React中生命週期使用詳解
這次帶給大家React中生命週期使用詳解,React中生命週期使用的注意事項有哪些,下面就是實戰案例,一起來看一下。
生命週期
React 是一個由虛擬 DOM 渲染成真實 DOM 的過程,這個過程稱為元件的生命週期。 React 把這個週期分成三個階段,每個階段都提供了 will 和 did 兩種處理方式,will 是指發生前,did 是指發生後。
Mounting:元件渲染過程
#componentWillMount()
componentDidMount()
Updating:元件更新過程
#componentWillReceiveProps(nextProps)
#shouldComponentUpdate(nextProps, nextState)
#componentWillUpdate(object nextProps, object nextState)
componentDidUpdate(object prevProps, #object prevProps
- Unmounting:元件移除過程
- #componentWillUnmount()
- 這個階段沒有對應的did 方法
- getDefaultProps
- #getInitialState ##componentWillMount
- render
- componentDidMount
- componentWillMount
該方法在render 之前被調用,也就是說在這個方法當中無法取得到真實的DOM 元素。
首次渲染前和當 state 改變時再次渲染前觸發方法。
componentDidMount
該方法是在 render 之後被調用,也就是這個方法可以直接取得到真實的 DOM 元素。
首次渲染後和當 state 發生變更再次渲染後觸發方法。var MountingComponent = React.createClass({ componentWillMount: function(){ console.log(this.refs.h1) // undefined }, componentDidMount: function(){ console.log(this.refs.h1) // h1 对象 }, render: function(){ return <h1 ref="h1">Lifecycle-Mounting</h1>; } }) ReactDOM.render(<MountingComponent />, document.getElementById('p1'));
Updating
當改變元件的props 或state 時候會觸發
執行順序
##componentWillReceiveProps- shouldComponentUpdate
- componentWillUpdate
- render
- componentDidUpdate
- componentWillReceiveProps
方法接受一個參數
newProps: 為更新後的props註:props 不能手動改變,正常場景是當前元件被當子元件調用,然後在父元件中改變該元件的props
shouldComponentUpdate
方法接受兩個參數
newProps:已更新的 propsnewState:已更新的 state
方法必須傳回 boolen,傳回 true 則執行後面的 componentWillUpdate、render、componentDidUpdate。反之則不執行。
componentWillUpdate
方法接受兩個參數
nextProps:將要更新的 propsnextState:將要更新的 state
componentDidUpdate
方法接受兩個參數
prevProps:更新前的 propsnextState:更新前的 state
var UpdatingComponent = React.createClass({ getInitialState: function() { return { data:0 }; }, setNewNumber: function() { //当 state 发生改变的时候,state 对应的组件会重新挂载 //会触发 componentWillUpdate、componentDidUpdate this.setState({data: this.state.data + 1}) }, //参数 newProps:已更新的 props componentWillReceiveProps:function(newProps) { console.log('Component WILL RECEIVE PROPS!', newProps) }, //参数 newProps:已更新的 props //参数 newState:已更新的 state //必须要返回 boolen,true 则执行componentWillUpdate、render、componentDidUpdate。反之则不执行。 shouldComponentUpdate: function(newProps, newState){ console.log('shouldComponentUpdate',newProps, newState); return (newState.data > 0 && newState.data % 2 == 0); }, //参数 nextProps:将要更新的 props //参数 nextState:将要更新的 state componentWillUpdate: function(nextProps, nextState){ console.log(nextProps, nextState, this.refs.p1) }, //参数 prevProps:更新前的 props //参数 nextState:更新前的 state componentDidUpdate: function(prevProps, prevState){ console.log(prevProps, prevState) }, render: function(){ return ( <p> <button onClick={this.setNewNumber}>INCREMENT</button> <h3>{this.state.data}</h3> </p> ); } }) ReactDOM.render(<UpdatingComponent/>, document.getElementById('p2'));
Unmounting
在组件从 DOM 中移除的时候立刻被调用,这个阶段没有对应的 did 方法
componentWillUnmount
方法适用在父子组件的结构中,当某个条件符合的场景下,该子组件会被渲染
重新渲染的执行顺序
getInitialState
componentWillMount
render
componentDidMount
var ChildrenComponent = React.createClass({ componentWillUnmount: function(){ console.log('componentWillUnmount'); }, render: function(){ return <h3>{this.props.myNumber}</h3> } }) var UnmountingComponent = React.createClass({ getInitialState: function() { return { data:0 }; }, setNewNumber: function() { this.setState({data: this.state.data + 1}) }, render: function () { var content; //当条件不符合时 ChildrenComponent 会被移除,然后会触发方组件的 componentWillUnmount 方法 //当条件重新符合时,会重新渲染组件 ChildrenComponent if(this.state.data % 2 == 0){ content = <ChildrenComponent myNumber = {this.state.data}></ChildrenComponent>; } else { content = <h3>{this.state.data}</h3>; } return ( <p> <button onClick = {this.setNewNumber}>INCREMENT</button> {content} </p> ); } }) ReactDOM.render(<UnmountingComponent/>, document.getElementById('p3'));
相信看了本文案例你已经掌握了方法,更多精彩请关注php中文网其它相关文章!
推荐阅读:
以上是React中生命週期使用詳解的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
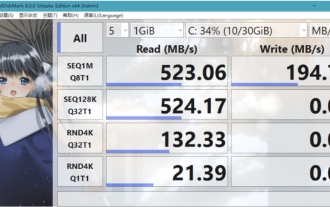
crystaldiskmark是什麼軟體? -crystaldiskmark如何使用?
