如何從 C 成員函數呼叫 Objective-C 方法?
從 C 成員函數呼叫 Objective-C 方法
問題
EAGLView 類別需要從 C 類別呼叫成員函數而不會出現問題。然而,在 C 類別中,需要呼叫 Objective-C 函數“[context renderbufferStorage:GL_RENDERBUFFER fromDrawable:(CAEAGLLayer*)self.layer];”,這是使用純 C 語法無法實現的。
解決方案
要混合 Objective-C 和 C ,請謹慎行事。以下是使用C 包裝函數包裝Objective-C 呼叫的逐步方法:
-
建立C 介面標頭:
- 建立建立:
#include <stdio.h> // for printf #include <stdint.h> // for uintptr_t typedef uintptr_t Id; // Assume a simplified EAGLView class extern void EAGLViewDoSomethingWith(Id* poself, void *aparam); int MyObjectDoSomethingWith(void *myObjectInstance, void *parameter) { printf("C wrapper function called!\n"); // Assuming Objective-C method takes a single int argument return EAGLViewDoSomethingWith(myObjectInstance, 21); }
-
- 定義 Objective-C類別:
// MyObject.h @interface MyObject : NSObject - (int)doSomethingWith:(void *)aParameter; @end
// MyObject.mm #import "MyObject.h" @implementation MyObject - (int)doSomethingWith:(void *)aParameter { // Implement Objective-C function return 42; } @end
-
- 實作 C類別:
#include "MyObject-C-Interface.h" class MyCPPClass { public: void someMethod(void *objectiveCObject) { int result = MyObjectDoSomethingWith(objectiveCObject, nullptr); } };
- 用於物件導向實現的PIMPL 慣用法
-
PIMPL(指向實作的指標)慣用法可用於物件導向>
- 定義一個 C介面:
建立一個頭檔“MyObject-C-Interface.h”,定義一個帶包裝器的類別
#include <stdio.h> // for printf #include <stdint.h> // for uintptr_t typedef uintptr_t Id; class MyClassImpl { public: MyClassImpl() : self(nullptr) {} ~MyClassImpl() { if (self) dealloc(); } int doSomethingWith(void *parameter) { return 42; } private: Id self; void dealloc() { if (self) free(self); } }; int EAGLViewDoSomethingWith(Id* poself, void* aparam); int MyObjectDoSomethingWith(void *myObjectInstance, void *parameter) { printf("C wrapper function called!\n"); return EAGLViewDoSomethingWith(myObjectInstance, 21); }
-
- 建立Objective-C類別介面:
定義一個頭檔"MyObject.h".
@interface MyObject : NSObject - (int)doSomethingWith:(void *)aParameter; @end
#import "MyObject.h" @implementation MyObject { MyClassImpl* _impl; } - (int)doSomethingWith:(void *)aParameter { if (!_impl) _impl = [MyClassImpl new]; return [_impl doSomethingWith:aParameter]; } @end
#include "MyObject-C-Interface.h" class MyCPPClass { public: void someMethod(void *objectiveCObject) { int result = MyObjectDoSomethingWith(objectiveCObject, nullptr); } };
定義一個實作檔案“MyObject.mm”,在其中實例化MyObject 實例MyObject.實作 C類別:包含C 介面C 類別頭檔「MyCPPClass.h」中的頭檔並使用C 包裝器 這種方法提供了一個更隔離和靈活的解決方案,使Objective-C 實作和 C包裝器能夠在不影響 C 程式碼的情況下進行修改。
以上是如何從 C 成員函數呼叫 Objective-C 方法?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
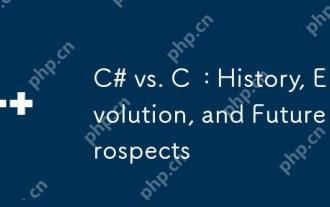
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
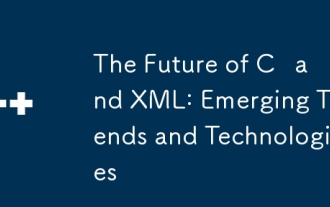
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
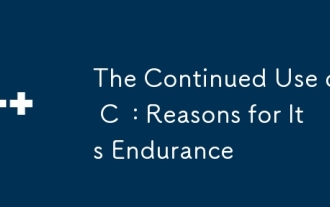
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
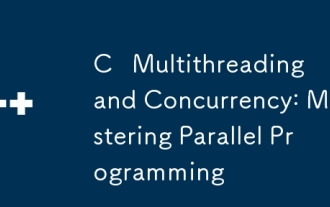
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
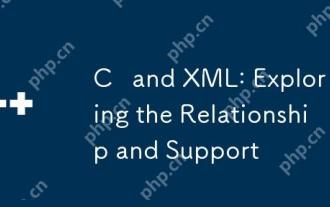
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
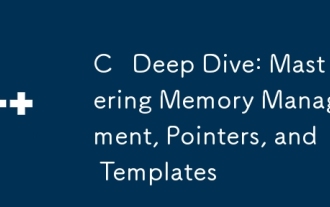
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
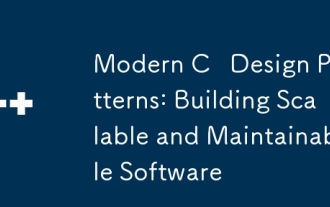
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
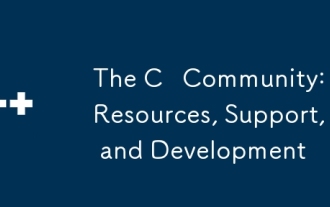
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
