React 中的函數元件與類別元件:選擇哪一個?
React 中的函數式元件與類別元件
React 讓開發者可以透過兩種主要方式建立元件:功能元件 和 類別元件。雖然兩者都具有定義可重複使用 UI 片段的相同目的,但它們在語法、功能和用法方面有所不同。
1.功能組件概述
函數式元件是傳回 JSX 的 JavaScript 函數。它們簡單且輕量級,主要設計用於呈現 UI。隨著 React Hooks 的引入,功能元件變得更加強大,現在可以管理狀態和生命週期方法。
功能組件範例
const Greeting = ({ name }) => { return <h1>Hello, {name}!</h1>; };
2.類別組件概述
類別元件是擴展 React.Component 的 ES6 類別。它們具有更複雜的結構,並且可以使用狀態和生命週期方法,而無需額外的工具。在 React Hooks 之前,類別元件對於管理狀態和邏輯至關重要。
類別組件範例
import React, { Component } from "react"; class Greeting extends Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
3.功能組件和類別組件之間的主要差異
Aspect | Functional Components | Class Components |
---|---|---|
Definition | Defined as a JavaScript function. | Defined as an ES6 class. |
State Management | Use React Hooks (e.g., useState). | Use this.state for state. |
Lifecycle Methods | Managed with Hooks like useEffect. | Use built-in lifecycle methods. |
Syntax Simplicity | Simple and concise. | More verbose with boilerplate. |
Performance | Generally faster and lightweight. | Slightly slower due to overhead. |
Usage Trend | Preferred in modern React development. | Common in legacy codebases. |
4.功能組件:優點與限制
優點
- 更簡單的語法:更容易寫和理解。
- Hooks:useState、useEffect 和 useContext 等強大的工具帶來了高級功能。
- 效能:與類別組件相比輕量級。
- 測試:更容易測試和調試。
限制
- 在引入 Hooks 之前依賴第三方解決方案進行狀態和生命週期處理。
5.類別元件:優點與限制
優點
- 狀態和生命週期:對管理狀態和生命週期方法的內建支援。
- 舊版相容性:對於 Hooks 之前的較舊 React 專案至關重要。
限制
- 詳細:需要更多樣板程式碼(例如建構子、this 綁定)。
- 效能:由於其開銷和類別結構,稍微慢一些。
- 過時的實踐:隨著功能組件主導現代開發,它變得不那麼常見。
6.管理狀態與生命週期
在功能組件
React Hooks 提供對功能元件中的狀態和生命週期方法的存取。
- 使用 useState 作為狀態:
const Greeting = ({ name }) => { return <h1>Hello, {name}!</h1>; };
- 使用 useEffect 進行生命週期:
import React, { Component } from "react"; class Greeting extends Component { render() { return <h1>Hello, {this.props.name}!</h1>; } }
類別組件
狀態和生命週期方法是內建的,但需要更多樣板。
- 管理狀態:
const Counter = () => { const [count, setCount] = React.useState(0); return ( <div> <p>Count: {count}</p> <button onClick={() => setCount(count + 1)}>Increment</button> </div> ); };
- 生命週期方法:
const Timer = () => { React.useEffect(() => { console.log("Component Mounted"); return () => console.log("Component Unmounted"); }, []); return <p>Timer is running...</p>; };
7.何時使用函數元件或類別元件
何時使用函數元件
- 建立現代 React 應用程式。
- 使用 Hooks 簡化 UI 邏輯。
- 最佳化輕量級組件的效能。
何時使用類別組件
- 維護或更新較舊的 React 程式碼庫。
- 依賴遺留項目中的內建狀態和生命週期方法。
8.您應該選擇哪一個?
由 Hooks 提供支援的功能元件是 React 開發的現代標準。它們提供了一種更乾淨、更有效的方式來管理狀態、生命週期和副作用。類組件仍然與舊項目相關,但正在逐漸被替換。
9.結論
函數式元件和類別元件在 React 開發中都佔有一席之地,但 React Hooks 的興起已經將偏好轉向了函數式元件。對於新項目,建議選擇功能組件,它提供簡單性、靈活性和增強的性能。
以上是React 中的函數元件與類別元件:選擇哪一個?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
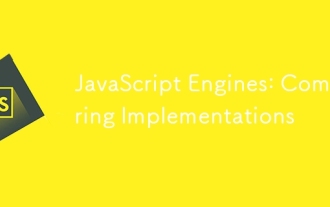
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
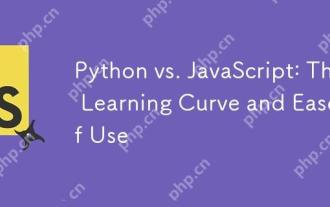
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
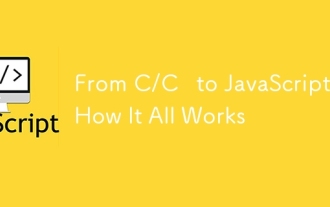
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
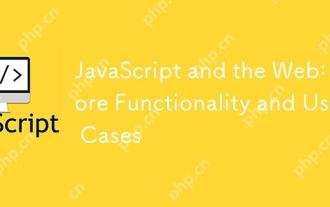
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
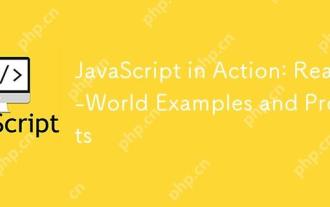
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
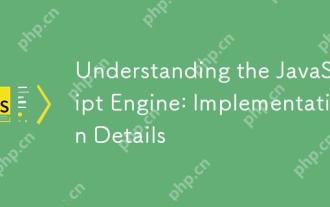
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。
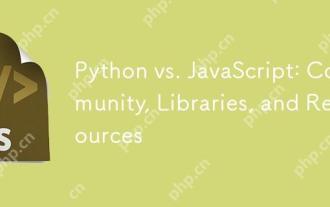
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
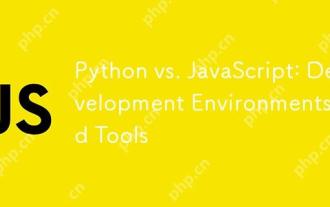
Python和JavaScript在開發環境上的選擇都很重要。 1)Python的開發環境包括PyCharm、JupyterNotebook和Anaconda,適合數據科學和快速原型開發。 2)JavaScript的開發環境包括Node.js、VSCode和Webpack,適用於前端和後端開發。根據項目需求選擇合適的工具可以提高開發效率和項目成功率。
