我從頭開始建立了終極教育網站 — 第 5 天
昨天,我們研究了週期性屬性,這是一篇特定的文章。今天,讓我們回到實際的網站設計和頁面。
我們將創建有機化學頁面。我們將從設定基本的 HTML 結構開始,包括互動式元素的佔位符。
第 23 小時:建立有機化學頁面
首先,我在 Chemistry 資料夾中建立了organic.html。這是基本結構:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Neuron IQ - Organic Chemistry</title> <meta name="description" content="Explore the fascinating world of organic chemistry with interactive articles, quizzes, and 3D molecule visualizations."> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600&display=swap" rel="stylesheet"> <!-- Favicon --> <link rel="icon" type="image/png" href="/favicon.png"> <!-- Stylesheets (Consider using a CSS preprocessor like Sass for better organization) --> <link rel="stylesheet" href="css/style-main.css"> <link rel="stylesheet" href="css/style.css"> <link rel="stylesheet" href="css/specific-chemistry.css"> </head> <body> <header> <nav> <div> <p>I've added basic structure, links to the main stylesheets, and CDN link to a 3D molecule viewer library molGL. I've also added a basic header and footer, consistent with our previous pages, and a button for hamburger menu.</p> <p>Now, I'll add the main content area, divided into sections for articles, key topics, and an interactive molecule viewer.<br> </p> <pre class="brush:php;toolbar:false"><section> <p>This code adds a three-column layout. The left column will house the article list with a search bar. The middle column will have a hero section with a call-to-action button and a grid of topic cards with images. Finally, the right column will feature the interactive 3D molecule viewer.</p> <p>I've also added placeholders for images within the topic cards (images/hydrocarbons.jpg, etc.). We'll need to replace these with actual images later.</p> <h2> Hour 24: Adding JavaScript for Interactivity </h2> <p>Now, let's add some basic JavaScript to handle the hamburger menu, the article search functionality, and the 3D molecule viewer. I'll add the following inside the <script> tag at the end of the body:<br> <pre class="brush:php;toolbar:false"> // Hamburger Menu Toggle const hamburgerBtn = document.getElementById('hamburger-btn'); const navMenu = document.getElementById('nav-menu'); hamburgerBtn.addEventListener('click', () => { navMenu.classList.toggle('show'); }); // Article Search Functionality (Basic Example) const articleSearch = document.getElementById('article-search'); const articleLinks = document.getElementById('article-links').querySelectorAll('a'); articleSearch.addEventListener('input', () => { const searchTerm = articleSearch.value.toLowerCase(); articleLinks.forEach(link => { const linkText = link.textContent.toLowerCase(); if (linkText.includes(searchTerm)) { link.style.display = 'block'; } else { link.style.display = 'none'; } }); }); // 3D Molecule Viewer (MolGL Example) const molContainer = document.getElementById('mol-container'); const moleculeSelect = document.getElementById('molecule-select'); // Initialize MolGL viewer let viewer = new MolGL({ container: molContainer, style: { stick: {}, sphere: { scale: 0.3 } } }); // Load a default molecule viewer.load('pdb:1crn'); // Example: Load a PDB structure // Change molecule on selection moleculeSelect.addEventListener('change', () => { const selectedMolecule = moleculeSelect.value; // We'll need to map molecule names to appropriate data sources (e.g., PDB IDs, SDF files) if (selectedMolecule === 'methane') { viewer.load('sdf:./molecules/methane.sdf'); // Example: Load from an SDF file } else if (selectedMolecule === 'ethanol') { viewer.load('pdb:1etn'); } else if (selectedMolecule === 'benzene') { viewer.load('sdf:./molecules/benzene.sdf'); } });
這是這個 JavaScript 的作用:
- 漢堡選單切換:為漢堡按鈕新增事件偵聽器以切換導覽選單上的顯示類,使其在小螢幕上可見或隱藏。
- 文章搜尋:實現簡單的搜尋功能,根據使用者在搜尋欄中的輸入過濾文章連結。
