如何使用 Shunting-Yard 演算法建立 PHP 計算器?
如何用 PHP 製作計算器
問題:
建立一個 PHP計算器,可以計算輸入的簡單代數表達式由使用者使用標準代數符號,包括表達式
常見方法:
一個低效但臨時的解涉及替換表達式的字串表示形式中的表達式,例如:
for ($a=1; $a < 1000; $a++) { for ($b=1; $b < 1000; $b++) { string_replace($a . '*' . $b, $a*$b, $string); } }
調車場演算法:
A更有效的方法是使用 Shunting Yard 演算法。
實作:
終端表達式(值和運算子):
abstract class TerminalExpression { protected $value = ''; public static function factory($value) { // Create terminal expressions based on the provided value (number, operator, or parenthesis) } abstract public function operate(Stack $stack); } class Number extends TerminalExpression { public function operate(Stack $stack) { return $this->value; } } class Operator extends TerminalExpression { protected $precidence = 0; protected $leftAssoc = true; public function getPrecidence() { return $this->precidence; } public function isLeftAssoc() { return $this->leftAssoc; } } class Addition extends Operator { protected $precidence = 4; } class Subtraction extends Operator { protected $precidence = 4; } class Multiplication extends Operator { protected $precidence = 5; } class Division extends Operator { protected $precidence = 5; } class Parenthesis extends TerminalExpression { protected $precidence = 7; public function isParenthesis() { return true; } }
堆疊實作:
class Stack { protected $data = []; public function push($element) { array_push($this->data, $element); } public function pop() { return array_pop($this->data); } }
數學類別(執行器):
class Math { protected $variables = []; public function evaluate($string) { $stack = $this->parse($string); return $this->run($stack); } public function parse($string) { // Tokenize expression $tokens = array_map('trim', preg_split('((\d+|\+|-|\(|\)|\*|/)|\s+)', $string, null, PREG_SPLIT_NO_EMPTY | PREG_SPLIT_DELIM_CAPTURE)); // Parse operators and parentheses using the Shunting Yard algorithm $output = new Stack(); $operators = new Stack(); foreach ($tokens as $token) { $expression = TerminalExpression::factory($token); if ($expression->isOperator()) { $this->parseOperator($expression, $output, $operators); } elseif ($expression->isParenthesis()) { $this->parseParenthesis($expression, $output, $operators); } else { $output->push($expression); } } // Pop remaining operators on stack and push to output while (($op = $operators->pop()) && $op->isOperator()) { if ($op->isParenthesis()) { throw new RuntimeException('Mismatched Parenthesis'); } $output->push($op); } return $output; } public function run(Stack $stack) { // Evaluate stack and return result while (($operator = $stack->pop()) && $operator->isOperator()) { $value = $operator->operate($stack); $stack->push(TerminalExpression::factory($value)); } return $operator ? $operator->render() : $this->render($stack); } protected function extractVariables($token) { if ($token[0] == '$') { $key = substr($token, 1); return isset($this->variables[$key]) ? $this->variables[$key] : 0; } return $token; } // ... }
使用此實現,您可以實現,您可以如下計算表達式:
$math = new Math(); $answer = $math->evaluate('(2 + 3) * 4'); // 20 $answer = $math->evaluate('1 + 2 * ((3 + 4) * 5 + 6)'); // 83 $answer = $math->evaluate('(1 + 2) * (3 + 4) * (5 + 6)'); // 231 $math->registerVariable('a', 4); $answer = $math->evaluate('($a + 3) * 4'); // 28
以上是如何使用 Shunting-Yard 演算法建立 PHP 計算器?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
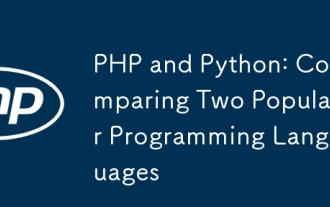
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
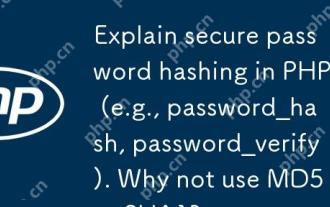
在PHP中,應使用password_hash和password_verify函數實現安全的密碼哈希處理,不應使用MD5或SHA1。1)password_hash生成包含鹽值的哈希,增強安全性。 2)password_verify驗證密碼,通過比較哈希值確保安全。 3)MD5和SHA1易受攻擊且缺乏鹽值,不適合現代密碼安全。
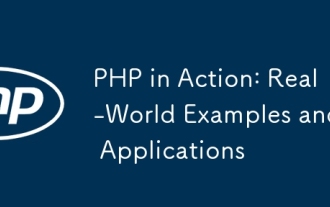
PHP在電子商務、內容管理系統和API開發中廣泛應用。 1)電子商務:用於購物車功能和支付處理。 2)內容管理系統:用於動態內容生成和用戶管理。 3)API開發:用於RESTfulAPI開發和API安全性。通過性能優化和最佳實踐,PHP應用的效率和可維護性得以提升。
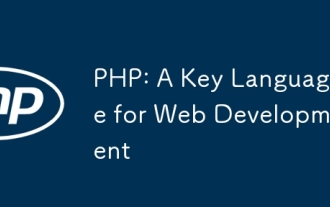
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
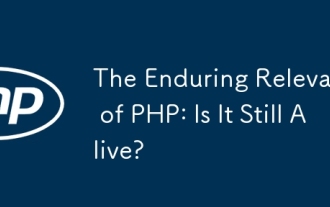
PHP仍然具有活力,其在現代編程領域中依然佔據重要地位。 1)PHP的簡單易學和強大社區支持使其在Web開發中廣泛應用;2)其靈活性和穩定性使其在處理Web表單、數據庫操作和文件處理等方面表現出色;3)PHP不斷進化和優化,適用於初學者和經驗豐富的開發者。
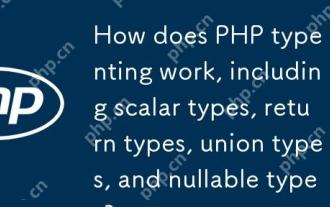
PHP類型提示提升代碼質量和可讀性。 1)標量類型提示:自PHP7.0起,允許在函數參數中指定基本數據類型,如int、float等。 2)返回類型提示:確保函數返回值類型的一致性。 3)聯合類型提示:自PHP8.0起,允許在函數參數或返回值中指定多個類型。 4)可空類型提示:允許包含null值,處理可能返回空值的函數。
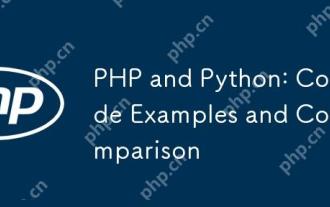
PHP和Python各有優劣,選擇取決於項目需求和個人偏好。 1.PHP適合快速開發和維護大型Web應用。 2.Python在數據科學和機器學習領域佔據主導地位。
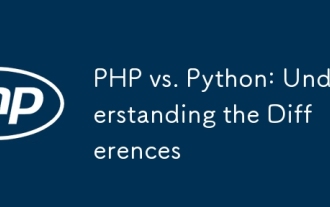
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
