如何在 C# 中設定全域熱鍵以觸發應用程式事件,即使應用程式未處於焦點狀態?
此 C# 程式碼建立一個全域熱鍵,即使應用程式最小化或未獲得焦點,該熱鍵也會觸發事件。 讓我們改進解釋和程式碼清晰度,以便更好地理解。
在 C# 中建立全域熱鍵
本文示範如何在 C# 中實現全域熱鍵,以響應鍵盤快捷鍵,而不管應用程式焦點如何。 我們將重點放在註冊和處理 Ctrl Alt J 等組合。
解:使用user32.dll
實現這一目標的關鍵在於 user32.dll
函式庫,特別是 RegisterHotKey
和 UnregisterHotKey
函數。 這些函數需要一個視窗句柄;因此,該解決方案最適合 Windows 窗體應用程式 (WinForms)。 控制台應用程式缺乏必要的視窗上下文。
程式碼實作:
下面改進的程式碼增強了可讀性並包含全面的註解:
using System; using System.Runtime.InteropServices; using System.Windows.Forms; public sealed class GlobalHotkey : IDisposable { // Import necessary functions from user32.dll [DllImport("user32.dll")] private static extern bool RegisterHotKey(IntPtr hWnd, int id, uint fsModifiers, uint vk); [DllImport("user32.dll")] private static extern bool UnregisterHotKey(IntPtr hWnd, int id); private const int WM_HOTKEY = 0x0312; // Windows message for hotkey events private readonly NativeWindow _window; // Internal window for message handling private int _hotKeyId; // Unique ID for each registered hotkey public GlobalHotkey() { _window = new NativeWindow(); _window.AssignHandle(new CreateParams().CreateHandle()); // Create the window handle _window.WndProc += WndProc; // Assign the window procedure } // Registers a hotkey public void Register(ModifierKeys modifiers, Keys key) { _hotKeyId++; // Generate a unique ID if (!RegisterHotKey(_window.Handle, _hotKeyId, (uint)modifiers, (uint)key)) { throw new Exception("Failed to register hotkey."); } } // Unregisters all hotkeys public void Unregister() { for (int i = 1; i <= _hotKeyId; i++) { UnregisterHotKey(_window.Handle, i); } _window.ReleaseHandle(); // Release the window handle } // Window procedure to handle hotkey messages private void WndProc(ref Message m) { if (m.Msg == WM_HOTKEY) { // Extract key and modifiers from message parameters Keys key = (Keys)(((int)m.LParam >> 16) & 0xFFFF); ModifierKeys modifiers = (ModifierKeys)((int)m.LParam & 0xFFFF); // Raise the HotkeyPressed event HotkeyPressed?.Invoke(this, new HotkeyPressedEventArgs(modifiers, key)); } } // Event fired when a registered hotkey is pressed public event EventHandler<HotkeyPressedEventArgs> HotkeyPressed; // IDisposable implementation public void Dispose() { Unregister(); _window.Dispose(); } } // Event arguments for HotkeyPressed event public class HotkeyPressedEventArgs : EventArgs { public ModifierKeys Modifiers { get; } public Keys Key { get; } public HotkeyPressedEventArgs(ModifierKeys modifiers, Keys key) { Modifiers = modifiers; Key = key; } } // Enum for hotkey modifiers [Flags] public enum ModifierKeys : uint { None = 0, Alt = 1, Control = 2, Shift = 4, Win = 8 }
