使用 Python 和 Boto3 尋找並驗證 AWS 中未使用的安全性群組
有效管理 AWS 安全群組對於維護安全且經濟高效的雲端環境至關重要。安全群組是 AWS 網路安全的重要組成部分,但隨著時間的推移,未使用的安全群組會不斷累積。這些未使用的群組不僅會使您的環境變得混亂,還可能帶來安全風險或不必要地增加成本。
在本文中,我們將探討如何使用 Python 和 Boto3 識別 AWS 環境中未使用的安全群組、驗證它們並確保它們不被任何其他資源引用。我們還將研究如何安全地確定是否可以刪除這些群組。
先決條件
要學習本教程,您需要以下內容:
AWS 帳戶:確保您有權存取要搜尋未使用的安全群組的 AWS 環境。
Boto3 已安裝:您可以透過執行以下命令來安裝 Boto3 Python SDK:
pip install boto3
已設定 AWS 憑證:確保您使用 AWS CLI 或使用 IAM 角色或環境變數直接在程式碼中配置了 AWS 憑證。
代碼分解
讓我們看一下程式碼,用於識別給定 AWS 區域中未使用的安全性群組、驗證它們並檢查它們是否被任何其他群組引用。
步驟 1:取得所有安全群組和 ENI
pip install boto3
- 取得安全群組:我們先呼叫describe_security_groups方法取得指定區域內的所有安全性群組。
- 檢索網路介面:接下來,我們使用describe_network_interfaces檢索所有網路介面。每個網路介面都可以有一個或多個與其關聯的安全群組。
- 識別已使用的安全群組:對於每個網路接口,我們將關聯的安全群組 ID 新增到名為used_sg_ids 的集合中。
- 尋找未使用的群組:然後我們將安全群組 ID 與正在使用的群組 ID 進行比較。如果一個群組沒有被使用(即它的ID不在used_sg_ids集合中),我們認為它沒有被使用,除了預設的安全性群組,它不能被刪除。
步驟 2:檢查安全群組引用
import boto3 from botocore.exceptions import ClientError def get_unused_security_groups(region='us-east-1'): """ Find security groups that are not being used by any resources. """ ec2_client = boto3.client('ec2', region_name=region) try: # Get all security groups security_groups = ec2_client.describe_security_groups()['SecurityGroups'] # Get all network interfaces enis = ec2_client.describe_network_interfaces()['NetworkInterfaces'] # Create set of security groups in use used_sg_ids = set() # Check security groups attached to ENIs for eni in enis: for group in eni['Groups']: used_sg_ids.add(group['GroupId']) # Find unused security groups unused_groups = [] for sg in security_groups: if sg['GroupId'] not in used_sg_ids: # Skip default security groups as they cannot be deleted if sg['GroupName'] != 'default': unused_groups.append({ 'GroupId': sg['GroupId'], 'GroupName': sg['GroupName'], 'Description': sg['Description'], 'VpcId': sg.get('VpcId', 'EC2-Classic') }) # Print results if unused_groups: print(f"\nFound {len(unused_groups)} unused security groups in {region}:") print("-" * 80) for group in unused_groups: print(f"Security Group ID: {group['GroupId']}") print(f"Name: {group['GroupName']}") print(f"Description: {group['Description']}") print(f"VPC ID: {group['VpcId']}") print("-" * 80) else: print(f"\nNo unused security groups found in {region}") return unused_groups except ClientError as e: print(f"Error retrieving security groups: {str(e)}") return None
- 檢查引用:此函數檢查特定安全群組是否被任何其他安全群組引用。它透過根據入站 (ip-permission.group-id) 和出站 (egress.ip-permission.group-id) 規則過濾安全群組來實現此目的。
- 傳回引用組:如果引用組,則函數傳回引用安全組的清單。如果沒有,則傳回 None。
步驟 3:驗證未使用的安全性群組
