關於React 實作專案的範例詳解 (三)
React在Github上已經有接近70000的 star 數了,是目前最熱門的前端框架。而我學習React也有一段時間了,現在就開始用 React+Redux 來實戰吧!
上回說到使用Redux進行狀態管理,這次我們使用Redux-saga 管理Redux 應用非同步操作
React 實踐專案(一)
#React實作專案(二)
React 實作專案(三)
- 首先我們來看看登陸的Reducer
export const auth = (state = initialState, action = {}) => { switch (action.type) { case LOGIN_USER: return state.merge({ 'user': action.data, 'error': null, 'token': null, }); case LOGIN_USER_SUCCESS: return state.merge({ 'token': action.data, 'error': null }); case LOGIN_USER_FAILURE: return state.merge({ 'token': null, 'error': action.data }); default: return state } };
Sagas 監聽發起的action,然後決定基於這個action 來做什麼:是發起一個非同步呼叫(例如一個Ajax 請求),還是發起其他的action 到Store,甚至是呼叫其他的Sagas。
具體到這個登陸功能就是我們在登陸彈窗點擊登陸時會發出一個 LOGIN_USER
action,Sagas 監聽到 LOGIN_USER
# action,發起一個Ajax 要求到後台,根據結果決定發起 LOGIN_USER_SUCCESS
action 或LOGIN_USER_FAILURE
action
- 建立Saga middleware 連接至Redux store
redux-saga 依賴
修改
<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>/**
* Created by Yuicon on 2017/6/27.
*/
import {createStore, applyMiddleware } from &#39;redux&#39;;
import createSagaMiddleware from &#39;redux-saga&#39;
import reducer from &#39;../reducer/reducer&#39;;
import rootSaga from &#39;../sagas/sagas&#39;;
const sagaMiddleware = createSagaMiddleware();
const store = createStore(
reducer,
applyMiddleware(sagaMiddleware)
);
sagaMiddleware.run(rootSaga);
export default store;</pre><div class="contentsignin">登入後複製</div></div>
Redux-saga 使用 Generator 函數實作
- #監聽action
- 建立src/redux/sagas/sagas.js
/** * Created by Yuicon on 2017/6/28. */ import { takeLatest } from 'redux-saga/effects'; import {registerUserAsync, loginUserAsync} from './users'; import {REGISTER_USER, LOGIN_USER} from '../action/users'; export default function* rootSaga() { yield [ takeLatest(REGISTER_USER, registerUserAsync), takeLatest(LOGIN_USER, loginUserAsync) ]; }
我們可以看到在rootSaga 中監聽了兩個action 登陸和註冊。
在上面的範例中,takeLatest 只允許執行一個 loginUserAsync 任務。而這個任務是最後啟動的那個。 如果之前已經有一個任務在執行,那麼之前的這個任務會自動被取消。
如果我們允許多個 loginUserAsync 實例同時啟動。在某個特定時刻,我們可以啟動一個新 loginUserAsync 任務, 儘管之前還有一個或多個 loginUserAsync 尚未結束。我們可以使用 takeEvery 輔助函數。
- 發起一個Ajax 請求
- # 取得Store state 上的資料
- ##selectors.js
/** * Created by Yuicon on 2017/6/28. */ export const getAuth = state => state.auth;
- api.js
/** * Created by Yuicon on 2017/7/4. * */ /** * 这是我自己的后台服务器,用 Java 实现 * 项目地址: * 文档:http://139.224.135.86:8080/swagger-ui.html#/ */ const getURL = (url) => `http://139.224.135.86:8080/${url}`; export const login = (user) => { return fetch(getURL("auth/login"), { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(user) }).then(response => response.json()) .then(json => { return json; }) .catch(ex => console.log('parsing failed', ex)); };
- select(selector, ...args) 用於取得Store state 上的資料
