如何使用Go語言和Vue.js建立地圖應用程式
在當今高度互聯的世界中,地圖應用程式已成為各種應用場景的重要組成部分。而Go語言和Vue.js分別代表著高效、輕量級的後端語言和現代、全面的前端框架,可以為地圖應用程式提供強大的技術支援。本文將介紹如何使用Go語言和Vue.js建立一個簡單的地圖應用程式。
第一步:選擇地圖API
首先,需要選擇一個可用的地圖API。 Google Maps、Baidu Maps、高德地圖等都是常見的選擇。這裡我們選擇了Mapbox,它提供了強大的地圖渲染和覆蓋物繪製功能,並提供了良好的開發者文件和SDK。
第二步:後端建構
使用Go語言來建構後端。這裡我們建議使用Echo框架,它的API設計簡單、易於使用,並已廣泛應用於生產環境。以下是引入所需的套件以及初始化Echo的程式碼:
import ( "github.com/labstack/echo" "github.com/labstack/echo/middleware" ) func main() { e := echo.New() // Middleware e.Use(middleware.Logger()) e.Use(middleware.Recover()) // Routes e.GET("/", hello) // Start server e.Logger.Fatal(e.Start(":1323")) }
在這裡,我們引用了Echo和middleware兩個套件,並使用Echo初始化了HTTP伺服器。透過e.GET("/", hello)
可以定義HTTP的GET方法,該方法將在根URL上呼叫hello
函數。使用e.Logger.Fatal(e.Start(":1323"))
可以輕鬆啟動HTTP伺服器,並監聽1323連接埠。
接下來,我們需要請求Mapbox API,並將結果傳回Vue.js前端。這裡我們將定義一個/api/location
路由,並在其中使用echo.Context
來非同步請求Mapbox API。下面是API邏輯的範例程式碼:
type MapboxResponse struct { Features []struct { Text string `json:"text"` Geometry struct { Coordinates []float64 `json:"coordinates"` } `json:"geometry"` } `json:"features"` } func getLocation(c echo.Context) error { address := c.QueryParam("address") url := fmt.Sprintf("https://api.mapbox.com/geocoding/v5/mapbox.places/%s.json?access_token=%s", address, "<your_mapbox_api_key>") req, err := http.NewRequest("GET", url, nil) if err != nil { return c.String(http.StatusInternalServerError, "Failed to create request: "+err.Error()) } client := &http.Client{} resp, err := client.Do(req) if err != nil { return c.String(http.StatusInternalServerError, "Failed to get location from mapbox: "+err.Error()) } defer resp.Body.Close() var mapboxResponse MapboxResponse if err := json.NewDecoder(resp.Body).Decode(&mapboxResponse); err != nil { return c.String(http.StatusInternalServerError, "Failed to decode mapbox response: "+err.Error()) } if len(mapboxResponse.Features) > 0 { return c.JSON(http.StatusOK, mapboxResponse.Features[0]) } else { return c.String(http.StatusNotFound, "Location not found") } }
在這裡,我們定義了MapboxResponse
結構體,該結構體的屬性與Mapbox API的回應欄位一一對應。在getLocation
函數中,我們先取得查詢參數address
,然後建構Mapbox API的URL,透過http.NewRequest方法來發起非同步請求。最後,我們將回應JSON解碼為MapboxResponse
結構體,並傳回HTTP的JSON回應。
第三個步驟:前端建置
使用Vue.js建構前端。使用Vue.js可以方便地處理資料綁定和組件化,從而使地圖應用程式更加靈活。以下是建立Vue實例和初始化地圖的程式碼:
import Vue from 'vue' import App from './App.vue' Vue.config.productionTip = false new Vue({ render: h => h(App), }).$mount('#app') mapboxgl.accessToken = '<your_mapbox_access_token>'; var map = new mapboxgl.Map({ container: 'map', style: 'mapbox://styles/mapbox/streets-v11', center: [-73.985753, 40.748817], zoom: 12 });
在這裡,我們先透過new Vue()
來建立Vue實例並綁定到id為#app
的DOM元素上。接著,我們使用mapboxgl.accessToken
來設定Mapbox API的存取令牌,並使用new mapboxgl.Map()
來初始化地圖物件。在此處,我們定義了初始的地圖樣式、中心點座標和縮放等級等屬性。
接下來,我們需要在Vue中定義一個輸入框和一個按鈕,當使用者點擊按鈕時,我們將查詢地址發給後端,並將結果顯示在地圖上。下面是Vue元件的程式碼:
<template> <div> <div> <input type="text" v-model="address"> <button @click="getLocation()">Search</button> </div> <div id="map"></div> </div> </template> <script> export default { name: 'app', data () { return { address: '', map: null, marker: null } }, methods: { getLocation () { fetch('/api/location?address=' + this.address) .then(res => res.json()) .then(location => { if (this.marker) { this.marker.remove() } this.marker = new mapboxgl.Marker().setLngLat(location.geometry.coordinates).addTo(this.map) this.map.flyTo({ center: location.geometry.coordinates, zoom: 15 }) }) .catch(error => console.error(error)) } }, mounted () { this.map = new mapboxgl.Map({ container: 'map', style: 'mapbox://styles/mapbox/streets-v11', center: [-73.985753, 40.748817], zoom: 12 }) } } </script> <style> #map { height: 500px; } </style>
在上述Vue元件中,我們定義了一個輸入框和一個按鈕,當使用者點擊按鈕時,呼叫getLocation
方法,並使用fetch
來非同步取得後端的Mapbox回應。如果回應成功,我們將透過地圖API的Map
和Marker
物件來在地圖上顯示結果,並執行flyTo
方法來平滑地移動地圖視圖。
第四步:啟動應用程式
最後,我們將後端和前端組裝起來,並啟動應用程式。可以使用以下步驟來執行該操作:
- 將上述Go程式碼儲存到某個目錄下,並執行
go mod init
來初始化專案。 - 將上述Vue程式碼儲存到
src/App.vue
檔案中,並將該檔案與它的依賴項一起編譯到dist
目錄中。 - 啟動後端服務:
go run .
