在 Pytest-Django 中處理非託管模型
測試非託管模型的挑戰
在 Django 專案中,我們偶爾會遇到非託管模型,也就是元選項中沒有 Managed = True 的模型。這些模型可能會使測試變得棘手,特別是當您的測試設定涉及託管和非託管模型或多個資料庫的混合時(例如,一個包含託管模型,另一個包含非託管模型)。
這篇部落格文章探討了使用 pytest-django 測試非託管模型的方法,重點介紹了優點、缺點和解決方法,以幫助您有效管理這些場景。
方法 1:將所有模型標記為託管
在測試期間處理非託管模型的一種直接方法是將它們暫時標記為託管。具體方法如下:
# Add this to conftest.py @pytest.hookimpl(tryfirst=True) def pytest_runtestloop(): from django.apps import apps unmanaged_models = [] for app in apps.get_app_configs(): unmanaged_models += [m for m in app.get_models() if not m._meta.managed] for m in unmanaged_models: m._meta.managed = True
注意:要使此方法發揮作用,您需要在 pytest 設定(或 pytest.ini)中新增 --no-migrations 選項
參考:Stack Overflow
優點:
- 易於實作。
缺點:
- 跳過遷移測試,當多個開發人員處理同一個專案時,這可能會導致問題。
方法 2:手動建立非託管模型
或者,您可以在測試設定期間手動建立非託管模型。此方法可確保遷移經過測試:
@pytest.fixture(scope="session", autouse=True) def django_db_setup(django_db_blocker, django_db_setup): with django_db_blocker.unblock(): for _connection in connections.all(): with _connection.schema_editor() as schema_editor: setup_unmanaged_models(_connection, schema_editor) yield def setup_unmanaged_models(connection, schema_editor): from django.apps import apps unmanaged_models = [ model for model in apps.get_models() if model._meta.managed is False ] for model in unmanaged_models: if model._meta.db_table in connection.introspection.table_names(): schema_editor.delete_model(model) schema_editor.create_model(model)
優點:
- 將遷移作為測試案例的一部分進行測試。
缺點:
- 稍微複雜一點。
- transaction=True 不適用於此方法(在下一節中討論)。
了解事務測試
Pytest-django 提供了資料庫固定裝置:django_db 和 django_db(transaction=True)。它們的差異如下:
django_db: 在測試案例結束時回滾更改,這表示不會對資料庫進行實際提交。
django_db(transaction=True): 在每個測試案例後提交更改並截斷資料庫表。由於每次測試後只有託管模型會被截斷,這就是為什麼非託管模型在交易測試期間需要特殊處理的原因。
範例測試案例
@pytest.mark.django_db def test_example(): # Test case logic here pass @pytest.mark.django_db(transaction=True) def test_transactional_example(): # Test case logic here pass
使事務測試適用於非託管模型
由於交易測試僅截斷託管模型,因此我們可以在測試運行期間將非託管模型修改為託管模型。這確保它們包含在截斷中:
# Add this to conftest.py @pytest.hookimpl(tryfirst=True) def pytest_runtestloop(): from django.apps import apps unmanaged_models = [] for app in apps.get_app_configs(): unmanaged_models += [m for m in app.get_models() if not m._meta.managed] for m in unmanaged_models: m._meta.managed = True
使用 on_commit Hooks 避免 transaction=True (如果可能的話)
在涉及 on_commit 掛鉤的場景中,您可以透過直接擷取和執行 on_commit 回呼來避免使用交易測試,使用 pytest-django(>= v.4.4) 中的固定裝置 django_capture_on_commit_callbacks:
@pytest.fixture(scope="session", autouse=True) def django_db_setup(django_db_blocker, django_db_setup): with django_db_blocker.unblock(): for _connection in connections.all(): with _connection.schema_editor() as schema_editor: setup_unmanaged_models(_connection, schema_editor) yield def setup_unmanaged_models(connection, schema_editor): from django.apps import apps unmanaged_models = [ model for model in apps.get_models() if model._meta.managed is False ] for model in unmanaged_models: if model._meta.db_table in connection.introspection.table_names(): schema_editor.delete_model(model) schema_editor.create_model(model)
參考
- pytest-django 文件
- Stack Overflow:測試非託管模型
您還有其他處理非託管模型的方法或技巧嗎?在下面的評論中分享它們!
以上是在 Pytest-Django 中處理非託管模型的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
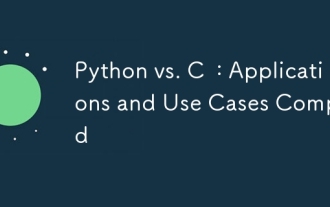
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
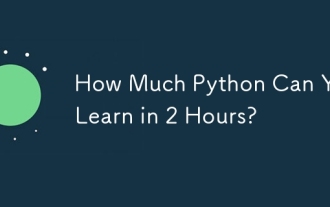
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
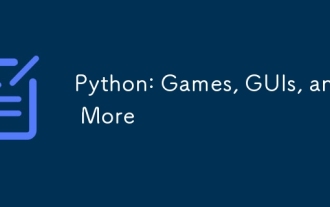
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
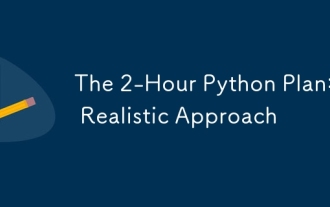
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
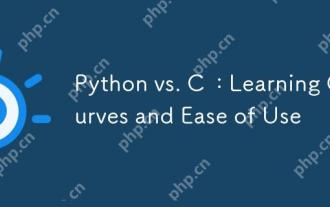
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
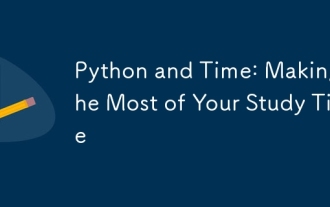
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
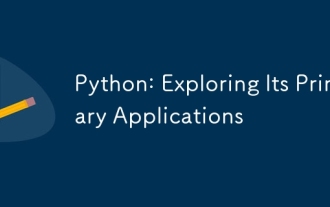
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
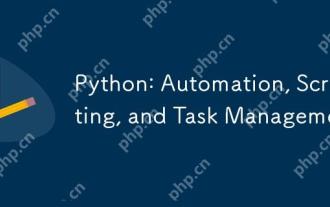
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
