[新人求救] python3.5 引用字典出錯
1.我在一份city.py 文件中收錄了城市名字對應的城市編號,並用這個編號去中國天氣網查詢數據
部分數據格式是這樣的:
<code>city = { '北京': '101010100', '海淀': '101010200', '朝阳': '101010300', '顺义': '101010400', '怀柔': '101010500', '通州': '101010600', '昌平': '101010700', '延庆': '101010800', '丰台': '101010900', '石景山': '101011000', '大兴': '101011100', '房山': '101011200', '密云': '101011300', '门头沟': '101011400', '平谷': '101011500', </code>
下面是我的程式碼
<code># -*- coding:utf-8 -*- import urllib3 import json from city import city cityname = input('您想查询哪个城市的天气?\n') citycode = city.get(cityname) if citycode: url = ('http://www.weather.com.cn/data/cityinfo/%s.html'%citycode) content = urllib3.urlopen(url).read() print(content) </code>
運行報錯 說:
<code>D:\learnpy\weather python weather.py 杭州 Traceback (most recent call last): File "weather.py", line 7, in <module> cityname = input() EOFError </code>
究竟哪一步出了問題呢?
回覆內容:
1.我在一份city.py 文件中收錄了城市名字對應的城市編號,並用這個編號去中國天氣網查詢數據
部分數據格式是這樣的:
<code>city = { '北京': '101010100', '海淀': '101010200', '朝阳': '101010300', '顺义': '101010400', '怀柔': '101010500', '通州': '101010600', '昌平': '101010700', '延庆': '101010800', '丰台': '101010900', '石景山': '101011000', '大兴': '101011100', '房山': '101011200', '密云': '101011300', '门头沟': '101011400', '平谷': '101011500', </code>
下面是我的程式碼
<code># -*- coding:utf-8 -*- import urllib3 import json from city import city cityname = input('您想查询哪个城市的天气?\n') citycode = city.get(cityname) if citycode: url = ('http://www.weather.com.cn/data/cityinfo/%s.html'%citycode) content = urllib3.urlopen(url).read() print(content) </code>
運行報錯 說:
<code>D:\learnpy\weather python weather.py 杭州 Traceback (most recent call last): File "weather.py", line 7, in <module> cityname = input() EOFError </code>
究竟哪一步出了問題呢?
EOFError
的錯誤是因為遇到了不期望的結尾, 這算是一個sublime
的 bug, 想解決可以看這裡
Running Python interactively from within Sublime Text 2
另外建議你用requests
, urllibx
系列太坑爹, 改了下你的代碼, 基本上可用
