在WooCommerce中設定最低結帳要求
>您想在客戶結帳之前在WooCommerce商店中設置某種最低要求。接下來是有關如何設置這些要求和限制的指南,而無需完全使用任何插件:
- 設置每個訂單的最小重量要求
- 設置最少每訂單所需的產品數量
- 設置最小數量
- 設置每個訂單的最低美元金額
鑰匙要點
-
在不使用插件的情況下,可以在WooCommerce中設置最低要求,從而可以更好地控制和自定義結帳過程。這包括設置每個訂單的最小重量要求,每訂單所需的最低產品數量,每種產品的最低數量以及每訂單的最低美元金額。
- 該過程涉及使用WooCommerce提供的“ Woocommerce_check_cart_items”操作來運行函數和執行代碼。該代碼應放置在主題的functions.php文件中,並與WordPress和WooCommerce的最新版本兼容。
- > 特定的WooCommerce功能可用於設置最低要求,例如重量,產品數量,產品數量和總購物車價值。每個要求都有其自己的功能,該功能會檢查購物車項目並將其比較它們與設定的最低要求,如果不滿足要求,請顯示錯誤消息。 WooCommerce還提供了為特定產品或類別設置最小和最大數量的靈活性,在產品頁面上顯示數量要求,並以編程方式更新產品庫存。這些可以通過內置功能或諸如“ WooCommerce Min/Max Wartities”和“ WooCommerce數量增量”之類的插件來實現。
- >本文中使用的方法
- >總是有一種不止一種在WooCommerce中設定最低要求的方法;結果甚至可能是相同的。但是我認為下面描述的方法是正確(或更好)這樣做的方法。始終歡迎並獲得有關如何完成這些任務或進一步改進的任何建議。
>以下代碼已在可用於WordPress(3.9.1)和WooCommerce(2.1.12)的最新版本中進行了測試。安裝插件時,我們將使用為WooCommerce提供的虛擬數據。該代碼應輸入您的主題函數。 php文件,並且對其進行了大量評論,因此在需要時更容易遵循和/或修改。
>>我們將使用WooCommerce_Check_cart_items操作由WooCommerce提供的操作來運行我們的功能並執行我們的代碼。訪問以下鏈接以獲取WooCommerce操作和過濾器的完整列表,也稱為掛鉤。
設置每個訂單的最小重量要求
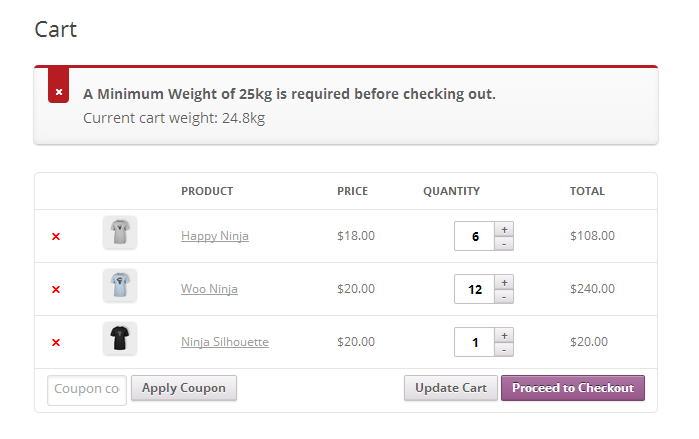
>通常限制客戶完成結帳過程而不滿足最小權重要求是有用的。減小的重量要求可以幫助您的運輸成本更易於管理,並且運輸過程更加精簡。不要忘記將最小的重量要求更改為最適合您的一切,並記住,在WooCommerce下設置的任何重量單元 - >設置 - > Products-> Products。
<span>// Set a minimum weight requirement before checking out </span><span>add_action( 'woocommerce_check_cart_items', 'spyr_set_weight_requirements' ); </span><span>function spyr_set_weight_requirements() { </span> <span>// Only run in the Cart or Checkout pages </span> <span>if( is_cart() || is_checkout() ) { </span> <span>global $woocommerce; </span> <span>// Set the minimum weight before checking out </span> <span>$minimum_weight = 25; </span> <span>// Get the Cart's content total weight </span> <span>$cart_contents_weight = WC()->cart->cart_contents_weight; </span> <span>// Compare values and add an error is Cart's total weight </span> <span>// happens to be less than the minimum required before checking out. </span> <span>// Will display a message along the lines of </span> <span>// A Minimum Weight of 25kg is required before checking out. (Cont. below) </span> <span>// Current cart weight: 12.5kg </span> <span>if( $cart_contents_weight < $minimum_weight ) { </span> <span>// Display our error message </span> <span>wc_add_notice( sprintf('<strong>A Minimum Weight of %s%s is required before checking out.</strong>' </span> <span>. '<br />Current cart weight: %s%s', </span> <span>$minimum_weight, </span> <span>get_option( 'woocommerce_weight_unit' ), </span> <span>$cart_contents_weight, </span> <span>get_option( 'woocommerce_weight_unit' ), </span> <span>get_permalink( wc_get_page_id( 'shop' ) ) </span> <span>), </span> <span>'error' ); </span> <span>} </span> <span>} </span><span>}</span>
