javascript与CSS复习(《精通javascript》)_javascript技巧
如:elem.style.height 或者 elem.style.height = '100px', 这里要注意的是设置任何几何属性必须明确尺寸单位(如px),同时任何几何属性返回的是表示样式的字符串而非数值(如'100px'而非100)。另外像elem.style.height这样的操作,也能获取元素style属性中设置的样式值,如果你把样式统一放在css文件中,上述方法只会返回一个空串。为了获取元素真实、最终的样式,书中给出了一个函数
//get a style property (name) of a specific element (elem)
function getStyle(elem, name) {
// if the property exists in style[], then it's been set
//recently (and is current)
if(elem.style[name]) return elem.style[name];
//otherwise, try to use IE's method
else if (elem.currentStyle) return elem.currentStyle[name];
//Or the W3C's method, if it exists
else if (document.defaultView && document.defaultView.getComputedStyle) {
//it uses the traditional 'text-align' style of rule writing
//instead of textAlign
name = name.replace(/[A-Z]/g, '-$1');
name = name.toLowerCase();
//get the style object and get the value of the property ( if it exists)
var s = document.defaultView.getComputedStyle(elem,'');
return s && s.getPropertyValue(name);
} else return null;
}
理解如何获取元素的在页面的位置是构造交互效果的关键。先复习下css中position属性值的特点。
static:静态定位,这是元素定位的默认方式,它简单的遵循文档流。但元素静态定位时,top和left属性无效。
relative:相对定位,元素会继续遵循文档流,除非受到其他指令的影响。top和left属性的设置会引起元素相对于它的原始位置进行偏移。
absolute:绝对定位,绝对定位的元素完全摆脱文档流,它会相对于它的第一个非静态定位的祖先元素而展示,如果没有这样的祖先元素,它的定位将相对于整个文档。
fixed:固定定位把元素相对于浏览器窗口而定位。它完全忽略浏览器滚动条的拖动。
作者封装了一个跨浏览器的获取元素页面位置的函数
其中有几个重要元素的属性:offsetParent,offsetLeft,offsetTop(可直接点击到Mozilla Developer Center的相关页面)
//find the x (horizontal, Left) position of an element
function pageX(elem) {
//see if we're at the root element, or not
return elem.offsetParent ?
//if we can still go up, add the current offset and recurse upwards
elem.offsetLeft + pageX(elem.offsetParent) :
//otherwise, just get the current offset
elem.offsetLeft;
}
//find the y (vertical, top) position of an element
function pageY(elem) {
//see if we're at the root element, or not
return elem.offsetParent ?
//if we can still go up, add the current offset and recurse upwards
elem.offsetTop + pageY(elem.offsetParent) :
//otherwise, just get the current offset
elem.offsetTop;
}
我们接着要获得元素相对于它父亲的水平和垂直位置,使用元素相对于父亲的位置,就可以为DOM增加额外的元素,并相对定位于它的父亲。
//find the horizontal position of an element within its parent
function parentX(elem) {
//if the offsetParent is the element's parent, break early
return elem.parentNode == elem.offsetParent ?
elem.offsetLeft :
// otherwise, we need to find the position relative to the entire
// page for both elements, and find the difference
pageX(elem) - pageX(elem.parentNode);
}
//find the vertical positioning of an element within its parent
function parentY(elem) {
//if the offsetParent is the element's parent, break early
return elem.parentNode == elem.offsetParent ?
elem.offsetTop :
// otherwise, we need to find the position relative to the entire
// page for both elements, and find the difference
pageY(elem) - pageY(elem.parentNode);
}
元素位置的最后一个问题,获取元素当对于css定位(非static)容器的位置,有了getStyle这个问题很好解决
//find the left position of an element
function posX(elem) {
//get the computed style and get the number out of the value
return parseInt(getStyle(elem, 'left'));
}
//find the top position of an element
function posY(elem) {
//get the computed style and get the number out of the value
return parseInt(getStyle(elem, 'top'));
}
接着是设置元素的位置,这个很简单。
//a function for setting the horizontal position of an element
function setX(elem, pos) {
//set the 'left' css property, using pixel units
elem.style.left = pos + 'px';
}
