go語言怎麼比較字串
golang
go語言
<blockquote><p>比較方法:1、直接使用“==”運算子比較,語法“str1 == str2”,該方法區分大小寫。 2.利用strings套件的Compare()函數比較,語法「strings.Compare(a,b)」;傳回值為int型別,0表示兩數相等,1表示a大於b,「-1」表示a小於b 。 3.利用strings套件的EqualFold()比較,語法「strings.EqualFold(a,b)」。 </p></blockquote>
<p><img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/article/000/000/024/63c224119dee6813.jpg" class="lazy" alt="go語言怎麼比較字串" ></p>
<p>本教學操作環境:windows7系統、GO 1.18版本、Dell G3電腦。 </p>
<h2 id="strong-Go語言比較字串方式-strong"><strong>Go語言比較字串方式</strong></h2>
<p>在go 語言中字串比較的方式有以下三種:</p>
<ul class="ul-level-0">
<li>
<code> ==</code> 直接比較,區分大小寫</li>
<li>
<code>strings.Compare(a,b)</code> 此函數傳回值為int, 0 表示兩數相等,1 表示a>b, -1 表示a<b><li>
<code>strings.EqualFold(a,b)</code> 直接回傳是否相等,不區分大小寫。 </li></b>
</li>
</ul>
<p>範例如下:<span style="background-color: rgb(248, 248, 248);">// 1-使用等號比較-區分大消息</span></p>
<div class="developer-code-block">#<div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>func Equal(s1, s2 string) bool {
return s1 == s2
}
// 2-使用 compare 比较——区分大小写
func Compare(s1, s2 string) bool {
return strings.Compare(s1, s2) == 0 //
}
//3-EqualFold 比较——不区分大小写. case-fold 即大小写同一处理
func EqualFold(s1, s2 string) bool {
return strings.EqualFold(s1, s2)
}
// 使用等号比较——忽略大小写
func Equal2(s1, s2 string) bool {
return strings.ToLower(s1) == strings.ToLower(s2)
}
// 使用 compare 比较——不区分大小写
func Compare2(s1, s2 string) bool {
return strings.Compare(strings.ToLower(s1), strings.ToLower(s2)) == 0
}
func StringCompareTest() {
fmt.Println("== 区分大小写", Equal("go", "Go")) //false
fmt.Println("== 忽略大小写",Equal2("go", "Go")) //true
fmt.Println("compare 区分大小写",Compare("go", "Go")) //false
fmt.Println("compare 忽略大小写",Compare2("go", "Go")) //true
fmt.Println("EqualFold 忽略大小写",EqualFold("go", "Go")) // true
}</pre><div class="contentsignin">登入後複製</div></div></div><h2 id="strong-效能比較-strong"><strong>效能比較</strong></h2><p>下面的程式碼使用Benchmark 做簡單的效能比較,測試專案的目錄結構為:</p><p><img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/image/916/800/564/167367265364778go語言怎麼比較字串" class="lazy" title="167367265364778go語言怎麼比較字串" alt="go語言怎麼比較字串"/></p><p>詳細程式碼:</p><div class="developer-code-block"> <div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>package test
import (
"../str"
"testing"
)
func BenchmarkStrEqual(b *testing.B) {
for i := 0; i < b.N; i++ {
str.Equal("go", "Go")
}
}
func BenchmarkStrEqual2(b *testing.B) {
for i := 0; i < b.N; i++ {
str.Equal2("go", "Go")
}
}
func BenchmarkStrCompare(b *testing.B) {
for i := 0; i < b.N; i++ {
str.Compare("go", "Go")
}
}
func BenchmarkStrCompare2(b *testing.B) {
for i := 0; i < b.N; i++ {
str.Compare2("go", "Go")
}
}
func BenchmarkStrEqualFold(b *testing.B) {
for i := 0; i < b.N; i++ {
str.EqualFold("go", "Go")
}
}</pre><div class="contentsignin">登入後複製</div></div></div><p>測試結果如下:</p><p><img src="/static/imghw/default1.png" data-src="https://img.php.cn/upload/image/725/103/124/1673672666716944.png" class="lazy" title="1673672666716944.png" alt="go語言怎麼比較字串"/></p><p>透過上圖可以看出,效率最高的還是==</p><h2 id="strong-原始碼簡單分析-strong"><strong>原始碼簡單分析</strong></h2><h4 id="strong-strings-Compare-strong"><strong>1、strings.Compare</strong></h4><div class="developer-code-block"><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>package strings
// Compare returns an integer comparing two strings lexicographically.
