實例詳解簡單實體類別和xml檔案的相互轉換方法
本文主要為大家帶來一篇簡單實體類別和xml檔案的相互轉換方法。小編覺得蠻不錯的,現在就分享給大家,也給大家做個參考。一起跟著小編過來看看吧,希望能幫助大家。大概思路是這樣的,只要能拿到實體類別的類型信息,我就能拿到實體類別的全部字段名稱和類型,拼屬性的set和get方法更是簡單明了,這時候只需要通過方法的反射,將xml檔的資料讀取出來給這個反射即可。反過來只要給我一個任意對象,我就能透過反射拿到該對象所有欄位的值,這時候在寫xml檔即可。
具體程式碼如下:
package com.pcq.entity; import java.io.*; import java.lang.reflect.Field; import java.lang.reflect.InvocationTargetException; import java.lang.reflect.Method; import java.util.ArrayList; import java.util.Iterator; import java.util.List; import org.dom4j.Document; import org.dom4j.DocumentException; import org.dom4j.DocumentHelper; import org.dom4j.Element; import org.dom4j.io.OutputFormat; import org.dom4j.io.SAXReader; import org.dom4j.io.XMLWriter; public class XMLAndEntityUtil { private static Document document = DocumentHelper.createDocument(); /** * 判断是否是个xml文件,目前类里尚未使用该方法 * @param filePath * @return */ @SuppressWarnings("unused") private static boolean isXMLFile(String filePath) { File file = new File(filePath); if(!file.exists() || filePath.indexOf(".xml") > -1) { return false; } return true; } /** * 将一组对象数据转换成XML文件 * @param list * @param filePath 存放的文件路径 */ public static <T> void writeXML(List<T> list, String filePath) { Class<?> c = list.get(0).getClass(); String root = c.getSimpleName().toLowerCase() + "s"; Element rootEle = document.addElement(root); for(Object obj : list) { try { Element e = writeXml(rootEle, obj); document.setRootElement(e); writeXml(document, filePath); } catch (NoSuchMethodException | SecurityException | IllegalAccessException | IllegalArgumentException | InvocationTargetException e) { e.printStackTrace(); } } } /** * 通过一个根节点来写对象的xml节点,这个方法不对外开放,主要给writeXML(List<T> list, String filePath)提供服务 * @param root * @param object * @return * @throws NoSuchMethodException * @throws SecurityException * @throws IllegalAccessException * @throws IllegalArgumentException * @throws InvocationTargetException */ private static Element writeXml(Element root, Object object) throws NoSuchMethodException, SecurityException, IllegalAccessException, IllegalArgumentException, InvocationTargetException { Class<?> c = object.getClass(); String className = c.getSimpleName().toLowerCase(); Element ele = root.addElement(className); Field[] fields = c.getDeclaredFields(); for(Field f : fields) { String fieldName = f.getName(); String param = fieldName.substring(0, 1).toUpperCase() + fieldName.substring(1); Element fieldElement = ele.addElement(fieldName); Method m = c.getMethod("get" + param, null); String s = ""; if(m.invoke(object, null) != null) { s = m.invoke(object, null).toString(); } fieldElement.setText(s); } return root; } /** * 默认使用utf-8 * @param c * @param filePath * @return * @throws UnsupportedEncodingException * @throws FileNotFoundException */ public static <T> List<T> getEntitys(Class<T> c, String filePath) throws UnsupportedEncodingException, FileNotFoundException { return getEntitys(c, filePath, "utf-8"); } /** * 将一个xml文件转变成实体类 * @param c * @param filePath * @return * @throws FileNotFoundException * @throws UnsupportedEncodingException */ public static <T> List<T> getEntitys(Class<T> c, String filePath, String encoding) throws UnsupportedEncodingException, FileNotFoundException { File file = new File(filePath); String labelName = c.getSimpleName().toLowerCase(); SAXReader reader = new SAXReader(); List<T> list = null; try { InputStreamReader in = new InputStreamReader(new FileInputStream(file), encoding); Document document = reader.read(in); Element root = document.getRootElement(); List elements = root.elements(labelName); list = new ArrayList<T>(); for(Iterator<Emp> it = elements.iterator(); it.hasNext();) { Element e = (Element)it.next(); T t = getEntity(c, e); list.add(t); } } catch (DocumentException e) { e.printStackTrace(); } catch (InstantiationException e1) { e1.printStackTrace(); } catch (IllegalAccessException e1) { e1.printStackTrace(); } catch (NoSuchMethodException e1) { e1.printStackTrace(); } catch (SecurityException e1) { e1.printStackTrace(); } catch (IllegalArgumentException e1) { e1.printStackTrace(); } catch (InvocationTargetException e1) { e1.printStackTrace(); } return list; } /** * 将一种类型 和对应的 xml元素节点传进来,返回该类型的对象,该方法不对外开放 * @param c 类类型 * @param ele 元素节点 * @return 该类型的对象 * @throws InstantiationException * @throws IllegalAccessException * @throws NoSuchMethodException * @throws SecurityException * @throws IllegalArgumentException * @throws InvocationTargetException */ @SuppressWarnings("unchecked") private static <T> T getEntity(Class<T> c, Element ele) throws InstantiationException, IllegalAccessException, NoSuchMethodException, SecurityException, IllegalArgumentException, InvocationTargetException { Field[] fields = c.getDeclaredFields(); Object object = c.newInstance();// for(Field f : fields) { String type = f.getType().toString();//获得字段的类型 String fieldName = f.getName();//获得字段名称 String param = fieldName.substring(0, 1).toUpperCase() + fieldName.substring(1);//把字段的第一个字母变成大写 Element e = ele.element(fieldName); if(type.indexOf("Integer") > -1) {//说明该字段是Integer类型 Integer i = null; if(e.getTextTrim() != null && !e.getTextTrim().equals("")) { i = Integer.parseInt(e.getTextTrim()); } Method m = c.getMethod("set" + param, Integer.class); m.invoke(object, i);//通过反射给该字段set值 } if(type.indexOf("Double") > -1) { //说明该字段是Double类型 Double d = null; if(e.getTextTrim() != null && !e.getTextTrim().equals("")) { d = Double.parseDouble(e.getTextTrim()); } Method m = c.getMethod("set" + param, Double.class); m.invoke(object, d); } if(type.indexOf("String") > -1) {//说明该字段是String类型 String s = null; if(e.getTextTrim() != null && !e.getTextTrim().equals("")) { s = e.getTextTrim(); } Method m = c.getMethod("set" + param, String.class); m.invoke(object, s); } } return (T)object; } /** * 用来写xml文件 * @param doc Document对象 * @param filePath 生成的文件路径 * @param encoding 写xml文件的编码 */ public static void writeXml(Document doc, String filePath, String encoding) { XMLWriter writer = null; OutputFormat format = OutputFormat.createPrettyPrint(); format.setEncoding(encoding);// 指定XML编码 try { writer = new XMLWriter(new FileWriter(filePath), format); writer.write(doc); } catch (IOException e) { e.printStackTrace(); } finally { try { writer.close(); } catch (IOException e) { e.printStackTrace(); } } } /** * 默认使用utf-8的格式写文件 * @param doc * @param filePath */ public static void writeXml(Document doc, String filePath) { writeXml(doc, filePath, "utf-8"); } }
假如有個實體類別是:
package com.pcq.entity; import java.io.Serializable; public class Emp implements Serializable{ private Integer id; private String name; private Integer deptNo; private Integer age; private String gender; private Integer bossId; private Double salary; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getDeptNo() { return deptNo; } public void setDeptNo(Integer deptNo) { this.deptNo = deptNo; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } public Integer getBossId() { return bossId; } public void setBossId(Integer bossId) { this.bossId = bossId; } public Double getSalary() { return salary; } public void setSalary(Double salary) { this.salary = salary; } }
那麼寫出來的xml檔案格式如下:
<?xml version="1.0" encoding="utf-8"?> <emps> <emp> <id>1</id> <name>张三</name> <deptNo>50</deptNo> <age>25</age> <gender>男</gender> <bossId>6</bossId> <salary>9000.0</salary> </emp> <emp> <id>2</id> <name>李四</name> <deptNo>50</deptNo> <age>22</age> <gender>女</gender> <bossId>6</bossId> <salary>8000.0</salary> </emp> </emps>
#假如有個實體類如下:
