Python中如何確保使用者輸入有效?
請求有效的使用者輸入直至收到
請求使用者輸入時,優雅地處理無效回應而不是崩潰或接受不正確的值至關重要。以下技術可確保獲得有效的輸入:
嘗試/排除異常輸入
使用 try/ except 擷取無法解析的特定輸入。例如:
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue break
附加規則的自訂驗證
有時,可以解析的輸入可能仍然不滿足某些條件。您可以新增自訂驗證邏輯來拒絕特定值:
while True: data = input("Enter a positive number: ") if int(data) < 0: print("Invalid input. Please enter a positive number.") continue break
結合異常處理和自訂驗證
結合這兩種技術來處理無效解析和自訂驗證規則:
while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue if age < 0: print("Invalid age. Please enter a positive number.") continue break
封裝在函數
要重複使用自定義輸入驗證邏輯,請將其封裝在函數中:
def get_positive_age(): while True: try: age = int(input("Please enter your age: ")) except ValueError: print("Sorry, that's not a valid age.") continue if age < 0: print("Invalid age. Please enter a positive number.") continue return age
進階輸入清理
您可以建立一個更通用的輸入函數來處理各種驗證場景:
def get_valid_input(prompt, type_=None, min_=None, max_=None, range_=None): while True: try: value = type_(input(prompt)) except ValueError: print(f"Invalid input type. Expected {type_.__name__}.") continue if max_ is not None and value > max_: print(f"Value must be less than or equal to {max_}.") continue if min_ is not None and value < min_: print(f"Value must be greater than or equal to {min_}.") continue if range_ is not None and value not in range_: template = "Value must be {}." if len(range_) == 1: print(template.format(*range_)) else: expected = " or ".join(( ", ".join(str(x) for x in range_[:-1]), str(range_[-1]) )) print(template.format(expected)) else: return value
此函數可讓您指定使用者輸入的資料類型、範圍和其他限制。
以上是Python中如何確保使用者輸入有效?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
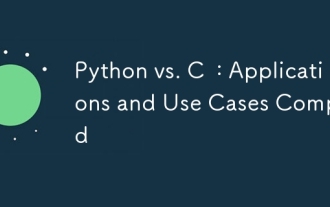
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
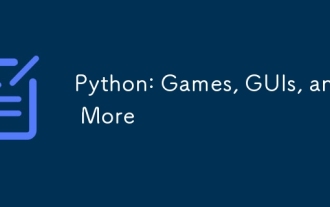
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
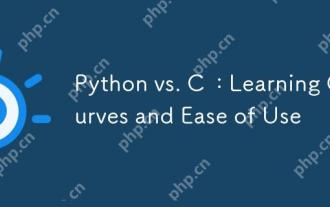
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
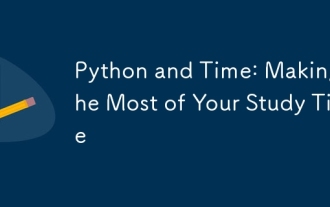
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
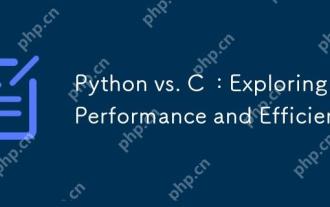
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
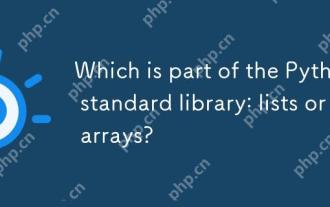
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
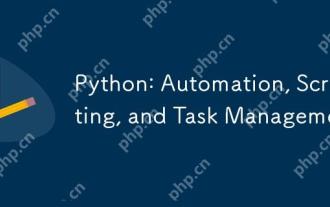
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
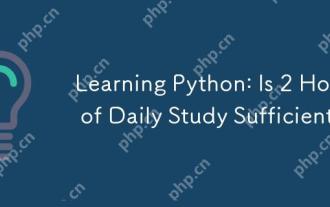
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