- 在瀏覽器中開啟
dist/index.html
文件,即可執行地圖應用程式。
綜上所述,我們使用了Go語言和Vue.js來建立了一個基本的地圖應用程式。該應用程式透過組合Mapbox API、Echo框架和Vue.js等工具,實現了簡單而高效的後端邏輯和現代而靈活的前端元件。利用這些技術,我們可以更輕鬆地建立更為複雜的地圖應用程序,並為用戶提供更好的體驗和功能。
以上是如何使用Go語言和Vue.js建立地圖應用程式的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
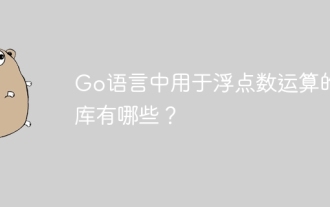
Go語言中用於浮點數運算的庫介紹在Go語言(也稱為Golang)中,進行浮點數的加減乘除運算時,如何確保精度是�...
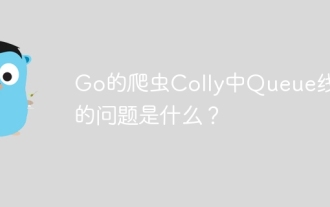
Go爬蟲Colly中的Queue線程問題探討在使用Go語言的Colly爬蟲庫時,開發者常常會遇到關於線程和請求隊列的問題。 �...
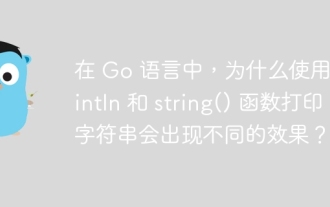
Go語言中字符串打印的區別:使用Println與string()函數的效果差異在Go...
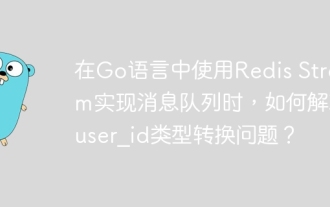
Go語言中使用RedisStream實現消息隊列時類型轉換問題在使用Go語言與Redis...
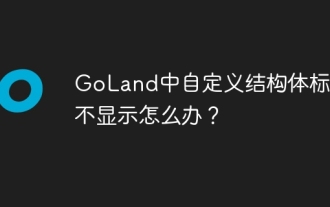
GoLand中自定義結構體標籤不顯示怎麼辦?在使用GoLand進行Go語言開發時,很多開發者會遇到自定義結構體標籤在�...
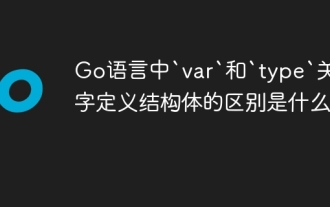
Go語言中結構體定義的兩種方式:var與type關鍵字的差異Go語言在定義結構體時,經常會看到兩種不同的寫法:一�...
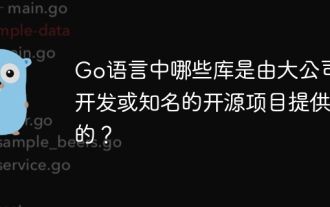
Go語言中哪些庫是大公司開發或知名開源項目?在使用Go語言進行編程時,開發者常常會遇到一些常見的需求,�...
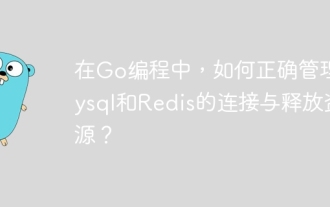
Go編程中的資源管理:Mysql和Redis的連接與釋放在學習Go編程過程中,如何正確管理資源,特別是與數據庫和緩存�...