def check_sg_references(ec2_client, group_id): """ Check if a security group is referenced in other security groups' rules """ try: # Check if the security group is referenced in other groups response = ec2_client.describe_security_groups( Filters=[ { 'Name': 'ip-permission.group-id', 'Values': [group_id] } ] ) referencing_groups = response['SecurityGroups'] # Check for egress rules response = ec2_client.describe_security_groups( Filters=[ { 'Name': 'egress.ip-permission.group-id', 'Values': [group_id] } ] ) referencing_groups.extend(response['SecurityGroups']) return referencing_groups except ClientError as e: print(f"Error checking security group references: {str(e)}") return None
- 驗證未使用的安全群組:在最後一步中,腳本首先檢索未使用的安全群組。然後,對於每個未使用的群組,它會檢查是否有任何其他安全性群組在其規則中引用它。
- 輸出:腳本輸出該群組是否被引用,如果沒有,則可以安全刪除。
運行腳本
要執行腳本,只需執行 validate_unused_groups 函數即可。例如,當區域設定為 us-east-1 時,腳本將:
- 檢索 us-east-1 中的所有安全群組和網路介面。
- 辨識未使用的安全群組。
- 驗證並報告這些未使用的群組是否被其他安全群組引用。
範例輸出
def validate_unused_groups(region='us-east-1'): """ Validate and provide detailed information about unused security groups """ ec2_client = boto3.client('ec2', region_name=region) unused_groups = get_unused_security_groups(region) if not unused_groups: return print("\nValidating security group references...") print("-" * 80) for group in unused_groups: group_id = group['GroupId'] referencing_groups = check_sg_references(ec2_client, group_id) if referencing_groups: print(f"\nSecurity Group {group_id} ({group['GroupName']}) is referenced by:") for ref_group in referencing_groups: print(f"- {ref_group['GroupId']} ({ref_group['GroupName']})") else: print(f"\nSecurity Group {group_id} ({group['GroupName']}) is not referenced by any other groups") print("This security group can be safely deleted if not needed")
結論
使用此腳本,您可以自動執行在 AWS 中尋找未使用的安全群組的流程,並確保您不會保留不必要的資源。這有助於減少混亂、改善安全狀況,並可能透過刪除未使用的資源來降低成本。
您可以將此腳本擴展為:
- 根據標籤、VPC 或其他條件處理額外的過濾。
- 在偵測到未使用的群組時實施更進階的報告或警報。
- 與 AWS Lambda 整合以進行自動定期檢查。
確保您的 AWS 環境安全且組織良好!
以上是使用 Python 和 Boto3 尋找並驗證 AWS 中未使用的安全性群組的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
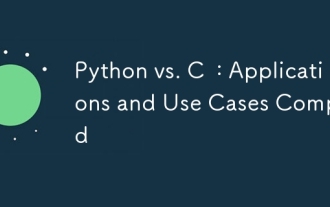
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
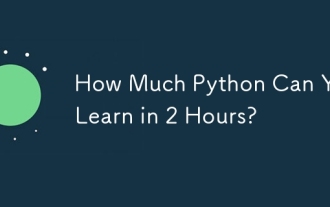
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
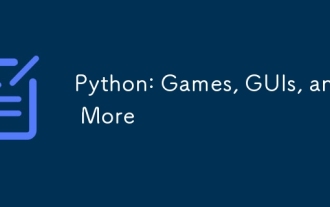
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
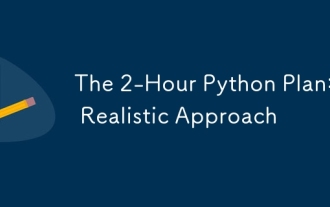
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
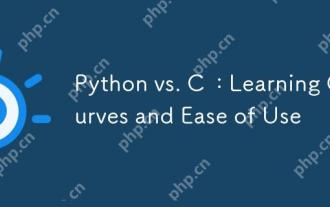
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
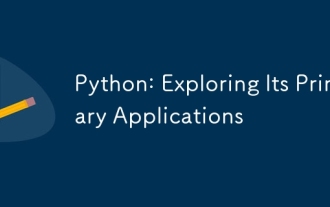
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
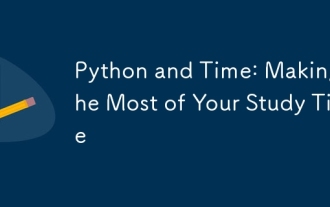
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
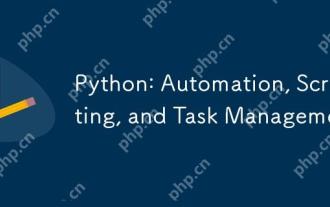
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