//a function for setting the vertical position of an element
function setY(elem, pos) {
//set the 'top' css property, using pixel units
elem.style.top = pos + 'px';
}
再来两个函数,用于调准元素的当前位置,在动画效果中很实用
//a function for adding a number of pixels to the horizontal
//position of an element
function addX(elem, pos) {
//get the current horz. position and add the offset to it
setX(elem, posX(elem) + pos);
}
//a function that can be used to add a number of pixels to the
//vertical position of an element
function addY(elem, pos) {
//get the current vertical position and add the offset to it
setY(elem, posY(elem) + pos);
}
知道如何获取元素位置之后,我们再来看看如何获取元素的尺寸,
获取元素当前的高度和宽度
function getHeight(elem) {
return parseInt(getStyle(elem, 'height'));
}
function getWidth(elem) {
return parseInt(getStyle(elem, 'width'));
}
大多数情况下,以上的方法够用了,但是在一些动画交互中会出现问题。比如以0像素开始的动画,你需要事先知道元素究竟能有多高或多宽,其二当元素的display属性为none时,你会得不到值。这两个问题都会在执行动画的时候发生。为此作者给出了获得元素潜在高度和宽度的函数。
//查找元素完整的、可能的高度
function fullHeight(elem) {
//如果元素是显示的,那么使用offsetHeight就能得到高度,如果没有offsetHeight,则使用getHeight()
if(getStyle(elem, 'display') != 'none')
return elem.offsetHeight || getHeight(elem);
//否则,我们必须处理display为none的元素,所以重置它的css属性以获得更精确的读数
var old = resetCSS(elem, {
display:'',
visibility:'hidden',
position:'absolute'
});
//使用clientHeigh找出元素的完整高度,如果还不生效,则使用getHeight函数
var h = elem.clientHeight || getHeight(elem);
//最后,恢复其css的原有属性
restoreCSS(elem, old);
//并返回元素的完整高度
return h;
}
//查找元素完整的、可能的宽度
function fullWidth(elem) {
//如果元素是显示的,那么使用offsetWidth就能得到宽度,如果没有offsetWidth,则使用getWidth()
if(getStyle(elem, 'display') != 'none')
return elem.offsetWidth || getWidth(elem);
//否则,我们必须处理display为none的元素,所以重置它的css以获取更精确的读数
var old = resetCSS(elem, {
display:'',
visibility:'hidden',
position:'absolute'
});
//使用clientWidth找出元素的完整高度,如果还不生效,则使用getWidth函数
var w = elem.clientWidth || getWidth(elem);
//最后,恢复原有CSS
restoreCSS(elem, old);
//返回元素的完整宽度
return w;
}
//设置一组CSS属性的函数
function resetCSS(elem, prop) {
var old = {};
//遍历每个属性
for(var i in prop) {
//记录旧的属性值
old[i] = elem.style[i];
//设置新的值
elem.style[i] = prop[i];
}
return old;
}
//恢复原有CSS属性
function restoreCSS(elem, prop) {
for(var i in prop)
elem.style[i] = prop[i];
}
还有不少内容,明天继续,写写效率低下了,笔记本屏幕太小,开个pdf,写着文章老来回切换,真是。。。是时候弄个双显了!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
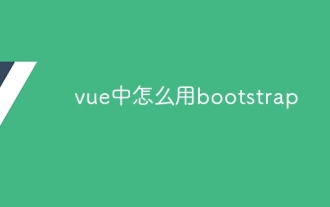
在 Vue.js 中使用 Bootstrap 分為五個步驟:安裝 Bootstrap。在 main.js 中導入 Bootstrap。直接在模板中使用 Bootstrap 組件。可選:自定義樣式。可選:使用插件。

WebDevelovermentReliesonHtml,CSS和JavaScript:1)HTMLStructuresContent,2)CSSStyleSIT和3)JavaScriptAddSstractivity,形成thebasisofmodernWebemodernWebExexperiences。

HTML定義網頁結構,CSS負責樣式和佈局,JavaScript賦予動態交互。三者在網頁開發中各司其職,共同構建豐富多彩的網站。
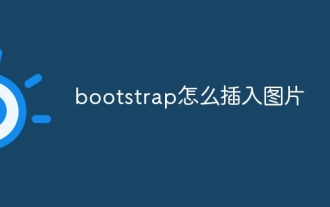
在 Bootstrap 中插入圖片有以下幾種方法:直接插入圖片,使用 HTML 的 img 標籤。使用 Bootstrap 圖像組件,可以提供響應式圖片和更多樣式。設置圖片大小,使用 img-fluid 類可以使圖片自適應。設置邊框,使用 img-bordered 類。設置圓角,使用 img-rounded 類。設置陰影,使用 shadow 類。調整圖片大小和位置,使用 CSS 樣式。使用背景圖片,使用 background-image CSS 屬性。
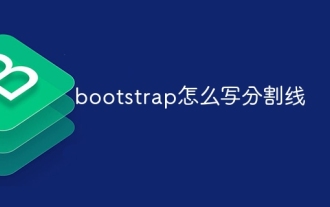
創建 Bootstrap 分割線有兩種方法:使用 標籤,可創建水平分割線。使用 CSS border 屬性,可創建自定義樣式的分割線。
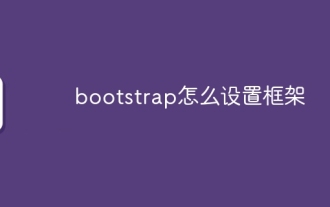
要設置 Bootstrap 框架,需要按照以下步驟:1. 通過 CDN 引用 Bootstrap 文件;2. 下載文件並將其託管在自己的服務器上;3. 在 HTML 中包含 Bootstrap 文件;4. 根據需要編譯 Sass/Less;5. 導入定製文件(可選)。設置完成後,即可使用 Bootstrap 的網格系統、組件和样式創建響應式網站和應用程序。
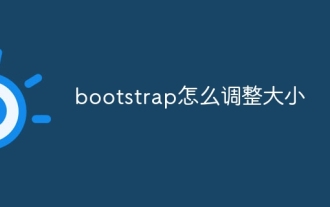
要調整 Bootstrap 中元素大小,可以使用尺寸類,具體包括:調整寬度:.col-、.w-、.mw-調整高度:.h-、.min-h-、.max-h-
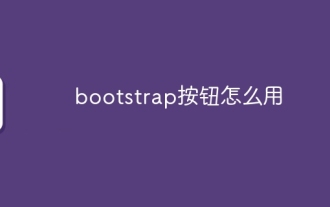
如何使用 Bootstrap 按鈕?引入 Bootstrap CSS創建按鈕元素並添加 Bootstrap 按鈕類添加按鈕文本
