PHP實作紅包金額拆分演算法案例詳解
May 17, 2018 am 10:51 AM
php
分割
演算法
這次帶給大家PHP實作紅包金額分割演算法案例詳解,PHP實作紅包金額分割演算法的注意事項有哪些,以下就是實戰案例,一起來看一下。
<?php // 新年红包金额拆分试玩 class CBonus { public $bonus;//红包 public $bonus_num;//红包个数 public $bonus_money;//红包总金额 public $money_single_max;//单个红包限额 public function construct(){ $this->bonus_num = 10; $this->bonus_money = 200; $this->money_single_max = 60; } private function randomFloat($min = 0, $max = 1) { $mt_rand = mt_rand(); $mt_getrandmax = mt_getrandmax(); echo 'mt_rand=' . $mt_rand . ', mt_getrandmax=' . $mt_getrandmax . '<hr/>'; return $min + $mt_rand / $mt_getrandmax * ($max - $min); } //计算 public function compute() { $this->bonus = array(); $bonus_money_temp = $this->bonus_money; $money_single_max = $this->money_single_max; $i = 1; while($i < $this->bonus_num) { if ($money_single_max > $bonus_money_temp) { $money_single_max = floatval(sprintf("%01.2f", $bonus_money_temp / 2));//剩余金额不够分时,把剩余金额的一半作为备用金 } $bonus_money_rad = $this->randomFloat(0.01, $money_single_max);//一个红包随机金额 最小的1分钱 $bonus_money_rad = floatval(sprintf("%01.2f", $bonus_money_rad)); $bonus_money_temp = $bonus_money_temp - $bonus_money_rad ;//待分配的总剩余金额 $bonus_money_temp = floatval(sprintf("%01.2f", $bonus_money_temp)); $this->bonus[] = $bonus_money_rad; //echo $bonus_money_rad . ',' . $bonus_money_temp . '<hr/>'; $i++; } $this->bonus[] = $bonus_money_temp;//分配剩余金额给最后一个红包 } //打印 public function output(){ $total = 0; foreach($this->bonus as $k => $v) { echo '红包' . ($k+1) . '=' . $v . '<br/>'; $total += $v; } echo '红包总金额:'.$total; } } $CBonus = new CBonus(); $CBonus->compute(); $CBonus->output(); ?>
登入後複製
示範結果:
紅包總金額:200紅包1=12.36
紅包10=7.15
紅包2=24.37
紅包3=42.71
紅包4=36.92
紅包5= 25.84
紅包6=23.17
紅包7=15.92
紅包8=1.35
紅包9=7.75
紅包10=9.61
紅包總金額:200
紅包1=24.59
紅包2=17.66
紅包3=29.67
紅包4=32.34
紅包5=12.67
紅包6=37.15
紅包7=17.41
紅包8=15.23
紅包9=6.13
相信看了本文案例你已經掌握了方法,更多精彩請關注php中文網其它相關文章!
## ####以上是PHP實作紅包金額拆分演算法案例詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!
本網站聲明
本文內容由網友自願投稿,版權歸原作者所有。本站不承擔相應的法律責任。如發現涉嫌抄襲或侵權的內容,請聯絡admin@php.cn

熱門文章
兩個點博物館:邦格荒地地點指南
4 週前
By 尊渡假赌尊渡假赌尊渡假赌
擊敗分裂小說需要多長時間?
3 週前
By DDD
倉庫:如何復興隊友
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island冒險:如何獲得巨型種子
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
公眾號網頁更新緩存難題:如何避免版本更新後舊緩存影響用戶體驗?
3 週前
By 王林

熱門文章
兩個點博物館:邦格荒地地點指南
4 週前
By 尊渡假赌尊渡假赌尊渡假赌
擊敗分裂小說需要多長時間?
3 週前
By DDD
倉庫:如何復興隊友
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
Hello Kitty Island冒險:如何獲得巨型種子
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
公眾號網頁更新緩存難題:如何避免版本更新後舊緩存影響用戶體驗?
3 週前
By 王林

熱門文章標籤

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
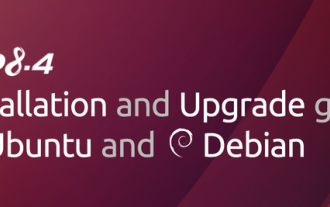
適用於 Ubuntu 和 Debian 的 PHP 8.4 安裝和升級指南
