如何在C#中有效地列出列表?
高效隨機排序C#列表
在C#中,對泛型列表進行隨機排序是軟件開發中常見的任務。例如,在彩票應用程序中,您可能需要對有限的數字集合進行隨機排序。
最佳實踐:
在C#中,隨機排序列表最有效的方法是使用基於Fisher-Yates洗牌算法的擴展方法。下面提供的此方法確保高效且真正隨機地洗牌列表元素:
private static Random rng = new Random(); public static void Shuffle<T>(this IList<T> list) { int n = list.Count; while (n > 1) { n--; int k = rng.Next(n + 1); T value = list[k]; list[k] = list[n]; list[n] = value; } }
使用方法:
使用此擴展方法很簡單。考慮以下示例:
List<Product> products = GetProducts(); products.Shuffle();
隨機數生成器的選擇:
雖然上面的代碼使用System.Random
方法,但必須承認其在隨機性方面的潛在局限性。如果您的應用程序需要更高質量的隨機性,您可以利用System.Security.Cryptography
中提供的隨機數生成器,如下所示:
using System.Security.Cryptography; ... public static void Shuffle<T>(this IList<T> list) { using (RNGCryptoServiceProvider provider = new RNGCryptoServiceProvider()) { int n = list.Count; while (n > 1) { byte[] box = new byte[1]; do provider.GetBytes(box); while (!(box[0] < (byte)((double)byte.MaxValue * (double)n / (double)uint.MaxValue))); int k = (int)(box[0] / ((double)byte.MaxValue / n)); T value = list[k]; list[k] = list[n - 1]; list[n - 1] = value; } } }
線程安全:
為了確保線程安全的隨機化,必須考慮以下修改後的擴展方法:
public static void Shuffle<T>(this IList<T> list) { int n = list.Count; while (n > 1) { n--; int k = ThreadSafeRandom.ThisThreadsRandom.Next(n + 1); T value = list[k]; list[k] = list[n]; list[n] = value; } }
其中ThreadSafeRandom
定義為:
public static class ThreadSafeRandom { [ThreadStatic] private static Random Local; public static Random ThisThreadsRandom { get { return Local ?? (Local = new Random(unchecked(Environment.TickCount * 31 + Thread.CurrentThread.ManagedThreadId))); } } }
通過這些方法,您可以根據您的需求選擇最合適的隨機排序方法。 請注意using
語句在使用RNGCryptoServiceProvider
時的重要性,以確保正確釋放資源。
以上是如何在C#中有效地列出列表?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
