如何在 Windows 窗體文字方塊中顯示即時命令輸出?
此程式碼示範如何執行命令列進程並在 Windows 窗體文字方塊中顯示其實時輸出。 讓我們對其進行改進,使其更加清晰和穩健。
改進的程式碼:
此版本添加了錯誤處理、更清晰的變數名稱和改進的線程實踐。
using System; using System.Diagnostics; using System.Text; using System.Threading; using System.Windows.Forms; public static class CommandExecutor { public delegate void OutputHandler(string line); public static int Run(string workingDirectory, string command, string arguments, OutputHandler outputHandler, bool hideWindow = true) { int exitCode = -1; // Initialize to an invalid value try { using (var process = new Process()) { process.StartInfo.FileName = "cmd.exe"; process.StartInfo.WorkingDirectory = workingDirectory; process.StartInfo.Arguments = $"/c \"{command} {arguments}\" 2>&1"; // Redirect stderr to stdout process.StartInfo.CreateNoWindow = hideWindow; process.StartInfo.UseShellExecute = false; process.StartInfo.RedirectStandardOutput = true; process.StartInfo.RedirectStandardError = true; if (outputHandler != null) { process.OutputDataReceived += (sender, e) => { if (e.Data != null) { outputHandler(e.Data); } }; process.ErrorDataReceived += (sender, e) => { if (e.Data != null) { outputHandler($"Error: {e.Data}"); //Clearly mark error messages } }; } process.Start(); if (outputHandler != null) { process.BeginOutputReadLine(); process.BeginErrorReadLine(); //Begin reading error stream process.WaitForExit(); } else { process.WaitForExit(); } exitCode = process.ExitCode; } } catch (Exception ex) { MessageBox.Show($"An error occurred: {ex.Message}", "Error", MessageBoxButtons.OK, MessageBoxIcon.Error); } return exitCode; } public static string GetOutput(string workingDirectory, string command, string arguments) { StringBuilder output = new StringBuilder(); Run(workingDirectory, command, arguments, line => output.AppendLine(line)); return output.ToString(); } } public partial class Form1 : Form { private TextBox txtOutput; //Declare TextBox public Form1() { InitializeComponent(); txtOutput = new TextBox { Dock = DockStyle.Fill, Multiline = true, ScrollBars = ScrollBars.Both }; Controls.Add(txtOutput); // Add the TextBox to the form //Add a button (btnExecute) to your form in the designer. } private void btnExecute_Click(object sender, EventArgs e) { //Get command and arguments from your textboxes (e.g., textBoxCommand, textBoxArguments) string command = textBoxCommand.Text; string arguments = textBoxArguments.Text; CommandExecutor.Run(@"C:\", command, arguments, line => { if (txtOutput.InvokeRequired) { txtOutput.Invoke(new MethodInvoker(() => txtOutput.AppendText(line + Environment.NewLine))); } else { txtOutput.AppendText(line + Environment.NewLine); } }); } }
主要改善:
-
錯誤處理:
try-catch
區塊處理流程執行期間潛在的異常。 -
更清楚的命名:更具描述性的變數名稱可提高可讀性(
CommandExecutor
、workingDirectory
)。 - 錯誤流處理:程式碼現在讀取並顯示指令的錯誤輸出。
-
執行緒安全:
InvokeRequired
檢查可確保從後台執行緒更新 TextBox 時的執行緒安全性。 -
Environment.NewLine: 使用
Environment.NewLine
實作跨平台的一致換行符。 - 簡化的參數處理:使用字串插值來實現更清晰的參數構造。
- 單獨的文字方塊聲明:單獨聲明文字方塊是為了更好的組織。
請記得在 Visual Studio 設計器中為表單新增一個文字方塊(例如 txtOutput
)和一個按鈕(例如 btnExecute
)。 您還需要文字方塊來輸入命令及其參數。 將 textBoxCommand
和 textBoxArguments
替換為文字方塊的實際名稱。 此改進的程式碼提供了更強大且使用者友好的解決方案,用於在 Windows 窗體應用程式中顯示即時命令輸出。
以上是如何在 Windows 窗體文字方塊中顯示即時命令輸出?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
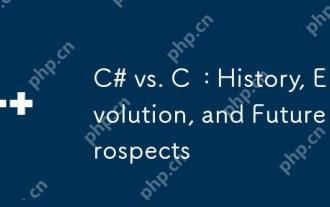
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
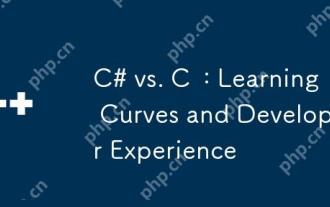
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
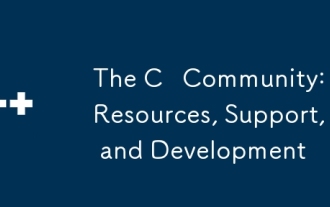
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
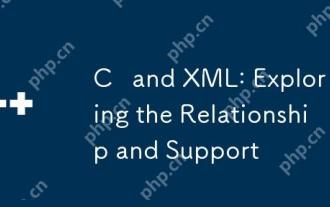
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
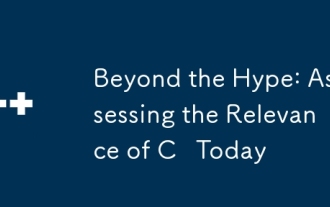
C 在現代編程中仍然具有重要相關性。 1)高性能和硬件直接操作能力使其在遊戲開發、嵌入式系統和高性能計算等領域佔據首選地位。 2)豐富的編程範式和現代特性如智能指針和模板編程增強了其靈活性和效率,儘管學習曲線陡峭,但其強大功能使其在今天的編程生態中依然重要。
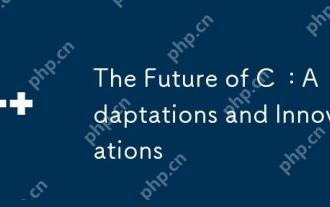
C 的未來將專注於並行計算、安全性、模塊化和AI/機器學習領域:1)並行計算將通過協程等特性得到增強;2)安全性將通過更嚴格的類型檢查和內存管理機制提升;3)模塊化將簡化代碼組織和編譯;4)AI和機器學習將促使C 適應新需求,如數值計算和GPU編程支持。
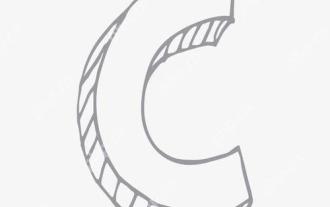
使用C 中的chrono庫可以讓你更加精確地控制時間和時間間隔,讓我們來探討一下這個庫的魅力所在吧。 C 的chrono庫是標準庫的一部分,它提供了一種現代化的方式來處理時間和時間間隔。對於那些曾經飽受time.h和ctime折磨的程序員來說,chrono無疑是一個福音。它不僅提高了代碼的可讀性和可維護性,還提供了更高的精度和靈活性。讓我們從基礎開始,chrono庫主要包括以下幾個關鍵組件:std::chrono::system_clock:表示系統時鐘,用於獲取當前時間。 std::chron
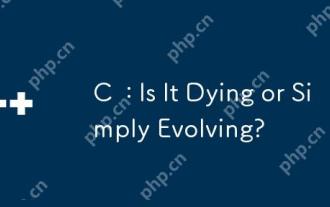
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
