javascript改變函數體內部指向的apply與call用法實例詳解
call 和apply 都是為了改變某個函數執行時間的context 即上下文而存在的,換句話說,就是為了改變函數體內部this 的指向。
call 和 apply二者的作用完全一樣,只是接受參數的方式不太一樣。
方法定義
apply
Function.apply(obj,args)方法能接收兩個參數:
obj:這個物件將取代Function類別裡this物件
#args:這個是數組或類別數組,apply方法把這個集合中的元素作為參數傳遞給被呼叫的函數。
call
call方法與apply方法的第一個參數是一樣的,只不過第二個參數是一個參數列表
在非嚴格模式下當我們第一個參數傳遞為null或undefined時,函數體內的this會指向預設的宿主對象,在瀏覽器中則是window
var test = function(){ console.log(this===window); } test.apply(null);//true test.call(undefined);//true
用法
"劫持"別人的方法
此時foo中的logName方法將被bar引用,this指向了bar
var foo = { name:"mingming", logName:function(){ console.log(this.name); } } var bar={ name:"xiaowang" }; foo.logName.call(bar);//xiaowang
實作繼承
function Animal(name){ this.name = name; this.showName = function(){ console.log(this.name); } } function Cat(name){ Animal.call(this, name); } var cat = new Cat("Black Cat"); cat.showName(); //Black Cat
在實際開發中,常常會遇到this指向被不經意改變的場景。
有一個局部的fun方法,fun被呼叫為普通函數時,fun內部的this#指向了window,但我們往往是想讓它指向該#test節點,請參見如下程式碼:
window.id="window"; document.querySelector('#test').onclick = function(){ console.log(this.id);//test var fun = function(){ console.log(this.id); } fun();//window }
使用call ,apply我們就可以輕鬆的解決這種問題了
window.id="window"; document.querySelector('#test').onclick = function(){ console.log(this.id);//test var fun = function(){ console.log(this.id); } fun.call(this);//test }
當然你也可以這樣做,不過在ECMAScript 5的strict模式下,這種情況下的this已經被規定為不會指向全域對象,而是undefined:
window.id="window"; document.querySelector('#test').onclick = function(){ var that = this; console.log(this.id);//test var fun = function(){ console.log(that.id); } fun();//test }
function func(){ "use strict" alert ( this ); // 输出:undefined } func();
以上是javascript改變函數體內部指向的apply與call用法實例詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
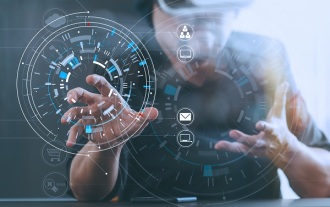