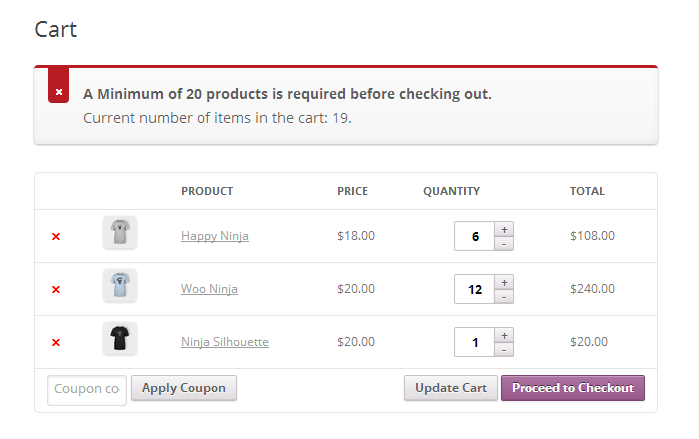
<span>// Set a minimum number of products requirement before checking out </span><span>add_action( 'woocommerce_check_cart_items', 'spyr_set_min_num_products' ); </span><span>function spyr_set_min_num_products() { </span> <span>// Only run in the Cart or Checkout pages </span> <span>if( is_cart() || is_checkout() ) { </span> <span>global $woocommerce; </span> <span>// Set the minimum number of products before checking out </span> <span>$minimum_num_products = 20; </span> <span>// Get the Cart's total number of products </span> <span>$cart_num_products = WC()->cart->cart_contents_count; </span> <span>// Compare values and add an error is Cart's total number of products </span> <span>// happens to be less than the minimum required before checking out. </span> <span>// Will display a message along the lines of </span> <span>// A Minimum of 20 products is required before checking out. (Cont. below) </span> <span>// Current number of items in the cart: 6 </span> <span>if( $cart_num_products < $minimum_num_products ) { </span> <span>// Display our error message </span> <span>wc_add_notice( sprintf( '<strong>A Minimum of %s products is required before checking out.</strong>' </span> <span>. '<br />Current number of items in the cart: %s.', </span> <span>$minimum_num_products, </span> <span>$cart_num_products ), </span> <span>'error' ); </span> <span>} </span> <span>} </span><span>}</span>
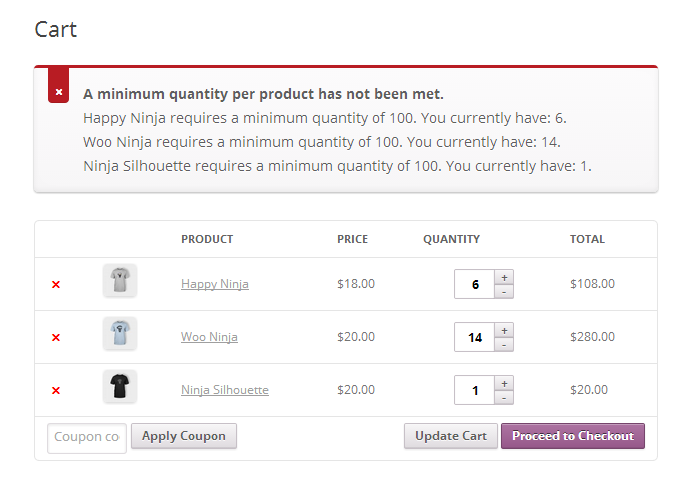
要設置這些限制,您需要創建一個數組,該數組將您的規則/限制保存在另一個數組中。編輯此數組時要小心,並確保准確輸入所有代碼,以防止錯誤和意外結果。您需要使用的格式如下:
>
<span>// Product Id and Min. Quantities per Product </span><span>// id = Product ID </span><span>// min = Minimum quantity </span><span>$product_min_qty = array( </span> <span>array( 'id' => 47, 'min' => 100 ), </span> <span>array( 'id' => 37, 'min' => 100 ), </span> <span>array( 'id' => 34, 'min' => 100 ), </span> <span>array( 'id' => 31, 'min' => 100 ), </span><span>);</span>
>