用法範例(WinForms):
public partial class Form1 : Form { private GlobalHotkey _globalHotkey; public Form1() { InitializeComponent(); _globalHotkey = new GlobalHotkey(); _globalHotkey.HotkeyPressed += GlobalHotkey_HotkeyPressed; _globalHotkey.Register(ModifierKeys.Control | ModifierKeys.Alt, Keys.J); } private void GlobalHotkey_HotkeyPressed(object sender, HotkeyPressedEventArgs e) { // Handle the hotkey press here MessageBox.Show($"Hotkey pressed: Ctrl+Alt+J"); } protected override void OnFormClosing(FormClosingEventArgs e) { _globalHotkey.Dispose(); // Important: Dispose of the hotkey when the form closes base.OnFormClosing(e); } }
請記得新增錯誤處理並正確處置GlobalHotkey
實例(如OnFormClosing
所示)以防止資源外洩。 此修訂後的程式碼為管理 C# WinForms 應用程式中的全域熱鍵提供了更強大且易於理解的解決方案。
以上是如何在 C# 中設定全域熱鍵以觸發應用程式事件,即使應用程式未處於焦點狀態?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
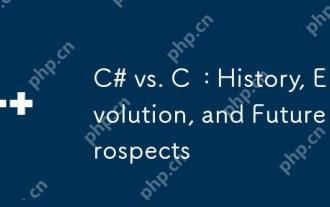
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
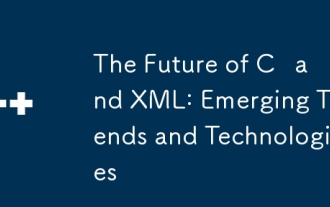
C 和XML的未來發展趨勢分別為:1)C 將通過C 20和C 23標準引入模塊、概念和協程等新特性,提升編程效率和安全性;2)XML將繼續在數據交換和配置文件中佔據重要地位,但會面臨JSON和YAML的挑戰,並朝著更簡潔和易解析的方向發展,如XMLSchema1.1和XPath3.1的改進。
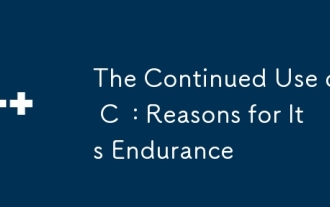
C 持續使用的理由包括其高性能、廣泛應用和不斷演進的特性。 1)高效性能:通過直接操作內存和硬件,C 在系統編程和高性能計算中表現出色。 2)廣泛應用:在遊戲開發、嵌入式系統等領域大放異彩。 3)不斷演進:自1983年發布以來,C 持續增加新特性,保持其競爭力。
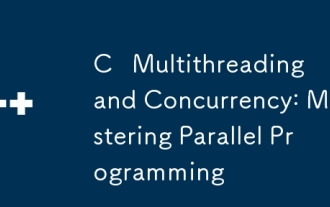
C 多線程和並發編程的核心概念包括線程的創建與管理、同步與互斥、條件變量、線程池、異步編程、常見錯誤與調試技巧以及性能優化與最佳實踐。 1)創建線程使用std::thread類,示例展示瞭如何創建並等待線程完成。 2)同步與互斥使用std::mutex和std::lock_guard保護共享資源,避免數據競爭。 3)條件變量通過std::condition_variable實現線程間的通信和同步。 4)線程池示例展示瞭如何使用ThreadPool類並行處理任務,提高效率。 5)異步編程使用std::as
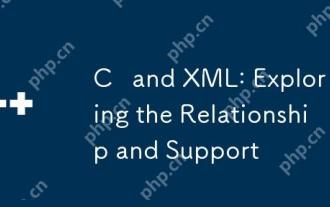
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
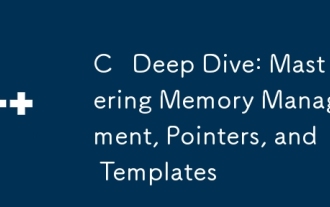
C 的內存管理、指針和模板是核心特性。 1.內存管理通過new和delete手動分配和釋放內存,需注意堆和棧的區別。 2.指針允許直接操作內存地址,使用需謹慎,智能指針可簡化管理。 3.模板實現泛型編程,提高代碼重用性和靈活性,需理解類型推導和特化。
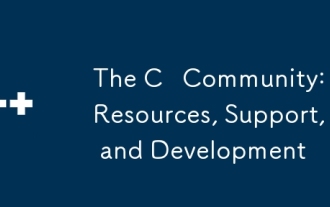
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
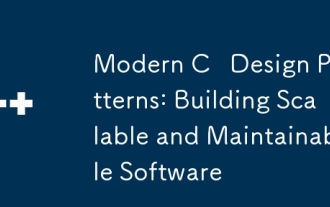
現代C 設計模式利用C 11及以後的新特性實現,幫助構建更靈活、高效的軟件。 1)使用lambda表達式和std::function簡化觀察者模式。 2)通過移動語義和完美轉發優化性能。 3)智能指針確保類型安全和資源管理。
