java實作獲取文字檔案的字元編碼
一、認識字元編碼:
1、Java中String的預設編碼為UTF-8,可以使用以下語句取得:Charset.defaultCharset( );
2、Windows作業系統下,文字檔案的預設編碼為ANSI,對中文Windows來說即為GBK。例如我們使用記事本程式新建一個文字文檔,其預設字元編碼即為ANSI。
3、Text文本文件有四種編碼選項:ANSI、Unicode(含Unicode Big Endian和Unicode Little Endian)、UTF-8、UTF-16
4、因此我們讀取txt檔案可能有時候不知道其編碼格式,所以需要用程式動態判斷取得txt檔案編碼。
ANSI :無格式定義,對中文作業系統為GBK或GB2312
UTF-8 :前三個位元組為:0xE59B9E(UTF-8)、0xEFBBBF(UTF-8含BOM)
UTF-16 :前兩個位元組為:0xFEFF
#Unicode:前兩個位元組為:0xFFFE
例如:Unicode文件以0xFFFE開頭,用程式取出前幾個位元組並進行判斷即可。
5、Java編碼與Text文字編碼對應關係:
#Java讀取Text文件,如果編碼格式不匹配,就會出現亂碼現象。所以讀取文字檔案的時候需要設定正確字元編碼。 Text文檔編碼格式都是寫在文件頭的,在程式中需要先解析文件的編碼格式,取得編碼格式後,再以此格式讀取檔就不會產生亂碼了。
免費線上影片教學推薦:java學習
二、舉例:
有一個文字檔:test.txt
##測試程式碼:
/** * 文件名:CharsetCodeTest.java * 功能描述:文件字符编码测试 */ import java.io.*; public class CharsetCodeTest { public static void main(String[] args) throws Exception { String filePath = "test.txt"; String content = readTxt(filePath); System.out.println(content); } public static String readTxt(String path) { StringBuilder content = new StringBuilder(""); try { String fileCharsetName = getFileCharsetName(path); System.out.println("文件的编码格式为:"+fileCharsetName); InputStream is = new FileInputStream(path); InputStreamReader isr = new InputStreamReader(is, fileCharsetName); BufferedReader br = new BufferedReader(isr); String str = ""; boolean isFirst = true; while (null != (str = br.readLine())) { if (!isFirst) content.append(System.lineSeparator()); //System.getProperty("line.separator"); else isFirst = false; content.append(str); } br.close(); } catch (Exception e) { e.printStackTrace(); System.err.println("读取文件:" + path + "失败!"); } return content.toString(); } public static String getFileCharsetName(String fileName) throws IOException { InputStream inputStream = new FileInputStream(fileName); byte[] head = new byte[3]; inputStream.read(head); String charsetName = "GBK";//或GB2312,即ANSI if (head[0] == -1 && head[1] == -2 ) //0xFFFE charsetName = "UTF-16"; else if (head[0] == -2 && head[1] == -1 ) //0xFEFF charsetName = "Unicode";//包含两种编码格式:UCS2-Big-Endian和UCS2-Little-Endian else if(head[0]==-27 && head[1]==-101 && head[2] ==-98) charsetName = "UTF-8"; //UTF-8(不含BOM) else if(head[0]==-17 && head[1]==-69 && head[2] ==-65) charsetName = "UTF-8"; //UTF-8-BOM inputStream.close(); //System.out.println(code); return charsetName; } }
執行結果:
相關文章教學建議: java入門學習
以上是java實作獲取文字檔案的字元編碼的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

AI Hentai Generator
免費產生 AI 無盡。

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)

熱門話題
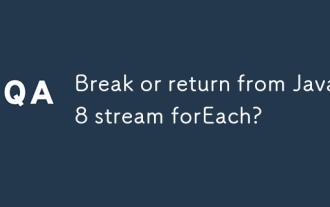
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
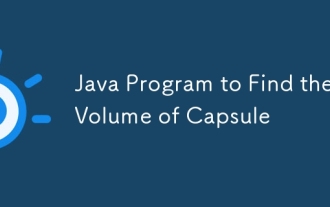
膠囊是一種三維幾何圖形,由一個圓柱體和兩端各一個半球體組成。膠囊的體積可以通過將圓柱體的體積和兩端半球體的體積相加來計算。本教程將討論如何使用不同的方法在Java中計算給定膠囊的體積。 膠囊體積公式 膠囊體積的公式如下: 膠囊體積 = 圓柱體體積 兩個半球體體積 其中, r: 半球體的半徑。 h: 圓柱體的高度(不包括半球體)。 例子 1 輸入 半徑 = 5 單位 高度 = 10 單位 輸出 體積 = 1570.8 立方單位 解釋 使用公式計算體積: 體積 = π × r2 × h (4
