如何使用 C# 中的自訂比較器按字母順序和數字對字串進行排序?
透過自訂比較按字母順序和數字對字串進行排序
此問題提出了在考慮數值的同時按字母順序對字串數字數組進行排序的挑戰。為此,必須實作自訂比較器來覆寫預設的字串比較。
實作詳細資訊
以下的程式碼示範如何實作此排序:
using System; using System.Collections.Generic; using System.Linq; namespace StringSort { class Program { static void Main(string[] args) { // Input array of string numbers string[] things = new string[] { "105", "101", "102", "103", "90" }; // Sort using custom comparer IEnumerable<string> sortedThings = things.OrderBy(x => x, new SemiNumericComparer()); // Print sorted array foreach (var thing in sortedThings) { Console.WriteLine(thing); } } public class SemiNumericComparer : IComparer<string> { // Check if a string is numeric public bool IsNumeric(string value) { return int.TryParse(value, out _); } // Compare two strings public int Compare(string s1, string s2) { const int S1GreaterThanS2 = 1; const int S2GreaterThanS1 = -1; // Check if both strings are numeric var IsNumeric1 = IsNumeric(s1); var IsNumeric2 = IsNumeric(s2); if (IsNumeric1 && IsNumeric2) { int i1 = Convert.ToInt32(s1); int i2 = Convert.ToInt32(s2); return i1.CompareTo(i2); } // If one string is numeric and the other is not, consider the numeric string greater if (IsNumeric1) return S2GreaterThanS1; if (IsNumeric2) return S1GreaterThanS2; // Otherwise, perform a case-insensitive alphabetical comparison return string.Compare(s1, s2, true, CultureInfo.InvariantCulture); } } } }
自訂比較器Logic
SemiNumericComparer 類別定義字串的比較邏輯。它首先使用 IsNumeric 方法檢查兩個字串是否都是數字。如果兩者都是數字,則會對它們進行數字比較。如果只有一個字串是數字,則認為該數字字串較大。對於非數字字串,它執行不區分大小寫的字母比較。
將此比較器與 Enumerable.OrderBy 一起使用時,字串數字數組將首先按數字值排序,然後按字母順序對非數字字串排序。上面範例的輸出將會是:
90 101 102 103 105
以上是如何使用 C# 中的自訂比較器按字母順序和數字對字串進行排序?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
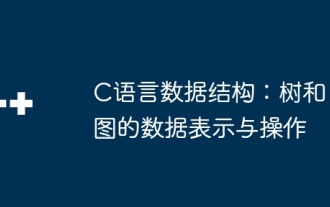
C語言數據結構:樹和圖的數據表示與操作樹是一個層次結構的數據結構由節點組成,每個節點包含一個數據元素和指向其子節點的指針二叉樹是一種特殊類型的樹,其中每個節點最多有兩個子節點數據表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作創建樹遍歷樹(先序、中序、後序)搜索樹插入節點刪除節點圖是一個集合的數據結構,其中的元素是頂點,它們通過邊連接在一起邊可以是帶權或無權的數據表示鄰
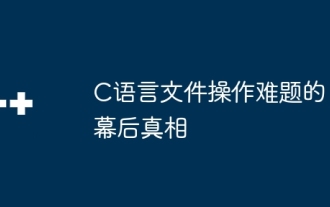
文件操作難題的真相:文件打開失敗:權限不足、路徑錯誤、文件被佔用。數據寫入失敗:緩衝區已滿、文件不可寫、磁盤空間不足。其他常見問題:文件遍歷緩慢、文本文件編碼不正確、二進製文件讀取錯誤。
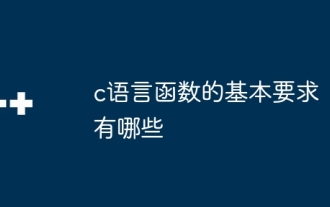
C語言函數是代碼模塊化和程序搭建的基礎。它們由聲明(函數頭)和定義(函數體)組成。 C語言默認使用值傳遞參數,但也可使用地址傳遞修改外部變量。函數可以有返回值或無返回值,返回值類型必須與聲明一致。函數命名應清晰易懂,使用駝峰或下劃線命名法。遵循單一職責原則,保持函數簡潔性,以提高可維護性和可讀性。
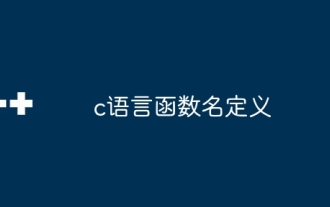
C語言函數名定義包括:返回值類型、函數名、參數列表和函數體。函數名應清晰、簡潔、統一風格,避免與關鍵字衝突。函數名具有作用域,可在聲明後使用。函數指針允許將函數作為參數傳遞或賦值。常見錯誤包括命名衝突、參數類型不匹配和未聲明的函數。性能優化重點在函數設計和實現上,而清晰、易讀的代碼至關重要。
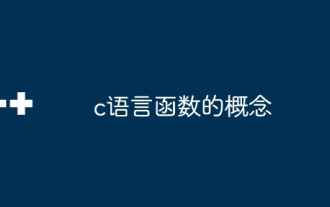
C語言函數是可重複利用的代碼塊,它接收輸入,執行操作,返回結果,可將代碼模塊化提高可複用性,降低複雜度。函數內部機制包含參數傳遞、函數執行、返回值,整個過程涉及優化如函數內聯。編寫好的函數遵循單一職責原則、參數數量少、命名規範、錯誤處理。指針與函數結合能實現更強大的功能,如修改外部變量值。函數指針將函數作為參數傳遞或存儲地址,用於實現動態調用函數。理解函數特性和技巧是編寫高效、可維護、易理解的C語言程序的關鍵。
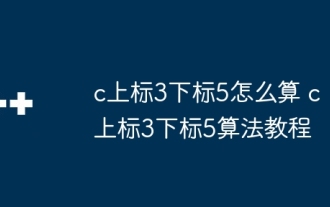
C35 的計算本質上是組合數學,代表從 5 個元素中選擇 3 個的組合數,其計算公式為 C53 = 5! / (3! * 2!),可通過循環避免直接計算階乘以提高效率和避免溢出。另外,理解組合的本質和掌握高效的計算方法對於解決概率統計、密碼學、算法設計等領域的許多問題至關重要。
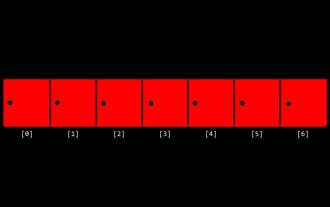
算法是解決問題的指令集,其執行速度和內存佔用各不相同。編程中,許多算法都基於數據搜索和排序。本文將介紹幾種數據檢索和排序算法。線性搜索假設有一個數組[20,500,10,5,100,1,50],需要查找數字50。線性搜索算法會逐個檢查數組中的每個元素,直到找到目標值或遍歷完整個數組。算法流程圖如下:線性搜索的偽代碼如下:檢查每個元素:如果找到目標值:返回true返回falseC語言實現:#include#includeintmain(void){i
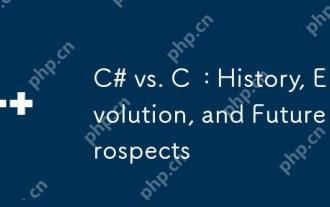
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
