如何在 C Lambda 中實現移動捕獲?
在Lambda 中移動捕獲
問題:
我們如何實現移動捕獲在C 111 lambda 中稱為右值引用?例如:
std::unique_ptr<int> myPointer(new int); std::function<void(void)> example = [std::move(myPointer)] { *myPointer = 4; };
答案:
C 14 中的廣義Lambda 捕獲
在廣義14 中,在廣義lambda capture 允許移動捕捉。此程式碼現在有效:
using namespace std; auto u = make_unique<some_type>(some, parameters); go.run([u = move(u)] { do_something_with(u); });
要將物件從lambda 移動到另一個函數,請使lambda 可變:
go.run([u = move(u)] mutable { do_something_with(std::move(u)); });
C 11 中移動捕獲的解決方法
輔助函數make_rref 可以促進移動捕捉。其實作如下:
#include <cassert> #include <memory> #include <utility> template <typename T> struct rref_impl { rref_impl() = delete; rref_impl(T&& x) : x{std::move(x)} {} rref_impl(rref_impl& other) : x{std::move(other.x)}, isCopied{true} { assert(other.isCopied == false); } rref_impl(rref_impl&& other) : x{std::move(other.x)}, isCopied{std::move(other.isCopied)} { } rref_impl& operator=(rref_impl other) = delete; T& operator&&() { return std::move(x); } private: T x; bool isCopied = false; }; template<typename T> rref_impl<T> make_rref(T&& x) { return rref_impl<T>{std::move(x)}; }
make_rref 的測試案例:
int main() { std::unique_ptr<int> p{new int(0)}; auto rref = make_rref(std::move(p)); auto lambda = [rref]() mutable -> std::unique_ptr<int> { return rref.move(); }; assert(lambda()); assert(!lambda()); }
在C 11 模擬廣義Lambda Capture
在C 11 中模擬廣義Lambda Capture#include <utility> template <typename T, typename F> class capture_impl { T x; F f; public: capture_impl(T&& x, F&& f) : x{std::forward<T>(x)}, f{std::forward<F>(f)} {} template <typename ...Ts> auto operator()(Ts&& ...args) -> decltype(f(x, std::forward<Ts>(args)...)) { return f(x, std::forward<Ts>(args)...); } template <typename ...Ts> auto operator()(Ts&& ...args) const -> decltype(f(x, std::forward<Ts>(args)...)) { return f(x, std::forward<Ts>(args)...); } }; template <typename T, typename F> capture_impl<T, F> capture(T&& x, F&& f) { return capture_impl<T, F>( std::forward<T>(x), std::forward<F>(f)); }
以上是如何在 C Lambda 中實現移動捕獲?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
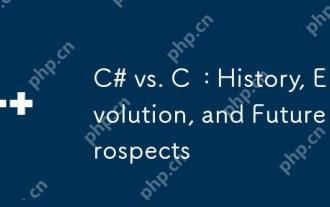
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
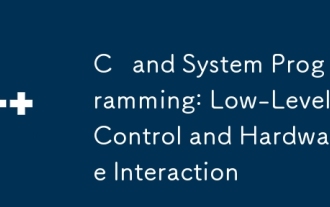
C 適合系統編程和硬件交互,因為它提供了接近硬件的控制能力和麵向對象編程的強大特性。 1)C 通過指針、內存管理和位操作等低級特性,實現高效的系統級操作。 2)硬件交互通過設備驅動程序實現,C 可以編寫這些驅動程序,處理與硬件設備的通信。
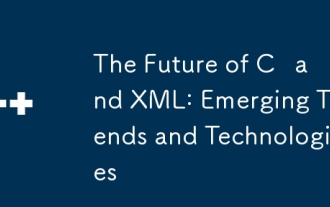
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
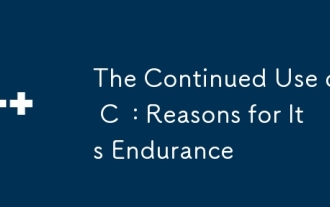
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
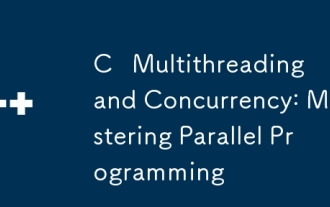
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
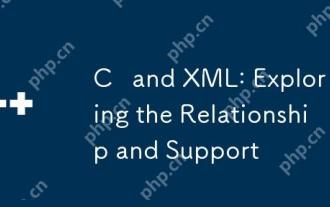
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
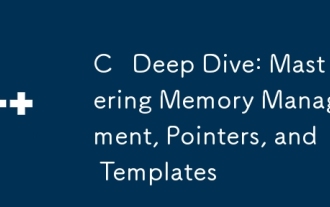
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
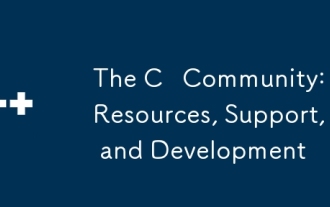
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
