逐步電子郵件驗證 JavaScript 教學:最佳實踐和程式碼範例
JavaScript 中的電子郵件驗證涉及兩個基本元件:客戶端格式驗證和透過確認連結的伺服器端驗證。這個綜合指南提供了可用於生產的程式碼範例和安全最佳實踐,以便在您的應用程式中實現強大的電子郵件驗證系統。
正確的電子郵件驗證對於維持電子郵件的送達率並保護您的應用程式免受無效或惡意電子郵件提交的影響至關重要。用戶端驗證可提供即時的使用者回饋,而伺服器端驗證可確保電子郵件實際存在並屬於使用者。
在深入實施之前,請確保您對以下內容有基本了解:
- JavaScript (ES6)
- 正規表示式
- Node.js(用於伺服器端實作)
- 基本電子郵件協定概念
從根本上了解電子郵件驗證的工作原理有助於您實施更安全、更有效率的解決方案。現代電子郵件驗證通常採用多個驗證層:
在實施電子郵件驗證最佳實務時,平衡安全性與使用者體驗至關重要。我們的實作將專注於創建一個強大的系統,防止無效電子郵件,同時保持流暢的使用者體驗。
在本教程中,我們將建立一個完整的電子郵件驗證系統,其中包括:
- 使用現代 JavaScript 模式進行客戶端驗證
- 透過安全性令牌產生進行伺服器端驗證
- 防範常見安全漏洞
- 確保可靠性的測試策略
提供的程式碼範例已可用於生產,並遵循當前的安全最佳實踐,允許您直接在應用程式中實現它們,同時保持根據您的特定需求進行客製化的靈活性。
在實施電子郵件驗證最佳實務時,平衡安全性與使用者體驗至關重要。強大的電子郵件驗證系統可以防禦各種威脅,同時保持使用者參與:
首先,客戶端驗證提供即時回饋,防止在伺服器提交之前出現明顯的格式錯誤。這種方法可以透過在流程早期發現錯誤來減少伺服器負載並改善使用者體驗。然而,僅客戶端驗證不足以保護您的應用程式。
伺服器端驗證透過執行更深入的驗證檢查來新增關鍵的安全層。這包括網域驗證和實施安全確認工作流程。客戶端和伺服器端驗證的結合創建了一個全面的安全框架。
您需要解決的常見安全挑戰包括:
- 防止自動表單提交
- 防止電子郵件確認連結利用
- 安全代幣產生與管理
- 速率限制以防止濫用
實施電子郵件驗證時,請考慮影響應用程式安全性和使用者體驗的以下關鍵因素:
現代 JavaScript 框架和函式庫可以顯著簡化實作過程。但是,了解基本原理可確保您可以根據您的特定要求調整解決方案,並透過更好的電子郵件驗證來改善您的行銷活動。
我們將探索的實作方法旨在隨著應用程式的成長而擴展。無論您是建立小型 Web 應用程式還是大型系統,這些模式都為可靠的電子郵件驗證提供了堅實的基礎。
遵循本教學課程,您將建立一個驗證系統:
- 使用現代 JavaScript 技術驗證電子郵件格式
- 實現安全的伺服器端驗證
- 處理邊緣情況和潛在的安全威脅
- 提供流暢的使用者體驗
- 隨著應用程式的成長而有效擴展
讓我們從實作客戶端驗證開始,我們將探索現代 JavaScript 模式以進行有效的電子郵件格式驗證。
客戶端電子郵件驗證
用戶端電子郵件驗證在表單提交之前向使用者提供即時回饋,從而增強使用者體驗並減少伺服器負載。讓我們使用現代 JavaScript 實踐和經過驗證的正規表示式模式來實現一個強大的驗證系統。
正規表示式模式驗證
電子郵件驗證的基礎始於可靠的正規表示式模式。雖然沒有任何正規表示式模式可以保證 100% 的準確性,但我們將使用一種平衡來驗證徹底性與實際使用的模式:
const emailRegex = /^[a-zA-Z0-9.!#$%&'* /=?^_{|}~-] @[a-zA-Z0-9-] (?:. [a-zA-Z0-9-] )*$/;`
此模式依據 RFC 5322 標準驗證電子郵件地址,檢查:
- 本地部分的有效字元(@之前)
- 存在單一@符號
- 有效網域結構
- 正確使用點和特殊字元
建構驗證函數
讓我們建立一個全面的驗證功能,不僅檢查格式,還提供有意義的回饋。此方法符合電子郵件格式最佳實務:
`函數 validateEmail(電子郵件) {
// 刪除前導/尾隨空格
const trimmEmail = email.trim();
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
}`
表單整合與錯誤處理
將驗證功能與您的 HTML 表單整合以提供即時回饋。此實作遵循當前驗證最佳實踐:
`document.addEventListener('DOMContentLoaded', () => {
const emailInput = document.getElementById('email');
const errorDisplay = document.getElementById('錯誤訊息');
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
});
// Debounce 函數以防止過多的驗證呼叫
函數 debounce(func, 等待) {
讓超時;
回傳函數executeFunction(...args) {
const 稍後 = () => {
清除超時(超時);