// The result will be 0 if a==b, -1 if a < b, and +1 if a > b.
//
// Compare is included only for symmetry with package bytes.
// It is usually clearer and always faster to use the built-in
// string comparison operators ==, <, >, and so on.
func Compare(a, b string) int {
// NOTE(rsc): This function does NOT call the runtime cmpstring function,
// because we do not want to provide any performance justification for
// using strings.Compare. Basically no one should use strings.Compare.
// As the comment above says, it is here only for symmetry with package bytes.
// If performance is important, the compiler should be changed to recognize
// the pattern so that all code doing three-way comparisons, not just code
// using strings.Compare, can benefit.
if a == b {
return 0
}
if a < b {
return -1
}
return +1
}</pre><div class="contentsignin">登入後複製</div></div></div><p>如上所示,我們發現,Compare 內部也是呼叫了<code>= =</code> , 而且函數的註解中也說了,這個函數only for symmetry with package bytes。而且推薦我們直接使用 <code>==</code> 和 <code>></code>、<code><</code>。 </p><h4 id="strong-strings-EqualFold-strong"><strong>2、strings.EqualFold</strong></h4><div class="developer-code-block"><div class="code" style="position:relative; padding:0px; margin:0px;"><pre class='brush:php;toolbar:false;'>// EqualFold reports whether s and t, interpreted as UTF-8 strings,
// are equal under Unicode case-folding, which is a more general
// form of case-insensitivity.
func EqualFold(s, t string) bool {
for s != "" && t != "" {
// Extract first rune from each string.
var sr, tr rune
if s[0] < utf8.RuneSelf {
sr, s = rune(s[0]), s[1:]
} else {
r, size := utf8.DecodeRuneInString(s)
sr, s = r, s[size:]
}
if t[0] < utf8.RuneSelf {
tr, t = rune(t[0]), t[1:]
} else {
r, size := utf8.DecodeRuneInString(t)
tr, t = r, t[size:]
}
// If they match, keep going; if not, return false.
// Easy case.
if tr == sr {
continue
}
// Make sr < tr to simplify what follows.
if tr < sr {
tr, sr = sr, tr
}
// Fast check for ASCII.
if tr < utf8.RuneSelf {
// ASCII only, sr/tr must be upper/lower case
if 'A' <= sr && sr <= 'Z' && tr == sr+'a'-'A' {
continue
}
return false
}
// General case. SimpleFold(x) returns the next equivalent rune > x
// or wraps around to smaller values.
r := unicode.SimpleFold(sr)
for r != sr && r < tr {
r = unicode.SimpleFold(r)
}
if r == tr {
continue
}
return false
}
// One string is empty. Are both?
return s == t
}</pre><div class="contentsignin">登入後複製</div></div></div>
<p>這個函數中做了一系列操作,將兩個字串轉換成<code>utf -8</code> 字串進行比較,並且在比較時忽略大小寫。 </p>
<h2 id="strong-總結-strong"><strong>總結</strong></h2>
<p>透過上面的簡單總結與分析,我們發現,字串比較還是直接用== 、>、 </p>
<p>【相關推薦:<a href="http://www.php.cn/course/list/44.html" target="_blank">Go影片教學</a>、<a href="https://www.php.cn/course.html" target="_blank" textvalue="编程教学">程式設計教學</a>】</p>
以上是go語言怎麼比較字串的詳細內容。更多資訊請關注PHP中文網其他相關文章!