<span>// Set minimum quantity per product before checking out </span><span>add_action( 'woocommerce_check_cart_items', 'spyr_set_min_qty_per_product' ); </span><span>function spyr_set_min_qty_per_product() { </span> <span>// Only run in the Cart or Checkout pages </span> <span>if( is_cart() || is_checkout() ) { </span> <span>global $woocommerce; </span> <span>// Product Id and Min. Quantities per Product </span> <span>$product_min_qty = array( </span> <span>array( 'id' => 47, 'min' => 100 ), </span> <span>array( 'id' => 37, 'min' => 100 ), </span> <span>array( 'id' => 34, 'min' => 100 ), </span> <span>array( 'id' => 31, 'min' => 100 ), </span> <span>); </span> <span>// Will increment </span> <span>$i = 0; </span> <span>// Will hold information about products that have not </span> <span>// met the minimum order quantity </span> <span>$bad_products = array(); </span> <span>// Loop through the products in the Cart </span> <span>foreach( $woocommerce->cart->cart_contents as $product_in_cart ) { </span> <span>// Loop through our minimum order quantities per product </span> <span>foreach( $product_min_qty as $product_to_test ) { </span> <span>// If we can match the product ID to the ID set on the minimum required array </span> <span>if( $product_to_test['id'] == $product_in_cart['product_id'] ) { </span> <span>// If the quantity required is less than than the quantity in the cart now </span> <span>if( $product_in_cart['quantity'] < $product_to_test['min'] ) { </span> <span>// Get the product ID </span> <span>$bad_products[$i]['id'] = $product_in_cart['product_id']; </span> <span>// Get the Product quantity already in the cart for this product </span> <span>$bad_products[$i]['in_cart'] = $product_in_cart['quantity']; </span> <span>// Get the minimum required for this product </span> <span>$bad_products[$i]['min_req'] = $product_to_test['min']; </span> <span>} </span> <span>} </span> <span>} </span> <span>// Increment $i </span> <span>$i++; </span> <span>} </span> <span>// Time to build our error message to inform the customer </span> <span>// About the minimum quantity per order. </span> <span>if( is_array( $bad_products) && count( $bad_products ) > 1 ) { </span> <span>// Lets begin building our message </span> <span>$message = '<strong>A minimum quantity per product has not been met.</strong><br />'; </span> <span>foreach( $bad_products as $bad_product ) { </span> <span>// Append to the current message </span> <span>$message .= get_the_title( $bad_product['id'] ) .' requires a minimum quantity of ' </span> <span>. $bad_product['min_req'] </span> <span>.'. You currently have: '. $bad_product['in_cart'] .'.<br />'; </span> <span>} </span> <span>wc_add_notice( $message, 'error' ); </span> <span>} </span> <span>} </span><span>}</span>