func(...args);
};
清除超時(超時);
timeout = setTimeout(稍後,等待);
};
}`
這是對應的 HTML 結構:
類型=“電子郵件”
> 名稱=“電子郵件”
必填
自動完成=“電子郵件”
>
提交按鈕>
表格>
此實作包括幾個重要功能:
- 去抖驗證以提高效能
- 使用 CSS 類別提供清晰的視覺回饋
- 可存取的錯誤訊息
- 支援自動完成
- 使用 novalidate 屬性漸進式增強
請記住,客戶端驗證只是第一道防線。始終實作伺服器端驗證,我們將在下一節中介紹。
伺服器端電子郵件驗證
用戶端驗證可提供即時回饋,而伺服器端驗證可確保電子郵件的真實性和使用者所有權。本節示範如何使用 Node.js 和 Express 實作安全的電子郵件驗證系統。
設定確認系統
首先,讓我們為我們的驗證系統設定必要的依賴項和配置:
`const express = require('express');
const crypto = require('crypto');
const nodemailer = require('nodemailer');
const mongoose = require('mongoose');
// 環境配置
require('dotenv').config();
const app = express();
app.use(express.json());
// 電子郵件傳輸設定
const Transporter = nodemailer.createTransport({
主機:process.env.SMTP_HOST,
連接埠:process.env.SMTP_PORT,
安全:正確,
授權:{
使用者:process.env.SMTP_USER,
通過:process.env.SMTP_PASS
}
});`
使用這些基本參數來設定您的電子郵件服務,以確保正確的電子郵件送達率:
代幣產生與管理
使用加密函數實現安全令牌產生:
`類 VerificationToken {
靜態非同步生成() {
const token = crypto.randomBytes(32).toString('hex');
const expiresAt = new Date(Date.now() 24 * 60 * 60 * 1000); // 24 小時
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
}`
建立驗證端點
設定必要的 API 端點來處理驗證要求。此實作遵循經過驗證的驗證方法:
`// 要求電子郵件驗證
app.post('/api/verify-email', async (req, res) => {
嘗試{
const { email } = req.body;
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
電子郵件驗證
請點擊下面的連結來驗證您的電子郵件地址:
驗證電子郵件
此連結將在 24 小時後過期。
});
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
});
// 確認電子郵件驗證
app.get('/api/confirm-verification', async (req, res) => {
嘗試{
const { token } = req.query;
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
});`
此實作包括幾個安全功能:
- 加密安全的令牌產生
- 令牌過期處理
- 速率限制(實現如下圖)
- 錯誤處理與日誌記錄
- 包含 HTML 內容的安全電子郵件範本
添加速率限制以防止濫用:
`const ratesLimit = require('express-rate-limit');
const verifyLimiter = ratesLimit({
windowMs: 60 * 60 * 1000, // 1 小時
max: 5, // 每個 IP 5 個請求
message: '驗證請求太多。請稍後再試。 '
});
app.use('/api/verify-email', verifyLimiter);`
請記住對您的驗證系統實施適當的錯誤處理和監控,以保持可靠性和安全性。
安全最佳實務
實施強大的安全措施對於保護您的電子郵件驗證系統免受各種威脅至關重要。本節涵蓋基本的安全實踐,以確保您的實施保持安全可靠,同時保持高交付率。
令牌安全措施
安全的代幣產生和管理構成了可靠驗證系統的基礎。實施這些關鍵的安全措施:
`類 TokenManager {
靜態異步generateSecureToken() {
// 使用 crypto.randomBytes 取得加密安全令牌
const tokenBuffer =等待 crypto.randomBytes(32);
