詳解Java Annotation特性的掌握
什麼是Annotation?
Annotation翻譯為中文即為註解,意思就是提供除了程式本身邏輯外的額外的資料資訊。 Annotation對於標註的程式碼沒有直接的影響,它不可以直接與標註的程式碼產生交互,但其他元件可以使用這些資訊。
Annotation資訊可以被編譯進class文件,也可以保留在Java 虛擬機器中,以便在執行時可以取得。甚至對於Annotation本身也可以加Annotation。
那些物件可以加Annotation
類,方法,變數,參數,套件都可以加Annotation。
內建的Annotation
@Override 重載父類別中方法@Deprecated 被標註的方法或型別已不再推薦使用
@SuppressWarnings阻止編譯時的警告訊息。其需要接收一個String的陣列作為參數。 可供使用的參數有:
unchecked
#path
- ##serial
- finally
- fallthrough
#@Retention
確定Annotation被儲存的生命週期, 需要接收一個Enum物件RetentionPolicy作為參數。
public enum RetentionPolicy { /** * Annotations are to be discarded by the compiler. */ SOURCE, /** * Annotations are to be recorded in the class file by the compiler * but need not be retained by the VM at run time. This is the default * behavior. */ CLASS, /** * Annotations are to be recorded in the class file by the compiler and * retained by the VM at run time, so they may be read reflectively. * * @see java.lang.reflect.AnnotatedElement */ RUNTIME }
@Documented 文檔化
#@Target
表示該Annotation可以修飾的範圍,接收一個Enum物件EnumType的數組作為參數。public enum ElementType { /** Class, interface (including annotation type), or enum declaration */ TYPE, /** Field declaration (includes enum constants) */ FIELD, /** Method declaration */ METHOD, /** Parameter declaration */ PARAMETER, /** Constructor declaration */ CONSTRUCTOR, /** Local variable declaration */ LOCAL_VARIABLE, /** Annotation type declaration */ ANNOTATION_TYPE, /** Package declaration */ PACKAGE }
@Inherited
該Annotation可以影響到被標註的類別的子類別。 自訂AnnotationJSE5.0以後我們可以自訂Annotation。下面就是一個簡單的例子。@Retention(RetentionPolicy.RUNTIME) @Target(ElementType.METHOD) public @interface MethodAnnotation { }
public class Person { public void eat() { System.out.println("eating"); } @MethodAnnotation public void walk() { System.out.print("walking"); } }
Class<Person> personClass = Person.class; Method[] methods = personClass.getMethods(); for(Method method : methods){ if (method.isAnnotationPresent(MethodAnnotation.class)){ method.invoke(personClass.newInstance()); } }
walking
@Target(ElementType.TYPE) public @interface personAnnotation { int id() default 1; String name() default "bowen"; }
@personAnnotation(id = 8, name = "john") public class Person { public void eat() { System.out.println("eating"); } @MethodAnnotation public void walk() { System.out.print("walking"); } }
介面,Java的反射物件類別Class,Constructor,Field,Method以及Package都實作了這個介面。這個介面用來表示目前在Java虛擬機器中運作的被加上了annotation的程式元素。透過這個介面可以使用反射讀取annotation。 AnnotatedElement介面可以存取被加上RUNTIME標記的annotation,對應的方法有getAnnotation,getAnnotations,isAnnotationPresent。由於Annotation類型被編譯和儲存在二進位檔案中就像class一樣,所以可以像查詢普通的Java物件一樣查詢這些方法傳回的Annotation。
Annotation的廣泛使用Annotation被廣泛用於各種JunitJunit是非常著名的單元測試框架,使用Junit的時候需要接觸大量的annotation。
- @Runwith 自訂測試類別的Runner
- #@ContextConfiguration 設定Spring的ApplicationContext
- # @DirtiesContext 當執行下一個測試前重新載入ApplicationContext.
