PHP学习笔记7:错误和异常处理
读《PHP和MySQL Web开发》笔记合集:
1、异常处理概念
1)异常处理在 try 代码块被调用执行
try
{
//code goes here
}
2)PHP中,异常必须手动抛出
throw new Exception('message',code);
这是一个语言结构,而不是一个函数。
可以在throw子句中传递任何其他对象。
3)在try代码块之后,必须至少给出一个catch代码块。
catch ( typehint exception)
{
// handle exception
}
可以将多个catch 代码块与一个try 代码块进行关联。
例子:
try { throw new Exception("A terrible error has occurred", 42); } catch (Exception $e) { echo "Exception ". $e->getCode(). ": ". $e->getMessage()." " in ". $e->getFile(). " on line ". $e->getLine(). " } ?> |
2、Exception类
Exception (PHP 5 >= 5.1.0)
简介Exception是所有异常的基类。
类摘要
Exception { /* 属性 */ protectedstring $message ; protectedint $code ; protectedstring $file ; protectedint $line ; /* 方法 */ public __construct ([ string $message = "" [, int $code = 0 [, Exception$previous = NULL ]]] ) final public string getMessage ( void ) final public Exception getPrevious ( void ) final public int getCode ( void ) final public string getFile ( void ) final public int getLine ( void ) final public array getTrace ( void ) final public string getTraceAsString ( void ) public string __toString ( void ) final private void __clone ( void ) }
属性message异常消息内容 code异常代码 file抛出异常的文件名 line抛出异常在该文件中的行号 Table of Contents
|
用户可以扩展Exception类来创建自己的异常类。
注意,Exception类大多数公有方法都是final,不能重载,我们可以创建自己的Exception子类,但是不能改变这些基本方法的行为。但是注意,__toString()函数可以重载。
例子:
class myException extends Exception { function __toString() { return "
"; } } try { throw new myException("A terrible error has occurred", 42); } catch (myException $m) { echo $m; } ?> |
I/O部分容易出异常,通常,良好的编码习惯要求try代码块的代码量较少,并且在代码块的结束处捕获相关异常。
注意:如果一场没有匹配的catch语句块,PHP将报告一个致命错误。
例子:
// open file for appending try { if (!($fp = @fopen("$DOCUMENT_ROOT/../orders/orders.txt", 'ab'))) throw new fileOpenException(); if (!flock($fp, LOCK_EX)) throw new fileLockException(); if (!fwrite($fp, $outputstring, strlen($outputstring))) throw new fileWriteException(); flock($fp, LOCK_UN); fclose($fp); echo " Order written. ";} catch (fileOpenException $foe) { echo " Orders file could not be opened. Please contact our webmaster for help. ";} catch (Exception $e) { echo " Your order could not be processed at this time. Please try again later. ";} |
5、异常与PHP其他错误处理机制
PHP还提供了复杂的错误处理机制,注意,比如异常处理和@错误抑制并不会影响。
例子:
if (!($fp = @fopen("$DOCUMENT_ROOT/../orders/orders.txt", 'ab'))) throw new fileOpenException(); |

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
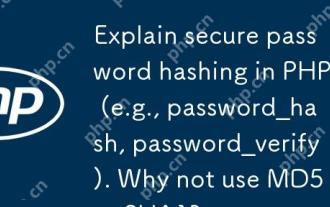
在PHP中,應使用password_hash和password_verify函數實現安全的密碼哈希處理,不應使用MD5或SHA1。1)password_hash生成包含鹽值的哈希,增強安全性。 2)password_verify驗證密碼,通過比較哈希值確保安全。 3)MD5和SHA1易受攻擊且缺乏鹽值,不適合現代密碼安全。
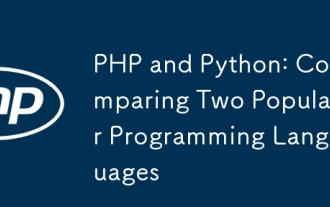
PHP和Python各有優勢,選擇依據項目需求。 1.PHP適合web開發,尤其快速開發和維護網站。 2.Python適用於數據科學、機器學習和人工智能,語法簡潔,適合初學者。
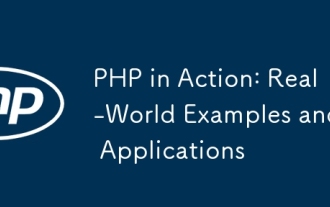
PHP在電子商務、內容管理系統和API開發中廣泛應用。 1)電子商務:用於購物車功能和支付處理。 2)內容管理系統:用於動態內容生成和用戶管理。 3)API開發:用於RESTfulAPI開發和API安全性。通過性能優化和最佳實踐,PHP應用的效率和可維護性得以提升。
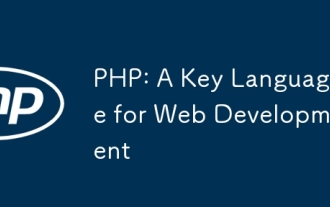
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
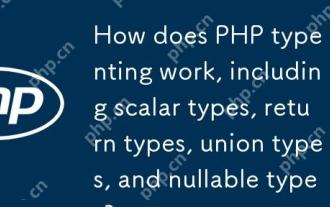
PHP類型提示提升代碼質量和可讀性。 1)標量類型提示:自PHP7.0起,允許在函數參數中指定基本數據類型,如int、float等。 2)返回類型提示:確保函數返回值類型的一致性。 3)聯合類型提示:自PHP8.0起,允許在函數參數或返回值中指定多個類型。 4)可空類型提示:允許包含null值,處理可能返回空值的函數。
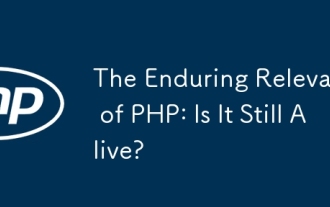
PHP仍然具有活力,其在現代編程領域中依然佔據重要地位。 1)PHP的簡單易學和強大社區支持使其在Web開發中廣泛應用;2)其靈活性和穩定性使其在處理Web表單、數據庫操作和文件處理等方面表現出色;3)PHP不斷進化和優化,適用於初學者和經驗豐富的開發者。
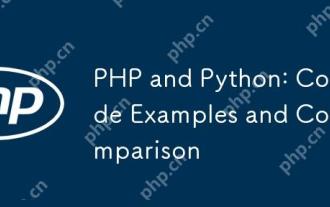
PHP和Python各有優劣,選擇取決於項目需求和個人偏好。 1.PHP適合快速開發和維護大型Web應用。 2.Python在數據科學和機器學習領域佔據主導地位。
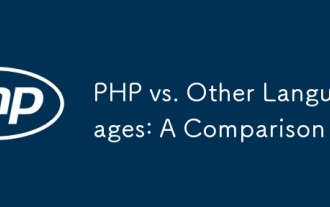
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