/** * Created by Yuicon on 2017/6/30. */ import {select, put, call} from 'redux-saga/effects'; import {getAuth, getUsers} from './selectors'; import {loginSuccessAction, loginFailureAction, registerSuccessAction, registerFailureAction} from '../action/users'; import {login, register} from './api'; import 'whatwg-fetch'; export function* loginUserAsync() { // 获取Store state 上的数据 const auth = yield select(getAuth); const user = auth.get('user'); // 发起 ajax 请求 const json = yield call(login.bind(this, user), 'login'); if (json.success) { localStorage.setItem('token', json.data); // 发起 loginSuccessAction yield put(loginSuccessAction(json.data)); } else { // 发起 loginFailureAction yield put(loginFailureAction(json.error)); } }
登入後複製
結語put(action)
發起一個action 到Storecall(fn, ...args)
呼叫fn 函數並以args 為參數,如果結果是一個Promise,middleware 會暫停直到這個Promise 被resolve,resolve 後Generator會繼續執行。 或者直到 Promise 被 reject 了,如果是這種情況,將在 Generator 中拋出錯誤。Redux-saga 詳細api文檔
- 我在工作時用的是Redux-Thunk,Redux- Thunk 相對來說更容易實現和維護。但對於複雜的操作,尤其是面對複雜非同步操作時,Redux-saga 更有優勢。到此我們完成了一個 Redux-saga 的入門教程,Redux-saga 還有很多奇妙的地方,
以上是關於React 實作專案的範例詳解 (三)的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
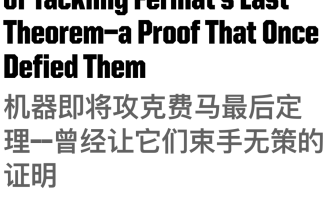
費馬大定理,即將被AI攻克?而整件事最有意義的地方在於,AI即將解決的費馬大定理,正是為了證明AI無用。曾經,數學屬於純粹的人類智力王國;如今,這片疆土正被先進的演算法所破解,所踐踏。圖片費馬大定理,是一個「臭名昭著」的謎題,在幾個世紀以來,一直困擾著數學家。它在1993年被證明,而現在,數學家們有一個偉大計畫:用電腦把證明過程重現。他們希望在這個版本的證明中,如果有任何邏輯上的錯誤,都可以由電腦檢查出來。專案網址:https://github.com/riccardobrasca/flt
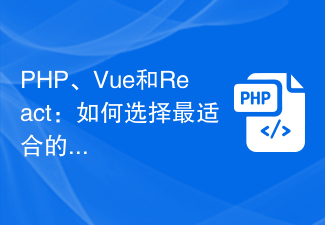
PHP、Vue和React:如何選擇最適合的前端框架?隨著互聯網技術的不斷發展,前端框架在Web開發中起著至關重要的作用。 PHP、Vue和React作為三種代表性的前端框架,每一種都具有其獨特的特徵和優勢。在選擇使用哪種前端框架時,開發人員需要根據專案需求、團隊技能和個人偏好做出明智的決策。本文將透過比較PHP、Vue和React這三種前端框架的特徵和使
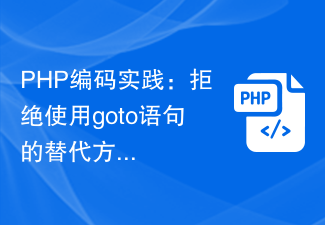
PHP編碼實踐:拒絕使用goto語句的替代方案近年來,隨著程式語言的不斷更新和迭代,程式設計師開始更加重視編碼規範和最佳實踐。在PHP程式設計中,goto語句作為一種控制流語句存在已久,但在實際應用中往往會導致程式碼的可讀性和可維護性下降。本文將分享一些替代方案,幫助開發人員拒絕使用goto語句,提升程式碼品質。一、為什麼拒絕使用goto語句?首先,讓我們來思考一下為
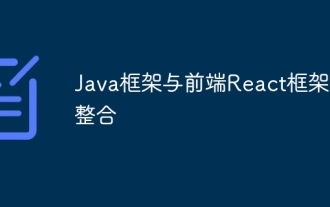
Java框架與React框架的整合:步驟:設定後端Java框架。建立專案結構。配置建置工具。建立React應用程式。編寫RESTAPI端點。配置通訊機制。實戰案例(SpringBoot+React):Java程式碼:定義RESTfulAPI控制器。 React程式碼:取得並顯示API回傳的資料。
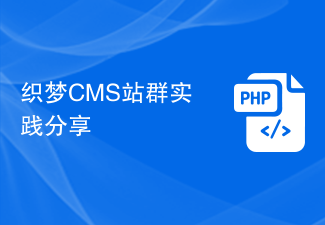
織夢CMS站群實務分享近年來,隨著網路的快速發展,網站建置變得越來越重要。在建立多個網站時,站群技術成為了一個非常有效的方法。而在眾多網站建立工具中,織夢CMS憑藉其靈活性和易用性成為了不少站群愛好者的首選。本文將分享一些關於織夢CMS站群的實務經驗,以及一些具體的程式碼範例,希望能為正在探索站群技術的讀者提供一些幫助。 1.什麼是織夢CMS站群?織夢CMS

Vue.js適合中小型項目和快速迭代,React適用於大型複雜應用。 1)Vue.js易於上手,適用於團隊經驗不足或項目規模較小的情況。 2)React的生態系統更豐富,適合有高性能需求和復雜功能需求的項目。

React通過JSX與HTML結合,提升用戶體驗。 1)JSX嵌入HTML,使開發更直觀。 2)虛擬DOM機制優化性能,減少DOM操作。 3)組件化管理UI,提高可維護性。 4)狀態管理和事件處理增強交互性。

NetflixusesAcustomFrameworkcalled“ Gibbon” BuiltonReact,notReactorVuedIrectly.1)TeamSperience:selectBasedonFamiliarity.2)ProjectComplexity:vueforsimplerprojects:reactforforforproproject,reactforforforcompleplexones.3)cocatizationneedneeds:reactoffipicatizationneedneedneedneedneedneeds:reactoffersizationneedneedneedneedneeds:reactoffersizatization needefersmoreflexibleise.4)
