GUI之 事件處理基礎
事件處理可以簡單地這麼理解,當有一個事件產生,程式要根據這個事件做出回應。例如,我們做了一個可以透過按鈕改變背景顏色的窗口,當我們點擊按鈕時便產生了一個事件,程式會根據這個事件來做出回應,也就是去改變背景的顏色。
那麼程式是怎麼做出回應的呢?這就需要事件監聽器ActionListener,這是一個接口,裡麵包含了actionPerformed方法(也就是根據事件去執行的操作),所以我們要實現這個接口(實現接口裡的actionPerformed方法)做出一個監聽器對像出來,並且用按鈕來註冊這個監聽器對象,這樣當按鈕被點擊的時候,就會調用這個監聽器來執行響應了。
運作結果
碼如果將ColorAction類別獨立出去,需要將buttonPanel傳遞到ColorAction,實作如下:
package buttonPanel; import java.awt.*; import java.awt.event.*; //事件监听器接口ActionListener的位置。 import javax.swing.*; public class ButtonFrame extends JFrame { private ButtonPanel buttonPanel; private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; public ButtonFrame() { setSize(DEFAULT_WIDTH,DEFAULT_HEIGHT); setLocationByPlatform(true); //构造按钮 JButton redButton = new JButton("RED"); JButton yellowButton = new JButton("YELLOW"); JButton blueButton = new JButton("BLUE"); buttonPanel = new ButtonPanel(); //添加按钮到面板 buttonPanel.add(redButton); buttonPanel.add(yellowButton); buttonPanel.add(blueButton); add(buttonPanel); //构造对应颜色的动作监听器 ColorAction redAction = new ColorAction(Color.red); ColorAction yellowAction = new ColorAction(Color.yellow); ColorAction blueAction = new ColorAction(Color.blue); //每个按钮注册对应的监听器 redButton.addActionListener(redAction); yellowButton.addActionListener(yellowAction); blueButton.addActionListener(blueAction); } //为了方便调用buttonPanel,将ColorAction作为ButtonFrame的内部类。 private class ColorAction implements ActionListener { private Color backgroundColor; public ColorAction(Color c) { backgroundColor = c; } public void actionPerformed(ActionEvent event) { buttonPanel.setBackground(backgroundColor); } } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { JFrame frame = new ButtonFrame(); frame.setTitle("ColorButton"); frame.setDefaultCloseOperation(EXIT_ON_CLOSE); frame.setVisible(true); } }); } } class ButtonPanel extends JPanel { private static final int DEFAUT_WIDTH = 300; private static final int DEFAUT_HEIGHT = 200; @Override protected void paintComponent(Graphics g) { g.create(); super.paintComponent(g); } @Override public Dimension getPreferredSize() { return new Dimension(DEFAUT_WIDTH,DEFAUT_HEIGHT); } }
程式碼有一個缺陷,就是在建構按鈕、新增按鈕到面板、建構對應顏色的監聽器和註冊監聽器的時候有程式碼複製的情況,為了避免程式碼複製,我們可以建立一個makeButton方法,把這些重複的操作包含在內,實作如下:
package buttonPanel2; import java.awt.*; import java.awt.event.*; import javax.swing.*; public class ButtonFrame2 extends JFrame { private ButtonPanel buttonPanel; private static final int DEFAULT_WIDTH = 300; private static final int DEFAULT_HEIGHT = 200; public ButtonFrame2() { setSize(DEFAULT_WIDTH,DEFAULT_HEIGHT); setLocationByPlatform(true); JButton redButton = new JButton("RED"); JButton yellowButton = new JButton("YELLOW"); JButton blueButton = new JButton("BLUE"); buttonPanel = new ButtonPanel(); buttonPanel.add(redButton); buttonPanel.add(yellowButton); buttonPanel.add(blueButton); add(buttonPanel); //将此对象通过this传到ColorAction的构造器。 