C#基礎知識整理:C#類別與結構(4)
1、什麼是介面? 功能特性? 實現代碼?
介面就是使用interface關鍵字定義的,由類別的成員的組合組成的,描述一些功能的一組規範。在C#中可以看到,系統的一些介面都是這樣命名的:IComparable(類型的比較方法)、ICloneable(支援克隆)、IDisposable(釋放資源)等等,I表示接口,able則反映了介面的特性:“能... ...”,顯示這一組規範能做什麼。
(1)、介面實作
public interface IPrintAble { void PrintString(); void PrintInt(); void PrintBool(); } public interface IComputeAble { void HandlerString(); void HandlerInt(); void HandlerBool(); } public class MyInplementInterface : IPrintAble, IComputeAble { //隐式实现 public void PrintString() { Console.WriteLine(@"1"); } public void PrintInt() { Console.WriteLine(1); } public void PrintBool() { Console.WriteLine(true); } public void HandlerString() { Console.WriteLine(@"1" + "1"); } public void HandlerInt() { Console.WriteLine(1 + 1); } public void HandlerBool() { Console.WriteLine(true || false); } //显示实现 //void IComputeAble.HandlerString() //{ // throw new NotImplementedException(); //} //void IComputeAble.HandlerInt() //{ // throw new NotImplementedException(); //} //void IComputeAble.HandlerBool() //{ // throw new NotImplementedException(); //} } class Program { static void Main(string[] args) { MyInplementInterface imple = new MyInplementInterface(); imple.PrintString(); imple.PrintInt(); imple.PrintBool(); imple.HandlerString(); imple.HandlerInt(); imple.HandlerBool(); Console.ReadLine(); } }
結果:
(2)實現專用接口,即C#已經定義好的接口
例:
public class ImplementSysInterface : IComparable { public int CompareTo(object obj) { //可以根据需要实现自己的比较方法 return 0; } private void UsingMenthod() { //报错,因为NoIDisposeableClass没有实现IDisposable接口,所以不支持using //using (NoIDisposeableClass my = new NoIDisposeableClass()) //{ //} //实现IDisposable接口后,可以使用using using (IDisposeableClass my = new IDisposeableClass()) { } } } public class NoIDisposeableClass { } public class IDisposeableClass : IDisposable { #region IDisposable 成员 public void Dispose() { } #endregion }
接口有以下特性:
a、接口類似於抽象基類,不能直接實例化介面;介面中的方法都是抽象方法,實作介面的任何非抽象型別都必須實作介面的所有成員:
b、當明確實作該介面的成員時,實作的成員不能透過類別實例訪問,只能透過介面實例訪問。
例如:
public class MyInplementInterface2 : IComputeAble { void IComputeAble.HandlerString() { Console.WriteLine(@"1" + "1"); } void IComputeAble.HandlerInt() { Console.WriteLine(true || false); } void IComputeAble.HandlerBool() { Console.WriteLine(true || false); } } class Program { static void Main(string[] args) { IComputeAble imple2 = new MyInplementInterface2(); imple2.HandlerString(); Console.ReadLine(); } }
c、當隱式實現該介面的成員時,實現的成員可以透過類別實例訪問,也可以透過介面實例訪問,但是實現的成員必須是公有的。
d、介面不能包含常數、欄位、運算子、實例建構子、析構函式或型別、不能包含靜態成員。
e、介面成員是自動公開的,且不能包含任何存取修飾符。
f、介面本身可從多個介面繼承,類別和結構可繼承多個接口,但介面不能繼承類別。
2、什麼是泛型? 泛型有哪些優點?
所謂泛型,是將類型參數的概念引入.NET,透過參數化類型來實現在同一份程式碼上操作多種資料型別。是引用型,是堆物件。
其實,一開始學泛型,是在學習java的時候,當時沒有搞明白,我一直都覺得泛型純屬多此一舉,用object一樣可以搞定。例如,如下,比如,有人以類型的值,都要印出來,於是object實現:
public class Test { private object model; public object Model { get { return model; } set { model = value; } } public Test(object model) { this.Model = model; } public void ShowRecord() { Console.WriteLine(model); } } class Program { static void Main(string[] args) { int recordI = 2; bool recordB = true; Test testI = new Test(recordI); testI.ShowRecord(); Test testB = new Test(recordB); testB.ShowRecord(); Console.ReadLine(); } }
但是當學的多了,就會發現還是有一定的問題的。首先,就是裝箱問題,int是值型,賦值給object型別時,要完成一次裝箱操作。什麼是裝箱?就是把recordI值複製到新的object分配的空間。浪費了時間和性能。所以泛型還是有作用的,那麼,用泛型來實現:
public class TestGeneric<T> { private T model; public T Model { get { return model; } set { model = value; } } public TestGeneric(T model) { this.Model = model; } public void ShowRecord() { Console.WriteLine(model); } } class Program { static void Main(string[] args) { int recordI = 2; bool recordB = true; TestGeneric<int> testGI = new TestGeneric<int>(recordI); testGI.ShowRecord(); TestGeneric<bool> testGB = new TestGeneric<bool>(recordB); testGB.ShowRecord(); Console.ReadLine(); } }
這樣,當TestGeneric
當然泛型不只是解決裝箱問題,功能特性如下:
a、避免裝箱拆箱,提升了效能;
b、提升了程式碼的重複使用;
c、型別安全的,因為在編譯的時候會偵測;
d、可以建立自己的泛型介面、泛型類別、泛型方法、泛型事件和泛型委託。
以上就是C#基礎知識整理:C#類別與結構(4)的內容,更多相關內容請關注PHP中文網(www.php.cn)!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
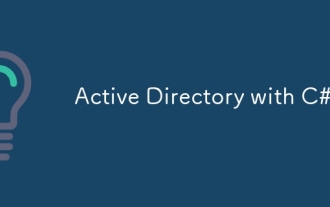
使用 C# 的 Active Directory 指南。在這裡,我們討論 Active Directory 在 C# 中的介紹和工作原理以及語法和範例。
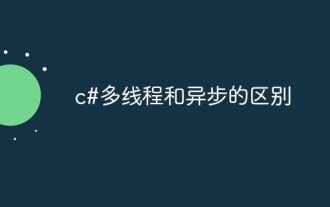
多線程和異步的區別在於,多線程同時執行多個線程,而異步在不阻塞當前線程的情況下執行操作。多線程用於計算密集型任務,而異步用於用戶交互操作。多線程的優勢是提高計算性能,異步的優勢是不阻塞 UI 線程。選擇多線程還是異步取決於任務性質:計算密集型任務使用多線程,與外部資源交互且需要保持 UI 響應的任務使用異步。

可以採用多種方法修改 XML 格式:使用文本編輯器(如 Notepad )進行手工編輯;使用在線或桌面 XML 格式化工具(如 XMLbeautifier)進行自動格式化;使用 XML 轉換工具(如 XSLT)定義轉換規則;或者使用編程語言(如 Python)進行解析和操作。修改時需謹慎,並備份原始文件。
