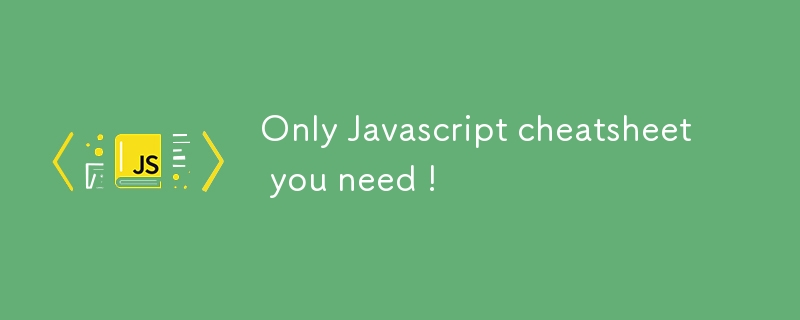
var、let 和 const 之間的區別
1. var、let 和 const 概述
Feature |
var |
let |
const |
Scope |
Function-scoped |
Block-scoped |
Block-scoped |
Re-declaration |
Allowed within the same scope |
Not allowed in the same scope |
Not allowed in the same scope |
Re-assignment |
Allowed |
Allowed |
Not allowed after initialization |
Initialization |
Can be declared without initialization |
Can be declared without initialization |
Must be initialized at the time of declaration |
Hoisting |
Hoisted but initialized to undefined
|
Hoisted but not initialized |
Hoisted but not initialized |
功能 |
var
|
讓 |
常數
|
標題>
Type |
Function Scope |
Block Scope |
var |
Variables are scoped to the enclosing function. |
Does not support block scope. A var inside a block (if, for, etc.) leaks into the enclosing function or global scope. |
let / const |
Not function-scoped. |
Variables are confined to the block they are declared in. |
範圍 |
函數範圍 |
區塊範圍 |
區塊範圍 |
重新聲明 |
同一範圍內允許 |
同一範圍內不允許 |
同一範圍內不允許 |
重新分配
|
允許 |
允許 |
初始化後不允許 |
初始化if (true) {
var x = 10;
let y = 20;
const z = 30;
}
console.log(x); // 10 (accessible because of function scope)
console.log(y); // ReferenceError (block-scoped)
console.log(z); // ReferenceError (block-scoped)
登入後複製 登入後複製 登入後複製 登入後複製
|
無需初始化即可宣告 |
無需初始化即可宣告 |
必須在宣告時初始化 |
吊裝
|
已提升但初始化為未定義
|
已提升但未初始化 |
已提升但未初始化 |
表>
2.範圍差異
Feature |
var |
let |
const |
Re-declaration |
Allowed |
Not allowed |
Not allowed |
Re-assignment |
Allowed |
Allowed |
Not allowed |
類型 |
函數範圍 |
區塊範圍 |
標題>
var |
變數的作用域為封閉函數。 |
不支援區塊作用域。區塊內的 var(if、for 等)會洩漏到封閉函數或全域作用域中。 |
let / const |
不是函數範圍的。 |
變數僅限於聲明它們的區塊內。 |
表>
範例:
3.重新聲明與重新分配
功能 |
var |
讓 |
常數 |
標題>
重新聲明 |
允許 |
不允許 |
不允許 |
重新分配 |
允許 |
允許 |
不允許 |
表>
範例:
if (true) {
var x = 10;
let y = 20;
const z = 30;
}
console.log(x); // 10 (accessible because of function scope)
console.log(y); // ReferenceError (block-scoped)
console.log(z); // ReferenceError (block-scoped)
登入後複製
登入後複製
登入後複製
登入後複製
4.提升行為
Type |
Hoisting Behavior |
var |
Hoisted to the top of the scope but initialized as undefined. |
let |
Hoisted but not initialized. Accessing it before declaration causes a ReferenceError. |
const |
Hoisted but not initialized. Must be initialized at the time of declaration. |
類型 |
提升行為 |
標題>
var
|
提升到範圍頂部,但初始化為未定義。 |
讓// Re-declaration
var a = 10;
var a = 20; // Allowed
let b = 30;
// let b = 40; // SyntaxError: Identifier 'b' has already been declared
const c = 50;
// const c = 60; // SyntaxError: Identifier 'c' has already been declared
// Re-assignment
a = 15; // Allowed
b = 35; // Allowed
// c = 55; // TypeError: Assignment to constant variable
登入後複製 登入後複製
|
已提升但未初始化。在聲明之前訪問它會導致引用錯誤。 |
常數
|
已提升但未初始化。必須在聲明時初始化。 |
表>
範例:
Feature |
let and const
|
Block Scope |
Both are confined to the block in which they are declared. |
No Hoisting Initialization |
Both are hoisted but cannot be accessed before initialization. |
Better Practice |
Preferred over var for predictable scoping. |
5. let 與 const 之間的相似之處
Scenario |
Recommended Keyword |
Re-declare variables or use function scope |
var (generally avoid unless necessary for legacy code). |
Variables that may change |
let (e.g., counters, flags, intermediate calculations). |
Variables that should not change |
const (e.g., configuration settings, fixed values). |
功能 |
let 和 const
標題>
塊範圍 |
兩者都僅限於聲明它們的區塊。 |
無提升初始化 |
兩者都被提升,但在初始化之前無法存取。 |
更好的實踐 |
在可預測範圍方面優於 var。 |
表>
6.何時使用哪一個?
