如何正確追加到 Go 結構中的切片?
Go - 附加到結構體中的切片
在 Go 中,附加到結構體中的切片需要仔細注意變數引用。在使用結構體中的切片時,這可能會變得令人困惑,尤其是當接收結構體的方法是指標接收器時。
問題
考慮以下程式碼:
package main import "fmt" type MyBoxItem struct { Name string } type MyBox struct { Items []MyBoxItem } func (box *MyBox) AddItem(item MyBoxItem) []MyBoxItem { return append(box.Items, item) } func main() { item1 := MyBoxItem{Name: "Test Item 1"} box := MyBox{[]MyBoxItem{}} // Initialize box with an empty slice AddItem(box, item1) // This is where the problem arises fmt.Println(len(box.Items)) }
問題出現在對 AddItem 方法的呼叫中。當呼叫 AddItem(box, item1) 方法而不是 box.AddItem(item1) 時,會建立 box 結構的新副本,而不是修改原始結構。
解
要解決此問題,請將AddItem 方法的結果分配回原始切片結構體:
func (box *MyBox) AddItem(item MyBoxItem) { box.Items = append(box.Items, item) }
透過這樣做,AddItem 方法中對切片所做的變更將反映在結構體的原始切片欄位中。
修訂的主函數
使用更新的AddItem 方法,修正後的main 函數應該是:
func main() { item1 := MyBoxItem{Name: "Test Item 1"} box := MyBox{[]MyBoxItem{}} box.AddItem(item1) // Call the method correctly fmt.Println(len(box.Items)) }
現在,輸出將正確列印Items 切片的長度,添加項目後應該為1。
以上是如何正確追加到 Go 結構中的切片?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
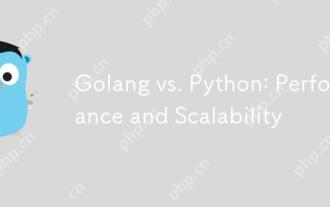
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
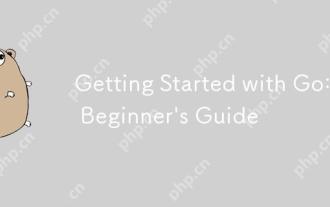
goisidealforbeginnersandsubableforforcloudnetworkservicesduetoitssimplicity,效率和concurrencyFeatures.1)installgromtheofficialwebsitealwebsiteandverifywith'.2)
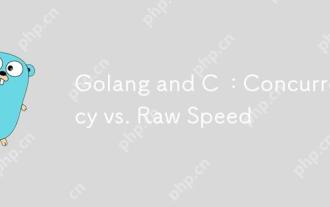
Golang在並發性上優於C ,而C 在原始速度上優於Golang。 1)Golang通過goroutine和channel實現高效並發,適合處理大量並發任務。 2)C 通過編譯器優化和標準庫,提供接近硬件的高性能,適合需要極致優化的應用。
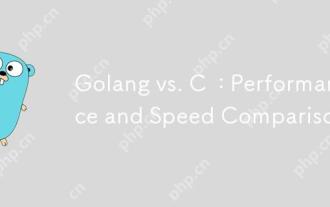
Golang適合快速開發和並發場景,C 適用於需要極致性能和低級控制的場景。 1)Golang通過垃圾回收和並發機制提升性能,適合高並發Web服務開發。 2)C 通過手動內存管理和編譯器優化達到極致性能,適用於嵌入式系統開發。
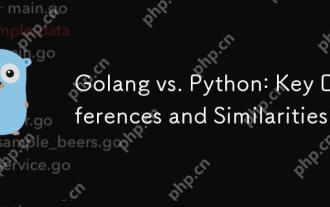
Golang和Python各有优势:Golang适合高性能和并发编程,Python适用于数据科学和Web开发。Golang以其并发模型和高效性能著称,Python则以简洁语法和丰富库生态系统著称。
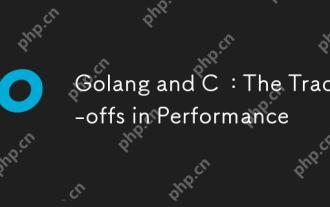
Golang和C 在性能上的差異主要體現在內存管理、編譯優化和運行時效率等方面。 1)Golang的垃圾回收機制方便但可能影響性能,2)C 的手動內存管理和編譯器優化在遞歸計算中表現更為高效。
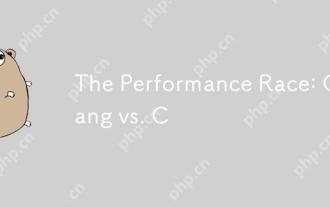
Golang和C 在性能競賽中的表現各有優勢:1)Golang適合高並發和快速開發,2)C 提供更高性能和細粒度控制。選擇應基於項目需求和團隊技術棧。
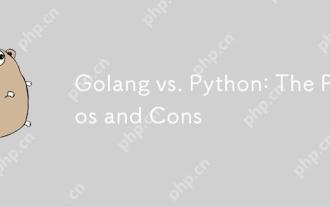
Golangisidealforbuildingscalablesystemsduetoitsefficiencyandconcurrency,whilePythonexcelsinquickscriptinganddataanalysisduetoitssimplicityandvastecosystem.Golang'sdesignencouragesclean,readablecodeanditsgoroutinesenableefficientconcurrentoperations,t