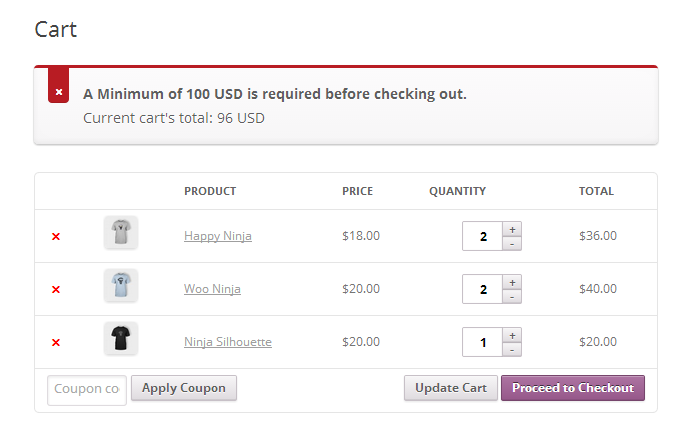
結論
<span>// Set a minimum dollar amount per order </span><span>add_action( 'woocommerce_check_cart_items', 'spyr_set_min_total' ); </span><span>function spyr_set_min_total() { </span> <span>// Only run in the Cart or Checkout pages </span> <span>if( is_cart() || is_checkout() ) { </span> <span>global $woocommerce; </span> <span>// Set minimum cart total </span> <span>$minimum_cart_total = 10; </span> <span>// Total we are going to be using for the Math </span> <span>// This is before taxes and shipping charges </span> <span>$total = WC()->cart->subtotal; </span> <span>// Compare values and add an error is Cart's total </span> <span>// happens to be less than the minimum required before checking out. </span> <span>// Will display a message along the lines of </span> <span>// A Minimum of 10 USD is required before checking out. (Cont. below) </span> <span>// Current cart total: 6 USD </span> <span>if( $total <= $minimum_cart_total ) { </span> <span>// Display our error message </span> <span>wc_add_notice( sprintf( '<strong>A Minimum of %s %s is required before checking out.</strong>' </span> <span>.'<br />Current cart\'s total: %s %s', </span> <span>$minimum_cart_total, </span> <span>get_option( 'woocommerce_currency'), </span> <span>$total, </span> <span>get_option( 'woocommerce_currency') ), </span> <span>'error' ); </span> <span>} </span> <span>} </span><span>}</span>
您是否可以看到,WooCommerce允許您使用操作和過濾器來更改正常的結帳過程。所有商店都是不同的,並且能夠在需要時設置這些限制至關重要。對於需要完成此類任務的我們的開發人員來說,知道如何做至關重要。
感謝您的閱讀,評論或有關如何改進代碼的建議。如果您對特定的WooCommerce修改有想法,請不要害羞,並在評論中發布鏈接。
wooCommerce中有關最低結帳要求的常見問題>我如何在WooCommerce中為特定產品設置最小數量? >
>
為WooCommerce中的特定產品設置最小數量,您需要導航到您想要的產品數據部分設置最小數量。在“庫存”選項卡下,您將找到設置“最小數量”的選項。輸入所需的號碼並保存您的更改。這將確保客戶必須至少購買這一數量的產品才能進行結帳。
我可以在WooCommerce中設置最大數量嗎?用於WooCommerce中的產品。類似於設置最小數量,您可以從“庫存”選項卡下的“產品數據”部分中執行此操作。在那裡,您將找到設置“最大數量”的選項。輸入所需的號碼並保存您的更改。這將限制客戶可以單訂單購買的每種產品的數量。
>
>如何在WooCommerce中以編程方式更新產品庫存?可以通過使用WooCommerce的內置功能來完成編程中的產品庫存。您可以使用WC_UPDATE_PRODUCT_STOCK()函數來更新產品的庫存數量。此功能採用兩個參數:產品ID和新的庫存數量。 >我可以在WooCommerce中設置最低訂單數量嗎?這可以通過使用“ WooCommerce Min/Max Wentities”之類的插件來完成。安裝和激活後,您可以從插件的設置中設置最低訂單金額。這將要求客戶在結帳之前達到此最低訂單金額。 >我如何設置WooCommerce中產品的數量增量?
