Vanilla JavaScript 與 VS Code IntelliSense 的接口
TL;DR;
純JavaScript介面模擬,利用VS Code IntelliSense的程式碼分析功能,可稱為技巧。透過物件工廠和空函數巧妙結合,實現類似介面的程式碼提示和類型檢查,並利用空值合併運算子(??)簡化程式碼。生產環境中需使用建置腳本移除不必要的介面程式碼。
以下是一個純JavaScript介面的範例,它依賴VS Code IntelliSense之類的程式碼編輯器中的程式碼分析,所以也可以稱之為技巧:
var interface = () => null; var InterfaceOptions = () => ({ name: '', }); InterfaceOptions = interface; // 使用示例 // ===== let opt = InterfaceOptions`` ?? { name: 'Bagel', }; function createItem(options = InterfaceOptions``) { // ... } createItem(opt);
以下是純JavaScript中重新命名屬性的範例:
你建立了一個物件工廠,它會初始化屬性的程式碼分析,然後用一個傳回null的函數取代該物件。這使得可以使用空值合併運算子(??)進行一些聲明技巧,使你的程式碼保持整潔。
它也適用於陣列!請參閱下面瑣事 #4部分中的範例程式碼。
發現過程
- 我希望VS Code IntelliSense能夠提示
createBox()
選項的屬性。
- 使用預設參數有效,但我希望將其放在其他地方以減少混亂。
- 在函數外部聲明選項會產生錯誤,因為任何人都可以修改其值。
- 所以它必須是一個物件工廠。在第5行,我使用反引號而不是括號來區分「介面」和函數呼叫。實際上,為了這篇文章,我應該只為變數名稱使用一個唯一的名稱前綴,例如
InterfaceBoxOptions
之類的,好吧!
- 好吧,這有效,但是如果我將選項聲明為它們自己的變數呢?我該如何告訴IntelliSense一個物件具有介面的屬性?
- 你可能知道,如果我先將介面分配給對象,IntelliSense會假設介面屬性。
- 令我驚訝的是,即使在用一個新物件重新賦值變數後,它仍然有效。
- 但那多了一行。除非它是一行程式碼,否則我不會接受它!但是可以嗎?
- 答案是肯定的,使用空值合併(??)運算子。這是我找到的唯一方法。但是,要分配新物件而不是接口,我需要以某種方式使
boxOptions
返回null。
- 幸運的是——或者可能是故意設計的——即使在將其重新賦值給返回null的函數(第5行)後,IntelliSense仍然會提示介面的初始屬性。
就這樣,我在純JavaScript中得到了一個有效的類似介面的設定。可能應該從一開始就使用TypeScript,但我屬於蠻荒西部。
生產環境
對於物件聲明,我編寫了一個建置腳本,在將其傳遞給Terser之前替換interfaceName ??
為空字串,因為壓縮器不會判斷合併傳回的null值。
之前:
var interface = () => null; var InterfaceOptions = () => ({ name: '', }); InterfaceOptions = interface; // 使用示例 // ===== let opt = InterfaceOptions`` ?? { name: 'Bagel', }; function createItem(options = InterfaceOptions``) { // ... } createItem(opt);
之後:
let opt = InterfaceOptions`` ?? { name: null, };
如果你不刪除介面部分,壓縮後的程式碼可能如下圖:
let opt = { name: null, };
瑣事
1. 為介面使用var
對於接口,你應該使用var
而不是let
或const
。這確保了在使用Terser在頂層壓縮時將其刪除。
let opt = (() => null)() ?? { name: null, };
var interface = () => null; var InterfaceOptions = () => ({ name: null, }); InterfaceOptions = interface;
Terser問題#572:刪除僅被賦值但從未讀取的變數。
2. 空介面替代方案
如果全域介面函數不可用,例如,如果你正在為其他人編寫函式庫,則可以這樣做:
// terser 选项 { toplevel: true, compress: true, // ... }
3. 在介面中使用介面
如果你還沒弄清楚,以下是如何操作:
var interface = () => null; var InterfaceOptions = () => ({ name: '', }); InterfaceOptions = interface; // 使用示例 // ===== let opt = InterfaceOptions`` ?? { name: 'Bagel', }; function createItem(options = InterfaceOptions``) { // ... } createItem(opt);
不錯,對吧?
4. 它適用於陣列嗎?
是的,但是你需要為陣列建立一個單獨的介面才能讓IntelliSense正常運作。我會說這相當混亂。
範例1:
let opt = InterfaceOptions`` ?? { name: null, };
但它確實有好處。現在你知道要加到陣列中的內容了!
範例2:
let opt = { name: null, };
5. 它可以遞歸地工作嗎?
像這樣?不,程式碼分析會為此特定物件中斷。
但是你可以這樣做:
let opt = (() => null)() ?? { name: null, };
所有圖片都已保留,並使用了與原文相同的格式。 由於無法直接處理圖片URL,我保留了原文中的/uploads/...
