如何在 Java 中設定資料庫連線嘗試的計時器?
在Java 中設定計時器以嘗試資料庫連線
在Java 中設定特定持續時間的計時器可以使用java.util 來實作.定時器類別。例如,要設定 2 分鐘的計時器並嘗試連接到資料庫,您可以按照以下步驟操作:
import java.util.Timer; import java.util.TimerTask; Timer timer = new Timer(); timer.schedule(new TimerTask() { @Override public void run() { // Database connection code try { // Connect to the database here } catch (Exception e) { // Handle the connection issue throw e; } } }, 2 * 60 * 1000); // Set the timer for 2 minutes
這將在 2 分鐘後執行任務並嘗試連接到資料庫。如果連接資料庫出現問題,則會拋出異常。
處理超時
如果您想在嘗試任務時專門處理超時,您可以使用java.util.concurrent 套件:
import java.util.concurrent.ExecutorService; import java.util.concurrent.Executors; import java.util.concurrent.TimeUnit; ExecutorService service = Executors.newSingleThreadExecutor(); try { Runnable task = () -> { // Database connection code try { // Connect to the database here } catch (Exception e) { // Handle connection issue here throw e; } }; Future<?> future = service.submit(task); future.get(2, TimeUnit.MINUTES); // Attempt the task for 2 minutes // If successful, the task will complete within 2 minutes } catch (TimeoutException e) { // Timeout occurred // Perform necessary cleanup or handle the error } catch (ExecutionException e) { // An exception occurred while executing the task } finally { service.shutdown(); }
此方法嘗試執行任務2 分鐘。如果任務完成時間超過 2 分鐘,則會拋出 TimeoutException。請注意,即使超時後,任務也可能會繼續在背景執行,最好相應地處理清理或錯誤。
以上是如何在 Java 中設定資料庫連線嘗試的計時器?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
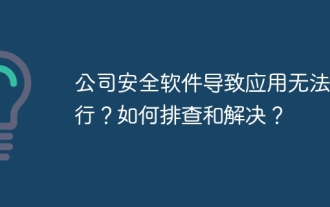
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
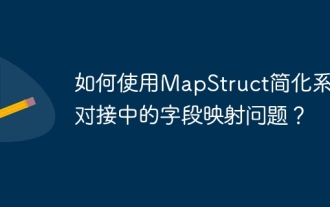
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
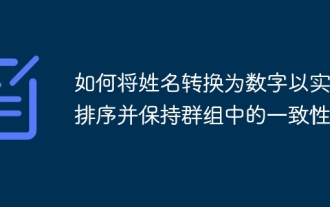
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
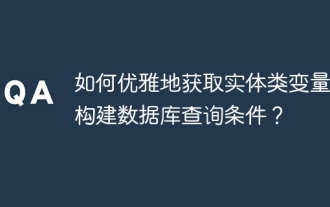
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
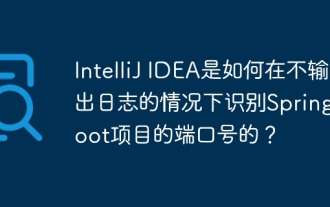
在使用IntelliJIDEAUltimate版本啟動Spring...
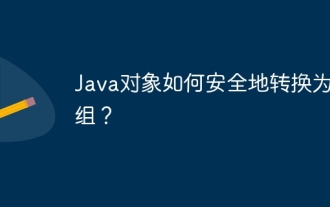
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
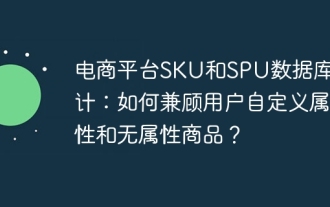
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
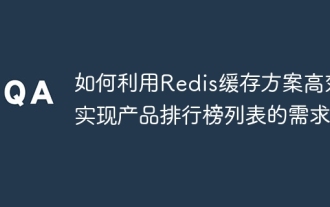
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
