在沒有直接 WSDL 支援的情況下,如何在 Go 中處理 SOAP 請求和回應?
Go 中的WSDL/SOAP 支援
雖然Go 中沒有對WSDL 的直接支持,但有多種選項可用於處理SOAP請求和回應。
建構SOAP 請求手動
標準編碼/xml套件可用於手動建立SOAP請求,但它缺乏處理SOAP某些方面所需的功能,例如命名空間和前綴。
使用 xmlutil 函式庫
github.com/webconnex/xmlutil 函式庫提供了處理 SOAP 請求和回應的更強大的解決方案。它包括以下功能:
- 命名空間和前綴註冊表
- 自訂類型註冊
- 帶有類型資訊的XML編碼和解碼
- 對類型的支援強制執行、xsi:type屬性等
手動解碼 SOAP 回應
要解碼 SOAP 回應,您可以使用encoding/xml 套件或 xmlutil 等程式庫。該過程涉及使用解碼器解析 XML 響應並提取所需的資料。
使用 xmlutil 的範例
這裡是答案中提供的範例的修改版本:
package main import ( "bytes" "github.com/webconnex/xmlutil" "log" "strings" "encoding/xml" ) type Envelope struct { Body `xml:"soap:"` } type Body struct { Msg interface{} } type MethodCall struct { One string Two string } type MethodCallResponse struct { Three string } func main() { x := xmlutil.NewXmlUtil() x.RegisterNamespace("http://www.w3.org/2001/XMLSchema-instance", "xsi") x.RegisterNamespace("http://www.w3.org/2001/XMLSchema", "xsd") x.RegisterNamespace("http://www.w3.org/2003/05/soap-envelope", "soap") x.RegisterTypeMore(Envelope{}, xml.Name{"http://www.w3.org/2003/05/soap-envelope", ""}, []xml.Attr{ xml.Attr{xml.Name{"xmlns", "xsi"}, "http://www.w3.org/2001/XMLSchema-instance"}, xml.Attr{xml.Name{"xmlns", "xsd"}, "http://www.w3.org/2001/XMLSchema"}, xml.Attr{xml.Name{"xmlns", "soap"}, "http://www.w3.org/2003/05/soap-envelope"}, }) x.RegisterTypeMore("", xml.Name{}, []xml.Attr{ xml.Attr{xml.Name{"http://www.w3.org/2001/XMLSchema-instance", "type"}, "xsd:string"}, }) buf := new(bytes.Buffer) buf.WriteString(`<soap:Envelope><soap:Body><MethodCall><One>one</One><Two>two</Two></MethodCall></soap:Body></soap:Envelope>`) enc := x.NewEncoder(buf) env := &Envelope{Body{MethodCall{ One: "one", Two: "two", }}} if err := enc.Encode(env); err != nil { log.Fatal(err) } response := `<?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:soap="http://www.w3.org/2003/05/soap-envelope"> <soap:Body> <MethodCallResponse> <Three>three</Three> </MethodCallResponse> </soap:Body> </soap:Envelope>` dec := x.NewDecoder(strings.NewReader(response)) var resp Envelope if err := dec.DecodeElement(&resp, nil); err != nil { log.Fatal(err) } fmt.Printf("%#v\n", resp) }
以上是在沒有直接 WSDL 支援的情況下,如何在 Go 中處理 SOAP 請求和回應?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
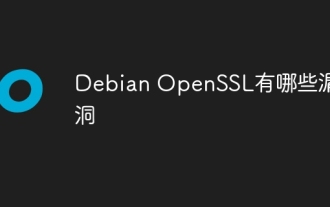
OpenSSL,作為廣泛應用於安全通信的開源庫,提供了加密算法、密鑰和證書管理等功能。然而,其歷史版本中存在一些已知安全漏洞,其中一些危害極大。本文將重點介紹Debian系統中OpenSSL的常見漏洞及應對措施。 DebianOpenSSL已知漏洞:OpenSSL曾出現過多個嚴重漏洞,例如:心臟出血漏洞(CVE-2014-0160):該漏洞影響OpenSSL1.0.1至1.0.1f以及1.0.2至1.0.2beta版本。攻擊者可利用此漏洞未經授權讀取服務器上的敏感信息,包括加密密鑰等。
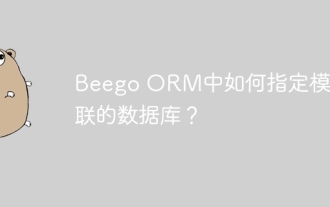
在BeegoORM框架下,如何指定模型關聯的數據庫?許多Beego項目需要同時操作多個數據庫。當使用Beego...
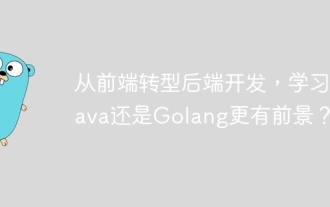
後端學習路徑:從前端轉型到後端的探索之旅作為一名從前端開發轉型的後端初學者,你已經有了nodejs的基礎,...
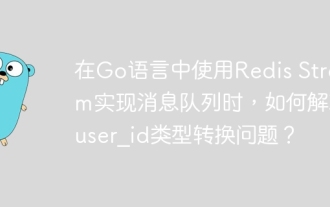
Go語言中使用RedisStream實現消息隊列時類型轉換問題在使用Go語言與Redis...
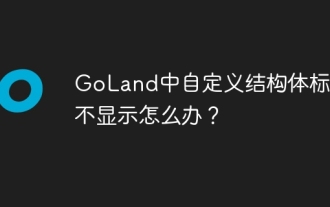
GoLand中自定義結構體標籤不顯示怎麼辦?在使用GoLand進行Go語言開發時,很多開發者會遇到自定義結構體標籤在�...
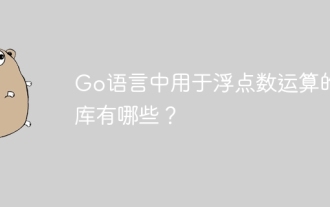
Go語言中用於浮點數運算的庫介紹在Go語言(也稱為Golang)中,進行浮點數的加減乘除運算時,如何確保精度是�...
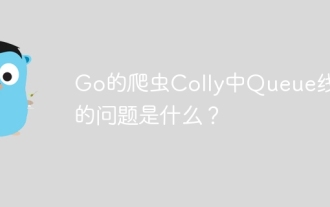
Go爬蟲Colly中的Queue線程問題探討在使用Go語言的Colly爬蟲庫時,開發者常常會遇到關於線程和請求隊列的問題。 �...

本文介紹如何在Debian系統上配置MongoDB實現自動擴容,主要步驟包括MongoDB副本集的設置和磁盤空間監控。一、MongoDB安裝首先,確保已在Debian系統上安裝MongoDB。使用以下命令安裝:sudoaptupdatesudoaptinstall-ymongodb-org二、配置MongoDB副本集MongoDB副本集確保高可用性和數據冗餘,是實現自動擴容的基礎。啟動MongoDB服務:sudosystemctlstartmongodsudosys