人臉偵測辨識技術已經是一個比較成熟且應用廣泛的技術。而目前最廣泛的網路應用語言非JS莫屬,在Web前端實現人臉偵測辨識相比後端的人臉辨識有優勢也有弱勢。優點包括減少網路互動、即時識別,大大縮短了使用者等待時間,提高了使用者體驗;弱勢是:受到模型大小限制,其中準確率也有限。如何在web端使用js實現人臉偵測呢?為了實現Web端人臉識別,需要熟悉相關的程式語言和技術,如JavaScript、HTML、CSS、WebRTC等。同時也需要掌握相關的電腦視覺和人工智慧技術。值得注意的是,由於Web端的計
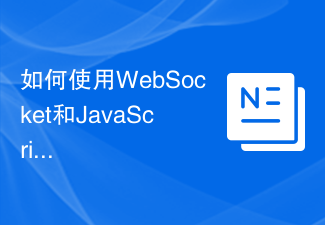
如何使用WebSocket和JavaScript實現線上語音辨識系統引言:隨著科技的不斷發展,語音辨識技術已成為了人工智慧領域的重要組成部分。而基於WebSocket和JavaScript實現的線上語音辨識系統,具備了低延遲、即時性和跨平台的特點,成為了廣泛應用的解決方案。本文將介紹如何使用WebSocket和JavaScript來實現線上語音辨識系
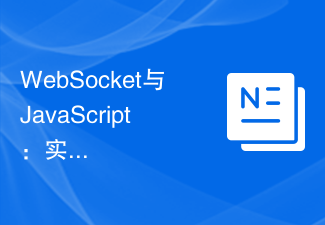
WebSocket與JavaScript:實現即時監控系統的關鍵技術引言:隨著互聯網技術的快速發展,即時監控系統在各個領域中得到了廣泛的應用。而實現即時監控的關鍵技術之一就是WebSocket與JavaScript的結合使用。本文將介紹WebSocket與JavaScript在即時監控系統中的應用,並給出程式碼範例,詳細解釋其實作原理。一、WebSocket技
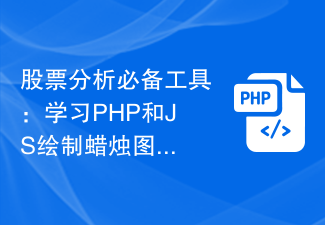
股票分析必備工具:學習PHP和JS繪製蠟燭圖的步驟,需要具體程式碼範例隨著網路和科技的快速發展,股票交易已成為許多投資者的重要途徑之一。而股票分析是投資人決策的重要一環,其中蠟燭圖被廣泛應用於技術分析。學習如何使用PHP和JS繪製蠟燭圖將為投資者提供更多直觀的信息,幫助他們更好地做出決策。蠟燭圖是一種以蠟燭形狀來展示股票價格的技術圖表。它展示了股票價格的
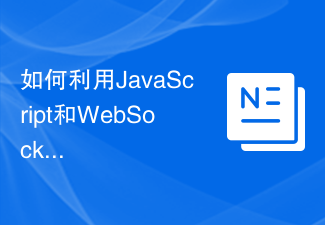
如何利用JavaScript和WebSocket實現即時線上點餐系統介紹:隨著網路的普及和技術的進步,越來越多的餐廳開始提供線上點餐服務。為了實現即時線上點餐系統,我們可以利用JavaScript和WebSocket技術。 WebSocket是一種基於TCP協定的全雙工通訊協議,可實現客戶端與伺服器的即時雙向通訊。在即時線上點餐系統中,當使用者選擇菜餚並下訂單
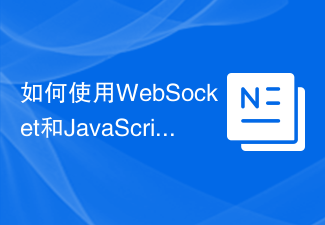
如何使用WebSocket和JavaScript實現線上預約系統在當今數位化的時代,越來越多的業務和服務都需要提供線上預約功能。而實現一個高效、即時的線上預約系統是至關重要的。本文將介紹如何使用WebSocket和JavaScript來實作一個線上預約系統,並提供具體的程式碼範例。一、什麼是WebSocketWebSocket是一種在單一TCP連線上進行全雙工
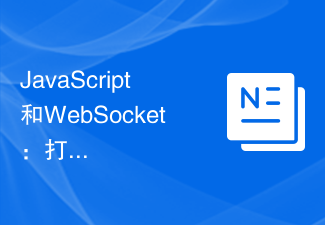
JavaScript和WebSocket:打造高效的即時天氣預報系統引言:如今,天氣預報的準確性對於日常生活以及決策制定具有重要意義。隨著技術的發展,我們可以透過即時獲取天氣數據來提供更準確可靠的天氣預報。在本文中,我們將學習如何使用JavaScript和WebSocket技術,來建立一個高效的即時天氣預報系統。本文將透過具體的程式碼範例來展示實現的過程。 We
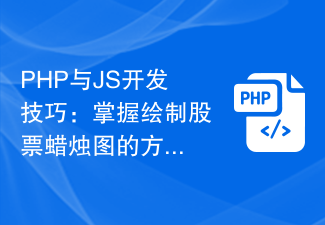
隨著網路金融的快速發展,股票投資已經成為了越來越多人的選擇。而在股票交易中,蠟燭圖是常用的技術分析方法,它能夠顯示股票價格的變動趨勢,幫助投資人做出更精準的決策。本文將透過介紹PHP和JS的開發技巧,帶領讀者了解如何繪製股票蠟燭圖,並提供具體的程式碼範例。一、了解股票蠟燭圖在介紹如何繪製股票蠟燭圖之前,我們首先需要先了解什麼是蠟燭圖。蠟燭圖是由日本人
