Java API 開發中使用 Apache Shiro 進行權限控制
Java API 開發中使用 Apache Shiro 進行權限控制
隨著網路技術的發展,越來越多的應用程式採用了基於 API 的架構。對於這種架構來說,資料或服務都以 API 的形式暴露給外部系統或應用程式。在這種情況下,使用者權限的控制是非常重要的。本文主要介紹如何使用 Apache Shiro 來管理 Java API 中的權限問題。
Apache Shiro 的概述
Apache Shiro 是 Apache 軟體基金會的開源框架,用於在應用程式中提供安全認證、授權、密碼管理和會話管理等基本功能。 Apache Shiro 是一個簡單、易於使用的安全框架,使得 Java 開發者能夠專注於業務邏輯而不用擔心安全性問題。
Apache Shiro 的主要組成部分包括:
- Subject:代表一個與我們的應用程式互動的用戶,可以是人、程式、服務等。每個 Subject 都有一組安全相關的「身分」(principal),這些身分可用於表示使用者的身分。
- SecurityManager:用於管理應用程式的安全性操作。其主要職責是進行身份驗證、授權、加密等。
- Realm:用於取得應用程式的數據,例如使用者、角色、權限等等。資料可能儲存在資料庫、檔案等等。
- SessionManager:針對每個使用者管理其會話訊息,包括建立、失效、維護等。
- Session:為每個使用者維護其會話訊息,包括使用者登入狀態等。
- Cryptography:提供加密和雜湊演算法等安全服務。
基於上述組成部分,Shiro 提供了一套完整的安全框架,可以用於開發 Java 應用程式中的安全模組。
使用 Apache Shiro 進行權限控制
在開發 Java API 過程中,經常需要對使用者進行權限控制,Shiro 提供了靈活精簡的方案來實現該功能。以下介紹具體的實作方法:
- 引入 Shiro 依賴套件
#首先需要將 Shiro 的相關依賴套件加入專案。例如,在Maven 專案中,可以在pom.xml 中新增以下程式碼:
<dependency> <groupId>org.apache.shiro</groupId> <artifactId>shiro-core</artifactId> <version>1.5.3</version> </dependency>
- #定義Realm 類別
在Shiro 中,Realm 負責定義使用者、角色和權限等相關數據,並將其與Shiro 框架整合。因此,定義 Realm 類別是進行權限控制的第一步。
我們可以自訂一個Realm 類,來實現從資料庫中獲取使用者相關資訊的功能,如下所示:
public class MyRealm extends AuthorizingRealm { // 根据用户名获取用户即其角色、权限等相关信息 protected AuthorizationInfo doGetAuthorizationInfo(PrincipalCollection principalCollection) { // 获取当前用户的身份信息 String username = (String) principalCollection.getPrimaryPrincipal(); // 从数据库中查询用户及其角色 User user = userService.loadByUsername(username); List<Role> roles = roleService.getRolesByUsername(username); // 添加角色 List<String> roleNames = new ArrayList<>(); for (Role role : roles) { roleNames.add(role.getName()); } SimpleAuthorizationInfo info = new SimpleAuthorizationInfo(); info.addRoles(roleNames); // 添加权限 List<String> permissionNames = new ArrayList<>(); for (Role role : roles) { List<Permission> permissions = permissionService.getPermissionsByRoleId(role.getId()); for (Permission permission : permissions) { permissionNames.add(permission.getName()); } } info.addStringPermissions(permissionNames); return info; } // 根据用户名和密码验证用户 protected AuthenticationInfo doGetAuthenticationInfo(AuthenticationToken authenticationToken) throws AuthenticationException { // 获取用户提交的身份信息 String username = (String) authenticationToken.getPrincipal(); String password = new String((char[]) authenticationToken.getCredentials()); // 根据用户名查询用户 User user = userService.loadByUsername(username); if (user == null) { throw new UnknownAccountException(); } // 验证用户密码 String encodedPassword = hashService.hash(password, user.getSalt()); if (!user.getPassword().equals(encodedPassword)) { throw new IncorrectCredentialsException(); } // 如果验证成功,则返回一个身份信息 SimpleAuthenticationInfo info = new SimpleAuthenticationInfo(username, password, getName()); return info; } }