場景 |
推薦關鍵字 |
標題>
重新宣告變數或使用函數作用域 |
var(除非遺留程式碼需要,否則通常避免使用)。 |
可能改變的變數 |
let(例如計數器、標誌、中間計算)。 |
不應更改的變數 |
const(例如配置設定、固定值)。 |
表>
7.吊掛說明
什麼是提升?
提升是 JavaScript 在編譯階段將宣告移到其作用域頂端的預設行為。
-
var:提升並初始化為未定義。
-
let / const:提升但未初始化。這會建立一個從區塊開始到遇到聲明的臨時死區(TDZ)。
為什麼吊掛要這樣運作?
-
編譯階段:
JavaScript 首先掃描程式碼為變數和函數宣告建立記憶體空間。在此階段:
-
var 變數被初始化為未定義。
-
let 和 const 變數被「提升」但未初始化,因此是 TDZ。
- 函數宣告全面提升。
-
執行階段:
JavaScript 開始逐行執行程式碼。變數和函數在此階段被賦值。
8.吊掛總結
Type |
Hoisting |
Access Before Declaration |
var |
Hoisted and initialized to undefined. |
Allowed but value is undefined. |
let |
Hoisted but not initialized. |
Causes a ReferenceError. |
const |
Hoisted but not initialized. |
Causes a ReferenceError. |
類型 |
吊掛 |
聲明前訪問 |
標題>
var |
提升並初始化為未定義。 |
允許,但值未定義。 |
讓 |
已提升但未初始化。 |
導致引用錯誤。 |
常數 |
已提升但未初始化。 |
導致引用錯誤。 |
表>
範例:
if (true) {
var x = 10;
let y = 20;
const z = 30;
}
console.log(x); // 10 (accessible because of function scope)
console.log(y); // ReferenceError (block-scoped)
console.log(z); // ReferenceError (block-scoped)
登入後複製
登入後複製
登入後複製
登入後複製
結論
- 對於不需要重新賦值的變量,盡可能使用 const。
- 對於需要在相同作用域內重新賦值的變數使用let。
- 避免 var 除非使用遺留程式碼或需要函數範圍的行為。
JavaScript 資料類型
JavaScript 有多種資料類型,分為 原始 和 非原始(參考) 類型。以下是每項的解釋,並附有範例和差異:
1. 原始資料型
原始型別是不可變的,這表示它們的值在建立後就無法變更。它們直接儲存在記憶體中。
Data Type |
Example |
Description |
String |
"hello", 'world'
|
Represents a sequence of characters (text). Enclosed in single (''), double (""), or backticks (). |
Number |
42, 3.14, NaN
|
Represents both integers and floating-point numbers. Includes NaN (Not-a-Number) and Infinity. |
BigInt |
123n, 9007199254740991n
|
Used for numbers larger than Number.MAX_SAFE_INTEGER (2^53 - 1). Add n to create a BigInt. |
Boolean |
true, false
|
Represents logical values, used in conditions to represent "yes/no" or "on/off". |
Undefined |
undefined |
Indicates a variable has been declared but not assigned a value. |
Null |
null |
Represents an intentional absence of value. Often used to reset or clear a variable. |
Symbol |
Symbol('id') |
Represents a unique identifier, mainly used as property keys for objects to avoid collisions. |
資料型別 |
範例 |
描述 |
標題>
字串 |
“你好世界'
|
表示字元序列(文字)。用單引號 ('')、雙引號 ("") 或反引號 () 括起來。 |
數字 |
42, 3.14, 南
|
表示整數和浮點數。包括 NaN(非數字)和無窮大。 |
BigInt |
123n, 9007199254740991n
|
用於大於 Number.MAX_SAFE_INTEGER (2^53 - 1) 的數字。新增 n 建立一個 BigInt。 |
布林值 |
真,假
|
表示邏輯值,用於條件中表示「是/否」或「開/關」。 |
未定義 |
未定義 |
表示變數已聲明但未賦值。 |
空 |
空 |
表示故意缺少值。通常用於重置或清除變數。 |
符號 |
符號('id') |
表示唯一標識符,主要用作物件的屬性鍵,避免碰撞。 |
表>
2. 非原始(參考)資料型
非原始類型是可變的並透過引用儲存。它們用於儲存資料集合或更複雜的實體。
Data Type |
Example |
Description |
Object |
{name: 'John', age: 30} |
A collection of key-value pairs. Keys are strings (or Symbols), and values can be any type. |
Array |
[1, 2, 3, "apple"] |