>我可以為不同產品設置不同的最低和最大數量嗎?這可以從每個產品的產品數據部分完成。在“庫存”選項卡下,您可以單獨設置每種產品的“最小數量”和“最大數量”。
可以為產品的變化設置最小和最大數量嗎?
>是的,可以為WooCommerce中產品的變化設置最小和最大數量。這可以從產品數據的變體部分完成。對於每種變體,您都可以設置“最小數量”和“最大數量”。 >如何在產品頁面上顯示最小和最大數量要求?產品頁面上的數量要求可以使用諸如“ WooCommerce Min/Max Nutities”之類的插件來完成。此插件在產品頁面上添加了一個通知,該頁面顯示了產品的最小和最大數量要求。>我可以為特定類別的產品設置最小和最大數量嗎?為WooCommerce中的特定類別產品設置最小和最大數量。這可以通過使用“ WooCommerce Min/Max Wentities”之類的插件來完成。安裝和激活後,您可以從插件的設置中為每個產品類別設置最小和最大數量。
以上是在WooCommerce中設定最低結帳要求的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
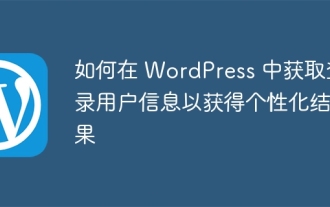
最近,我們向您展示瞭如何通過允許用戶將自己喜歡的帖子保存在個性化庫中來為用戶創建個性化體驗。您可以通過在某些地方(即歡迎屏幕)使用他們的名字,將個性化結果提升到另一個水平。幸運的是,WordPress使獲取登錄用戶的信息變得非常容易。在本文中,我們將向您展示如何檢索與當前登錄用戶相關的信息。我們將利用get_currentuserinfo(); 功能。這可以在主題中的任何地方使用(頁眉、頁腳、側邊欄、頁面模板等)。為了使其工作,用戶必須登錄。因此我們需要使用
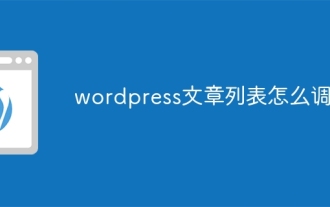
有四種方法可以調整 WordPress 文章列表:使用主題選項、使用插件(如 Post Types Order、WP Post List、Boxy Stuff)、使用代碼(在 functions.php 文件中添加設置)或直接修改 WordPress 數據庫。
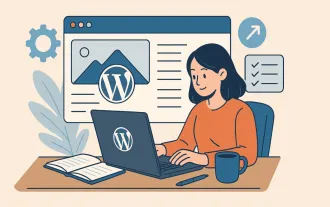
博客是人們在網上表達觀點、意見和見解的理想平台。許多新手渴望建立自己的網站,卻因擔心技術障礙或成本問題而猶豫不決。然而,隨著平台不斷發展以滿足初學者的能力和需求,現在開始變得比以往任何時候都更容易。 本文將逐步指導您如何建立一個WordPress博客,從主題選擇到使用插件提升安全性和性能,助您輕鬆創建自己的網站。 選擇博客主題和方向 在購買域名或註冊主機之前,最好先確定您計劃涵蓋的主題。個人網站可以圍繞旅行、烹飪、產品評論、音樂或任何激發您興趣的愛好展開。專注於您真正感興趣的領域可以鼓勵持續寫作