<code>import json import requests from city import city cityname = input('您想查询哪个城市的天气?\n') citycode = city[cityname] if citycode: url = ('http://www.weather.com.cn/data/cityinfo/%s.html' % citycode) content = requests.get(url) string = content.text.encode(content.encoding).decode("utf-8") print(json.dumps(json.loads(string), ensure_ascii=False, indent=4)) </code>
輸出
<code>您想查询哪个城市的天气? 北京 { "weatherinfo": { "ptime": "18:00", "weather": "晴", "cityid": "101010100", "temp2": "16℃", "temp1": "-2℃", "city": "北京", "img2": "d0.gif", "img1": "n0.gif" } } </code>
你用的是不是sunlime text編輯程式碼的?可以把你的程式碼用IDLE或pycharm之類的試試,看看能不能執行出結果。
嘗試把input函數換成sys.stdin.readline()
import sys city = sys.stdin.readline()[:-1:] # readline函数的返回值会包括换行符

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
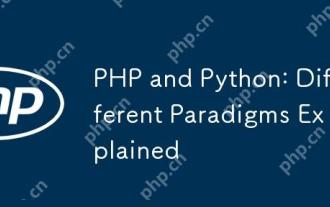
PHP主要是過程式編程,但也支持面向對象編程(OOP);Python支持多種範式,包括OOP、函數式和過程式編程。 PHP適合web開發,Python適用於多種應用,如數據分析和機器學習。
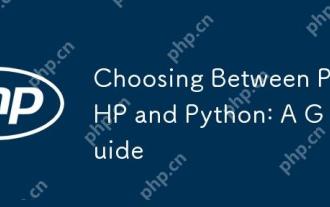
PHP適合網頁開發和快速原型開發,Python適用於數據科學和機器學習。 1.PHP用於動態網頁開發,語法簡單,適合快速開發。 2.Python語法簡潔,適用於多領域,庫生態系統強大。
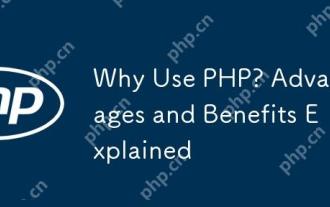
PHP的核心優勢包括易於學習、強大的web開發支持、豐富的庫和框架、高性能和可擴展性、跨平台兼容性以及成本效益高。 1)易於學習和使用,適合初學者;2)與web服務器集成好,支持多種數據庫;3)擁有如Laravel等強大框架;4)通過優化可實現高性能;5)支持多種操作系統;6)開源,降低開發成本。
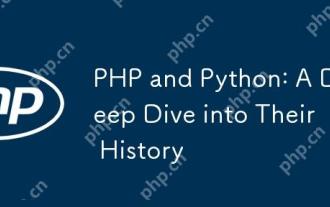
PHP起源於1994年,由RasmusLerdorf開發,最初用於跟踪網站訪問者,逐漸演變為服務器端腳本語言,廣泛應用於網頁開發。 Python由GuidovanRossum於1980年代末開發,1991年首次發布,強調代碼可讀性和簡潔性,適用於科學計算、數據分析等領域。
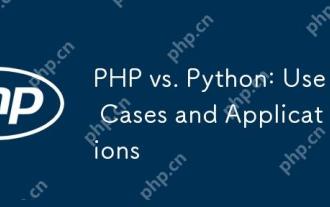
PHP適用於Web開發和內容管理系統,Python適合數據科學、機器學習和自動化腳本。 1.PHP在構建快速、可擴展的網站和應用程序方面表現出色,常用於WordPress等CMS。 2.Python在數據科學和機器學習領域表現卓越,擁有豐富的庫如NumPy和TensorFlow。
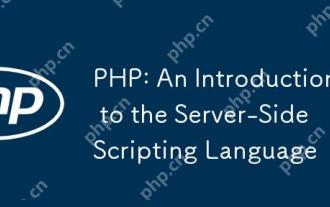
PHP是一種服務器端腳本語言,用於動態網頁開發和服務器端應用程序。 1.PHP是一種解釋型語言,無需編譯,適合快速開發。 2.PHP代碼嵌入HTML中,易於網頁開發。 3.PHP處理服務器端邏輯,生成HTML輸出,支持用戶交互和數據處理。 4.PHP可與數據庫交互,處理表單提交,執行服務器端任務。
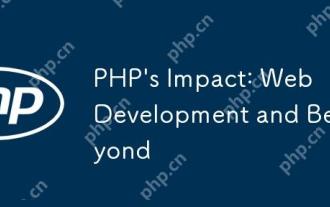
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
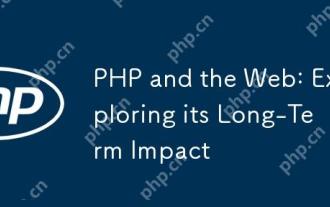
PHP在過去幾十年中塑造了網絡,並將繼續在Web開發中扮演重要角色。 1)PHP起源於1994年,因其易用性和與MySQL的無縫集成成為開發者首選。 2)其核心功能包括生成動態內容和與數據庫的集成,使得網站能夠實時更新和個性化展示。 3)PHP的廣泛應用和生態系統推動了其長期影響,但也面臨版本更新和安全性挑戰。 4)近年來的性能改進,如PHP7的發布,使其能與現代語言競爭。 5)未來,PHP需應對容器化、微服務等新挑戰,但其靈活性和活躍社區使其具備適應能力。
