如何有效率地上傳大檔案(≥3GB)到FastAPI後端?
如何上傳大檔案(≥3GB)到FastAPI後端?
使用Requests-Toolbelt
使用requests-toolbelt程式庫時,在宣告 upload_file 欄位時,請務必指定檔案名稱和 Content-Type 標頭。以下是一個範例:
filename = 'my_file.txt' m = MultipartEncoder(fields={'upload_file': (filename, open(filename, 'rb'))}) r = requests.post( url, data=m, headers={'Content-Type': m.content_type}, verify=False, ) print(r.request.headers) # confirm that the 'Content-Type' header has been set.
使用 Python Requests/HTTPX
另一個選擇是使用 Python 的 requests 或 HTTPX 函式庫,它們都可以有效地處理串流檔案上傳。以下是每個範例:
使用請求:
import requests url = '...' filename = '...' with open(filename, 'rb') as file: r = requests.post( url, files={'upload_file': file}, headers={'Content-Type': 'multipart/form-data'}, )
自動使用HTTPX:
import httpx url = '...' filename = '...' with open(filename, 'rb') as file: r = httpx.post( url, files={'upload_file': file}, )
自動支援串流檔案上傳,而請求則需要設定Content-Type header為'multipart/form-data'。
使用 FastAPI Stream() 方法
FastAPI 的 .stream() 方法可讓您透過將請求正文作為流存取來避免將大檔案載入到記憶體中。若要使用此方法,請依照下列步驟操作:
- 安裝streaming-form-data 函式庫: 此函式庫為多部分/表單資料資料提供串流解析器。
- 建立FastAPI端點:使用.stream()方法將請求體解析為流,並利用該流ing_form_data 函式庫來處理 multipart/form-data 的解析。
- 註冊目標: 定義 FileTarget 和 ValueTarget 物件分別處理檔案和表單資料解析。
上傳檔案大小驗證
確保上傳檔案大小符合不超過指定的限制,可以使用 MaxSizeValidator。這是一個範例:
from streaming_form_data import streaming_form_data from streaming_form_data import MaxSizeValidator FILE_SIZE_LIMIT = 1024 * 1024 * 1024 # 1 GB def validate_file_size(chunk: bytes): if FILE_SIZE_LIMIT > 0: streaming_form_data.validators.MaxSizeValidator( FILE_SIZE_LIMIT). __call__(chunk)
實作端點
這是一個包含這些技術的範例端點:
from fastapi import FastAPI, File, Request from fastapi.responses import HTMLResponse from streaming_form_data.targets import FileTarget, ValueTarget from streaming_form_data import StreamingFormDataParser app = FastAPI() @app.post('/upload') async def upload(request: Request): # Parse the HTTP headers to retrieve the boundary string. parser = StreamingFormDataParser(headers=request.headers) # Register FileTarget and ValueTarget objects. file_ = FileTarget() data = ValueTarget() parser.register('upload_file', file_) parser.register('data', data) async for chunk in request.stream(): parser.data_received(chunk) # Validate file size (if necessary) validate_file_size(file_.content) # Process the uploaded file and data. return {'message': 'File uploaded successfully!'}
以上是如何有效率地上傳大檔案(≥3GB)到FastAPI後端?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
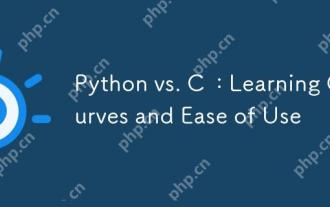
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
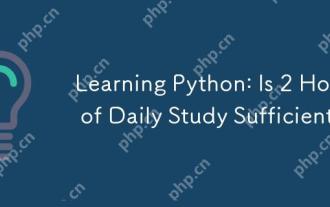
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
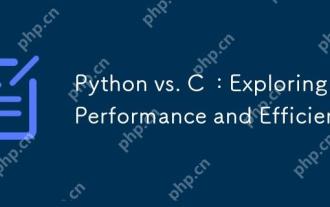
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
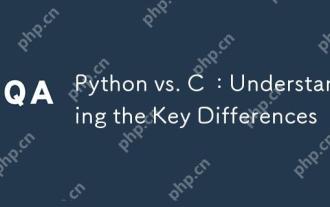
Python和C 各有優勢,選擇應基於項目需求。 1)Python適合快速開發和數據處理,因其簡潔語法和動態類型。 2)C 適用於高性能和系統編程,因其靜態類型和手動內存管理。
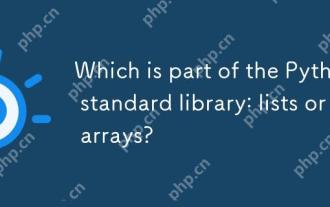
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
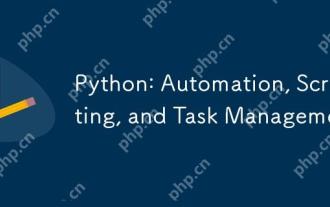
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
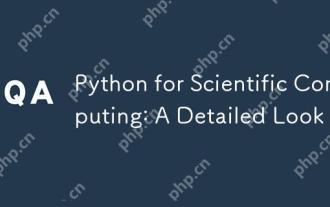
Python在科學計算中的應用包括數據分析、機器學習、數值模擬和可視化。 1.Numpy提供高效的多維數組和數學函數。 2.SciPy擴展Numpy功能,提供優化和線性代數工具。 3.Pandas用於數據處理和分析。 4.Matplotlib用於生成各種圖表和可視化結果。
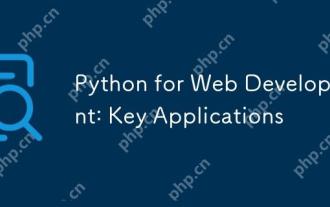
Python在Web開發中的關鍵應用包括使用Django和Flask框架、API開發、數據分析與可視化、機器學習與AI、以及性能優化。 1.Django和Flask框架:Django適合快速開發複雜應用,Flask適用於小型或高度自定義項目。 2.API開發:使用Flask或DjangoRESTFramework構建RESTfulAPI。 3.數據分析與可視化:利用Python處理數據並通過Web界面展示。 4.機器學習與AI:Python用於構建智能Web應用。 5.性能優化:通過異步編程、緩存和代碼優