在上述程式碼中,我們透過實作AuthorizingRealm 抽象類別提供的doGetAuthorizationInfo 方法和doGetAuthenticationInfo 方法來獲取使用者的角色和權限信息,以及驗證使用者的身份。這些方法中都會呼叫 UserService、RoleService、PermissionService 等服務層來查詢資料庫中的相關信息,以便取得使用者資料。
- 設定 Shiro Filter
在 Java API 中,我們可以設定 Shiro Filter 對請求進行攔截,實現對使用者權限的控制。 Shiro Filter 是一個 Servlet 過濾器,可用於在 Web 應用中進行權限過濾、會話管理等。
在設定 Shiro Filter 時,我們需要設定 Shiro 的安全管理器、自訂的 Realm 類別、編寫好的登入頁面等等。範例如下所示:
@Configuration public class ShiroConfig { @Bean public ShiroFilterFactoryBean shirFilter(SecurityManager securityManager) { ShiroFilterFactoryBean shiroFilter = new ShiroFilterFactoryBean(); shiroFilter.setSecurityManager(securityManager); // 设置登录URL shiroFilter.setLoginUrl("/login"); // 设置无权访问的URL shiroFilter.setUnauthorizedUrl("/unauthorized"); // 配置拦截器规则 Map<String,String> filterChainDefinitionMap = new LinkedHashMap<>(); filterChainDefinitionMap.put("/login", "anon"); filterChainDefinitionMap.put("/logout", "logout"); filterChainDefinitionMap.put("/home", "authc"); filterChainDefinitionMap.put("/admin/**", "roles[admin]"); filterChainDefinitionMap.put("/**", "authc"); shiroFilter.setFilterChainDefinitionMap(filterChainDefinitionMap); return shiroFilter; } @Bean public SecurityManager securityManager(MyRealm myRealm) { DefaultWebSecurityManager securityManager = new DefaultWebSecurityManager(); securityManager.setRealm(myRealm); return securityManager; } @Bean public MyRealm myRealm(HashService hashService, UserService userService, RoleService roleService, PermissionService permissionService) { return new MyRealm(userService, roleService, permissionService, hashService); } @Bean public HashService hashService() { return new SimpleHashService(); } }
在上述程式碼中,我們使用 @Configuration 註解定義了一個 ShiroConfig 類,用於配置 Shiro 相關的參數。在這個類別中,我們定義了 shirFilter() 方法、securityManager() 方法以及 myRealm() 方法,用於配置 Shiro 過濾器、安全管理器以及自訂 Realm 類別。在對應的方法中,我們可以透過依賴注入的方式,使用 UserService、RoleService、PermissionService、HashService 等依賴來注入相關的服務,以實現權限控制和使用者驗證。
- 在 Java API 中使用 Shiro 進行權限控制
在完成上述步驟後,我們就可以在 Java API 中使用 Shiro 進行權限控制了。在具體實作時,我們可以使用 Shiro 提供的 Subject 類別來表示目前使用者。我們可以透過該類別的 hasRole()、isPermitted() 等方法來檢查使用者是否擁有某個角色或權限。以下是一個例子:
@Controller public class ApiController { @RequestMapping("/api/test") @ResponseBody public String test() { Subject currentUser = SecurityUtils.getSubject(); if (currentUser.hasRole("admin")) { return "Hello, admin!"; } else if (currentUser.isAuthenticated()) { return "Hello, user!"; } else { return "Please login first!"; } } }
在上述程式碼中,我們定義了一個 ApiController 類,並在其中定義了一個 test() 方法。在這個方法中,我們首先取得目前使用者 Subject 對象,然後透過呼叫 hasRole()、isPermitted() 等方法來進行權限判斷。
總結
Java API 開發中,權限控制是一個非常重要的問題。 Apache Shiro 提供了一套方便易用的安全框架,可協助 Java 開發者快速實現使用者認證、授權、密碼管理和會話管理等功能。透過本文的介紹,希望讀者能清楚了解如何在 Java API 開發中使用 Shiro 來實現權限控制的功能。
以上是Java API 開發中使用 Apache Shiro 進行權限控制的詳細內容。更多資訊請關注PHP中文網其他相關文章!