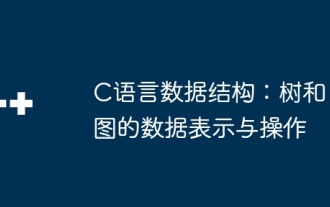
C語言數據結構:樹和圖的數據表示與操作樹是一個層次結構的數據結構由節點組成,每個節點包含一個數據元素和指向其子節點的指針二叉樹是一種特殊類型的樹,其中每個節點最多有兩個子節點數據表示structTreeNode{intdata;structTreeNode*left;structTreeNode*right;};操作創建樹遍歷樹(先序、中序、後序)搜索樹插入節點刪除節點圖是一個集合的數據結構,其中的元素是頂點,它們通過邊連接在一起邊可以是帶權或無權的數據表示鄰
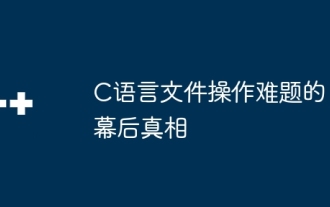
文件操作難題的真相:文件打開失敗:權限不足、路徑錯誤、文件被佔用。數據寫入失敗:緩衝區已滿、文件不可寫、磁盤空間不足。其他常見問題:文件遍歷緩慢、文本文件編碼不正確、二進製文件讀取錯誤。
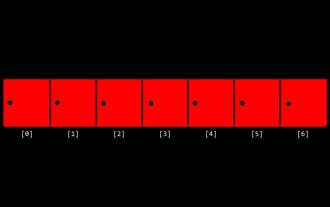
算法是解決問題的指令集,其執行速度和內存佔用各不相同。編程中,許多算法都基於數據搜索和排序。本文將介紹幾種數據檢索和排序算法。線性搜索假設有一個數組[20,500,10,5,100,1,50],需要查找數字50。線性搜索算法會逐個檢查數組中的每個元素,直到找到目標值或遍歷完整個數組。算法流程圖如下:線性搜索的偽代碼如下:檢查每個元素:如果找到目標值:返回true返回falseC語言實現:#include#includeintmain(void){i
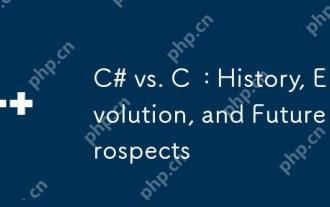
C#和C 的歷史與演變各有特色,未來前景也不同。 1.C 由BjarneStroustrup在1983年發明,旨在將面向對象編程引入C語言,其演變歷程包括多次標準化,如C 11引入auto關鍵字和lambda表達式,C 20引入概念和協程,未來將專注於性能和系統級編程。 2.C#由微軟在2000年發布,結合C 和Java的優點,其演變注重簡潔性和生產力,如C#2.0引入泛型,C#5.0引入異步編程,未來將專注於開發者的生產力和雲計算。
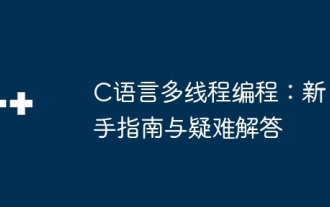
C語言多線程編程指南:創建線程:使用pthread_create()函數,指定線程ID、屬性和線程函數。線程同步:通過互斥鎖、信號量和條件變量防止數據競爭。實戰案例:使用多線程計算斐波那契數,將任務分配給多個線程並同步結果。疑難解答:解決程序崩潰、線程停止響應和性能瓶頸等問題。
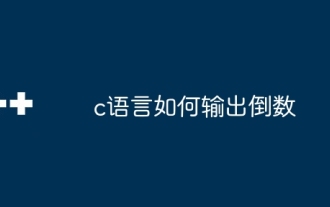
如何在 C 語言中輸出倒數?回答:使用循環語句。步驟:1. 定義變量 n 存儲要輸出的倒數數字;2. 使用 while 循環持續打印 n 直到 n 小於 1;3. 在循環體內,打印出 n 的值;4. 在循環末尾,將 n 減去 1 以輸出下一個更小的倒數。
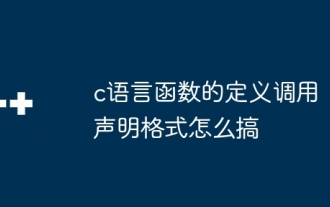
C語言函數包含定義、調用和聲明。函數定義指定函數名、參數和返回類型,函數體實現功能;函數調用執行函數並提供參數;函數聲明告知編譯器函數類型。值傳遞用於參數傳遞,注意返回類型,保持一致的代碼風格,並在函數中處理錯誤。掌握這些知識有助於編寫優雅、健壯的C代碼。
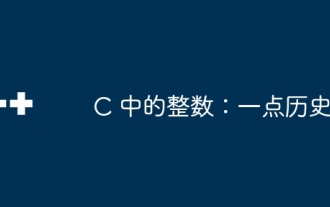
整數是編程中最基礎的數據類型,堪稱編程的基石。程序員的工作就是賦予這些數字意義,無論軟件多麼複雜,最終都歸結於整數運算,因為處理器只理解整數。為了表示負數,我們引入了二進制補碼;為了表示小數,我們創造了科學計數法,於是有了浮點數。但歸根結底,一切仍然離不開0和1。整數的簡史在C語言中,int幾乎是默認類型。儘管編譯器可能會發出警告,但在許多情況下,你仍然可以寫下這樣的代碼:main(void){return0;}從技術角度來看,這與以下代碼等效:intmain(void){return0;}這種