- #@Before 呼叫測試方法前初始化 ##@After 呼叫測試方法後處理
- @Test 表示方法是測試方法
- #@Ignore 可以加在測試類別或測試方法上,忽略運行。
- @BeforeClass:在該測試類別中的所有測試方法執行前調用,只被調用一次(被標註的方法必須是
- static
@AfterClass:在該測試類別中的所有的測試方法執行完後調用,只被執行一次(被標註的方法必須是static)
Spring
Spring 號稱配置地獄,Annotation也不少。
@Service 給service類別加註解
#@Repository 給DAO類別加註解
# @Component 給元件類別加註解
@Autowired 讓Spring自動組裝bean
@Transactional 設定事物
@Scope 設定物件存活範圍
@Controller 為控制器類別加註解
@RequestMapping url路徑對應
- ##@PathVariable 將方法參數對應到路徑
- #@RequestParam 將請求參數綁定到方法變數
- @ModelAttribute 與model綁定
- Hibernate
- ## @Entity 修飾entity bean
- @Table 將entity類別與資料庫中的table映射起來
- @OrderBy 排序規則
- #@Lob 大物件標註
- Hibernate還有大量的關於聯合的annotation和 繼承
- 的annotation,這裡就不意義列舉了。 JSR 303 – Bean Validation
- JSR 303 – Bean Validation是一個資料驗證的規範,其對Java bean的驗證主要透過Java annotation來實現。
- @
Null
被 註解 - 的元素必須為null
- @NotNull被註解的元素必須不為null
- @AssertTrue被註解的元素必須為true@AssertFalse被註解的元素必須為false@Min(value)被註解的元素必須是一個數字,其值必須大於等於指定的最小值
- @Max(value)被註解的元素必須是一個數字,其值必須小於等於指定的最大值
- #@DecimalMin(value)被註解的元素必須是一個數字,其值必須大於等於指定的最小值@DecimalMax(value)被註解的元素必須是一個數字,其值必須小於等於指定的最大值
以上是詳解Java Annotation特性的掌握的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
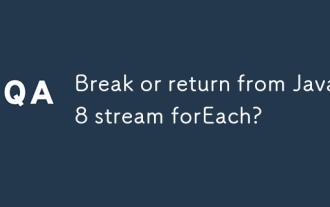
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
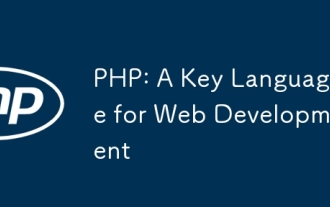
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
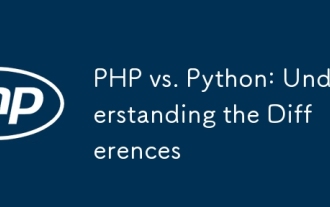
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
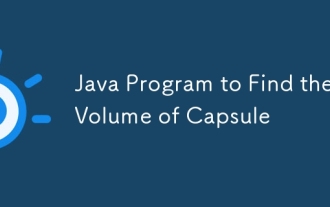
膠囊是一種三維幾何圖形,由一個圓柱體和兩端各一個半球體組成。膠囊的體積可以通過將圓柱體的體積和兩端半球體的體積相加來計算。本教程將討論如何使用不同的方法在Java中計算給定膠囊的體積。 膠囊體積公式 膠囊體積的公式如下: 膠囊體積 = 圓柱體體積 兩個半球體體積 其中, r: 半球體的半徑。 h: 圓柱體的高度(不包括半球體)。 例子 1 輸入 半徑 = 5 單位 高度 = 10 單位 輸出 體積 = 1570.8 立方單位 解釋 使用公式計算體積: 體積 = π × r2 × h (4
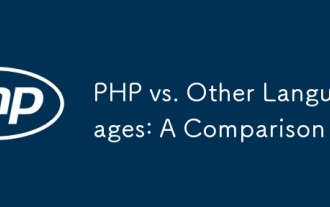
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
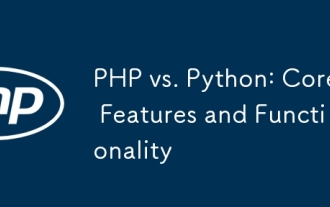
PHP和Python各有優勢,適合不同場景。 1.PHP適用於web開發,提供內置web服務器和豐富函數庫。 2.Python適合數據科學和機器學習,語法簡潔且有強大標準庫。選擇時應根據項目需求決定。
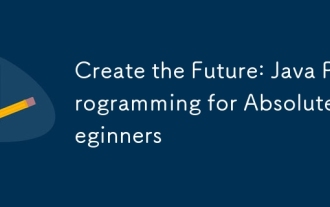
Java是熱門程式語言,適合初學者和經驗豐富的開發者學習。本教學從基礎概念出發,逐步深入解說進階主題。安裝Java開發工具包後,可透過建立簡單的「Hello,World!」程式來實踐程式設計。理解程式碼後,使用命令提示字元編譯並執行程序,控制台上將輸出「Hello,World!」。學習Java開啟了程式設計之旅,隨著掌握程度加深,可創建更複雜的應用程式。
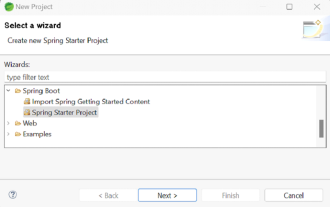
Spring Boot簡化了可靠,可擴展和生產就緒的Java應用的創建,從而徹底改變了Java開發。 它的“慣例慣例”方法(春季生態系統固有的慣例),最小化手動設置