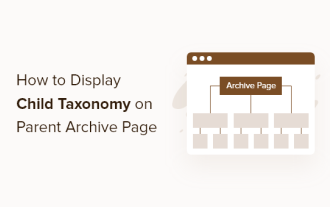
您想了解如何在父分類存檔頁面上顯示子分類嗎?在自定義分類存檔頁面時,您可能需要執行此操作,以使其對訪問者更有用。在本文中,我們將向您展示如何在父分類存檔頁面上輕鬆顯示子分類。為什麼在父分類存檔頁面上顯示子分類?通過在父分類存檔頁面上顯示所有子分類,您可以使其不那麼通用,對訪問者更有用。例如,如果您運行一個關於書籍的WordPress博客,並且有一個名為“主題”的分類法,那麼您可以添加“小說”、“非小說”等子分類法,以便您的讀者可以
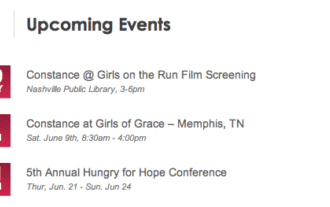
過去,我們分享過如何使用PostExpirator插件使WordPress中的帖子過期。好吧,在創建活動列表網站時,我們發現這個插件非常有用。我們可以輕鬆刪除過期的活動列表。其次,多虧了這個插件,按帖子過期日期對帖子進行排序也非常容易。在本文中,我們將向您展示如何在WordPress中按帖子過期日期對帖子進行排序。更新了代碼以反映插件中更改自定義字段名稱的更改。感謝Tajim在評論中讓我們知道。在我們的特定項目中,我們將事件作為自定義帖子類型。現在
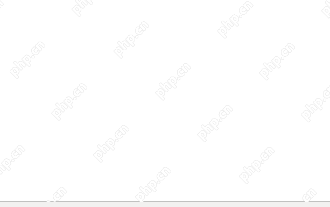
您是否正在尋找自動化 WordPress 網站和社交媒體帳戶的方法? 通過自動化,您將能夠在 Facebook、Twitter、LinkedIn、Instagram 等平台上自動分享您的 WordPress 博客文章或更新。 在本文中,我們將向您展示如何使用 IFTTT、Zapier 和 Uncanny Automator 輕鬆實現 WordPress 和社交媒體的自動化。 為什麼要自動化 WordPress 和社交媒體? 自動化您的WordPre
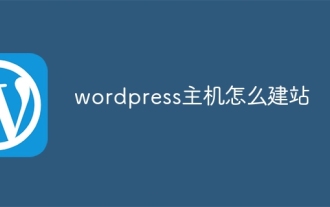
要使用 WordPress 主機建站,需要:選擇一個可靠的主機提供商。購買一個域名。設置 WordPress 主機帳戶。選擇一個主題。添加頁面和文章。安裝插件。自定義您的網站。發布您的網站。
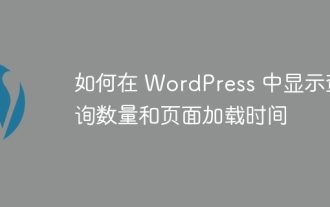
我們的一位用戶詢問其他網站如何在頁腳中顯示查詢數量和頁面加載時間。您經常會在網站的頁腳中看到這一點,它可能會顯示類似以下內容:“1.248秒內64個查詢”。在本文中,我們將向您展示如何在WordPress中顯示查詢數量和頁面加載時間。只需將以下代碼粘貼到主題文件中您喜歡的任何位置(例如footer.php)。 queriesin