return { token, expiresAt }; } static async verify(token) { const user = await User.findOne({ 'verification.token': token, 'verification.expiresAt': { $gt: Date.now() } }); return user; }
}`
防止系統濫用
實施全面的速率限制和監控,以防止垃圾郵件機器人和濫用:
`const ratesLimit = require('express-rate-limit');
const RedisStore = require('rate-limit-redis');
// 設定分級限速
constrateLimitConfig = {
// 基於IP的限制
ipLimiter: {
windowMs: 60 * 60 * 1000, // 1 小時
max: 5, // 每個 IP 的請求
標準標頭:true,
遺留標題:假,
處理程序:(req,res)=> {
res.status(429).json({
錯誤:'超出速率限制。請稍後重試。 ',
retryAfter: Math.ceil(req.rateLimit.resetTime / 1000)
});
}
},
// Check if email already verified const existingUser = await User.findOne({ email, verified: true }); if (existingUser) { return res.status(400).json({ error: 'Email already verified' }); } // Generate verification token const { token, expiresAt } = await VerificationToken.generate(); // Store or update user with verification token await User.findOneAndUpdate( { email }, { email, verification: { token, expiresAt }, verified: false }, { upsert: true } ); // Send verification email const verificationLink = \`${process.env.APP_URL}/verify-email?token=${token}\`; await transporter.sendMail({ from: process.env.SMTP_FROM, to: email, subject: 'Verify Your Email Address', html: \``
};
// 應用速率限制中間件
app.use('/api/verify-email',rateLimit(rateLimitConfig.ipLimiter));
app.use('/api/verify-email',rateLimit(rateLimitConfig.globalLimiter));`
實施這些額外的安全措施來防止常見漏洞:
這是實現安全令牌加密的範例:
`類 TokenEncryption {
靜態非同步 encryptToken(token) {
const 演算法 = 'aes-256-gcm';
const key = Buffer.from(process.env.ENCRYPTION_KEY, 'hex');
const iv = crypto.randomBytes(12);
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
}`
使用日誌記錄和分析來監控您的驗證系統是否存在可疑模式:
`const Winston = require('winston');
const logger = winston.createLogger({
等級:'訊息',
格式:winston.format.json(),
運輸:[
新的winston.transports.File({
檔案名稱:'驗證錯誤.log',
等級:'錯誤'
}),
新的winston.transports.File({
檔案名稱:'驗證組合.log'
})
]
});
// 監控驗證嘗試
app.use('/api/verify-email', (req, res, next) => {
logger.info('驗證嘗試', {
ip: 請求.ip,
電子郵件:req.body.email,
時間戳:new Date(),
userAgent: req.headers['user-agent']
});
下一個();
});`
定期檢視您的安全措施,並根據新出現的威脅和電子郵件安全方面的最佳實踐進行更新。
測試與部署
適當的測試和部署程序可確保您的電子郵件驗證系統保持可靠並保持高送達率。本節涵蓋基本的測試策略和部署注意事項。
測試策略