A list-like ordered collection of values. Access elements via index (e.g., array[0]). |
Function |
function greet() {} |
A reusable block of code that can be executed. Functions are first-class citizens in JavaScript. |
Date |
new Date() |
Represents date and time. Provides methods for manipulating dates and times. |
RegExp |
/pattern/ |
Represents regular expressions used for pattern matching and string searching. |
Map |
new Map() |
A collection of key-value pairs where keys can be of any type, unlike plain objects. |
Set |
new Set([1, 2, 3]) |
A collection of unique values, preventing duplicates. |
WeakMap |
new WeakMap() |
Similar to Map, but keys are weakly held, meaning they can be garbage-collected. |
WeakSet |
new WeakSet() |
Similar to Set, but holds objects weakly to prevent memory leaks. |
3. 原始類型和非原始類型之間的主要區別
Aspect |
Primitive Types |
Non-Primitive Types |
Mutability |
Immutable: Values cannot be changed. |
Mutable: Values can be modified. |
Storage |
Stored directly in memory. |
Stored as a reference to a memory location. |
Copy Behavior |
Copied by value (creates a new value). |
Copied by reference (points to the same object). |
Examples |
string, number, boolean, etc. |
object, array, function, etc. |
4. 特殊情況
運算子類型
-
typeof null:由於 JavaScript 中的歷史錯誤,返回“object”,但 null 不是物件。
-
typeof NaN:傳回“數字”,即使它意味著“非數字”。
-
typeof function:傳回“function”,它是物件的子類型。
動態打字
JavaScript 允許變數在執行時保存不同類型的值:
if (true) {
var x = 10;
let y = 20;
const z = 30;
}
console.log(x); // 10 (accessible because of function scope)
console.log(y); // ReferenceError (block-scoped)
console.log(z); // ReferenceError (block-scoped)
登入後複製
登入後複製
登入後複製
登入後複製
5. 每種資料類型的範例
原型
// Re-declaration
var a = 10;
var a = 20; // Allowed
let b = 30;
// let b = 40; // SyntaxError: Identifier 'b' has already been declared
const c = 50;
// const c = 60; // SyntaxError: Identifier 'c' has already been declared
// Re-assignment
a = 15; // Allowed
b = 35; // Allowed
// c = 55; // TypeError: Assignment to constant variable
登入後複製
登入後複製
非原始型
console.log(a); // undefined (hoisted)
var a = 10;
console.log(b); // ReferenceError (temporal dead zone)
let b = 20;
console.log(c); // ReferenceError (temporal dead zone)
const c = 30;
登入後複製
6. typeof結果總結
Expression |
Result |
typeof "hello" |
"string" |
typeof 42 |
"number" |
typeof 123n |
"bigint" |
typeof true |
"boolean" |
typeof undefined |
"undefined" |
typeof null |
"object" |
typeof Symbol() |
"symbol" |
typeof {} |
"object" |
typeof [] |
"object" |
typeof function(){} |
"function" |
表情 |
結果 |
標題>
類型“你好” |
“字串” |
類型42 |
“數字” |
類型123n |
“bigint” |
類型為true |
“布林值” |
類型未定義 |
“未定義” |
類型為空 |
“對象” |
符號類型() |
“符號” |
類型{} |
“對象” |
類型[] |
“對象” |
typeof 函數(){} |
“功能” |
表>
以上是您只需要 Javascript 備忘單!的詳細內容。更多資訊請關注PHP中文網其他相關文章!