Laravel開發:如何使用Laravel Eloquent建立資料庫模型?
Laravel開發:如何使用Laravel Eloquent建立資料庫模型?
Laravel是一款廣受歡迎的PHP框架,其提供了強大且易於使用的資料庫操作工具-Laravel Eloquent。在過去,要使用PHP進行資料庫操作難免要寫大量冗長的SQL語句和繁瑣的程式碼,而使用Laravel Eloquent則能夠輕鬆地建立資料庫模型,實現快速開發和維護。本文將介紹如何使用Laravel Eloquent建立資料庫模型。
一、建立資料庫表
首先,需要透過資料庫遷移(Migration)建立資料庫表。在Laravel中,可以使用命令列工具artisan來實現此過程。在命令列中輸入:
php artisan make:migration create_users_table
該命令會在app/database/migrations目錄下建立一個遷移文件,文件名稱為當前日期和時間加上遷移的名稱,例如2019_08_17_000000_create_users_table.php。修改遷移文件,編寫對應的資料庫結構。
Schema::create('users', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->string('email')->unique(); $table->timestamp('email_verified_at')->nullable(); $table->string('password'); $table->rememberToken(); $table->timestamps(); });
以上程式碼建立了一個名為users的表,其中包含了id、name、email、email_verified_at、password、remember_token、created_at和updated_at共8個欄位。接下來,運行遷移文件,建立資料庫表。
php artisan migrate
二、建立模型
在應用程式中建立模型(Model)是使用Laravel Eloquent的第一步。可以透過artisan工具來建立一個模型:
php artisan make:model User
上面的指令將在app目錄下建立一個名為User的模型,該模型對應著資料庫中的users表。預設情況下,Laravel Eloquent假定資料庫表名是模型名稱的複數形式,如果需要對應的是不同的表名或使用不同的資料庫連接,可以在模型中定義屬性$table和$connection。
模型的定義如下:
namespace App; use IlluminateDatabaseEloquentModel; class User extends Model { // }
三、模型屬性
在模型中,Laravel Eloquent已定義了一些預設屬性和方法,其中包括:
- $fillable屬性:定義可以被批次賦值的屬性,以防止注入攻擊。可以從建立/更新的請求中使用create()和update()方法進行填充。
protected $fillable = [ 'name', 'email', 'password', ];
- $hidden屬性:定義應在陣列中隱藏的屬性。當進行序列化操作時,這些屬性將會被隱藏。
protected $hidden = [ 'password', 'remember_token', ];
- $casts屬性:定義屬性應轉換為原生類型(整數、布林型、浮點型等)或自訂的物件。
protected $casts = [ 'email_verified_at' => 'datetime', ];
四、模型方法
Laravel Eloquent提供了一些方法,以便在模型中進行資料運算。以下是一些最常見的模型方法:
- where():用於新增WHERE條件。
$user = User::where('name', 'John')->first();
- find():用於透過模型的主鍵ID來尋找記錄。
$user_id = 1; $user = User::find($user_id);
- first():傳回第一個查找到的記錄。
$user = User::where('name', 'John')->first();
- get():傳回查找到的所有記錄。
$users = User::all();
- create():用於建立一筆新的資料記錄。
User::create(['name' => 'Taylor', 'email' => 'taylor@example.com', 'password' => 'password']);
- update():用於更新記錄。
$user = User::find($user_id); $user->name = 'Updated Name'; $user->save();
- delete():用於刪除記錄。
$user = User::find($user_id); $user->delete();
以上是一些基本的Laravel Eloquent模型方法,可以快速的實作對資料庫的增、刪、改、查操作。
五、關聯關係
Laravel Eloquent也提供了一種便捷的方式來定義各種關聯性:一對一(One to One)、一對一(One to Many) 、多對多(Many to Many)和多態關聯(Polymorphic Relations)。以下是一些例子:
- 一對一(One to One)
在一對一的關聯關係中,每個模型實例只與另一個相關模型實例相關聯。例如,在users表中的每行資料都可能與一個phone表中的行相關聯,phone表儲存了使用者的電話號碼。在User模型中,定義一個phone()方法,表示模型與phone模型之間的一對一關係。
class User extends Model { public function phone() { return $this->hasOne('AppPhone'); } }
在Phone模型中,定義相反的hasOne()方法。
class Phone extends Model { public function user() { return $this->belongsTo('AppUser'); } }
