如何在 Java 中以數組形式高效檢索所有正規表示式匹配項?
以陣列形式擷取正規表示式符合
在 Java 中,判斷給定模式是否與特定字串相符非常簡單。然而,當處理多個匹配時,將它們收集到一個陣列中可能具有挑戰性。為了解決這個問題,請深入研究本指南,以了解如何有效地利用正規表示式配對。
迭代方法
要將匹配累積到數組中,請結合使用Matcher 和String:
import java.util.regex.Matcher; import java.util.regex.Pattern; ... List<String> allMatches = new ArrayList<>(); Matcher m = Pattern.compile("your regular expression here") .matcher(yourStringHere); while (m.find()) { allMatches.add(m.group()); }
此程式碼建立Matcher 物件,該物件系統地尋找輸入字串中的匹配子字串。每個成功的配對都會附加到 allMatches 清單中。最後,使用 allMatches.toArray(new String[0]) 將其轉換為陣列:
String[] matchesArr = allMatches.toArray(new String[0]);
用於 Lazier Matches的自訂可迭代
或者,考慮實現惰性迭代器遍歷並立即消耗匹配項,而無需不必要的操作處理:
public static Iterable<MatchResult> allMatches( final Pattern p, final CharSequence input) { return new Iterable<MatchResult>() { public Iterator<MatchResult> iterator() { return new Iterator<MatchResult>() { // Internal Matcher final Matcher matcher = p.matcher(input); // Lazy-filled MatchResult MatchResult pending; public boolean hasNext() { if (pending == null && matcher.find()) { pending = matcher.toMatchResult(); } return pending != null; } public MatchResult next() { if (!hasNext()) { throw new NoSuchElementException(); } MatchResult next = pending; pending = null; return next; } public void remove() { throw new UnsupportedOperationException(); } }; } }; }
使用如下:
for (MatchResult match : allMatches( Pattern.compile("[abc]"), "abracadabra")) { System.out.println(match.group() + " at " + match.start()); }
以上是如何在 Java 中以數組形式高效檢索所有正規表示式匹配項?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
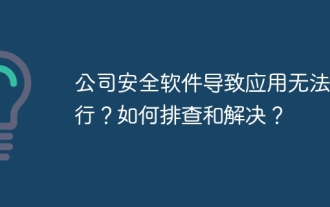
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
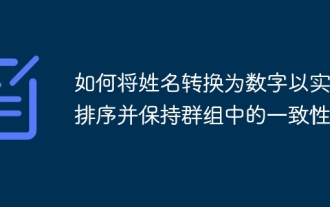
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
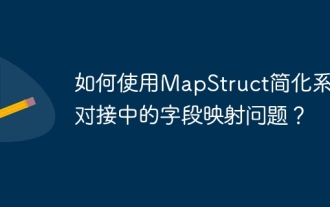
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
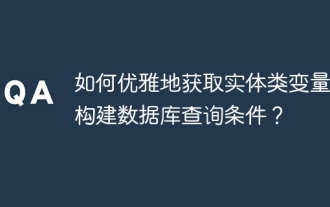
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
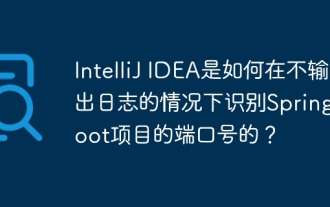
在使用IntelliJIDEAUltimate版本啟動Spring...
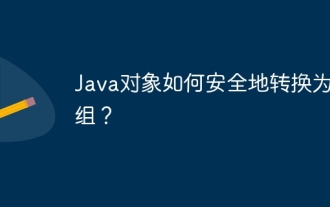
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
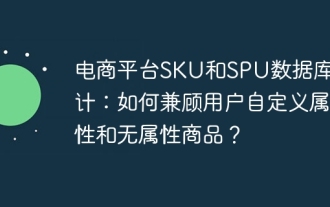
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
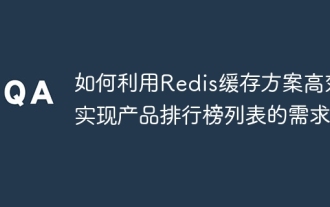
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
