詳解python中Threadpool執行緒池任務終止範例程式碼
需求
加入我們需要處理一串個位數(0~9),奇數時需要循環列印它;偶數則等待對應時長並完成所有任務;0則是錯誤,但不需要終止任務,可以自訂一些處理。
關鍵點
定義func函數處理需求
callback處理回傳結果,只有偶數和0回傳;奇數會一直執行;要控制執行緒池狀態,則需要針對偶數和0時拋出異常,並捕獲異常處理。
threadpool定義執行緒池並發
實作
# -*- coding: utf-8 -*- from threadpool import makeRequests, ThreadPool import time from multiprocessing import Process
例外定義與特殊值(0)定義
class Finish(SyntaxWarning): pass
class PauseInfo(SyntaxWarning): pass pause_num = 0
func函數定義
# 0時傳回False,其他偶數傳回True
def func(para): if para == pause_num: print('start for %d and wait %ds' % (para, 4)) time.sleep(4) print('error bcs ',para) return False if para % 2 == 0: print('start for %d and wait %ds' % (para, para)) time.sleep(para) print('stop for', para) return True while True: print('continue for', para) time.sleep(para)
callback定義
def callback(request, result): if result: raise Finish else: raise PauseInfo
執行緒池處理
Finish標識任務完成,再次誘發異常退出執行緒池處理;
def main_thread(paras): pool = ThreadPool(10) requests = makeRequests(callable_=func, args_list=paras, callback=callback) [pool.putRequest(req) for req in requests] while True: try: pool.wait() except Finish as e: raise SystemExit except PauseInfo as e: print('Pause bcs %d but will continue' % pause_num) except Exception as e: print('Unknown error so will quit') raise SystemExit
主函數起一個測試進程
if name == 'main': while True: s = input('Input number list to test and any other word to quit\n') paras = [] for para in s: if para.isnumeric(): paras.append(int(para)) else: break try: thread_test = Process(target=main_thread, args=(paras,)) thread_test.start() thread_test.join(timeout=20) except TimeoutError as e: print('task timeout') except Exception as e: print('unknow error:',e)
結果驗證
#處理108,看列印可以看到,1被循環處理,0處理的時候報錯;8處理完畢則任務結束
Input number list to test and any other word to quit
108
continue for 1
start for 0 and wait 4s
start for 8 and wait 8s
continue for 1
continue for 1
continue for 1
error bcs 0
continue for 1
Pause bcs 0 will continue
continue for 1
continue for 1
continue for 1
continue for 1
stop for 8
Input number list to test and any other word to quit
以上是詳解python中Threadpool執行緒池任務終止範例程式碼的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
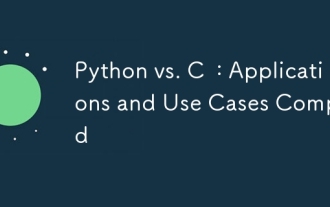
Python适合数据科学、Web开发和自动化任务,而C 适用于系统编程、游戏开发和嵌入式系统。Python以简洁和强大的生态系统著称,C 则以高性能和底层控制能力闻名。
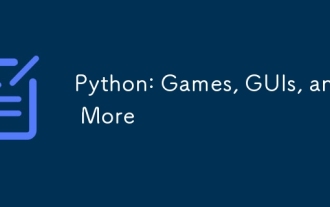
Python在遊戲和GUI開發中表現出色。 1)遊戲開發使用Pygame,提供繪圖、音頻等功能,適合創建2D遊戲。 2)GUI開發可選擇Tkinter或PyQt,Tkinter簡單易用,PyQt功能豐富,適合專業開發。
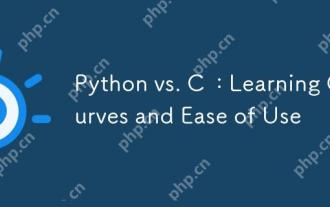
Python更易學且易用,C 則更強大但複雜。 1.Python語法簡潔,適合初學者,動態類型和自動內存管理使其易用,但可能導致運行時錯誤。 2.C 提供低級控制和高級特性,適合高性能應用,但學習門檻高,需手動管理內存和類型安全。
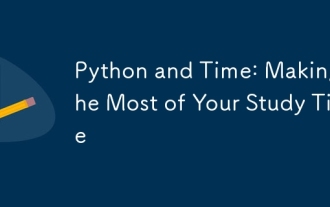
要在有限的時間內最大化學習Python的效率,可以使用Python的datetime、time和schedule模塊。 1.datetime模塊用於記錄和規劃學習時間。 2.time模塊幫助設置學習和休息時間。 3.schedule模塊自動化安排每週學習任務。
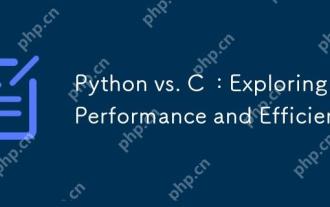
Python在開發效率上優於C ,但C 在執行性能上更高。 1.Python的簡潔語法和豐富庫提高開發效率。 2.C 的編譯型特性和硬件控制提升執行性能。選擇時需根據項目需求權衡開發速度與執行效率。
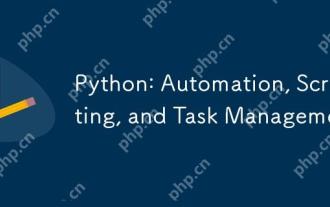
Python在自動化、腳本編寫和任務管理中表現出色。 1)自動化:通過標準庫如os、shutil實現文件備份。 2)腳本編寫:使用psutil庫監控系統資源。 3)任務管理:利用schedule庫調度任務。 Python的易用性和豐富庫支持使其在這些領域中成為首選工具。
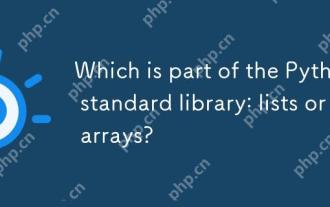
pythonlistsarepartofthestAndArdLibrary,herilearRaysarenot.listsarebuilt-In,多功能,和Rused ForStoringCollections,而EasaraySaraySaraySaraysaraySaraySaraysaraySaraysarrayModuleandleandleandlesscommonlyusedDduetolimitedFunctionalityFunctionalityFunctionality。
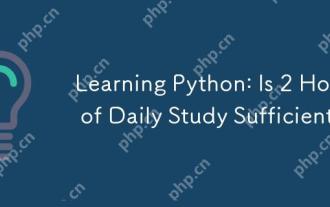
每天學習Python兩個小時是否足夠?這取決於你的目標和學習方法。 1)制定清晰的學習計劃,2)選擇合適的學習資源和方法,3)動手實踐和復習鞏固,可以在這段時間內逐步掌握Python的基本知識和高級功能。