- 一對多(One to Many)
在一對多的關聯關係中,一個模型實例與另一個模型實例相關聯,而另一個實例可以關聯多個模型實例。例如,在一個論壇網站中,每個範本可能與許多評論相關聯。在Thread模型中,定義一個comments()方法,表示模型與Comment模型之間的一對多關係。
class Thread extends Model { public function comments() { return $this->hasMany('AppComment'); } }
在Comment模型中,定義相反的belongsTo()方法。
class Comment extends Model { public function thread() { return $this->belongsTo('AppThread'); } }
- 多對多(Many to Many)
在多對多的關聯關係中,該模型實例與許多其他模型實例相關聯,而每個相關的模型實例也可以與多個模型實例關聯。例如,在一個部落格中,每篇文章可能有多個分類標籤,每個標籤也可能有多篇文章。在Post模型中,定義一個tags()方法,表示模型與Tag模型之間的多對多關係。
class Post extends Model { public function tags() { return $this->belongsToMany('AppTag'); } }
在Tag模型中,定義相反的belongsToMany()方法。
class Tag extends Model { public function posts() { return $this->belongsToMany('AppPost'); } }
- 多态关联(Polymorphic Relations)
多态关联允许模型通过多个中介模型与其他模型进行多对多关联。例如,在应用中可以使用comments模型对其他类型的模型进行评论。在Comment模型中,定义一个commentable()方法,表示该模型与所有支持评论的模型之间的多态关系。
class Comment extends Model { public function commentable() { return $this->morphTo(); } }
在支持评论的模型中,例如Post和Video模型中,定义morphMany()方法。
class Post extends Model { public function comments() { return $this->morphMany('AppComment', 'commentable'); } } class Video extends Model { public function comments() { return $this->morphMany('AppComment', 'commentable'); } }
以上是Laravel Eloquent提供的关联关系,可以让开发者在数据库模型中轻松处理复杂的关系结构。
七、总结
本文介绍了使用Laravel Eloquent构建数据库模型的基础知识,包括创建数据库表、创建模型、模型属性和方法,以及关联关系。Laravel Eloquent提供了一种简单和直观的方式来操作数据库,使得开发者能够快速构建应用程序,并为复杂的数据库结构提供了更干净、易于维护的解决方案。希望这篇文章对你的学习和开发有所帮助。
以上是Laravel開發:如何使用Laravel Eloquent建立資料庫模型?的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
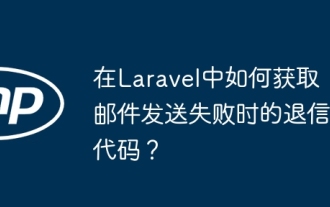
Laravel郵件發送失敗時的退信代碼獲取方法在使用Laravel開發應用時,經常會遇到需要發送驗證碼的情況。而在實�...
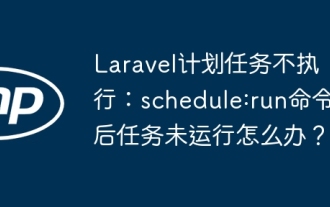
Laravel計劃任務運行無響應排查在使用Laravel的計劃任務調度時,不少開發者會遇到這樣的問題:schedule:run...
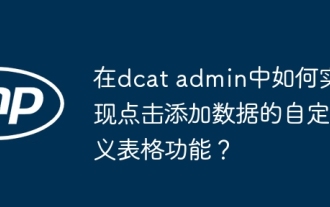
在dcatadmin(laravel-admin)中如何實現自定義點擊添加數據的表格功能在使用dcat...
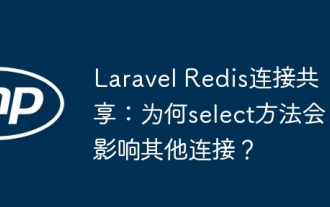
Laravel框架中Redis連接的共享與select方法的影響在使用Laravel框架和Redis時,開發者可能會遇到一個問題:通過配置...
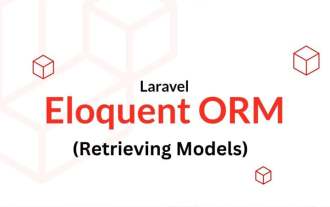
LaravelEloquent模型檢索:輕鬆獲取數據庫數據EloquentORM提供了簡潔易懂的方式來操作數據庫。本文將詳細介紹各種Eloquent模型檢索技巧,助您高效地從數據庫中獲取數據。 1.獲取所有記錄使用all()方法可以獲取數據庫表中的所有記錄:useApp\Models\Post;$posts=Post::all();這將返回一個集合(Collection)。您可以使用foreach循環或其他集合方法訪問數據:foreach($postsas$post){echo$post->
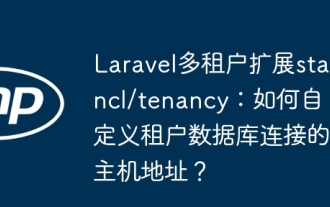
在Laravel多租戶擴展包stancl/tenancy中自定義租戶數據庫連接使用Laravel多租戶擴展包stancl/tenancy構建多租戶應用時,...
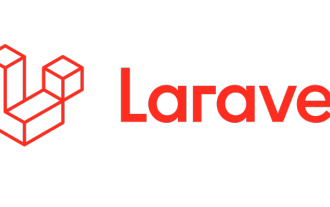
利用地理空間技術高效處理700萬條記錄並創建交互式地圖本文探討如何使用Laravel和MySQL高效處理超過700萬條記錄,並將其轉換為可交互的地圖可視化。初始挑戰項目需求:利用MySQL數據庫中700萬條記錄,提取有價值的見解。許多人首先考慮編程語言,卻忽略了數據庫本身:它能否滿足需求?是否需要數據遷移或結構調整? MySQL能否承受如此大的數據負載?初步分析:需要確定關鍵過濾器和屬性。經過分析,發現僅少數屬性與解決方案相關。我們驗證了過濾器的可行性,並設置了一些限制來優化搜索。地圖搜索基於城