package com.pcq.entity; public class Student { private Integer id; private String name; private Integer age; private String gender; public Integer getId() { return id; } public void setId(Integer id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public Integer getAge() { return age; } public void setAge(Integer age) { this.age = age; } public String getGender() { return gender; } public void setGender(String gender) { this.gender = gender; } }
那麼寫出來的xml文件如下
<?xml version="1.0" encoding="utf-8"?> <students> <student> <id></id> <name>pcq</name> <age>18</age> <gender>男</gender> </student> </students>
讀取也必須讀這種格式的xml文件,才能轉換成實體類,要求是實體類的類別類型資訊(Class)必須要獲得到。
另外這裡的實體類別的屬性類型都是Integer,String,Double,可以看到工具類別裡只對這三種類型做了判斷。而且可以預想的是,如果出現一對多的關係,即一個實體類別擁有一組另一個類別物件的引用,
那xml和實體類別的相互轉換要比上述的情況複雜的多。 lz表示短時間內甚至長時間不一定能做的出來,歡迎同道高人指點。
相關推薦:
以上是實例詳解簡單實體類別和xml檔案的相互轉換方法的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
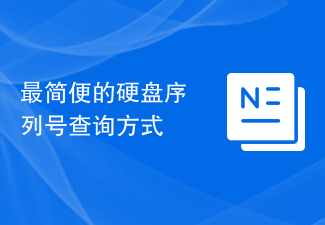
硬碟序號是硬碟的一個重要標識,通常用於唯一標識硬碟以及進行硬體識別。在某些情況下,我們可能需要查詢硬碟序號,例如在安裝作業系統、尋找正確裝置驅動程式或進行硬碟維修等情況下。本文將介紹一些簡單的方法,幫助大家查詢硬碟序號。方法一:使用Windows命令提示字元開啟命令提示字元。在Windows系統中,按下Win+R鍵,輸入"cmd"並按下回車鍵即可開啟命
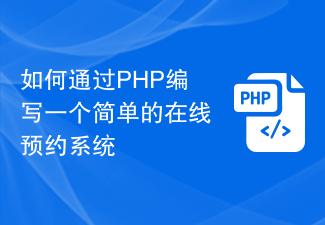
如何透過PHP編寫一個簡單的線上預約系統隨著網路的普及和使用者對便利性的追求,線上預約系統越來越受到歡迎。無論是餐廳、醫院、美容院或其他服務業,都可以透過簡單的線上預約系統來提高效率並為使用者提供更好的服務體驗。本文將介紹如何使用PHP編寫一個簡單的線上預約系統,並提供具體的程式碼範例。建立資料庫和表格首先,我們需要建立一個資料庫來儲存預約資訊。在MyS
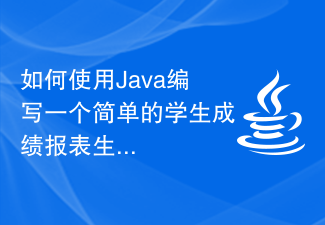
如何使用Java來寫一個簡單的學生成績報表產生器?學生成績報表產生器是可以幫助老師或教育者快速產生學生成績報告的工具。本文將介紹如何使用Java來撰寫簡單的學生成績報表產生器。首先,我們要定義學生對象和學生成績對象。學生對象包含學生的姓名、學號等基本訊息,而學生成績對象則包含學生的科目成績和平均成績等資訊。以下是一個簡單的學生物件的定義:public
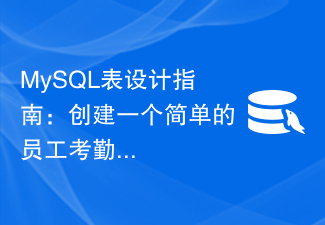
MySQL表設計指南:建立一個簡單的員工考勤表在企業管理中,員工的考勤管理是至關重要的任務。為了準確記錄和統計員工的考勤情況,我們可以利用MySQL資料庫來建立一個簡單的員工考勤表。本篇文章將指導您如何設計和建立這個表,並提供相應的程式碼範例。首先,我們需要確定員工考勤表所需的欄位。一般來說,員工考勤表至少需要包含以下欄位:員工ID、日期、上班時間、下班時
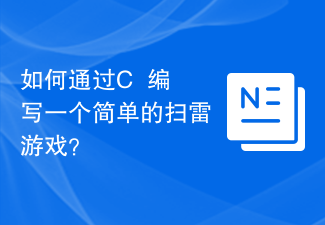
如何透過C++寫一個簡單的掃雷遊戲?掃雷遊戲是一款經典的益智類遊戲,它要求玩家根據已知的雷區佈局,在沒有踩到地雷的情況下,揭示所有的方塊。在這篇文章中,我們將介紹如何使用C++來寫一個簡單的掃雷遊戲。首先,我們需要定義一個二維陣列來表示掃雷遊戲的地圖。數組中的每個元素可以是一個結構體,用於儲存方塊的狀態,例如是否揭示、是否有雷等資訊。另外,我們還需要定義
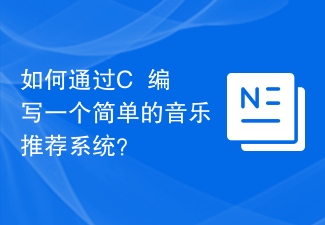
如何透過C++寫一個簡單的音樂推薦系統?引言:音樂推薦系統是現代資訊科技的研究熱點,它可以根據使用者的音樂偏好和行為習慣,向使用者推薦符合其口味的歌曲。本文將介紹如何使用C++來寫一個簡單的音樂推薦系統。一、收集用戶資料首先,我們需要收集用戶的音樂偏好資料。可以透過線上調查、問卷調查等方式來獲得使用者對不同類型音樂的喜好程度。將資料保存在一個文字檔案或資料庫
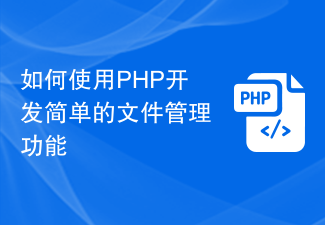
如何使用PHP開發簡單的文件管理功能簡介:文件管理功能在許多Web應用中都是不可或缺的一部分。它允許用戶上傳、下載、刪除和展示文件,為用戶提供了便捷的文件管理方式。本文將介紹如何使用PHP開發一個簡單的檔案管理功能,並提供具體的程式碼範例。一、創建專案首先,我們需要建立一個基本的PHP專案。在專案目錄下建立以下檔案:index.php:主頁面,用於顯示上傳表
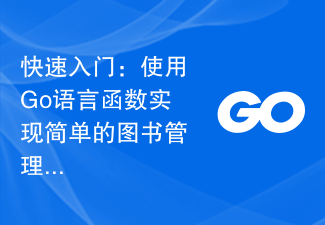
快速入門:使用Go語言函數實現簡單的圖書管理系統引言:隨著電腦科學領域的不斷發展,軟體應用的需求也越來越多樣化。圖書管理系統作為常見的管理工具,也成為許多圖書館、學校和企業必備的系統之一。在本文中,我們將使用Go語言函數來實作一個簡單的圖書管理系統。透過這個例子,讀者可以學習到Go語言中函數的基本用法以及如何建立一個實用的程式。一、設計思路:我們首先來
