseService) {} async ngOnInit() { await this.databaseService.addUser('Max Mustermann', 25); await this.databaseService.addUser('Erika Musterfrau', 30); this.users = await this.databaseService.getUsers(); } }
筆記
- 連線一致性:確保使用 checkConnectionsConsistency() 檢查連線一致性,以避免重複的連線錯誤。
- 單例服務:使用Angular的@Injectable和providedIn:'root'來確保DatabaseService是單例。
- 在兩個平台上進行測試:始終在 Android 和 iOS 裝置上測試您的實作。
結論
透過這些步驟,您已成功使用 CapacitorJS 將 SQLite 整合到 Angular 18 獨立應用程式中。此實作可確保連線一致性並將資料庫操作封裝在可重複使用服務中。
以上是Ionic:Angular CapacitorJS 和 SQLite的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
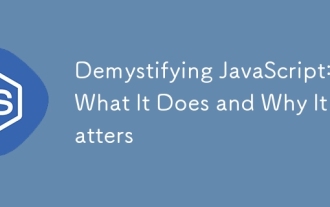
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
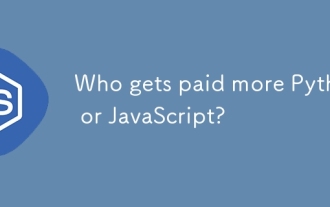
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
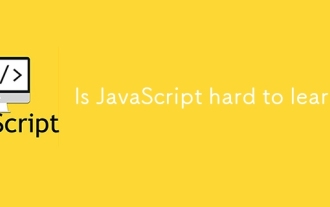
學習JavaScript不難,但有挑戰。 1)理解基礎概念如變量、數據類型、函數等。 2)掌握異步編程,通過事件循環實現。 3)使用DOM操作和Promise處理異步請求。 4)避免常見錯誤,使用調試技巧。 5)優化性能,遵循最佳實踐。
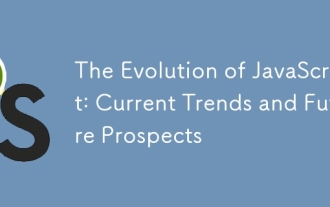
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
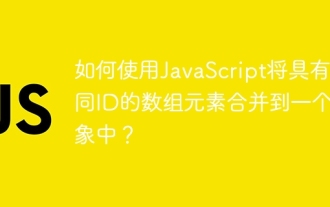
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
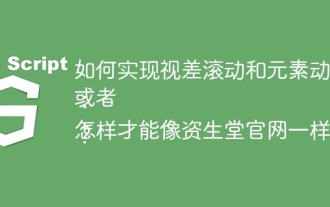
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
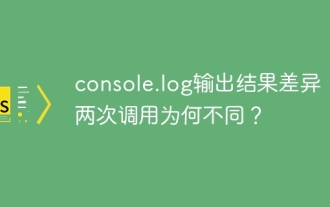
深入探討console.log輸出差異的根源本文將分析一段代碼中console.log函數輸出結果的差異,並解釋其背後的原因。 �...
