原型設計模式解釋
原型設計模式提供了一種通過克隆現有對象來創建新對象的強大方法,避免了直接實例化的開銷。 當對象創建需要大量資源時,這尤其有用。
理想用例:
原型圖案在以下情況下會發光:
- 對象創建成本高昂:構建具有大量依賴項或需要大量設置(數據庫連接、大型圖形結構)的複雜對象可以得到顯著優化。
- 需要類似的對象:創建具有較小變化的多個對像被簡化;克隆基礎對象並調整特定屬性比重複構建更有效。
- 對像類型是動態的:當確切的對像類型直到運行時才知道時,原型模式提供了靈活性。
機制:
該模式取決於兩個關鍵組成部分:
-
原型接口:定義對象複製的
Clone()
方法的通用接口。 -
具體原型: 實現
Clone()
方法的類,為每種對像類型提供特定的克隆邏輯。
說明該模式的類圖:
Golang 示例:遊戲角色克隆
在遊戲開發中,角色創建通常涉及定義基本角色類型(戰士、法師等),然後自定義各個玩家角色。 原型模式優雅地處理了這個問題:
實施
package prototype import "fmt" // Prototype interface type Prototype interface { Clone() Prototype GetDetails() string } // Concrete Prototype: GameCharacter type GameCharacter struct { Name string Class string Level int Health int Stamina int Weapon string Armor string Speciality string } // Clone method for GameCharacter func (c *GameCharacter) Clone() Prototype { return &GameCharacter{ Name: c.Name, Class: c.Class, Level: c.Level, Health: c.Health, Stamina: c.Stamina, Weapon: c.Weapon, Armor: c.Armor, Speciality: c.Speciality, } } // GetDetails method for GameCharacter func (c *GameCharacter) GetDetails() string { return fmt.Sprintf("Name: %s, Class: %s, Level: %d, Health: %d, Stamina: %d, Weapon: %s, Armor: %s, Speciality: %s", c.Name, c.Class, c.Level, c.Health, c.Stamina, c.Weapon, c.Armor, c.Speciality) }
package main import ( "example.com/prototype" "fmt" ) func main() { // Warrior template warrior := &prototype.GameCharacter{ Name: "Base Warrior", Class: "Warrior", Level: 1, Health: 100, Stamina: 50, Weapon: "Sword", Armor: "Steel Armor", Speciality: "Melee Combat", } // Clone and customize for players player1 := warrior.Clone().(*prototype.GameCharacter) player1.Name = "Arthas" player1.Level = 10 player1.Weapon = "Frostmourne" player2 := warrior.Clone().(*prototype.GameCharacter) player2.Name = "Leonidas" player2.Level = 8 player2.Weapon = "Spear" player2.Armor = "Golden Armor" // Output character details fmt.Println("Template:", warrior.GetDetails()) fmt.Println("Player 1:", player1.GetDetails()) fmt.Println("Player 2:", player2.GetDetails()) }
輸出
<code>Template: Name: Base Warrior, Class: Warrior, Level: 1, Health: 100, Stamina: 50, Weapon: Sword, Armor: Steel Armor, Speciality: Melee Combat Player 1: Name: Arthas, Class: Warrior, Level: 10, Health: 100, Stamina: 50, Weapon: Frostmourne, Armor: Steel Armor, Speciality: Melee Combat Player 2: Name: Leonidas, Class: Warrior, Level: 8, Health: 100, Stamina: 50, Weapon: Spear, Armor: Golden Armor, Speciality: Melee Combat</code>
主要優勢:
- 減少重複:可重用的基礎對象最大限度地減少冗餘代碼。
- 性能提升:克隆比重複對象構造更快。
- 增強靈活性:輕鬆自定義克隆對象而不影響原始對象。
潛在挑戰:
- 深拷貝與淺拷貝:正確處理嵌套對像對於避免意外修改至關重要。
- 接口遵循:所有可克隆對像都必須實現 Prototype 接口。
結論:
原型模式是一種有價值的設計工具,可用於高效的對象創建和管理,特別是在對象構造複雜或計算成本高昂的情況下。 它的靈活性使其能夠適應各種需要動態對像生成的情況。
以上是原型設計模式解釋的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
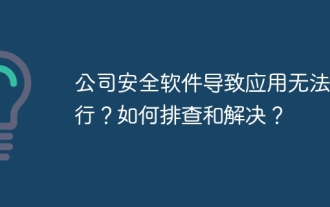
公司安全軟件導致部分應用無法正常運行的排查與解決方法許多公司為了保障內部網絡安全,會部署安全軟件。 ...
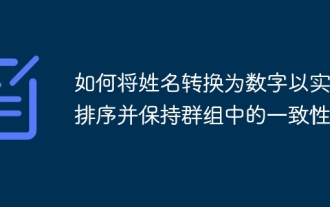
將姓名轉換為數字以實現排序的解決方案在許多應用場景中,用戶可能需要在群組中進行排序,尤其是在一個用...
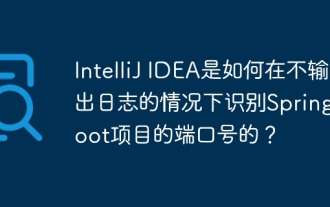
在使用IntelliJIDEAUltimate版本啟動Spring...
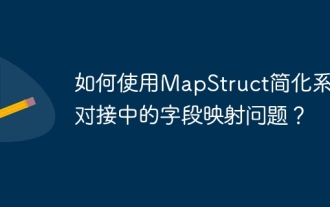
系統對接中的字段映射處理在進行系統對接時,常常會遇到一個棘手的問題:如何將A系統的接口字段有效地映�...
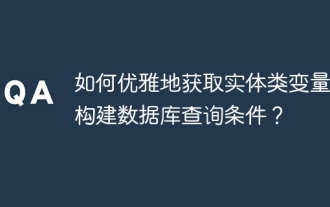
在使用MyBatis-Plus或其他ORM框架進行數據庫操作時,經常需要根據實體類的屬性名構造查詢條件。如果每次都手動...
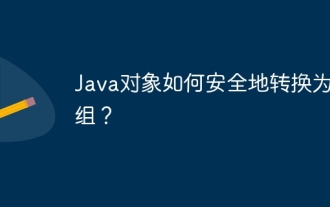
Java對象與數組的轉換:深入探討強制類型轉換的風險與正確方法很多Java初學者會遇到將一個對象轉換成數組的�...
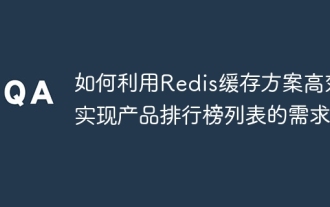
Redis緩存方案如何實現產品排行榜列表的需求?在開發過程中,我們常常需要處理排行榜的需求,例如展示一個�...
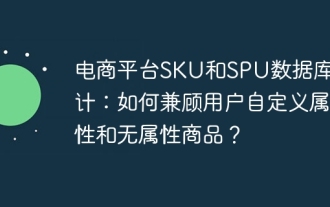
電商平台SKU和SPU表設計詳解本文將探討電商平台中SKU和SPU的數據庫設計問題,特別是如何處理用戶自定義銷售屬...