使用 Jest 或 Mocha 實作全面測試來驗證您的電子郵件驗證系統:
`describe('電子郵件驗證系統', () => {
描述('格式驗證', () => {
test('應驗證正確的電子郵件格式', () => {
const validEmails = [
'user@domain.com',
'user.name@domain.com',
'使用者標籤@domain.com'
];
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
});`
常見問題及解決方案
在實施電子郵件驗證時解決這些常見挑戰:
對生產環境實施監控與日誌記錄:
`const 監控 = {
// 追蹤驗證嘗試
trackVerification: async (email, success, error = null) =>; {
等待 VerificationMetric.create({
電子郵件,
成功,
錯誤,
時間戳:new Date()
});
},
// Basic structure check if (!trimmedEmail) { return { isValid: false, error: 'Email address is required' }; } // Length validation if (trimmedEmail.length > 254) { return { isValid: false, error: 'Email address is too long' }; } // RegEx validation if (!emailRegex.test(trimmedEmail)) { return { isValid: false, error: 'Please enter a valid email address' }; } // Additional checks for common mistakes if (trimmedEmail.includes('..')) { return { isValid: false, error: 'Invalid email format: consecutive dots not allowed' }; } return { isValid: true, error: null };
};`
遵循這些部署最佳實務以確保系統可靠性:
- 使用特定於環境的配置
- 實現優雅的錯誤處理
- 設定自動監控
- 配置適當的日誌記錄等級
- 建立備份和還原程序
定期維護和監控有助於在問題影響使用者之前發現並解決問題:
`// 實現健康檢查端點
app.get('/health', async (req, res) => {
嘗試{
const 指標=等待監控.healthCheck();
常量狀態=指標.成功率> = 0.95? '健康' : '降級';
emailInput.addEventListener('input', debounce(function(e) { const result = validateEmail(e.target.value); if (!result.isValid) { errorDisplay.textContent = result.error; emailInput.classList.add('invalid'); emailInput.classList.remove('valid'); } else { errorDisplay.textContent = ''; emailInput.classList.add('valid'); emailInput.classList.remove('invalid'); } }, 300));
});`
常見問題
為什麼我應該同時實作客戶端和伺服器端電子郵件驗證?
客戶端驗證可提供即時的使用者回饋,並透過儘早發現明顯的格式錯誤來減少伺服器負載。然而,伺服器端驗證對於確認電子郵件的存在和所有權至關重要。使用兩者創建了一個全面的驗證系統,可以在保持安全性的同時改善使用者體驗。為了獲得最佳結果,請實施用戶端驗證以取得即時回饋,並實施伺服器端驗證以進行實際電子郵件確認。
如何防止驗證令牌濫用?
透過實施以下安全措施來防止令牌濫用:
- 使用加密安全令牌產生
- 設定適當的令牌過期時間(通常為 24 小時)
- 對驗證請求實施速率限制
- 監控並記錄驗證嘗試
- 驗證成功後使令牌失效
處理電子郵件驗證錯誤的最佳方法是什麼?
實作全面的錯誤處理策略,包括:
- 清晰、使用者友善的錯誤訊息
- 正確記錄所有驗證嘗試
- 暫時失敗的重試機制
- 替代驗證方法作為備份
此外,請遵循電子郵件驗證最佳實踐,以最大限度地減少錯誤發生。
驗證令牌應該多久過期一次?
驗證令牌通常應在 24 小時後過期,以平衡安全性與使用者便利性。此時間範圍為使用者提供了足夠的機會來完成驗證,同時限制了潛在令牌濫用的視窗。為了增強安全性,請考慮為需要更多時間的使用者實施更短的過期時間(4-8 小時)和令牌刷新機制。
我應該實施即時電子郵件驗證嗎?