熱AI工具

Undresser.AI Undress
人工智慧驅動的應用程序,用於創建逼真的裸體照片

AI Clothes Remover
用於從照片中去除衣服的線上人工智慧工具。

Undress AI Tool
免費脫衣圖片

Clothoff.io
AI脫衣器

Video Face Swap
使用我們完全免費的人工智慧換臉工具,輕鬆在任何影片中換臉!

熱門文章

熱工具

記事本++7.3.1
好用且免費的程式碼編輯器

SublimeText3漢化版
中文版,非常好用

禪工作室 13.0.1
強大的PHP整合開發環境

Dreamweaver CS6
視覺化網頁開發工具

SublimeText3 Mac版
神級程式碼編輯軟體(SublimeText3)
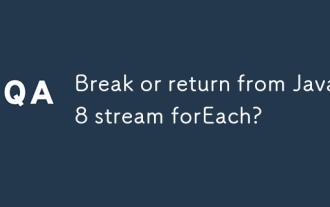
Java 8引入了Stream API,提供了一種強大且表達力豐富的處理數據集合的方式。然而,使用Stream時,一個常見問題是:如何從forEach操作中中斷或返回? 傳統循環允許提前中斷或返回,但Stream的forEach方法並不直接支持這種方式。本文將解釋原因,並探討在Stream處理系統中實現提前終止的替代方法。 延伸閱讀: Java Stream API改進 理解Stream forEach forEach方法是一個終端操作,它對Stream中的每個元素執行一個操作。它的設計意圖是處
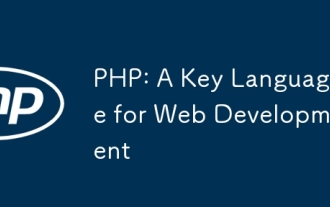
PHP是一種廣泛應用於服務器端的腳本語言,特別適合web開發。 1.PHP可以嵌入HTML,處理HTTP請求和響應,支持多種數據庫。 2.PHP用於生成動態網頁內容,處理表單數據,訪問數據庫等,具有強大的社區支持和開源資源。 3.PHP是解釋型語言,執行過程包括詞法分析、語法分析、編譯和執行。 4.PHP可以與MySQL結合用於用戶註冊系統等高級應用。 5.調試PHP時,可使用error_reporting()和var_dump()等函數。 6.優化PHP代碼可通過緩存機制、優化數據庫查詢和使用內置函數。 7
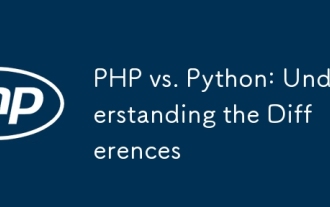
PHP和Python各有優勢,選擇應基於項目需求。 1.PHP適合web開發,語法簡單,執行效率高。 2.Python適用於數據科學和機器學習,語法簡潔,庫豐富。
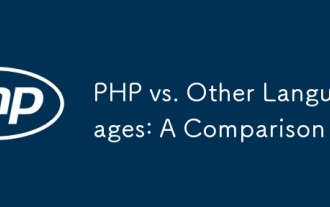
PHP適合web開發,特別是在快速開發和處理動態內容方面表現出色,但不擅長數據科學和企業級應用。與Python相比,PHP在web開發中更具優勢,但在數據科學領域不如Python;與Java相比,PHP在企業級應用中表現較差,但在web開發中更靈活;與JavaScript相比,PHP在後端開發中更簡潔,但在前端開發中不如JavaScript。
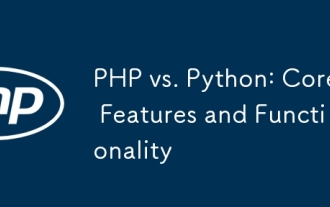
PHP和Python各有優勢,適合不同場景。 1.PHP適用於web開發,提供內置web服務器和豐富函數庫。 2.Python適合數據科學和機器學習,語法簡潔且有強大標準庫。選擇時應根據項目需求決定。
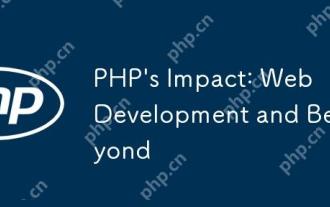
PHPhassignificantlyimpactedwebdevelopmentandextendsbeyondit.1)ItpowersmajorplatformslikeWordPressandexcelsindatabaseinteractions.2)PHP'sadaptabilityallowsittoscaleforlargeapplicationsusingframeworkslikeLaravel.3)Beyondweb,PHPisusedincommand-linescrip
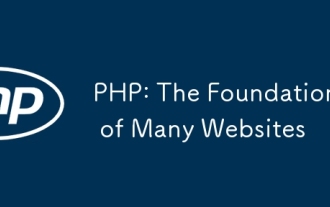
PHP成為許多網站首選技術棧的原因包括其易用性、強大社區支持和廣泛應用。 1)易於學習和使用,適合初學者。 2)擁有龐大的開發者社區,資源豐富。 3)廣泛應用於WordPress、Drupal等平台。 4)與Web服務器緊密集成,簡化開發部署。
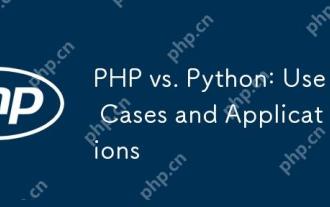
PHP適用於Web開發和內容管理系統,Python適合數據科學、機器學習和自動化腳本。 1.PHP在構建快速、可擴展的網站和應用程序方面表現出色,常用於WordPress等CMS。 2.Python在數據科學和機器學習領域表現卓越,擁有豐富的庫如NumPy和TensorFlow。
