Golang的memcache如何簡單實現
下面由golang教學欄位來介紹Golang簡單的memcache實作方法,希望對需要的朋友有幫助!
這兩天在做專案的過程中遇到了一個存取全域變數的問題場景:寫一個方法,取得id對應的token值,token需要快取起來(全域變數記憶體快取),如果取得不到或token的時間過期,那麼發送http請求到其他端去取,然後快取起來,然後再返回,那麼程式碼如下:
code.go:
package person import ( "time" ) var gAccId2Token map[int]interface{} = make(map[int]interface{}) func GetTokenByAccountId(accountId uint, acl string) (map[string]interface{}, error) { //get token from cache if token, ok := gAccId2Token[accountId]; ok { if token != nil { now := time.Now().Unix() if int(now) < int(token.(map[string]interface{})["expireDate"].(float64)) { return token.(map[string]interface{}), nil } } } token, err := getTokenByHttpUrl(apiUrl) if err != nil { return map[string]interface{}{}, err } gAccId2Token[accountId] = token return token.(map[string]interface{}), nil }
那麼問題來了:
1.由於gAccId2Token變數是全域變量,那麼會出現同時讀寫的情況,則會可能出現讀寫不一致的情況。
2.就本例來看,獲取id=2的token,而緩存的token已經過期了,那麼就會發送http請求去獲取,之後寫緩存,假設寫緩存的時間很長,而在這段時間內,又恰好有大量請求來獲取id=2的token,由於token都過期了,就會出現大量請求http服務端的問題,不僅沒有起到獲取緩存的目的,又增大了後端的壓力,同時又有多個寫快取的操作,而golang的map應該不是原子的,那麼大量寫記憶體也可能會造成crash的問題。
因此,我們需要對讀寫操作進行加鎖:
memcache.go:
package person import ( "sync" "time" ) type memoryCache struct { lock *sync.RWMutex items map[interface{}]interface{} } func (mc *memoryCache) set(key interface{}, value interface{}) error { mc.lock.Lock() defer mc.lock.Unlock() mc.items[key] = value return nil } func (mc *memoryCache) get(key interface{}) interface{} { mc.lock.RLock() defer mc.lock.RUnlock() if val, ok := mc.items[key]; ok { return val } return nil } var gAccId2Token *memoryCache = &memoryCache{ lock: new(sync.RWMutex), items: make(map[interface{}]interface{}), } func GetTokenByAccountId(accountId uint, acl string) (map[string]interface{}, error) { //get token from cache token := gAccId2Token.get(accountId) if token != nil { now := time.Now().Unix() if int(now) < int(token.(map[string]interface{})["expireDate"].(float64)) { return token.(map[string]interface{}), nil } } token, err := getTokenByHttpUrl(apiUrl) if err != nil { return map[string]interface{}{}, err } gAccId2Token.set(accountId, token) return token.(map[string]interface{}), nil }
幾點說明:
#1.為寫入操作上了全域鎖,一旦Lock()之後,其他lock就無法上鎖,直到釋放鎖Unlock()之後才行,也就是說保證寫入作業的原子性。
2.而為讀取操作上了讀鎖,那麼可以有多個線程Rlock()對一個區域枷鎖,從而保證區域是可讀的,直到所有讀鎖都RUnlock()之後,才可以上寫鎖。
3.將map的key和value的類型定義為interface{}類型,interface{}可以接收任何類型,就像是Java中的Object。
4.interface{}類型轉換的方法,value.(type),即將value轉換成為type類型,例如:value.(int),value.(map[string]interface{})等等。
以上是Golang的memcache如何簡單實現的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
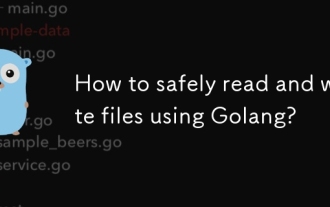
在Go中安全地讀取和寫入檔案至關重要。指南包括:檢查檔案權限使用defer關閉檔案驗證檔案路徑使用上下文逾時遵循這些準則可確保資料的安全性和應用程式的健全性。
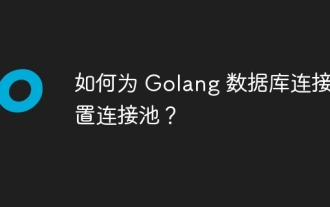
如何為Go資料庫連線配置連線池?使用database/sql包中的DB類型建立資料庫連線;設定MaxOpenConns以控制最大並發連線數;設定MaxIdleConns以設定最大空閒連線數;設定ConnMaxLifetime以控制連線的最大生命週期。
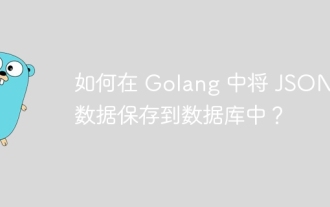
可以透過使用gjson函式庫或json.Unmarshal函數將JSON資料儲存到MySQL資料庫中。 gjson函式庫提供了方便的方法來解析JSON字段,而json.Unmarshal函數需要一個目標類型指標來解組JSON資料。這兩種方法都需要準備SQL語句和執行插入操作來將資料持久化到資料庫中。
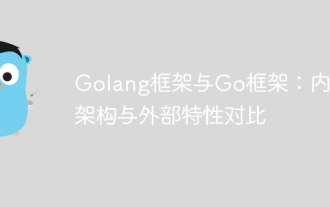
GoLang框架與Go框架的差異體現在內部架構與外部特性。 GoLang框架基於Go標準函式庫,擴充其功能,而Go框架由獨立函式庫組成,以實現特定目的。 GoLang框架更靈活,Go框架更容易上手。 GoLang框架在效能上稍有優勢,Go框架的可擴充性更高。案例:gin-gonic(Go框架)用於建立RESTAPI,而Echo(GoLang框架)用於建立Web應用程式。
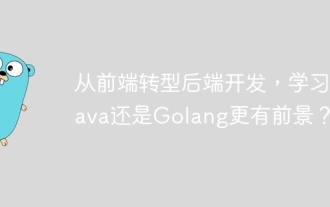
後端學習路徑:從前端轉型到後端的探索之旅作為一名從前端開發轉型的後端初學者,你已經有了nodejs的基礎,...
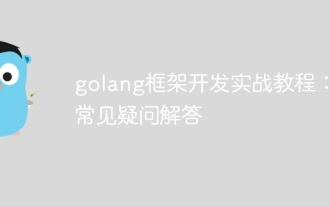
Go框架開發常見問題:框架選擇:取決於應用需求和開發者偏好,如Gin(API)、Echo(可擴展)、Beego(ORM)、Iris(效能)。安裝和使用:使用gomod指令安裝,導入框架並使用。資料庫互動:使用ORM庫,如gorm,建立資料庫連線和操作。身份驗證和授權:使用會話管理和身份驗證中間件,如gin-contrib/sessions。實戰案例:使用Gin框架建立一個簡單的部落格API,提供POST、GET等功能。
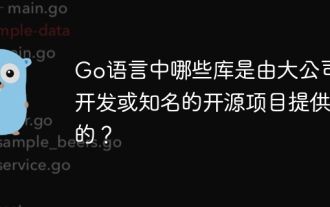
Go語言中哪些庫是大公司開發或知名開源項目?在使用Go語言進行編程時,開發者常常會遇到一些常見的需求,�...
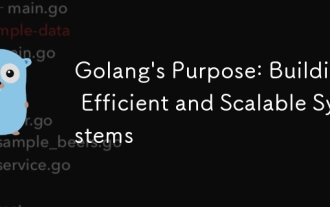
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