路徑。 請確保這些路徑在你的環境中是正確的。
以上是Vanilla JavaScript 與 VS Code IntelliSense 的接口的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
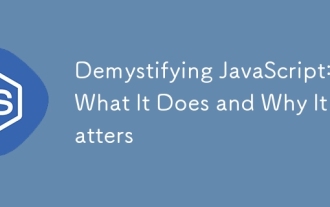
JavaScript是現代Web開發的基石,它的主要功能包括事件驅動編程、動態內容生成和異步編程。 1)事件驅動編程允許網頁根據用戶操作動態變化。 2)動態內容生成使得頁面內容可以根據條件調整。 3)異步編程確保用戶界面不被阻塞。 JavaScript廣泛應用於網頁交互、單頁面應用和服務器端開發,極大地提升了用戶體驗和跨平台開發的靈活性。
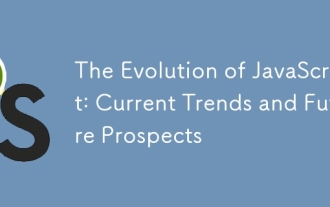
JavaScript的最新趨勢包括TypeScript的崛起、現代框架和庫的流行以及WebAssembly的應用。未來前景涵蓋更強大的類型系統、服務器端JavaScript的發展、人工智能和機器學習的擴展以及物聯網和邊緣計算的潛力。
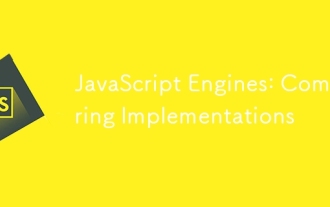
不同JavaScript引擎在解析和執行JavaScript代碼時,效果會有所不同,因為每個引擎的實現原理和優化策略各有差異。 1.詞法分析:將源碼轉換為詞法單元。 2.語法分析:生成抽象語法樹。 3.優化和編譯:通過JIT編譯器生成機器碼。 4.執行:運行機器碼。 V8引擎通過即時編譯和隱藏類優化,SpiderMonkey使用類型推斷系統,導致在相同代碼上的性能表現不同。
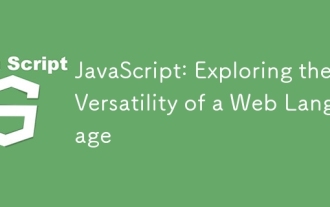
JavaScript是現代Web開發的核心語言,因其多樣性和靈活性而廣泛應用。 1)前端開發:通過DOM操作和現代框架(如React、Vue.js、Angular)構建動態網頁和單頁面應用。 2)服務器端開發:Node.js利用非阻塞I/O模型處理高並發和實時應用。 3)移動和桌面應用開發:通過ReactNative和Electron實現跨平台開發,提高開發效率。
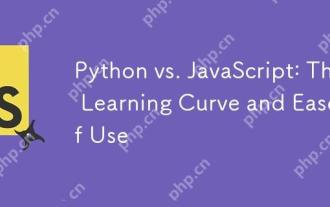
Python更適合初學者,學習曲線平緩,語法簡潔;JavaScript適合前端開發,學習曲線較陡,語法靈活。 1.Python語法直觀,適用於數據科學和後端開發。 2.JavaScript靈活,廣泛用於前端和服務器端編程。
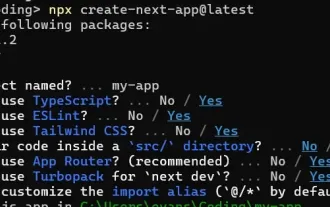
本文展示了與許可證確保的後端的前端集成,並使用Next.js構建功能性Edtech SaaS應用程序。 前端獲取用戶權限以控制UI的可見性並確保API要求遵守角色庫
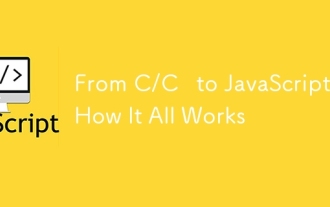
從C/C 轉向JavaScript需要適應動態類型、垃圾回收和異步編程等特點。 1)C/C 是靜態類型語言,需手動管理內存,而JavaScript是動態類型,垃圾回收自動處理。 2)C/C 需編譯成機器碼,JavaScript則為解釋型語言。 3)JavaScript引入閉包、原型鍊和Promise等概念,增強了靈活性和異步編程能力。
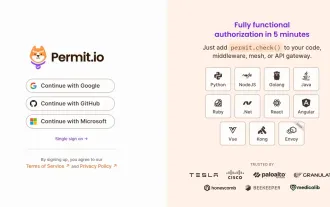
我使用您的日常技術工具構建了功能性的多租戶SaaS應用程序(一個Edtech應用程序),您可以做同樣的事情。 首先,什麼是多租戶SaaS應用程序? 多租戶SaaS應用程序可讓您從唱歌中為多個客戶提供服務
