Python:重構模式
攝影:Patric Ho
這份簡明指南將 Python 程式碼氣味對應到對應的設計模式解決方案。
class CodeSmellSolutions: DUPLICATED_CODE = [ "form_template_method", "introduce_polymorphic_creation_with_factory_method", "chain_constructors", "replace_one__many_distinctions_with_composite", "extract_composite", "unify_interfaces_with_adapter", "introduce_null_object", ] LONG_METHOD = [ "compose_method", "move_accumulation_to_collecting_parameter", "replace_conditional_dispatcher_with_command", "move_accumulation_to_visitor", "replace_conditional_logic_with_strategy", ] CONDITIONAL_COMPLEXITY = [ # Complex conditional logic "replace_conditional_logic_with_strategy", "move_emblishment_to_decorator", "replace_state_altering_conditionals_with_state", "introduce_null_object", ] PRIMITIVE_OBSESSION = [ "replace_type_code_with_class", "replace_state_altering_conditionals_with_state", "replace_conditional_logic_with_strategy", "replace_implict_tree_with_composite", "replace_implicit_language_with_interpreter", "move_emblishment_to_decorator", "encapsulate_composite_with_builder", ] INDECENT_EXPOSURE = [ # Lack of information hiding "encapsulate_classes_with_factory" ] SOLUTION_SPRAWL = [ # Scattered logic/responsibility "move_creation_knowledge_to_factory" ] ALTERNATIVE_CLASSES_WITH_DIFFERENT_INTERFACES = [ # Similar classes, different interfaces "unify_interfaces_with_adapter" ] LAZY_CLASS = [ # Insufficient functionality "inline_singleton" ] LARGE_CLASS = [ "replace_conditional_dispatcher_with_command", "replace_state_altering_conditionals_with_state", "replace_implict_tree_with_composite", ] SWITCH_STATEMENTS = [ # Complex switch statements "replace_conditional_dispatcher_with_command", "move_accumulation_to_visitor", ] COMBINATION_EXPLOSION = [ # Similar code for varying data "replace_implicit_language_with_interpreter" ] ODDBALL_SOLUTIONS = [ # Multiple solutions for same problem "unify_interfaces_with_adapter" ]
Python 中的重構範例
專案將重構範例從 Refactoring to Patterns (Joshua Kerievsky) 翻譯成 Python。每個範例都顯示原始和重構的程式碼,突出顯示改進。 重構過程涉及解釋 UML 圖並使 Java 程式碼適應 Python 的細微差別(處理循環導入和介面)。
範例:撰寫方法
「Compose Method」重構透過擷取更小、更有意義的方法來簡化複雜的程式碼。
# Original (complex) code def add(element): readonly = False size = 0 elements = [] if not readonly: new_size = size + 1 if new_size > len(elements): new_elements = [] for i in range(size): new_elements[i] = elements[i] # Potential IndexError elements = new_elements size += 1 elements[size] = element # Potential IndexError # Refactored (simplified) code def is_at_capacity(new_size, elements): return new_size > len(elements) def grow_array(size, elements): new_elements = [elements[i] for i in range(size)] # List comprehension for clarity return new_elements def add_element(elements, element, size): elements.append(element) # More Pythonic approach return len(elements) -1 def add_refactored(element): readonly = False if readonly: return size = len(elements) new_size = size + 1 if is_at_capacity(new_size, elements): elements = grow_array(size, elements) size = add_element(elements, element, size)
範例:多態性(測試自動化)
