在Python中編寫專業的單位測試
單元測試是構建可靠軟件的基礎。測試類型多種多樣,但單元測試最為重要。單元測試使您可以放心地將經過充分測試的代碼片段作為基本單元,並在構建程序時依賴它們。它們擴展了您對可信代碼的儲備,超越了語言內置功能和標準庫的範圍。此外,Python 對編寫單元測試提供了強大的支持。
運行示例
在深入探討所有原則、啟發式方法和指南之前,讓我們先來看一個實際的單元測試示例。
創建一個名為 python_tests 的新目錄,並添加兩個文件:
- car.py
- test_car.py
通過添加 init.py 文件,將該目錄設為 Python 包。文件的結構應如下所示:
<code>python_tests/ -__init__.py - car.py - test_car.py</code>
car.py 文件將用於編寫我們在此示例中使用的自動駕駛汽車程序的邏輯,而 test_car.py 文件將用於編寫所有測試。
car.py 文件內容:
class SelfDrivingCar: def __init__(self): self.speed = 0 self.destination = None def _accelerate(self): self.speed += 1 def _decelerate(self): if self.speed > 0: self.speed -= 1 def _advance_to_destination(self): distance = self._calculate_distance_to_object_in_front() if distance < 1: # 假设距离单位为米 self.stop() elif distance < 5: self._decelerate() elif self.speed < self._get_speed_limit(): self._accelerate() def _has_arrived(self): pass # 需要实现到达目的地判断逻辑 def _calculate_distance_to_object_in_front(self): pass # 需要实现计算前方物体距离的逻辑 def _get_speed_limit(self): pass # 需要实现获取速度限制的逻辑 def stop(self): self.speed = 0 def drive(self, destination): self.destination = destination while not self._has_arrived(): self._advance_to_destination() self.stop()
這是一個針對 TestCase 類的單元測試。如下所示,獲取 unittest 模塊。
from unittest import TestCase
然後,您可以重寫 unittest 測試框架提供的 unittest.main 模塊,方法是在測試文件的底部添加以下測試腳本。
if __name__ == '__main__': unittest.main()
繼續並在 test_car.py 文件的底部添加測試腳本,如下所示。
import unittest from car import SelfDrivingCar class SelfDrivingCarTest(unittest.TestCase): def setUp(self): self.car = SelfDrivingCar() def test_stop(self): self.car.speed = 5 self.car.stop() self.assertEqual(0, self.car.speed) self.car.stop() self.assertEqual(0, self.car.speed) if __name__ == '__main__': unittest.main(verbosity=2)
要運行測試,請運行 Python 程序:
python test_car.py
您應該看到以下輸出:
<code>test_stop (__main__.SelfDrivingCarTest) ... ok ---------------------------------------------------------------------- Ran 1 test in 0.000s OK</code>
測試發現
另一種方法,也是最簡單的方法,是測試發現。此選項僅在 Python 2.7 中添加。在 2.7 之前,您可以使用 nose 來發現和運行測試。 Nose 還有一些其他優點,例如運行測試函數而無需為測試用例創建類。但就本文而言,讓我們堅持使用 unittest。
顧名思義,-v 標誌:
SelfDrivingCarTest。
有幾個標誌可以控制操作:
python -m unittest -h
測試覆蓋率
測試覆蓋率是一個經常被忽視的領域。覆蓋率是指您的測試實際上測試了多少代碼。例如,如果您有一個帶有 if 語句的函數,則需要編寫測試來覆蓋 if 語句的真值和假值分支。理想情況下,您的代碼應該在一個包中。每個包的測試應該在包的同級目錄中。在測試目錄中,應該為包的每個模塊提供一個名為 unittest 模塊的文件。
結論
單元測試是可靠代碼的基礎。在本教程中,我探討了單元測試的一些原則和指南,並解釋了幾個最佳實踐背後的原因。您構建的系統越大,單元測試就越重要。但是單元測試是不夠的。大型系統還需要其他類型的測試:集成測試、性能測試、負載測試、滲透測試、驗收測試等。
此文章已更新,並包含 Esther Vaati 的貢獻。 Esther 是 Envato Tuts 的軟件開發人員和撰稿人。
以上是在Python中編寫專業的單位測試的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
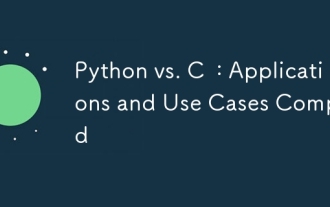
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
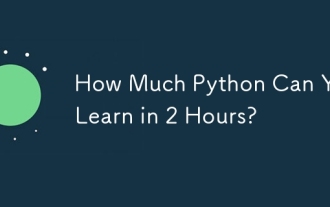
兩小時內可以學到Python的基礎知識。 1.學習變量和數據類型,2.掌握控制結構如if語句和循環,3.了解函數的定義和使用。這些將幫助你開始編寫簡單的Python程序。
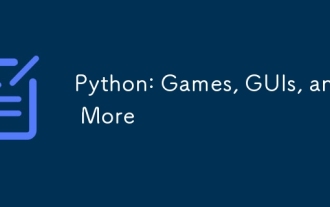
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
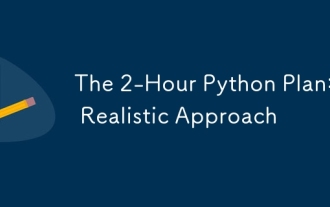
2小時內可以學會Python的基本編程概念和技能。 1.學習變量和數據類型,2.掌握控制流(條件語句和循環),3.理解函數的定義和使用,4.通過簡單示例和代碼片段快速上手Python編程。
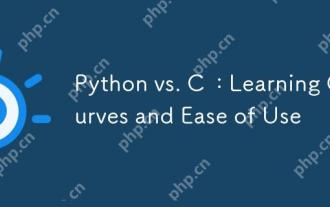
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
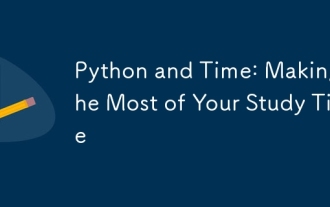
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
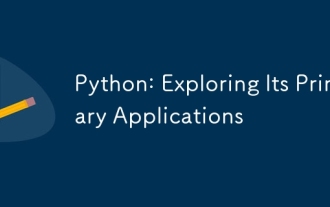
Python在web開發、數據科學、機器學習、自動化和腳本編寫等領域有廣泛應用。 1)在web開發中,Django和Flask框架簡化了開發過程。 2)數據科學和機器學習領域,NumPy、Pandas、Scikit-learn和TensorFlow庫提供了強大支持。 3)自動化和腳本編寫方面,Python適用於自動化測試和系統管理等任務。
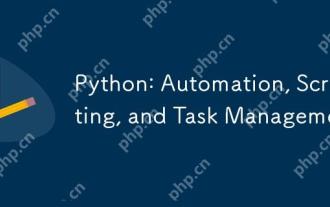
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
