``typeof'','getType'和'在C#類型檢查中有所不同嗎?
C# 類型檢查:typeof
、GetType
和 is
的比較
在 C# 中處理類型時,有多種方法可以檢查對像或變量的類型。理解 typeof
、GetType
和 is
之間的區別對於有效的類型檢查至關重要。
typeof
typeof
運算符是一個編譯時運算符,它返回一個表示指定類型的 Type
對象。它通常用於在編譯時比較對象的類型。例如:
Type t = typeof(int); if (t == typeof(int)) // 一些代码
GetType
GetType
方法返回實例的運行時類型。它用於確定對像在運行時的類型,即使在編譯時不知道實際類型。例如:
object obj1 = 5; if (obj1.GetType() == typeof(int)) // 一些代码
is
is
運算符是一個運行時運算符,如果實例位於指定類型的繼承樹中,則返回 true
。它通常用於檢查對像是否為特定類型或其派生類型。例如:
object obj1 = 5; if (obj1 is int) // 一些代码
主要區別
typeof
: 在編譯時操作,根據指定的類型名稱提供類型信息。GetType
: 在運行時操作,檢索實例的實際類型。is
: 在運行時操作,檢查實例是否屬於給定類型或其繼承樹。
使用注意事項
這三種方法中最佳的選擇取決於具體的場景。 typeof
優先用於在編譯時執行類型檢查,儘早確保類型兼容性。 GetType
在運行時檢查實例類型時很有用,例如在動態代碼場景中。 is
運算符方便在運行時檢查繼承關係。
示例
考慮以下代碼:
class Animal { } class Dog : Animal { } void PrintTypes(Animal a) { Console.WriteLine(a.GetType() == typeof(Animal)); // 可能为false,取决于a的实际类型 Console.WriteLine(a is Animal); // true (Dog继承自Animal) Console.WriteLine(a.GetType() == typeof(Dog)); // true 如果a是Dog实例,否则为false Console.WriteLine(a is Dog); // true 如果a是Dog实例,否则为false } Dog spot = new Dog(); PrintTypes(spot);
在這個例子中,如果 a
是 Dog
的實例,a is Animal
將返回 true
,因為 Dog
繼承自 Animal
。但是,a.GetType() == typeof(Dog)
和 a is Dog
只有在 a
實際上是 Dog
類實例時才返回 true
。 a.GetType() == typeof(Animal)
只有在 a
是 Animal
實例時才返回 true
。
以上是``typeof'','getType'和'在C#類型檢查中有所不同嗎?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
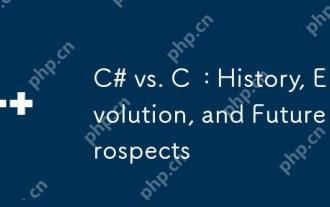
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
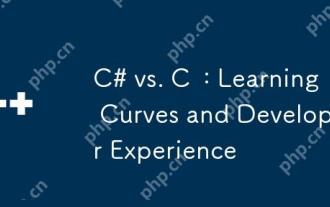
C#和C 的学习曲线和开发者体验有显著差异。1)C#的学习曲线较平缓,适合快速开发和企业级应用。2)C 的学习曲线较陡峭,适用于高性能和低级控制的场景。
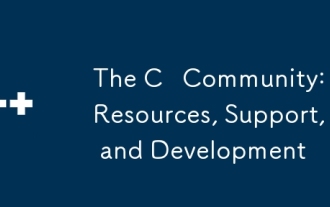
C 學習者和開發者可以從StackOverflow、Reddit的r/cpp社區、Coursera和edX的課程、GitHub上的開源項目、專業諮詢服務以及CppCon等會議中獲得資源和支持。 1.StackOverflow提供技術問題的解答;2.Reddit的r/cpp社區分享最新資訊;3.Coursera和edX提供正式的C 課程;4.GitHub上的開源項目如LLVM和Boost提陞技能;5.專業諮詢服務如JetBrains和Perforce提供技術支持;6.CppCon等會議有助於職業
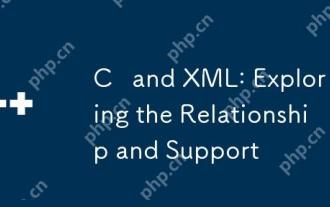
C 通過第三方庫(如TinyXML、Pugixml、Xerces-C )與XML交互。 1)使用庫解析XML文件,將其轉換為C 可處理的數據結構。 2)生成XML時,將C 數據結構轉換為XML格式。 3)在實際應用中,XML常用於配置文件和數據交換,提升開發效率。
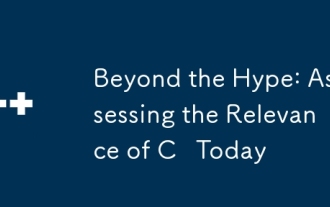
C 在現代編程中仍然具有重要相關性。 1)高性能和硬件直接操作能力使其在遊戲開發、嵌入式系統和高性能計算等領域佔據首選地位。 2)豐富的編程範式和現代特性如智能指針和模板編程增強了其靈活性和效率,儘管學習曲線陡峭,但其強大功能使其在今天的編程生態中依然重要。
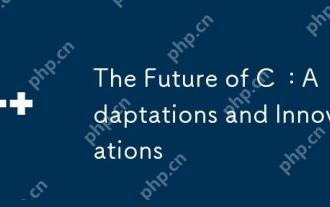
C 的未來將專注於並行計算、安全性、模塊化和AI/機器學習領域:1)並行計算將通過協程等特性得到增強;2)安全性將通過更嚴格的類型檢查和內存管理機制提升;3)模塊化將簡化代碼組織和編譯;4)AI和機器學習將促使C 適應新需求,如數值計算和GPU編程支持。
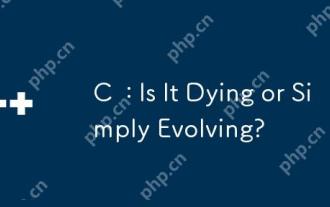
1)c relevantduetoItsAverity and效率和效果臨界。 2)theLanguageIsconTinuellyUped,withc 20introducingFeaturesFeaturesLikeTuresLikeSlikeModeLeslikeMeSandIntIneStoImproutiMimproutimprouteverusabilityandperformance.3)
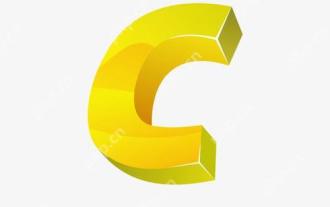
靜態分析在C 中的應用主要包括發現內存管理問題、檢查代碼邏輯錯誤和提高代碼安全性。 1)靜態分析可以識別內存洩漏、雙重釋放和未初始化指針等問題。 2)它能檢測未使用變量、死代碼和邏輯矛盾。 3)靜態分析工具如Coverity能發現緩衝區溢出、整數溢出和不安全API調用,提升代碼安全性。