此範例示範了測試自動化中的多態性,抽象化了測試設定以實現可重複使用性。
# Original code (duplicate setup) class TestCase: pass class DOMBuilder: def __init__(self, orders): pass def calc(self): return 42 class XMLBuilder: def __init__(self, orders): pass def calc(self): return 42 class DOMTest(TestCase): def run_dom_test(self): expected = 42 builder = DOMBuilder("orders") assert builder.calc() == expected class XMLTest(TestCase): def run_xml_test(self): expected = 42 builder = XMLBuilder("orders") assert builder.calc() == expected # Refactored code (polymorphic setup) class OutputBuilder: def calc(self): raise NotImplementedError class DOMBuilderRefac(OutputBuilder): def calc(self): return 42 class XMLBuilderRefac(OutputBuilder): def calc(self): return 42 class TestCaseRefac: def create_builder(self): raise NotImplementedError def run_test(self): expected = 42 builder = self.create_builder() assert builder.calc() == expected class DOMTestRefac(TestCaseRefac): def create_builder(self): return DOMBuilderRefac() class XMLTestRefac(TestCaseRefac): def create_builder(self): return XMLBuilderRefac()
範例:訪客模式
訪客模式將類別與其方法解耦。
# Original code (conditional logic in TextExtractor) class Node: pass class LinkTag(Node): pass class Tag(Node): pass class StringNode(Node): pass class TextExtractor: def extract_text(self, nodes): result = [] for node in nodes: if isinstance(node, StringNode): result.append("string") elif isinstance(node, LinkTag): result.append("linktag") elif isinstance(node, Tag): result.append("tag") else: result.append("other") return result # Refactored code (using Visitor) class NodeVisitor: def visit_link_tag(self, node): return "linktag" def visit_tag(self, node): return "tag" def visit_string_node(self, node): return "string" class Node: def accept(self, visitor): pass class LinkTagRefac(Node): def accept(self, visitor): return visitor.visit_link_tag(self) class TagRefac(Node): def accept(self, visitor): return visitor.visit_tag(self) class StringNodeRefac(Node): def accept(self, visitor): return visitor.visit_string_node(self) class TextExtractorVisitor(NodeVisitor): def extract_text(self, nodes): result = [node.accept(self) for node in nodes] return result
結論
這種透過重構學習設計模式的實用方法可以顯著增強理解。 翻譯程式碼時遇到的挑戰鞏固了理論知識。
以上是Python:重構模式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
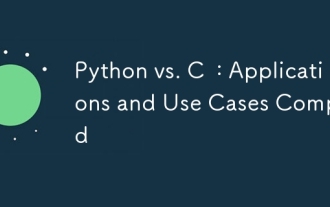
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
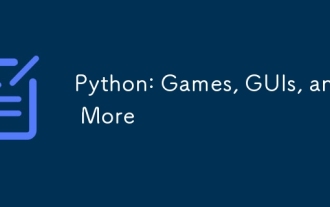
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
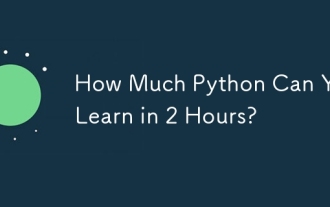
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
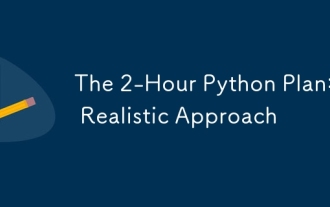
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
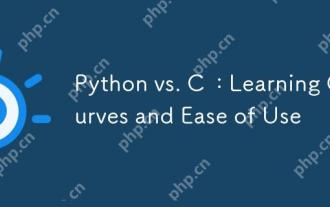
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
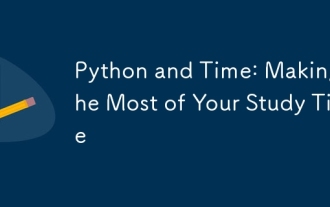
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
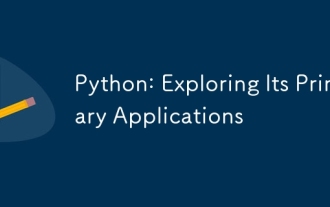
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
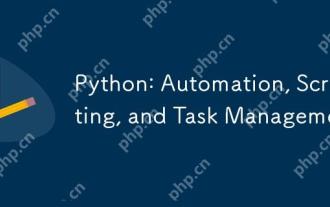
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