本網站聲明
本文內容由網友自願投稿,版權歸原作者所有。本站不承擔相應的法律責任。如發現涉嫌抄襲或侵權的內容,請聯絡admin@php.cn

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章
<🎜>:種植花園 - 完整的突變指南
3 週前
By DDD
<🎜>:泡泡膠模擬器無窮大 - 如何獲取和使用皇家鑰匙
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
如何修復KB5055612無法在Windows 10中安裝?
3 週前
By DDD
北端:融合系統,解釋
3 週前
By 尊渡假赌尊渡假赌尊渡假赌
Mandragora:巫婆樹的耳語 - 如何解鎖抓鉤
3 週前
By 尊渡假赌尊渡假赌尊渡假赌

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
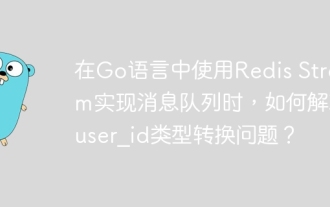
Go語言中使用RedisStream實現消息隊列時類型轉換問題在使用Go語言與Redis...
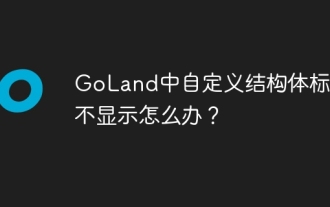
GoLand中自定義結構體標籤不顯示怎麼辦?在使用GoLand進行Go語言開發時,很多開發者會遇到自定義結構體標籤在�...
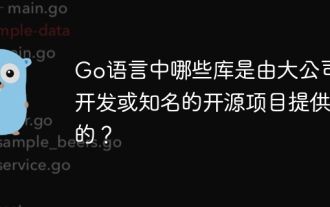
Go語言中哪些庫是大公司開發或知名開源項目?在使用Go語言進行編程時,開發者常常會遇到一些常見的需求,�...
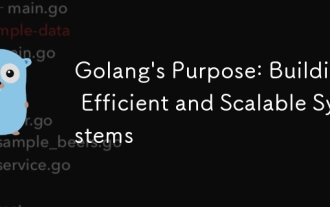
Go語言在構建高效且可擴展的系統中表現出色,其優勢包括:1.高性能:編譯成機器碼,運行速度快;2.並發編程:通過goroutines和channels簡化多任務處理;3.簡潔性:語法簡潔,降低學習和維護成本;4.跨平台:支持跨平台編譯,方便部署。
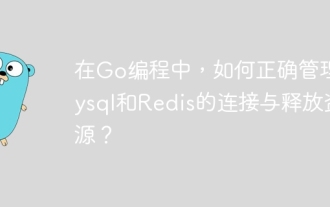
Go編程中的資源管理:Mysql和Redis的連接與釋放在學習Go編程過程中,如何正確管理資源,特別是與數據庫和緩存�...
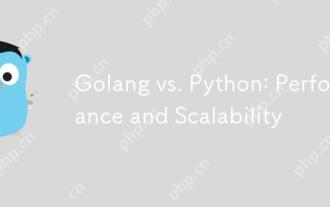
Golang在性能和可擴展性方面優於Python。 1)Golang的編譯型特性和高效並發模型使其在高並發場景下表現出色。 2)Python作為解釋型語言,執行速度較慢,但通過工具如Cython可優化性能。
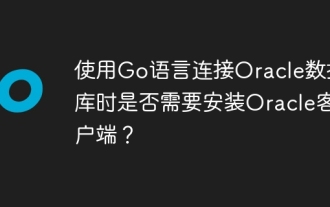
使用Go語言連接Oracle數據庫時是否需要安裝Oracle客戶端?在使用Go語言開發時,連接Oracle數據庫是一個常見需求�...