即時驗證可以增強使用者體驗,但應謹慎實施。使用去抖客戶端驗證進行即時格式檢查,但避免即時伺服器端驗證以防止過多的 API 呼叫。相反,當使用者提交表單時,執行全面的電子郵件送達率檢查。
以上是逐步電子郵件驗證 JavaScript 教學:最佳實踐和程式碼範例的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
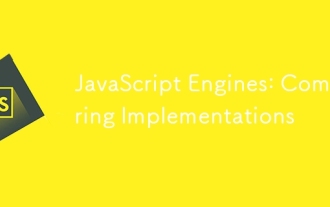
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
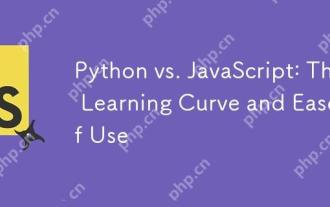
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
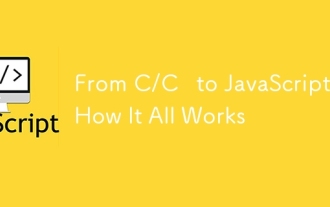
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
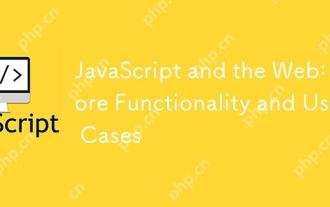
JavaScript在Web開發中的主要用途包括客戶端交互、表單驗證和異步通信。 1)通過DOM操作實現動態內容更新和用戶交互;2)在用戶提交數據前進行客戶端驗證,提高用戶體驗;3)通過AJAX技術實現與服務器的無刷新通信。
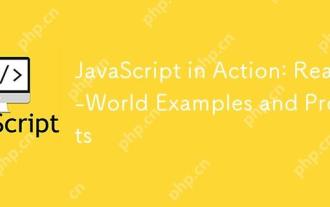
JavaScript在現實世界中的應用包括前端和後端開發。 1)通過構建TODO列表應用展示前端應用,涉及DOM操作和事件處理。 2)通過Node.js和Express構建RESTfulAPI展示後端應用。
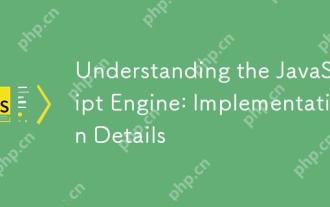
理解JavaScript引擎內部工作原理對開發者重要,因為它能幫助編寫更高效的代碼並理解性能瓶頸和優化策略。 1)引擎的工作流程包括解析、編譯和執行三個階段;2)執行過程中,引擎會進行動態優化,如內聯緩存和隱藏類;3)最佳實踐包括避免全局變量、優化循環、使用const和let,以及避免過度使用閉包。
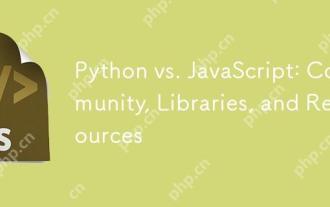
Python和JavaScript在社區、庫和資源方面的對比各有優劣。 1)Python社區友好,適合初學者,但前端開發資源不如JavaScript豐富。 2)Python在數據科學和機器學習庫方面強大,JavaScript則在前端開發庫和框架上更勝一籌。 3)兩者的學習資源都豐富,但Python適合從官方文檔開始,JavaScript則以MDNWebDocs為佳。選擇應基於項目需求和個人興趣。
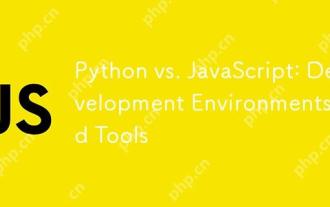
Python和JavaScript在開發環境上的選擇都很重要。 1)Python的開發環境包括PyCharm、JupyterNotebook和Anaconda,適合數據科學和快速原型開發。 2)JavaScript的開發環境包括Node.js、VSCode和Webpack,適用於前端和後端開發。根據項目需求選擇合適的工具可以提高開發效率和項目成功率。