ColorAction redAction = new ColorAction(this,Color.red); ColorAction yellowAction = new ColorAction(this,Color.yellow); ColorAction blueAction = new ColorAction(this,Color.blue); redButton.addActionListener(redAction); yellowButton.addActionListener(yellowAction); blueButton.addActionListener(blueAction); } public void setButtonPanelsBackground(Color backgroundColor) { buttonPanel.setBackground(backgroundColor); } public static void main(String[] args) { EventQueue.invokeLater(new Runnable() { public void run() { JFrame frame = new ButtonFrame2(); frame.setTitle("ColorButton"); frame.setDefaultCloseOperation(EXIT_ON_CLOSE); frame.setVisible(true); } }); } } class ColorAction implements ActionListener { private ButtonFrame2 buttonFrame; private Color backgroundColor; //通过构造器的方法把ButtonFrame2对象传过来,这个对象包含了成员变量buttonPanel,以便对其更换背景色。 public ColorAction(ButtonFrame2 buttonFrame,Color c) { this.buttonFrame = buttonFrame; //this.buttonFrame只是对象管理者,管理的还是ButtonFrame的对象frame。 backgroundColor = c; } public void actionPerformed(ActionEvent event) { buttonFrame.setButtonPanelsBackground(backgroundColor); //这是我们在ButtonFrame2中添加的新方法。 } } class ButtonPanel extends JPanel { private static final int DEFAUT_WIDTH = 300; private static final int DEFAUT_HEIGHT = 200; public ButtonPanel() { setBackground(Color.pink); } @Override protected void paintComponent(Graphics g) { g.create(); super.paintComponent(g); } @Override public Dimension getPreferredSize() { return new Dimension(DEFAUT_WIDTH,DEFAUT_HEIGHT); } } ButtonFrame2
在程式碼中,監聽器只被呼叫了一次,也就是在addActionListener()時。所以我們沒有必要為監聽器單獨做一個類別出來,而是只需在用到監聽器時直接new一個ActionListener接口出來,並在花括號裡實現接口方法即可。

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
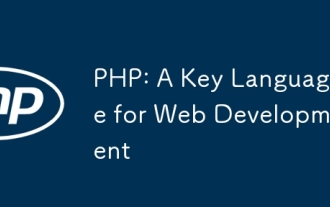
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
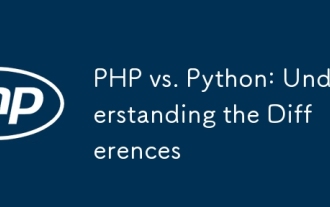
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
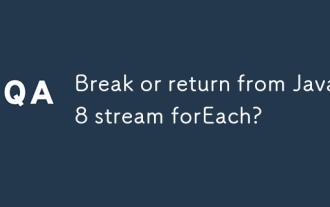
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
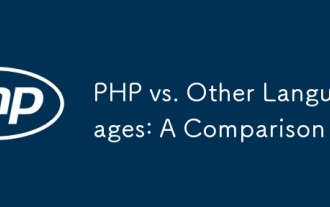
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
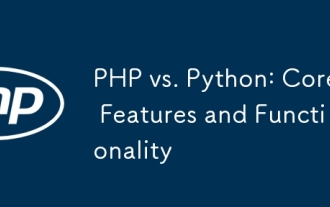
PHP和Python各有優勢,適合不同場景。 1.PHP適用於web開發,提供內置web服務器和豐富函數庫。 2.Python適合數據科學和機器學習,語法簡潔且有強大標準庫。選擇時應根據項目需求決定。
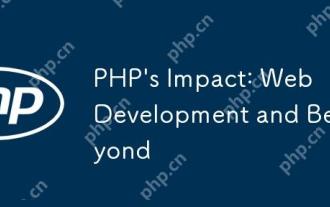
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
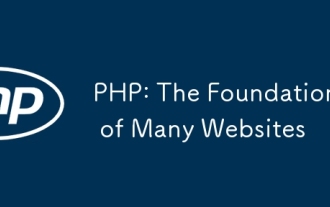
PHP成為許多網站首選技術棧的原因包括其易用性、強大社區支持和廣泛應用。 1)易於學習和使用,適合初學者。 2)擁有龐大的開發者社區,資源豐富。 3)廣泛應用於WordPress、Drupal等平台。 4)與Web服務器緊密集成,簡化開發部署。
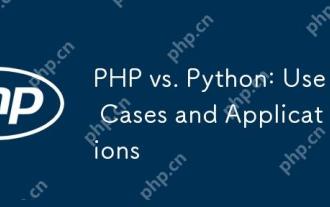
PHP適用於Web開發和內容管理系統,Python適合數據科學、機器學習和自動化腳本。 1.PHP在構建快速、可擴展的網站和應用程序方面表現出色,常用於WordPress等CMS。 2.Python在數據科學和機器學習領域表現卓越,擁有豐富的庫如NumPy和TensorFlow。