-
3D 分子檢視器:
- 在 mol-container 元素中初始化 MolGL 檢視器實例。
- 從外部連結載入 PDB 格式的預設分子(crambin 蛋白,1crn)。
- 將事件偵聽器新增至分子選擇下拉清單中,以便在使用者進行選擇時載入不同的分子。
- 使用佔位符分子資料來源(./molecules/macet.sdf 等)。我們需要以正確的格式(SDF、PDB 等)提供實際的分子數據,並相應地繪製分子名稱。
即時頁面在這裡,如果你想看:
有機化學 - NeuronIQ
第 25 小時:建立無機化學頁面
讓我們建立一個無機化學的新頁面,其結構與有機化學頁面類似。
首先,我在Chemistry資料夾中建立了organic.html,其基本結構如下:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Neuron IQ - Inorganic Chemistry</title> <link rel="stylesheet" href="style.css"> <link rel="preconnect" href="https://fonts.googleapis.com"> <link rel="preconnect" href="https://fonts.gstatic.com" crossorigin> <link href="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;500;600&display=swap" rel="stylesheet"> <link rel="stylesheet" href="style-main.css"> <link rel="stylesheet" href="specific-chemistry.css"> </head> <body> <header> <nav> <div> <p>This code sets up the HTML for the page with:</p> <ul> <li> A basic header with navigation links.</li> <li> An aside element for the article list.</li> <li> A main content area with a heading, introductory text, and a grid for key topics.</li> <li> Placeholder links for articles and topics.</li> <li> A footer.</li> </ul> <p>We are using style.css, style-main.css and specific-chemistry.css for the styling.</p> <p>Next, I added some content to the page:<br> </p> <pre class="brush:php;toolbar:false"><section> <p>I also added a search bar and some links, that we will add later.</p> <h2> Hour 26: Adding JavaScript for Search Functionality </h2> <p>Since we used a similar structure to the organic chemistry page, I copied over the JavaScript code for the hamburger menu and the article search functionality from organic.html to this inorganic chemistry page, removing the 3D molecule viewer part.<br> </p> <pre class="brush:php;toolbar:false">// Hamburger Menu Toggle const hamburgerBtn = document.getElementById('hamburger-btn'); const navMenu = document.getElementById('nav-menu'); hamburgerBtn.addEventListener('click', () => { navMenu.classList.toggle('show'); }); // Article Search Functionality (Basic Example) const articleSearch = document.getElementById('article-search'); const articleLinks = document.getElementById('article-links').querySelectorAll('a'); articleSearch.addEventListener('input', () => { const searchTerm = articleSearch.value.toLowerCase(); articleLinks.forEach(link => { const linkText = link.textContent.toLowerCase(); if (linkText.includes(searchTerm)) { link.style.display = 'block'; } else { link.style.display = 'none'; } }); });
我必須調整選擇器以符合此頁面中使用的 ID。該腳本現在可以處理基本的漢堡菜單切換和文章搜尋過濾。
我們現在有一個基本的無機.html 頁面,其結構與有機化學頁面類似。我們可以透過以下方式進一步增強它:
-
新增內容:
- 針對列出的主題撰寫實際文章。
- 將圖像或圖表新增至主題網格。
-
造型:
- 使用 CSS 改善視覺呈現。
- 確保不同螢幕尺寸的反應能力。
-
更多互動:
- 依需求新增測驗或互動式圖表。
-
導航:
- 如果網站變得更加複雜,則實施更強大的導航系統,因為目前的導航系統非常基本
由於我們今天的主要重點是設定結構和基本功能,因此我們可以在接下來的步驟中考慮這些增強功能。我們還可以透過添加更多相關內容並提高頁面的回應能力來改善這一點。
以上是我從頭開始建立了終極教育網站 — 第 5 天的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
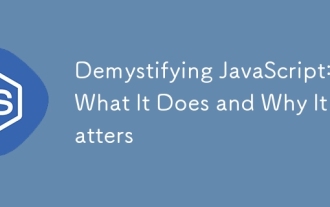
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
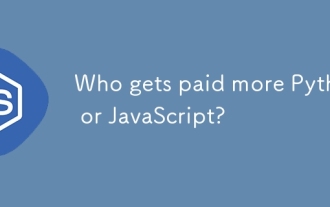
Python和JavaScript開發者的薪資沒有絕對的高低,具體取決於技能和行業需求。 1.Python在數據科學和機器學習領域可能薪資更高。 2.JavaScript在前端和全棧開發中需求大,薪資也可觀。 3.影響因素包括經驗、地理位置、公司規模和特定技能。
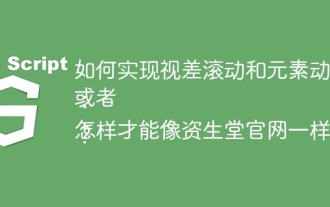
實現視差滾動和元素動畫效果的探討本文將探討如何實現類似資生堂官網(https://www.shiseido.co.jp/sb/wonderland/)中�...
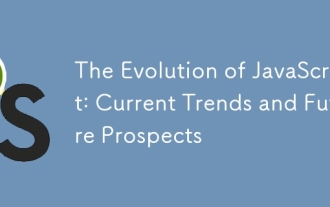
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
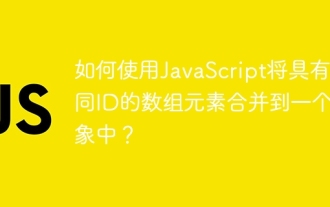
如何在JavaScript中將具有相同ID的數組元素合併到一個對像中?在處理數據時,我們常常會遇到需要將具有相同ID�...
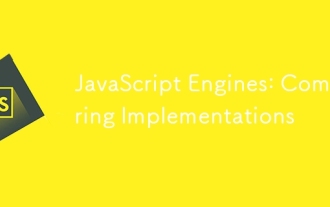
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
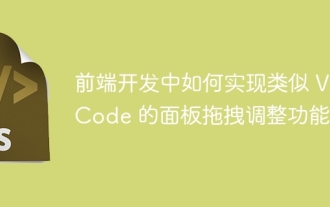
探索前端中類似VSCode的面板拖拽調整功能的實現在前端開發中,如何實現類似於VSCode...
